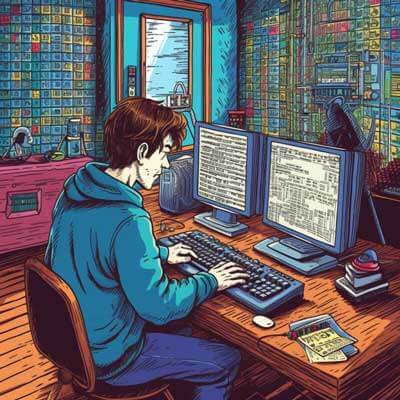
Table of Contents
Tecursion is a useful technique that allows a function to call itself repeatedly until a specific condition is met. It is a fundamental concept in many programming languages, including Java. In this article, we will dive into the data structure that controls recursion in Java and explore various aspects of recursion in Java programming.
How does recursion work in Java?
Recursion in Java involves a method calling itself within its own body. This self-referential behavior allows for the repetition of a certain task until a base case is reached. When a method is called recursively, each subsequent call creates a new instance of the method on the call stack, forming a recursive stack frame.
Let's take a look at a simple example to understand how recursion works in Java. Consider a factorial function that calculates the factorial of a given number:
public class Factorial { public static int factorial(int n) { if (n == 0) { return 1; } else { return n * factorial(n - 1); } } public static void main(String[] args) { int result = factorial(5); System.out.println("Factorial of 5 is: " + result); } }
In this example, the factorial
method calls itself with a smaller value of n
until n
becomes 0. This is the base case, where the recursion stops. The method then returns the factorial of the number.
Related Article: Java Inheritance Tutorial
What is the difference between a recursive case and a base case?
In recursive algorithms, there are two essential components: the recursive case and the base case.
The recursive case is the part of the algorithm that calls itself with a smaller or simpler input. It helps break down the problem into smaller subproblems until the base case is reached. In the factorial example above, the recursive case is n * factorial(n - 1)
, where factorial(n - 1)
is the recursive call.
The base case, on the other hand, is the stopping condition for the recursion. It defines when the recursion should stop and provides the result for the simplest case. In the factorial example, the base case is if (n == 0)
, where the method returns 1 when n
is 0.
The combination of the recursive case and the base case ensures that the recursion terminates and produces the desired result.
What is the base case in recursive algorithms?
The base case is the condition that determines when recursion should stop. It provides the result for the simplest case of the problem. In recursive algorithms, the base case acts as the termination condition and prevents infinite recursion.
In the factorial example mentioned earlier, the base case is if (n == 0)
, where the method returns 1 when n
is 0. This base case ensures that the recursion stops when the input reaches 0 and prevents the factorial method from calling itself indefinitely.
Having a well-defined base case is crucial in recursive algorithms to avoid infinite recursion and ensure the correctness of the algorithm.
How are method calls handled in recursive functions?
In recursive functions, method calls are handled by creating new instances of the method on the call stack. Each recursive call adds a new stack frame to the call stack, allowing the function to maintain separate instances of variables and parameters for each recursive call.
When a method is called recursively, the current state of the method (including variables and parameters) is saved on the stack. The new instance of the method is then executed with the updated values, and the process repeats until the base case is reached.
Once the base case is reached, the method returns its result, and the stack frames are popped off the call stack one by one, allowing the program to resume execution from where it left off.
It's important to note that recursive functions can consume a significant amount of memory if the recursion depth is large. Therefore, it's important to optimize recursive algorithms and ensure that the base case is reached within a reasonable number of recursive calls.
Related Article: How to Use the JsonProperty in Java
What are some common examples of recursive algorithms in Java?
Recursive algorithms can be found in various areas of Java programming. Here are some common examples of recursive algorithms:
1. Factorial: As shown in the earlier example, the factorial algorithm calculates the product of all positive integers up to a given number.
public static int factorial(int n) { if (n == 0) { return 1; } else { return n * factorial(n - 1); } }
2. Fibonacci series: The Fibonacci series is a sequence of numbers where each number is the sum of the two preceding ones. The recursive Fibonacci algorithm calculates the nth Fibonacci number.
public static int fibonacci(int n) { if (n <= 1) { return n; } else { return fibonacci(n - 1) + fibonacci(n - 2); } }
3. Binary search: The binary search algorithm searches for a specific element in a sorted array by repeatedly dividing the search space in half. It can be implemented using recursion.
public static int binarySearch(int[] arr, int target, int left, int right) { if (right >= left) { int mid = left + (right - left) / 2; if (arr[mid] == target) { return mid; } else if (arr[mid] > target) { return binarySearch(arr, target, left, mid - 1); } else { return binarySearch(arr, target, mid + 1, right); } } return -1; }
These are just a few examples of recursive algorithms in Java. Recursive algorithms provide an elegant and concise solution to a wide range of problems.
What is a tail recursion in Java?
A tail recursion is a special case of recursion where the recursive call is the last operation performed in a method. In other words, the recursive call is in the "tail position" of the method.
Tail recursion is significant because it allows the Java compiler to optimize the recursive function by converting it into an iterative loop. This optimization eliminates the need to create new stack frames for each recursive call, resulting in improved performance and reduced memory consumption.
To illustrate the concept of tail recursion, let's consider an example of calculating the factorial of a number using tail recursion:
public class Factorial { public static int factorial(int n, int result) { if (n == 0) { return result; } else { return factorial(n - 1, n * result); } } public static void main(String[] args) { int result = factorial(5, 1); System.out.println("Factorial of 5 is: " + result); } }
In this tail-recursive implementation, the factorial
method takes an additional parameter result
to track the intermediate result. The recursive call return factorial(n - 1, n * result)
is the last operation performed in the method, making it a tail recursion.
Tail recursion is a useful technique to improve the efficiency of recursive algorithms, especially when dealing with large input sizes.
How does a stack control recursion in Java?
In Java, the call stack is a data structure that controls the flow of execution in recursive functions. It keeps track of the sequence of method calls and their corresponding stack frames.
Each time a method is called, a new stack frame is created and pushed onto the call stack. The stack frame contains information about the method's local variables, parameters, and the return address.
When a method finishes its execution, its stack frame is popped off the call stack, and the program resumes execution from where it left off.
In the context of recursion, the call stack plays a crucial role in controlling the flow of recursive calls. Each recursive call creates a new stack frame, allowing the function to maintain separate instances of variables and parameters for each recursive call.
As the recursion progresses, the stack grows in size, and each recursive call is added on top of the previous calls. When the base case is reached, the stack starts to unwind as the stack frames are popped off one by one until the initial call is reached.
It's important to note that the call stack has a finite size, and a large number of recursive calls can lead to a stack overflow error. Therefore, it's crucial to optimize recursive algorithms and ensure that the recursion depth is within a reasonable limit.
How can a recursive data structure be implemented in Java?
A recursive data structure is a data structure that contains references to other instances of the same type. It allows for the creation of complex hierarchical structures by reusing the same structure at different levels.
In Java, recursive data structures can be implemented using classes and references. Let's consider an example of a recursive data structure: a binary tree.
public class TreeNode { private int value; private TreeNode left; private TreeNode right; // Constructors, getters, and setters // Other methods }
In this example, the TreeNode
class represents a node in a binary tree. Each TreeNode
object contains a value and references to its left and right child nodes. By using references to other TreeNode
objects, a binary tree can be constructed recursively.
To create a binary tree, we can use the following code snippet:
TreeNode root = new TreeNode(1); root.setLeft(new TreeNode(2)); root.setRight(new TreeNode(3));
In this example, we create a root node with a value of 1 and attach two child nodes with values 2 and 3, respectively. Each child node can further have its own child nodes, forming a recursive structure.
Recursive data structures are useful tools for representing complex hierarchical relationships, such as trees, graphs, and linked lists.
Related Article: How to Print a Hashmap in Java
What are the benefits of using recursion in Java?
Recursion offers several benefits in Java programming:
1. Concise and readable code: Recursive algorithms often provide a more elegant and concise solution to problems that involve repetitive tasks. The recursive code is often easier to read and understand, especially for problems that have a natural recursive structure.
2. Simplified problem-solving: Recursive algorithms allow programmers to break down complex problems into simpler subproblems. By solving the subproblems recursively, the overall problem becomes more manageable and easier to solve.
3. Code reusability: Recursive algorithms can be written in a generic way to solve a wide range of problems. Once a recursive function is implemented, it can be reused for different inputs and variations of the problem.
4. Handling hierarchical structures: Recursive algorithms are particularly useful for handling hierarchical data structures, such as trees, graphs, and linked lists. The recursive nature of these structures can be naturally represented and processed using recursive algorithms.
However, it's important to note that recursion is not always the best solution for every problem. It can be less efficient in terms of memory usage and execution time compared to iterative approaches. Therefore, it's essential to consider the specific problem and its requirements before choosing recursion as the solution.
How does a tree structure relate to recursion in Java?
Tree structures and recursion have a close relationship in Java programming. Trees are hierarchical data structures that consist of nodes connected by edges. Each node in a tree can have zero or more child nodes, forming a recursive structure.
Recursion is a natural fit for processing and manipulating tree structures. The recursive nature of trees allows for elegant and concise algorithms that can traverse, search, insert, and delete nodes in a tree.
Let's consider an example of a binary search tree (BST) and its recursive operations:
public class BinarySearchTree { private TreeNode root; // Constructors, getters, and setters public boolean search(int value) { return searchRecursive(root, value); } private boolean searchRecursive(TreeNode node, int value) { if (node == null) { return false; } if (value == node.getValue()) { return true; } else if (value < node.getValue()) { return searchRecursive(node.getLeft(), value); } else { return searchRecursive(node.getRight(), value); } } // Other methods }
In this example, the BinarySearchTree
class represents a binary search tree. The search
method performs a recursive search operation on the tree by calling the private searchRecursive
method.
The searchRecursive
method takes a TreeNode
object and a value to search for. It checks if the current node is null (base case) and returns false. If the value matches the value of the current node, it returns true. Otherwise, it recursively calls itself on the left or right child node, depending on the value being searched.
This recursive approach allows for an efficient search operation on a binary search tree. Similar recursive algorithms can be implemented for other tree operations, such as insertion, deletion, and traversal.
Code Snippet: Implementing recursion in Java
public class RecursionExample { public static void recursiveMethod(int n) { if (n > 0) { System.out.println("Number: " + n); recursiveMethod(n - 1); } } public static void main(String[] args) { recursiveMethod(5); } }
In this code snippet, the recursiveMethod
is a simple recursive method that prints the value of n
and calls itself with n - 1
until n
becomes 0. The base case is when n
is no longer greater than 0, and the recursion stops.
When the main
method calls recursiveMethod
with an initial value of 5, the output will be:
Number: 5 Number: 4 Number: 3 Number: 2 Number: 1
This example demonstrates the basic concept of recursion in Java.
Code Snippet: Writing a recursive algorithm in Java
public class RecursiveAlgorithm { public static int recursiveSum(int[] arr, int index) { if (index < 0) { return 0; } return arr[index] + recursiveSum(arr, index - 1); } public static void main(String[] args) { int[] numbers = {1, 2, 3, 4, 5}; int sum = recursiveSum(numbers, numbers.length - 1); System.out.println("Sum of the array: " + sum); } }
In this code snippet, the recursiveSum
method calculates the sum of an array of integers using recursion. It takes an array and an index as parameters. If the index is less than 0 (base case), it returns 0. Otherwise, it adds the value at the current index to the sum of the remaining elements obtained through recursive calls.
When the main
method calls recursiveSum
with an array [1, 2, 3, 4, 5]
, the output will be:
Sum of the array: 15
This example demonstrates how recursion can be used to solve problems that involve repetitive calculations.
Related Article: Proper Placement of MySQL Connector JAR File in Java
Code Snippet: Implementing a recursive data structure in Java
public class LinkedListNode { private int value; private LinkedListNode next; // Constructors, getters, and setters // Other methods }
In this code snippet, the LinkedListNode
class represents a node in a linked list. Each LinkedListNode
object contains a value and a reference to the next node in the list. By using references to other LinkedListNode
objects, a linked list can be constructed recursively.
To create a linked list, we can use the following code snippet:
LinkedListNode first = new LinkedListNode(1); LinkedListNode second = new LinkedListNode(2); LinkedListNode third = new LinkedListNode(3); first.setNext(second); second.setNext(third);
In this example, we create three nodes with values 1, 2, and 3, respectively. Each node maintains a reference to the next node, forming a linked list.
Recursive data structures, such as linked lists, allow for the representation of dynamic and flexible data structures that can grow or shrink as needed.
Code Snippet: Implementing tail recursion in Java
public class TailRecursionExample { public static int tailRecursiveSum(int[] arr, int index, int sum) { if (index < 0) { return sum; } return tailRecursiveSum(arr, index - 1, sum + arr[index]); } public static void main(String[] args) { int[] numbers = {1, 2, 3, 4, 5}; int sum = tailRecursiveSum(numbers, numbers.length - 1, 0); System.out.println("Sum of the array: " + sum); } }
In this code snippet, the tailRecursiveSum
method calculates the sum of an array of integers using tail recursion. It takes an array, an index, and a sum as parameters. If the index is less than 0 (base case), it returns the sum. Otherwise, it adds the value at the current index to the sum and passes the updated sum to the next recursive call.
When the main
method calls tailRecursiveSum
with an array [1, 2, 3, 4, 5]
, the output will be:
Sum of the array: 15
This example demonstrates how tail recursion can be used to optimize recursive algorithms by converting them into iterative loops.
Case Study: Applying recursion in a real-world Java project
Let's consider a real-world example of applying recursion in a Java project. Suppose we are building a file system explorer that needs to traverse a directory tree and print the names of all files and directories within a given directory.
import java.io.File; public class FileSystemExplorer { public static void exploreDirectory(File directory) { if (directory.isDirectory()) { System.out.println("Directory: " + directory.getAbsolutePath()); File[] files = directory.listFiles(); if (files != null) { for (File file : files) { exploreDirectory(file); } } } else { System.out.println("File: " + directory.getAbsolutePath()); } } public static void main(String[] args) { File rootDirectory = new File("path/to/directory"); exploreDirectory(rootDirectory); } }
In this example, the exploreDirectory
method recursively explores a directory and its subdirectories using recursion. If the current file is a directory, it prints the directory name and recursively calls exploreDirectory
on each file within the directory. If the current file is a regular file, it prints the file name.
This case study demonstrates how recursion can be applied to solve real-world problems, such as traversing and processing complex data structures.
Best practices for using recursion in Java
When using recursion in Java, it's important to follow some best practices to ensure the correctness and efficiency of the algorithm:
1. Define a clear base case: Every recursive algorithm should have a well-defined base case that determines when the recursion should stop. Without a base case, the recursion can lead to infinite loops.
2. Handle edge cases: Consider edge cases, such as empty input or minimum input size, and handle them explicitly. This ensures that the algorithm handles all possible scenarios correctly.
3. Optimize tail recursion: Whenever possible, try to write tail-recursive algorithms to allow for potential compiler optimizations. Tail recursion can improve performance and reduce memory consumption.
4. Avoid unnecessary recursion: Carefully analyze the problem and consider alternative approaches before choosing recursion. In some cases, iterative or other algorithmic techniques may be more efficient or easier to implement.
5. Optimize recursive algorithms: Recursive algorithms can be optimized by using memoization, dynamic programming, or other techniques to avoid redundant calculations or duplicate work.
6. Test and debug carefully: Recursive algorithms can be more challenging to test and debug compared to iterative algorithms. It's important to thoroughly test the algorithm with different input sizes and edge cases to ensure its correctness.
Related Article: How to Use Spring Restcontroller in Java
Common mistakes to avoid when using recursion in Java
While recursion is a useful technique, it can also lead to common mistakes if not used correctly. Here are some common mistakes to avoid when using recursion in Java:
1. Infinite recursion: Forgetting to include a base case or incorrectly defining the stopping condition can result in infinite recursion. This can lead to stack overflow errors and crash the program.
2. Stack overflow: Recursive algorithms that have a large recursion depth can cause stack overflow errors. It's important to optimize the algorithm and limit the recursion depth to prevent such errors.
3. Redundant calculations: Recursive algorithms that perform redundant calculations can be inefficient. Avoid repeating calculations by using memoization or dynamic programming techniques.
4. Incorrect base case: Defining an incorrect base case can lead to incorrect results or unexpected behavior. Carefully consider the problem and define the base case correctly.
5. Incorrect state management: Recursive algorithms require proper management of state variables and parameters. Incorrect state management can lead to incorrect results or infinite recursion.
6. Lack of testing: Recursive algorithms can be challenging to test due to their recursive nature. Failing to thoroughly test the algorithm with different input sizes and edge cases can result in incorrect behavior.
Exploring the efficiency of recursive algorithms in Java
The efficiency of recursive algorithms in Java depends on various factors, including the problem complexity, recursion depth, and the algorithm's design.
Recursive algorithms have several advantages, such as simplicity and code readability. However, they can also have certain drawbacks in terms of efficiency:
1. Memory usage: Recursive algorithms can consume a significant amount of memory due to the creation of multiple stack frames on the call stack. Large recursion depth can lead to stack overflow errors.
2. Time complexity: Recursive algorithms may have higher time complexity compared to iterative algorithms. Each recursive call incurs additional function call overhead and can result in redundant calculations.
3. Redundant calculations: Recursive algorithms can perform redundant calculations, especially when solving dynamic programming problems. Redundant calculations can be avoided using memoization or other optimization techniques.
It's important to analyze the problem and consider the specific requirements before choosing recursion as the solution. In some cases, iterative or other algorithmic techniques may provide better performance and efficiency.
If you decide to use recursion, it's crucial to optimize the algorithm and ensure that the recursion depth is within a reasonable limit. Techniques such as tail recursion, memoization, and dynamic programming can be applied to improve the efficiency of recursive algorithms.
Comparing recursion with other programming paradigms in Java
Recursion is a programming paradigm that offers an elegant and concise way to solve certain types of problems. However, it's not the only programming paradigm available in Java. Let's compare recursion with other programming paradigms commonly used in Java:
1. Iteration: Iteration is a fundamental programming paradigm that involves using loops to repeat a set of instructions until a specific condition is met. Iteration is often more efficient than recursion in terms of memory usage and execution time. It's particularly suitable for problems that involve repetitive calculations or looping through data structures.
2. Object-oriented programming (OOP): OOP is a programming paradigm that focuses on organizing code into objects that encapsulate data and behavior. OOP is well-suited for modeling real-world entities and building complex systems. While recursion can be used in an object-oriented context, it's not inherently tied to OOP principles.
3. Functional programming (FP): FP is a programming paradigm that emphasizes immutability, pure functions, and higher-order functions. Recursion is a natural fit for functional programming due to its ability to express complex computations in a concise and declarative manner. Functional programming languages, such as Haskell, heavily rely on recursion to solve problems.
4. Procedural programming: Procedural programming is a programming paradigm that focuses on a sequence of instructions and procedures. Recursion can be used in procedural programming, but it's not as common as in functional programming. Procedural programming languages, such as C, often rely on iterative constructs like loops for repetitive tasks.
Each programming paradigm has its strengths and weaknesses, and the choice of paradigm depends on the problem domain, requirements, and personal preferences. Recursion is a useful tool in the programmer's toolbox, but it's important to understand its limitations and consider alternative approaches when appropriate.
Understanding the limitations of recursion in Java
While recursion is a useful technique, it has certain limitations that programmers should be aware of:
1. Memory consumption: Recursive algorithms can consume a significant amount of memory due to the creation of multiple stack frames on the call stack. Large recursion depth can lead to stack overflow errors. It's important to optimize recursive algorithms and ensure that the recursion depth is within a reasonable limit.
2. Performance: Recursive algorithms may have higher time complexity compared to iterative algorithms due to additional function call overhead. Redundant calculations can also impact performance. It's crucial to analyze the problem and consider alternative approaches before choosing recursion.
3. Stack overflow errors: Recursive algorithms that have a large recursion depth can cause stack overflow errors, which occur when the call stack exceeds its maximum size. Stack overflow errors can crash the program and should be avoided by optimizing the algorithm and limiting the recursion depth.
4. Code complexity: Recursive code can be more challenging to understand and debug compared to iterative code. Recursive algorithms require a clear understanding of the base case, recursive case, and how the method calls are handled. Proper testing and careful debugging are crucial to ensure the correctness of recursive algorithms.
5. Limited applicability: Recursion is not suitable for all types of problems. Some problems are better solved using iterative or other algorithmic techniques. It's important to consider the problem requirements and constraints before choosing recursion as the solution.
Related Article: How to Declare and Initialize a New Array in Java
Additional Resources
- Recursion in Java