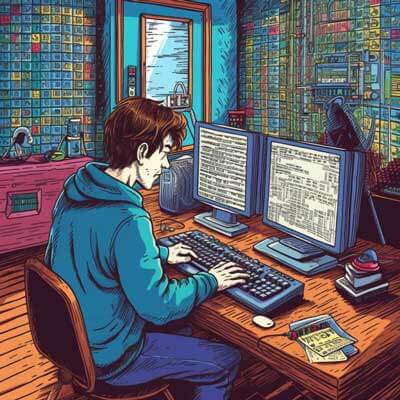
Converting a number to a string in JavaScript can be achieved in several ways. In this answer, we will explore two common methods: using the toString()
method and using the String()
function.
Using the toString() Method
The toString()
method is available on all JavaScript number objects and allows you to convert a number to its corresponding string representation. Here’s how you can use it:
const number = 42; const stringNumber = number.toString(); console.log(stringNumber); // Output: "42"
In this example, we have a number 42
, and by calling the toString()
method on it, we get the string representation "42"
.
It’s important to note that the toString()
method can also take an optional radix parameter, which specifies the base to use for representing the number as a string. The radix can be between 2 and 36. Here’s an example:
const number = 42; const binaryString = number.toString(2); console.log(binaryString); // Output: "101010"
In this example, we convert the number 42
to its binary representation by passing 2
as the radix to the toString()
method.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Using the String() Function
Another way to convert a number to a string in JavaScript is by using the String()
function. The String()
function can be used with or without the new
keyword. Here’s how you can use it:
const number = 42; const stringNumber = String(number); console.log(stringNumber); // Output: "42"
In this example, we pass the number 42
to the String()
function, and it returns the string representation "42"
.
Just like with the toString()
method, the String()
function also accepts an optional radix parameter. Here’s an example:
const number = 42; const binaryString = String(number, 2); console.log(binaryString); // Output: "101010"
In this example, we convert the number 42
to its binary representation by passing 2
as the radix to the String()
function.
Best Practices
When converting a number to a string in JavaScript, it’s important to consider potential edge cases and best practices. Here are a few tips:
– Be aware of the potential loss of precision when converting large numbers to strings. JavaScript has a maximum safe integer value, which is Number.MAX_SAFE_INTEGER
. If you need to handle larger numbers, consider using libraries like BigInt
or Decimal.js
.
– When converting numbers with decimal places, be mindful of the desired level of precision. JavaScript’s built-in number type (Number
) uses floating-point arithmetic, which can introduce rounding errors. If precision is critical, consider using libraries like Decimal.js
or Big.js
that provide more accurate decimal arithmetic.
– If you’re working with numbers that have leading zeros, such as postal codes or phone numbers, converting them to strings directly may remove the leading zeros. In such cases, you can use the padStart()
function to add leading zeros if needed. Here’s an example:
const number = 42; const paddedString = number.toString().padStart(5, '0'); console.log(paddedString); // Output: "00042"
In this example, we convert the number 42
to a string and use padStart()
to add leading zeros to make it a five-digit string.
These are just a few considerations to keep in mind when converting numbers to strings in JavaScript. The choice of method (toString()
or String()
) and any additional handling depends on the specific requirements of your application.
Alternative Approaches
In addition to the toString()
method and the String()
function, there are other alternative approaches for converting numbers to strings in JavaScript. Here are a few:
– Using template literals: Template literals, denoted by backticks (), allow you to embed expressions within strings. You can use template literals to convert numbers to strings by simply enclosing the number within
${}. Here's an example:
<pre>{{EJS5}}</pre>
- Concatenating with an empty string: Another simple way to convert a number to a string is by concatenating it with an empty string (
”). JavaScript automatically coerces the number into a string when it's concatenated with a string. Here's an example:
<pre>{{EJS6}}</pre>
Both of these alternative approaches work effectively for converting numbers to strings in JavaScript. However, they may not provide the same level of control and flexibility as the
() method or the
()` function.
Related Article: How to Use the forEach Loop with JavaScript