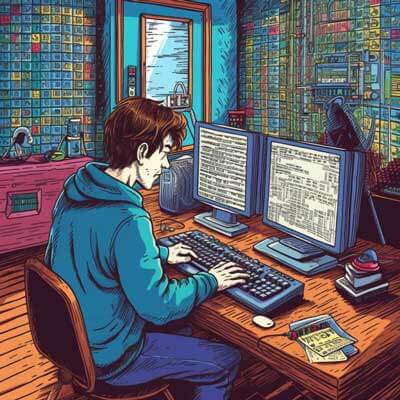
To sum an array of numbers in Javascript, you can use various approaches. In this answer, we will explore two common methods for achieving this task.
Method 1: Using a for loop
One way to sum an array of numbers in Javascript is by using a for loop. This method involves iterating over each element of the array and adding it to a running total. Here’s an example:
function sumArray(arr) { var total = 0; for (var i = 0; i < arr.length; i++) { total += arr[i]; } return total; } var numbers = [1, 2, 3, 4, 5]; var result = sumArray(numbers); console.log(result); // Output: 15
In the above code, we define a function called sumArray
that takes an array arr
as its parameter. We initialize a variable total
to 0, which will store the sum of the array elements. The for loop iterates over each element of the array using the index i
, and adds the value of each element to the total
variable. Finally, the function returns the calculated sum.
Related Article: How To Merge Arrays And Remove Duplicates In Javascript
Method 2: Using the reduce method
Another approach to summing an array of numbers in Javascript is by using the reduce
method. The reduce
method applies a function to each element in the array, resulting in a single value. Here’s an example:
function sumArray(arr) { var total = arr.reduce(function(acc, curr) { return acc + curr; }, 0); return total; } var numbers = [1, 2, 3, 4, 5]; var result = sumArray(numbers); console.log(result); // Output: 15
In the above code, we define the sumArray
function that takes an array arr
as its parameter. Inside the function, we use the reduce
method on the array arr
. The reduce
method takes a callback function as its parameter, which receives two arguments: an accumulator (acc
) and the current element (curr
). In each iteration, the callback function adds the current element to the accumulator. We initialize the accumulator to 0 by passing it as the second argument to the reduce
method. Finally, the function returns the calculated sum.
Why would you need to sum an array of numbers?
Summing an array of numbers is a common task in programming. There are various reasons why you might need to perform this operation. Some potential scenarios include:
– Calculating the total revenue or sales from an array of transactions.
– Computing the average of a set of values by dividing the sum by the number of elements.
– Finding the highest or lowest value in an array by comparing each element to the current maximum or minimum.
Being able to sum an array of numbers efficiently is a fundamental skill for many programming tasks.
Suggestions and alternative ideas
While the above methods are commonly used for summing an array of numbers in Javascript, there are alternative approaches you can consider:
– Using the forEach
method: Instead of a for loop or the reduce
method, you can also use the forEach
method, which executes a provided function once for each array element. Within the callback function, you can update a running total variable. However, this approach may not be as concise as the other methods.
function sumArray(arr) { var total = 0; arr.forEach(function(num) { total += num; }); return total; }
– Using the eval
function: Although not recommended for security reasons, you can use the eval
function to evaluate a string expression containing the sum of the array elements. This approach can be useful if you have an array of numbers represented as strings.
function sumArray(arr) { var sum = eval(arr.join("+")); return sum; }
However, it’s important to note that using eval
can introduce security vulnerabilities, especially if the array elements are not properly validated.
Related Article: JavaScript HashMap: A Complete Guide
Best practices
When summing an array of numbers in Javascript, it’s good to keep the following best practices in mind:
– Initialize the sum variable: Before iterating over the array, initialize the variable that will hold the sum to an appropriate initial value. For numbers, the initial value is typically 0.
– Use a for loop or the reduce
method: Both the for loop and the reduce
method are efficient and widely understood ways to sum an array of numbers. Choose the method that suits your coding style and requirements.
– Consider handling edge cases: If the array is empty or contains non-numeric values, you may want to handle those cases appropriately. For example, you could return 0 for an empty array or skip non-numeric values during the summing process.
– Avoid modifying the original array: Unless necessary, avoid modifying the original array while summing its elements. This helps maintain data integrity and prevents unexpected behavior in other parts of your code.