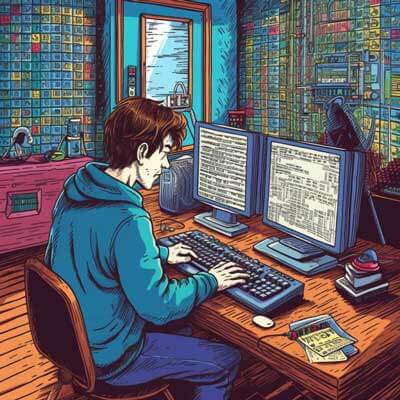
- Event Listeners
- DOM Manipulation
- User Interactions
- Interactive Elements
- JavaScript Frameworks
- Front-end Development
- Web Applications
- Interactivity
- User Interface
- Dynamic Content
- Popular JavaScript Frameworks for Interactive Components
- Adding Event Listeners for Interactivity
- DOM Manipulation for Interactive Elements
- Common User Interactions with JavaScript
- Creating Dynamic User Interfaces with JavaScript
- Best Practices for Front-end Development of Interactive Components
- Examples of Interactive Elements in Web Applications
- Handling User Input and Interactive Behavior with JavaScript
- Adding Interactivity to Static HTML Content with JavaScript
- Tools and Libraries for Creating Interactive Components in JavaScript
- Additional Resources
Event Listeners
Event listeners are an essential part of constructing interactive elements with JavaScript. They allow you to listen for specific events, such as user actions or system events, and execute a function in response. This enables you to create dynamic and interactive web pages.
Here’s an example of adding an event listener to a button element:
const button = document.querySelector('button'); button.addEventListener('click', () => { console.log('Button clicked!'); });
In this example, we select a button element using the querySelector
method and then attach an event listener to it using the addEventListener
method. The event we are listening for is the 'click'
event, and the function we provide as the second argument will be executed when the button is clicked.
You can add event listeners to various elements and listen for different types of events, such as 'mouseover'
, 'keydown'
, or 'submit'
. This allows you to create interactive elements that respond to user actions in real-time.
Related Article: Accessing Parent State from Child Components in Next.js
DOM Manipulation
DOM (Document Object Model) manipulation is another crucial aspect of constructing interactive elements with JavaScript. The DOM represents the structure of an HTML document, and by manipulating it, you can dynamically update the content, style, and structure of a web page.
Here’s an example of manipulating the text content of an HTML element:
const heading = document.querySelector('h1'); heading.textContent = 'New Heading';
In this example, we select an h1
element using the querySelector
method and then update its textContent
property to 'New Heading'
. This will change the text displayed within the h1
element to the new value.
You can also manipulate other properties of HTML elements, such as innerHTML
, classList
, or style
, to modify their content, apply CSS styles, or add/remove classes dynamically. This allows you to create interactive elements that change their appearance or behavior based on user interactions.
User Interactions
User interactions play a vital role in creating interactive elements with JavaScript. By capturing and responding to user actions, you can create engaging and dynamic web experiences.
Here’s an example of handling a button click event and changing the background color of a div element:
const button = document.querySelector('button'); const div = document.querySelector('div'); button.addEventListener('click', () => { div.style.backgroundColor = 'blue'; });
In this example, we select a button and a div element using the querySelector
method. When the button is clicked, we change the background color of the div element to 'blue'
by updating its style.backgroundColor
property.
You can capture and respond to other user actions, such as mouse movements, keyboard input, or form submissions, to create interactive elements that provide feedback or trigger specific behaviors.
Interactive Elements
Interactive elements are HTML elements that allow user interactions, such as buttons, forms, sliders, or menus. By combining event listeners, DOM manipulation, and user interactions, you can create interactive elements that respond to user actions and provide dynamic functionality.
Here’s an example of creating a button element dynamically and adding an event listener to it:
const button = document.createElement('button'); button.textContent = 'Click me'; button.addEventListener('click', () => { console.log('Button clicked!'); }); document.body.appendChild(button);
In this example, we create a button element using the createElement
method and set its text content to 'Click me'
. Then, we add an event listener to the button and log a message to the console when it is clicked. Finally, we append the button to the body
element to make it visible on the web page.
Related Article: How to Integrate Redux with Next.js
JavaScript Frameworks
JavaScript frameworks provide a set of tools, libraries, and guidelines for building interactive web applications. They simplify the process of creating interactive elements by abstracting away low-level details and providing high-level abstractions and reusable components.
Some popular JavaScript frameworks for creating interactive components include React, Angular, and Vue.js.
React example:
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button>Increment</button> </div> ); }
In this example, we use React’s useState
hook to manage the state of a counter. The count
state variable represents the current count value, and the setCount
function allows us to update it. When the button is clicked, the increment
function is called, which updates the count state by incrementing it. The updated count value is then rendered in the paragraph element.
Angular example:
import { Component } from '@angular/core'; @Component({ selector: 'app-counter', template: ` <div> <p>Count: {{ count }}</p> <button>Increment</button> </div> `, }) export class CounterComponent { count = 0; increment() { this.count++; } }
In this example, we define an Angular component called CounterComponent
with a count property and an increment method. The count value is displayed in the paragraph element using interpolation, and the increment method is bound to the button’s click event using Angular’s event binding syntax.
Vue.js example:
<div> <p>Count: {{ count }}</p> <button>Increment</button> </div> export default { data() { return { count: 0, }; }, methods: { increment() { this.count++; }, }, };
In this example, we define a Vue.js component with a count data property and an increment method. The count value is displayed using template interpolation, and the increment method is bound to the button’s click event using Vue.js’s event handling syntax.
These examples demonstrate how JavaScript frameworks simplify the creation of interactive components by providing abstractions for managing state, handling events, and rendering dynamic content.
Front-end Development
Front-end development involves building the user interface and user experience of a web application. It focuses on creating interactive elements, designing visually appealing layouts, and optimizing performance.
To develop interactive components, front-end developers use HTML, CSS, and JavaScript to create the structure, style, and behavior of web pages. They leverage event listeners, DOM manipulation, and user interactions to create dynamic and engaging user experiences.
Front-end development also involves optimizing web applications for different devices and browsers, ensuring accessibility and usability, and following best practices for performance and security.
Web Applications
Web applications are software applications that run on web browsers and utilize web technologies, such as HTML, CSS, and JavaScript, to provide interactive functionality and deliver content to users over the internet.
Web applications can range from simple single-page applications (SPAs) to complex enterprise applications. They can be used for various purposes, such as e-commerce, social networking, productivity tools, or content management systems.
Related Article: Next.js Bundlers Quick Intro
Interactivity
Interactivity refers to the ability of a system, application, or user interface to respond to user actions and provide feedback or perform specific actions in real-time. It is a key aspect of creating engaging and interactive user experiences.
JavaScript plays a crucial role in adding interactivity to web applications. By capturing and responding to user interactions, such as clicks, mouse movements, or keyboard input, JavaScript enables developers to create dynamic and interactive elements that respond to user actions.
Interactivity can range from simple actions like changing the color of a button on hover to complex functionality like form validation, real-time updates, or interactive data visualizations. It enhances user engagement, improves usability, and makes web applications more intuitive and enjoyable to use.
User Interface
The user interface (UI) is the visual representation of a web application and the means by which users interact with it. It encompasses the layout, design, and interactive elements of a web page.
JavaScript is commonly used to enhance and manipulate the user interface of web applications. By adding event listeners, manipulating the DOM, and responding to user interactions, JavaScript enables developers to create dynamic and interactive UI components.
UI design principles, such as consistency, simplicity, and responsiveness, play a crucial role in creating user-friendly and intuitive interfaces. Front-end developers use HTML, CSS, and JavaScript to implement UI designs and create visually appealing and interactive web pages.
Dynamic Content
Dynamic content refers to the ability of web applications to update and display data in real-time without requiring a full page reload. It allows for a more interactive and engaging user experience by providing up-to-date information and responding to user actions.
JavaScript is widely used to create dynamic content in web applications. By utilizing AJAX (Asynchronous JavaScript and XML) or newer technologies like Fetch API or WebSockets, developers can retrieve data from servers, update the DOM dynamically, and provide real-time updates to users.
Here’s an example of fetching data from an API and dynamically updating the content of a web page:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { const element = document.getElementById('dynamic-content'); element.textContent = data.message; }) .catch(error => { console.error('Error:', error); });
In this example, we use the Fetch API to make a GET request to an API endpoint and retrieve data in JSON format. We then update the content of an element with the id 'dynamic-content'
by setting its textContent
property to the value of the 'message'
property in the response data.
Dynamic content allows web applications to provide personalized experiences, display real-time data, and create interactive elements that adapt to user actions and input.
Related Article: Integrating React Router with Next.js in JavaScript
Popular JavaScript Frameworks for Interactive Components
Several JavaScript frameworks are popular for creating interactive components in web applications. These frameworks provide a wide range of features, tools, and libraries that simplify the process of building interactive elements and enhance developer productivity.
Here are some popular JavaScript frameworks for interactive components:
1. React: React is a JavaScript library for building user interfaces. It provides a component-based architecture, virtual DOM, and a declarative syntax that allows developers to create reusable and interactive UI components.
2. Angular: Angular is a full-featured framework for building web applications. It provides a useful template system, two-way data binding, dependency injection, and a wide range of built-in features for creating interactive components.
3. Vue.js: Vue.js is a progressive JavaScript framework that focuses on simplicity and flexibility. It allows developers to build interactive components using a combination of HTML templates, JavaScript, and CSS. Vue.js provides reactivity, component composition, and a rich ecosystem of plugins and libraries.
These frameworks offer various features for managing state, handling events, manipulating the DOM, and creating dynamic user interfaces. They have extensive documentation, active communities, and large ecosystems of third-party libraries and tools.
Adding Event Listeners for Interactivity
Adding event listeners is a fundamental step in creating interactive components with JavaScript. Event listeners allow you to capture user actions or system events and execute custom code in response.
To add an event listener, you need to select the target element and specify the event you want to listen for. Here’s an example of adding an event listener to a button element:
const button = document.querySelector('button'); button.addEventListener('click', () => { console.log('Button clicked!'); });
In this example, we use the querySelector
method to select a button element, and then we add an event listener to it using the addEventListener
method. The event we are listening for is the 'click'
event, and the function we provide as the second argument will be executed when the button is clicked.
You can add event listeners to various elements and listen for different types of events, such as 'mouseover'
, 'keydown'
, or 'submit'
. This allows you to create interactive components that respond to user actions in real-time.
DOM Manipulation for Interactive Elements
DOM (Document Object Model) manipulation is a key technique for creating interactive elements with JavaScript. It allows you to modify the structure, content, or style of HTML elements dynamically, enabling you to create dynamic and responsive user interfaces.
Here’s an example of manipulating the text content of an HTML element:
const heading = document.querySelector('h1'); heading.textContent = 'New Heading';
In this example, we use the querySelector
method to select an h1
element, and then we update its textContent
property to 'New Heading'
. This will change the text displayed within the h1
element to the new value.
You can also manipulate other properties of HTML elements, such as innerHTML
, classList
, or style
, to modify their content, apply CSS styles, or add/remove classes dynamically. This allows you to create interactive elements that change their appearance or behavior based on user interactions.
DOM manipulation is a useful technique for creating interactive components, but it’s important to use it judiciously and follow best practices to ensure performance, maintainability, and accessibility.
Related Article: How to Create a Navbar in Next.js
Common User Interactions with JavaScript
JavaScript enables you to capture and respond to various user interactions, allowing you to create interactive components that respond to user actions in real-time. Here are some common user interactions that can be handled with JavaScript:
1. Button clicks: You can add event listeners to buttons and execute custom code when they are clicked. This allows you to perform actions like submitting a form, navigating to a different page, or triggering an animation.
2. Mouse movements: You can listen for mouse events, such as 'mouseover'
or 'mousemove'
, and respond by updating the UI, displaying tooltips, or triggering interactive animations.
3. Keyboard input: You can capture keyboard events, such as 'keydown'
or 'keyup'
, to enable keyboard navigation, implement shortcuts, or validate form input in real-time.
4. Form submissions: You can intercept form submissions using event listeners and prevent the default behavior to perform custom validation, submit the form asynchronously, or update the UI based on the form data.
5. Drag and drop: You can listen for drag and drop events and implement custom functionality, such as reordering items, uploading files, or creating interactive visualizations.
These are just a few examples of the many user interactions that can be handled with JavaScript. By capturing and responding to user actions, you can create engaging and interactive web experiences.
Creating Dynamic User Interfaces with JavaScript
JavaScript allows you to create dynamic user interfaces by manipulating the DOM, responding to user interactions, and updating the UI in real-time. This enables you to create interactive components that adapt to user actions and provide a rich and engaging user experience.
Here’s an example of creating a dynamic user interface that updates based on user input:
const input = document.querySelector('input'); const output = document.querySelector('p'); input.addEventListener('input', () => { output.textContent = input.value; });
In this example, we use the querySelector
method to select an input element and a paragraph element. We then add an event listener to the input element, listening for the 'input'
event, which is triggered whenever the input value changes. In the event handler, we update the text content of the paragraph element to reflect the current value of the input element.
Best Practices for Front-end Development of Interactive Components
When developing interactive components, it’s important to follow best practices to ensure maintainable, performant, and accessible code. Here are some best practices for front-end development of interactive components:
1. Use a modular and component-based architecture: Break your code into reusable and independent components to promote code reusability, maintainability, and scalability.
2. Separate concerns: Use the separation of concerns principle to keep your HTML, CSS, and JavaScript code separate and maintain a clear structure.
3. Optimize performance: Minimize the number of DOM manipulations, avoid unnecessary re-renders, and optimize your code for performance. Use tools like code bundlers, minifiers, and compression techniques to reduce file sizes and improve loading times.
4. Follow accessibility guidelines: Ensure your interactive components are accessible to users with disabilities. Use semantic HTML, provide appropriate ARIA attributes, and test your components with assistive technologies.
5. Test thoroughly: Write unit tests and integration tests to ensure the correctness and reliability of your interactive components. Use testing frameworks and tools to automate testing and catch potential bugs early in the development process.
6. Document your code: Document your code, including API references, usage examples, and explanations of complex functionality. This will make it easier for other developers to understand and use your interactive components.
Related Article: Server-side rendering (SSR) Basics in Next.js
Examples of Interactive Elements in Web Applications
Web applications often include various interactive elements that allow users to perform actions, provide input, or receive feedback. Here are some examples of interactive elements commonly found in web applications:
1. Buttons: Buttons are interactive elements that allow users to trigger actions, such as submitting a form, navigating to a different page, or performing an operation.
2. Forms: Forms enable users to provide input, such as text, numbers, or selections, and submit it to the server for processing. Form validation, input masking, and autocomplete are common interactive features in forms.
3. Sliders: Sliders allow users to select a value within a range by dragging a handle along a track. They are often used for selecting values like volume, brightness, or price ranges.
4. Menus: Menus provide a list of options that users can select from. Dropdown menus, context menus, and navigation menus are examples of interactive menus.
5. Accordions: Accordions are collapsible sections that allow users to expand or collapse content by clicking on a header. They are commonly used to display FAQs, expandable sections, or tabbed interfaces.
6. Modals: Modals are overlay windows that appear on top of the main content and require user interaction to dismiss or take action. They are often used for displaying alerts, confirmation dialogs, or displaying additional information.
These are just a few examples of interactive elements commonly used in web applications. The choice of interactive elements depends on the specific requirements and functionalities of the application.
Handling User Input and Interactive Behavior with JavaScript
JavaScript is commonly used to handle user input and implement interactive behavior in web applications. By capturing user actions, such as clicks, keystrokes, or form submissions, and responding to them, you can create dynamic and interactive elements.
Here’s an example of handling user input and implementing interactive behavior with JavaScript:
const button = document.querySelector('button'); const input = document.querySelector('input'); const output = document.querySelector('p'); button.addEventListener('click', () => { const inputValue = input.value; output.textContent = `Hello, ${inputValue}!`; });
In this example, we select a button element, an input element, and a paragraph element using the querySelector
method. When the button is clicked, we retrieve the value of the input element and update the text content of the paragraph element to display a personalized greeting.
Adding Interactivity to Static HTML Content with JavaScript
JavaScript allows you to add interactivity to static HTML content by manipulating the DOM, capturing user interactions, and updating the UI dynamically. This enables you to enhance the user experience and create dynamic elements even in static web pages.
Here’s an example of adding interactivity to static HTML content with JavaScript:
<title>Adding Interactivity</title> .highlight { background-color: yellow; } <p>This is a static paragraph.</p> const paragraph = document.querySelector('p'); paragraph.addEventListener('click', () => { paragraph.classList.toggle('highlight'); });
In this example, we have a static HTML paragraph element. We use JavaScript to add an event listener to the paragraph element, listening for the 'click'
event. When the paragraph is clicked, we toggle the 'highlight'
class on it, changing its background color to yellow.
Related Article: Is Next.js a Frontend or a Backend Framework?
Tools and Libraries for Creating Interactive Components in JavaScript
There are numerous tools and libraries available for creating interactive components in JavaScript. These tools and libraries provide abstractions, utilities, and pre-built components that simplify the process of building interactive elements and enhance developer productivity.
Here are some popular tools and libraries for creating interactive components in JavaScript:
1. jQuery: jQuery is a fast, small, and feature-rich JavaScript library. It simplifies HTML document traversal, event handling, and DOM manipulation, making it easier to create interactive components.
2. D3.js: D3.js is a useful library for creating data visualizations with JavaScript. It provides a set of reusable charting components and utilities for manipulating, animating, and interacting with data.
3. Lodash: Lodash is a utility library that provides a wide range of helper functions for working with arrays, objects, and other data structures. It simplifies common programming tasks and enhances the productivity of JavaScript developers.
4. Bootstrap: Bootstrap is a popular CSS framework that includes a set of pre-built components, styles, and utilities for creating responsive and interactive web applications. It provides a solid foundation for building interactive components quickly.
5. Material-UI: Material-UI is a React component library that implements the Material Design guidelines. It provides a set of beautiful and interactive UI components that can be easily customized and integrated into React applications.
These are just a few examples of the many tools and libraries available for creating interactive components in JavaScript. The choice of tools and libraries depends on the specific requirements and technologies used in your project.
Additional Resources
– Using Event Listeners in JavaScript to Create Interactive Components
– The Role of DOM Manipulation in Creating Interactive Components