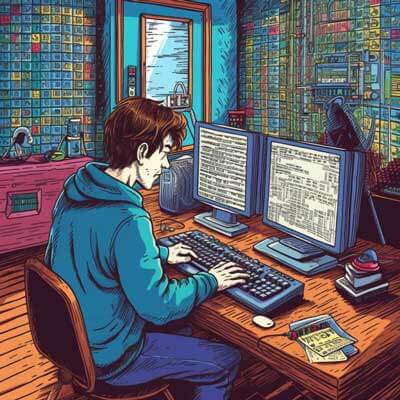
In Javascript, there are several ways to check if a variable is a string. Here are two possible approaches:
Approach 1: Using the typeof Operator
One way to check if a variable is a string in Javascript is by using the typeof
operator. The typeof
operator returns a string that indicates the type of the operand.
var variable = "Hello, World!"; if (typeof variable === 'string') { console.log("The variable is a string."); } else { console.log("The variable is not a string."); }
In this example, we use the typeof
operator to check if the variable variable
is a string. If the typeof
operator returns the string 'string'
, it means that the variable is a string.
Related Article: Accessing Parent State from Child Components in Next.js
Approach 2: Using the instanceof Operator
Another way to check if a variable is a string in Javascript is by using the instanceof
operator. The instanceof
operator tests whether an object has in its prototype chain the prototype property of a constructor.
var variable = "Hello, World!"; if (variable instanceof String) { console.log("The variable is a string."); } else { console.log("The variable is not a string."); }
In this example, we use the instanceof
operator to check if the variable variable
is an instance of the String
object. If the instanceof
operator returns true
, it means that the variable is a string.
Best Practices
When checking if a variable is a string in Javascript, it is important to consider the following best practices:
1. Use the typeof
operator when checking primitive string values. The typeof
operator works well for checking variables that contain primitive string values.
2. Use the instanceof
operator when checking string objects. The instanceof
operator is more suitable for checking variables that contain instances of the String
object.
3. Be aware of the difference between primitive string values and string objects. In Javascript, a primitive string is a sequence of characters enclosed in quotes, while a string object is an instance of the String
object.
4. Consider using regular expressions for more complex string checks. Regular expressions provide a useful way to check if a string matches a specific pattern.
Alternative Ideas
Apart from the typeof
and instanceof
operators, there are other alternative approaches to check if a variable is a string in Javascript:
1. Using the String.prototype.constructor
property:
var variable = "Hello, World!"; if (variable.constructor === String) { console.log("The variable is a string."); } else { console.log("The variable is not a string."); }
2. Using regular expressions:
var variable = "Hello, World!"; if (/^[\x00-\x7F]*$/.test(variable)) { console.log("The variable is a string."); } else { console.log("The variable is not a string."); }
Both of these alternative approaches can be effective in checking if a variable is a string in Javascript.
Related Article: How to Apply Ngstyle Conditions in Angular