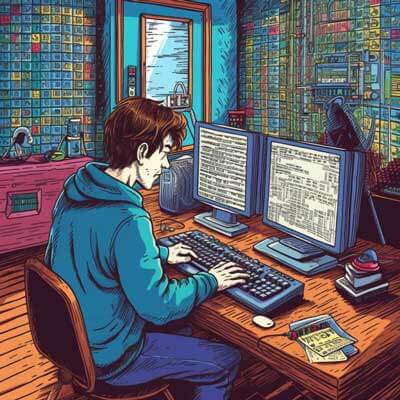
- What is GraphQL Playground?
- How to use query variables in GraphQL Playground
- Benefits of using GraphQL
- Programming languages compatible with GraphQL
- Passing query variables in a GraphQL query
- Using GraphQL Playground with other programming languages
- Best practices for using query variables in GraphQL
- Limitations of using query variables in GraphQL
- Alternatives to GraphQL Playground
- Common use cases for GraphQL
- Additional Resources
What is GraphQL Playground?
GraphQL Playground is an interactive IDE (Integrated Development Environment) that allows developers to explore and interact with GraphQL APIs. It provides a user-friendly interface for testing queries, mutations, and subscriptions, making it easier to understand and work with GraphQL.
Related Article: Managing Data Queries with GraphQL Count
How to use query variables in GraphQL Playground
Query variables in GraphQL Playground are used to pass dynamic values to your GraphQL queries. Instead of hardcoding values directly into your query, you can use variables to make your queries more reusable and flexible.
To use query variables in GraphQL Playground, you need to follow these steps:
1. Define your query variables at the top of the Playground interface using the $
symbol followed by the variable name and its type. For example:
query ($id: ID!) { user(id: $id) { name email } }
2. Provide the values for your query variables in the “Query Variables” pane on the bottom left of the Playground interface. For example:
{ "id": "123" }
3. Use the query variables in your GraphQL query by referencing them using the same syntax as defined in step 1. For example:
query ($id: ID!) { user(id: $id) { name email } }
Benefits of using GraphQL
GraphQL offers several benefits over traditional REST APIs:
1. Efficient data fetching: With GraphQL, clients can specify exactly what data they need and receive only that data, reducing the amount of unnecessary data transfer and improving performance.
2. Flexible queries: GraphQL allows clients to request multiple resources in a single request and specify nested data structures, avoiding the problem of over-fetching and under-fetching data.
3. Strong typing and introspection: GraphQL schemas provide a strong type system and allow clients to introspect the available fields, types, and relationships, making it easier to understand and work with the API.
4. Rapid development: GraphQL simplifies the process of building APIs by providing a clear and declarative syntax for defining schemas and resolving data, allowing developers to iterate quickly and deliver features faster.
5. Versioning and deprecation: GraphQL provides built-in versioning and deprecation mechanisms, allowing APIs to evolve over time without breaking existing clients.
Programming languages compatible with GraphQL
GraphQL is a language-agnostic query language, which means it can be used with any programming language that supports HTTP requests and JSON serialization. Some of the popular programming languages and frameworks that have GraphQL implementations or libraries include:
– JavaScript (Node.js, React, Vue.js)
– Python (Django, Flask)
– Ruby (Ruby on Rails)
– Java (Spring Boot)
– PHP (Laravel, Symfony)
– .NET (ASP.NET Core)
– Go
– Swift (iOS)
– Kotlin (Android)
These implementations provide tools and utilities for working with GraphQL, making it easier to integrate GraphQL into your existing projects.
Related Article: Step by Step Process: Passing Enum in GraphQL Query
Passing query variables in a GraphQL query
To pass query variables in a GraphQL query, you need to define the variables in your query and provide their values when making the request.
Here’s an example of a GraphQL query with query variables:
query GetPost($id: ID!) { post(id: $id) { title author } }
In this example, $id
is a query variable of type ID
. To pass the value for the id
variable, you can include the variables
field in your GraphQL request payload:
{ "query": "query GetPost($id: ID!) { post(id: $id) { title author } }", "variables": { "id": "123" } }
Using GraphQL Playground with other programming languages
GraphQL Playground is a versatile tool that can be used with any programming language that supports GraphQL. Regardless of the programming language you are using, you can interact with GraphQL Playground by sending HTTP requests to the GraphQL server.
Here’s an example of how to use GraphQL Playground with JavaScript and Node.js:
1. Install the necessary dependencies:
npm install graphql graphql-playground-middleware-express express
2. Create an Express server and configure it to use GraphQL Playground:
const express = require('express'); const { graphqlHTTP } = require('express-graphql'); const { buildSchema } = require('graphql'); const { playground } = require('graphql-playground-middleware-express'); const schema = buildSchema(` type Query { hello: String } `); const root = { hello: () => 'Hello, World!', }; const app = express(); app.use('/graphql', graphqlHTTP({ schema, rootValue: root })); app.get('/playground', playground({ endpoint: '/graphql' })); app.listen(3000, () => { console.log('Server running on http://localhost:3000'); });
3. Start the server:
node server.js
Now, you can access GraphQL Playground by visiting http://localhost:3000/playground in your browser and interact with the GraphQL API.
Best practices for using query variables in GraphQL
When using query variables in GraphQL, there are some best practices you can follow to improve the readability and maintainability of your code:
1. Use descriptive variable names: Use meaningful names for your query variables that accurately reflect their purpose and usage.
2. Define variable types: Specify the types of your query variables to ensure type safety and prevent unexpected errors. This also helps with documentation and understanding the expected input.
3. Provide default values: To make your queries more flexible, consider providing default values for optional query variables. This allows you to reuse the same query with different default values when needed.
4. Validate user input: Always validate and sanitize user input to prevent security vulnerabilities like SQL injection or cross-site scripting (XSS) attacks.
5. Test with different variable values: Test your queries with different variable values to ensure they handle edge cases and unexpected input correctly.
Related Article: Achieving Production-Ready GraphQL
Limitations of using query variables in GraphQL
While query variables provide many benefits in GraphQL, there are some limitations to be aware of:
1. Limited support for complex variables: GraphQL query variables are typically limited to scalar types like strings, numbers, booleans, and enums. Support for more complex types like arrays or objects may vary depending on the GraphQL implementation or library you are using.
2. Lack of variable validation: GraphQL query variables do not provide built-in validation mechanisms. It’s up to the server implementation to validate and sanitize the input values to prevent security vulnerabilities.
3. Performance impact: Using query variables can have a slight performance impact compared to hardcoding values directly in the query. This is because the server needs to parse and process the variables separately from the query itself.
Despite these limitations, query variables are still a useful tool in GraphQL that can greatly improve the flexibility and reusability of your queries.
Alternatives to GraphQL Playground
While GraphQL Playground is a popular and widely used tool for exploring and testing GraphQL APIs, there are also alternative tools available that provide similar functionality. Some of these alternatives include:
– GraphiQL: GraphiQL is another popular GraphQL IDE that provides a similar interface to GraphQL Playground. It offers features like auto-completion, syntax highlighting, and interactive documentation.
– Insomnia: Insomnia is a general-purpose REST and GraphQL client that supports query variables and provides a user-friendly interface for testing and debugging GraphQL APIs.
– Postman: Postman is a widely used API development and testing tool that supports GraphQL. It allows you to send GraphQL queries, mutations, and subscriptions, and provides advanced features like environment variables and collections.
These alternatives offer different features and interfaces, so you can choose the one that best fits your needs and preferences.
Common use cases for GraphQL
GraphQL is a versatile technology that can be used in various scenarios, including:
1. Building APIs: GraphQL is commonly used to build APIs that provide flexible and efficient data fetching capabilities. It allows clients to retrieve exactly the data they need and enables developers to iterate quickly and deliver features faster.
2. Mobile applications: GraphQL’s ability to request only the required data makes it a good fit for mobile applications, where network bandwidth and data consumption are important considerations.
3. Microservices architecture: GraphQL can be used as a communication layer between microservices, allowing each microservice to expose its own GraphQL schema and enabling clients to retrieve data from multiple services in a single request.
4. Real-time applications: GraphQL supports subscriptions, which allow clients to subscribe to real-time data updates. This makes it a suitable choice for building real-time applications like chat apps or dashboards.
5. Legacy system integration: GraphQL can be used to integrate with existing legacy systems by providing a layer of abstraction and allowing clients to interact with the legacy system using a modern and flexible API.
Related Article: Implementing TypeORM with GraphQL and NestJS
Additional Resources
– Query Variables in GraphQL
– How does GraphQL work
– Role of Schema in GraphQL