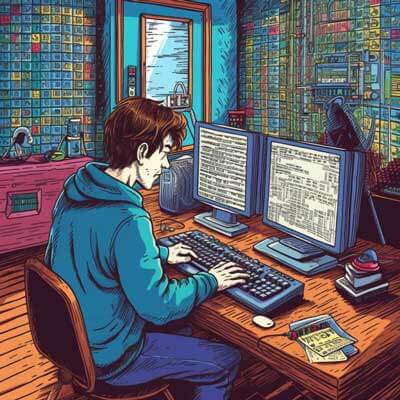
To find all Mat Icons in Angular, you can follow these steps:
Step 1: Importing the MatIconModule
First, you need to make sure that you have imported the MatIconModule in your Angular project. The MatIconModule is part of the Angular Material package and provides a collection of Material Design icons. To import the MatIconModule, you can add the following line of code to your module file (e.g., app.module.ts):
import { MatIconModule } from '@angular/material/icon'; @NgModule({ ... imports: [ ... MatIconModule, ... ], ... }) export class AppModule { }
Related Article: How to Compare Arrays in Javascript
Step 2: Using the MatIcons
Once you have imported the MatIconModule, you can start using the Material Design icons in your Angular templates. The MatIconModule provides a wide range of icons that you can use in your application. To use a MatIcon, you can add the following code to your template file (e.g., app.component.html):
favorite
The “favorite” icon in the above example will render the heart-shaped favorite icon from the Material Design icon collection. You can replace “favorite” with the name of any other MatIcon to display a different icon.
Step 3: Finding All Mat Icons
To find a complete list of all available Mat Icons, you can visit the official Angular Material documentation. The documentation provides a comprehensive list of all the icons along with their names and codes.
You can find the list of Mat Icons in the Angular Material documentation by navigating to the following URL:
https://material.angular.io/components/icon/overview#icon-sets
In the documentation, you will find a section titled “Icon Sets” which lists different categories of icons. Each category contains a list of icons along with their names and codes. You can use the names provided in the documentation to display the desired Mat Icons in your application.
Step 4: Filtering Mat Icons
If you have a specific requirement and want to filter the Mat Icons based on certain criteria, you can use the search functionality provided in the Angular Material documentation. The search functionality allows you to search for icons based on keywords or names.
To use the search functionality, you can enter a keyword or the name of the desired icon in the search bar located at the top of the Angular Material documentation page. The search results will display icons that match your search criteria, making it easier for you to find the icons you need.
Related Article: How to Create a Countdown Timer with Javascript
Step 5: Best Practices
When using Mat Icons in your Angular application, it is important to follow some best practices to ensure a consistent and efficient development process. Here are a few best practices to consider:
1. Use icons that are relevant to the content and purpose of your application. Avoid using icons unnecessarily or using icons that may confuse users.
2. Organize your icons based on their context or usage. You can create separate files or folders to store icons that are used in specific parts of your application.
3. Consider using icon font libraries like Material Icons or Font Awesome instead of individual SVG files. Icon font libraries provide a wide range of icons and offer scalability and flexibility.
4. Optimize your icon usage by utilizing techniques like lazy loading or asynchronous loading. This can improve the performance of your application, especially if you have a large number of icons.
5. Keep your icon library up to date. Regularly check for updates and new additions to the Mat Icons collection to ensure that you have access to the latest icons and features.
Alternative Ideas
While the MatIconModule provides a rich collection of Material Design icons, you may also consider using other icon libraries or custom icons in your Angular application. Some popular alternatives to Mat Icons include:
1. Font Awesome: Font Awesome is a widely used icon library that offers a vast collection of icons. It provides scalable vector icons that can be easily customized and styled.
2. SVG Icons: You can also create or use custom SVG icons in your Angular application. SVG icons are vector-based and offer flexibility in terms of size and styling.
3. Icon Fonts: Icon fonts, like Font Awesome, provide a scalable and customizable solution for using icons in your application. They are easy to implement and offer a wide range of icons.
Consider exploring these alternatives based on your specific requirements and preferences.