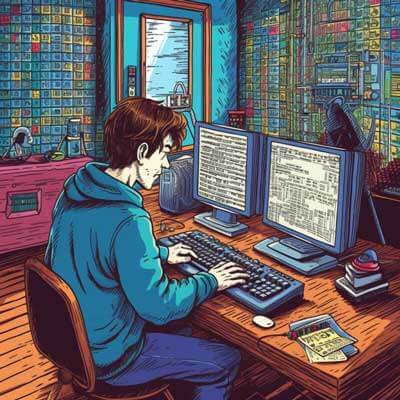
Table of Contents
Formatting dates is a common task in JavaScript, and Moment.js is a popular library that makes it easier to work with dates and times. Moment.js provides a simple and intuitive API for parsing, manipulating, and displaying dates and times. In this answer, we will explore how to format dates using Moment.js in JavaScript.
Step 1: Installing Moment.js
To start using Moment.js, you need to include it in your project. There are a few ways to install Moment.js, but the most common method is through npm, the Node.js package manager. You can install Moment.js by running the following command in your project directory:
npm install moment
Alternatively, you can include Moment.js directly in your HTML file by adding the following script tag:
Related Article: How To Sum An Array Of Numbers In Javascript
Step 2: Importing and Using Moment.js
Once you have installed Moment.js, you can import it into your JavaScript file using the require
or import
statement:
const moment = require('moment'); // or import moment from 'moment';
With Moment.js imported, you can now use its functions to format dates.
Step 3: Formatting Dates
Moment.js provides the format
function to format dates. This function takes a formatting string as an argument and returns the formatted date string.
Here are some common formatting tokens that you can use in the formatting string:
- YYYY
: 4-digit year
- YY
: 2-digit year
- MM
: 2-digit month (01-12)
- DD
: 2-digit day of the month (01-31)
- HH
: 2-digit hour (00-23)
- mm
: 2-digit minute (00-59)
- ss
: 2-digit second (00-59)
For example, to format the current date as "YYYY-MM-DD", you can use the following code:
const formattedDate = moment().format('YYYY-MM-DD'); console.log(formattedDate); // Output: 2022-01-01
You can also pass a specific date to the moment
function to format that date:
const date = moment('2021-12-25'); const formattedDate = date.format('dddd, MMMM Do YYYY'); console.log(formattedDate); // Output: Saturday, December 25th 2021
Step 4: Localizing Date Formats
Moment.js provides built-in support for localizing date formats. You can set the locale using the locale
function and then format the date accordingly.
Here's an example of formatting a date in French:
moment.locale('fr'); const formattedDate = moment().format('LL'); console.log(formattedDate); // Output: 1er janvier 2022
You can find a list of available locales in the Moment.js documentation.
Related Article: Sharing State Between Two Components in React JavaScript
Step 5: Additional Formatting Options
In addition to the basic formatting tokens, Moment.js provides many other options for formatting dates. Here are a few examples:
- Do
: Day of the month with ordinal suffix (1st, 2nd, 3rd, etc.)
- ddd
: Abbreviated weekday name (Sun, Mon, Tue, etc.)
- MMMM
: Full month name (January, February, March, etc.)
- h
: Hour (1-12)
- A
: Uppercase AM or PM
You can find a comprehensive list of formatting tokens in the Moment.js documentation.
Step 6: Best Practices
When formatting dates with Moment.js, it's important to follow some best practices to ensure consistent and reliable results:
- Use the appropriate formatting tokens for the desired output. Moment.js provides a wide range of formatting options, so choose the ones that match your requirements.
- Consider the locale when formatting dates. Moment.js supports localization, allowing you to display dates in different languages and formats.
- Test your date formats thoroughly to ensure they work as expected in different scenarios, such as different time zones or leap years.