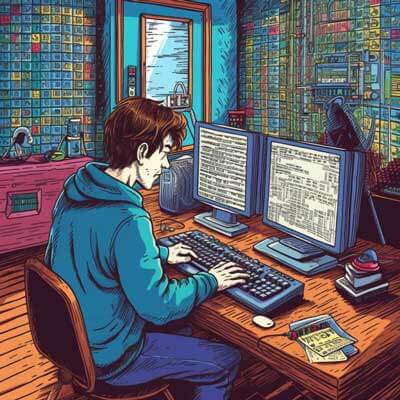
There are several ways to access the index in Python for loops. In this guide, we will explore these methods and discuss their use cases.
Why is this question asked?
When working with loops in Python, it is often necessary to access the index of each iteration. This can be useful for various reasons, such as updating specific elements in a list or performing calculations based on the index value. Additionally, understanding how to access the index can help improve the efficiency and readability of your code.
Related Article: How To Limit Floats To Two Decimal Points In Python
Potential reasons for accessing the index in Python for loops:
1. Modifying elements of a list: In certain cases, you may need to modify specific elements of a list during each iteration of a loop. By accessing the index, you can easily target the desired element and update its value.
2. Performing calculations or operations based on the index: There might be situations where you need to perform calculations or operations that depend on the current index value. Accessing the index allows you to incorporate this information into your logic.
Methods to access the index in Python for loops:
Method 1: Using the enumerate()
function
The enumerate()
function is a built-in Python function that allows you to iterate over a sequence while also keeping track of the index of each element. It returns an iterator that yields pairs of the form (index, element)
.
Here’s an example that demonstrates how to use enumerate()
to access the index in a Python for loop:
fruits = ['apple', 'banana', 'orange'] for index, fruit in enumerate(fruits): print(f"Index: {index}, Fruit: {fruit}")
Output:
Index: 0, Fruit: apple Index: 1, Fruit: banana Index: 2, Fruit: orange
In the above example, the enumerate()
function is used to iterate over the list of fruits. The index
variable stores the index of each element, while the fruit
variable stores the corresponding fruit. The values are then printed to the console.
Method 2: Using the range()
function with the length of the sequence
Another way to access the index in Python for loops is by using the range()
function with the length of the sequence. This method allows you to generate a sequence of numbers that correspond to the indices of the elements in the sequence.
Here’s an example that demonstrates how to use range()
to access the index in a Python for loop:
fruits = ['apple', 'banana', 'orange'] for index in range(len(fruits)): fruit = fruits[index] print(f"Index: {index}, Fruit: {fruit}")
Output:
Index: 0, Fruit: apple Index: 1, Fruit: banana Index: 2, Fruit: orange
In the above example, the range()
function is used to generate a sequence of numbers from 0 to the length of the list minus one. The index
variable iterates over this sequence, and the corresponding fruit is accessed using the index.
Best practices and suggestions:
– Prefer using enumerate()
over range()
: The enumerate()
function provides a more concise and readable way to access the index in Python for loops. It eliminates the need for an additional line of code to access the element using the index.
– Use descriptive variable names: When accessing the index in a for loop, it is a good practice to use a meaningful variable name that indicates the purpose of the index. This can enhance the readability of your code and make it easier to understand.
– Avoid modifying the list while iterating: Modifying the list while iterating over it can lead to unexpected behavior and errors. If you need to modify the elements of a list, consider creating a copy of the list or using a different approach such as list comprehension.
– Consider using a for loop with a counter: In some cases, you may not need to access the index directly. Instead, you can use a counter variable to keep track of the iteration number. This can be useful when the index itself is not required, but you still need to perform certain actions based on the iteration number.
Related Article: How To Rename A File With Python