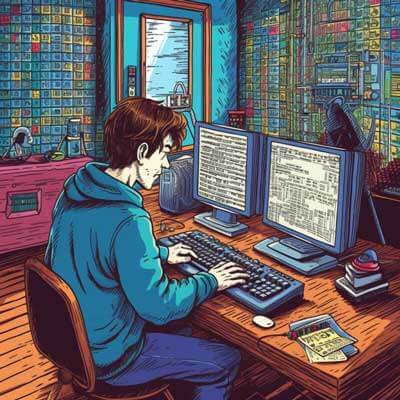
Table of Contents
Querying Methods in MySQL
MySQL is one of the most popular relational database management systems (RDBMS) used by developers around the world. It provides various querying methods to retrieve and manipulate data stored in MySQL databases. In this section, we will explore some of the commonly used querying methods in MySQL.
1. SELECT Statement:
The SELECT statement is the fundamental querying method in MySQL. It allows you to retrieve data from one or more database tables based on specific conditions. Here's an example:
SELECT column1, column2 FROM table WHERE condition;
2. INSERT Statement:
The INSERT statement is used to insert new rows into a database table. It allows you to specify the values for each column in the table. Here's an example:
INSERT INTO table (column1, column2) VALUES (value1, value2);
3. UPDATE Statement:
The UPDATE statement is used to modify existing rows in a database table. It allows you to update specific columns based on certain conditions. Here's an example:
UPDATE table SET column1 = value1, column2 = value2 WHERE condition;
4. DELETE Statement:
The DELETE statement is used to delete one or more rows from a database table. It allows you to specify the conditions for deleting rows. Here's an example:
DELETE FROM table WHERE condition;
5. JOIN Statement:
The JOIN statement allows you to combine rows from two or more tables based on a related column between them. It is useful for retrieving data from multiple tables simultaneously. Here's an example:
SELECT column1, column2 FROM table1 JOIN table2 ON table1.column = table2.column;
Related Article: Preventing Locking Queries in Read-Only PostgreSQL Databases
Querying Methods in PostgreSQL
PostgreSQL is another popular open-source relational database management system known for its robustness and advanced features. It provides a wide range of useful querying methods to manipulate and retrieve data. Let's explore some of these querying methods in PostgreSQL.
1. SELECT Statement:
Like MySQL, the SELECT statement is the primary querying method in PostgreSQL. It allows you to retrieve data from one or more tables based on specific conditions. Here's an example:
SELECT column1, column2 FROM table WHERE condition;
2. INSERT Statement:
The INSERT statement in PostgreSQL is used to insert new rows into a table. It allows you to specify the values for each column in the table. Here's an example:
INSERT INTO table (column1, column2) VALUES (value1, value2);
3. UPDATE Statement:
The UPDATE statement is used to modify existing rows in a table. It allows you to update specific columns based on certain conditions. Here's an example:
UPDATE table SET column1 = value1, column2 = value2 WHERE condition;
4. DELETE Statement:
The DELETE statement in PostgreSQL is used to delete one or more rows from a table. It allows you to specify the conditions for deleting rows. Here's an example:
DELETE FROM table WHERE condition;
5. JOIN Statement:
The JOIN statement in PostgreSQL allows you to combine rows from two or more tables based on a related column between them. It is useful for retrieving data from multiple tables simultaneously. Here's an example:
SELECT column1, column2 FROM table1 JOIN table2 ON table1.column = table2.column;
Syntax Differences in MySQL and PostgreSQL
While MySQL and PostgreSQL share many similarities in terms of querying methods, there are some syntax differences between the two. These syntax differences need to be considered when writing queries for MySQL or PostgreSQL databases. Let's explore some of the key syntax differences.
1. String Concatenation:
In MySQL, string concatenation is done using the CONCAT()
function. Here's an example:
SELECT CONCAT(first_name, ' ', last_name) AS full_name FROM table;
In PostgreSQL, string concatenation is done using the ||
operator. Here's an example:
SELECT first_name || ' ' || last_name AS full_name FROM table;
2. Case Sensitivity:
MySQL is case-insensitive by default, which means that it treats column and table names as case-insensitive. However, PostgreSQL is case-sensitive, which means that it treats column and table names as case-sensitive unless they are quoted. Here's an example:
-- MySQL SELECT column1 FROM table; -- PostgreSQL SELECT "column1" FROM "table";
3. Date and Time Functions:
MySQL and PostgreSQL have some differences in their date and time functions. For example, in MySQL, you can use the DATE_ADD()
function to add a specific time interval to a date. Here's an example:
SELECT DATE_ADD(date_column, INTERVAL 1 DAY) AS next_day FROM table;
In PostgreSQL, you can use the interval
keyword to achieve the same result. Here's an example:
SELECT date_column + INTERVAL '1 DAY' AS next_day FROM table;
4. Limiting the Number of Rows:
In MySQL, you can use the LIMIT
clause to limit the number of rows returned by a query. Here's an example:
SELECT column1 FROM table LIMIT 10;
In PostgreSQL, you can use the LIMIT
clause as well, but you also have the option to use the FETCH FIRST
clause. Here's an example:
SELECT column1 FROM table FETCH FIRST 10 ROWS ONLY;
These are just a few examples of syntax differences between MySQL and PostgreSQL. It's important to consult the official documentation for each database to understand the specific syntax and features available.
Performance Comparison: MySQL vs PostgreSQL
When it comes to performance, MySQL and PostgreSQL have their own strengths and weaknesses. The performance of a database system depends on various factors such as the hardware, the workload, the database schema, and the query optimization techniques used. Let's compare the performance of MySQL and PostgreSQL in different scenarios.
1. Read Performance:
In general, MySQL is known for its excellent read performance. It is optimized for handling read-heavy workloads and can handle a large number of concurrent read requests efficiently. MySQL achieves this by using various caching mechanisms, such as the query cache and the InnoDB buffer pool. These caching mechanisms help reduce disk I/O and improve response times for read requests.
PostgreSQL also has good read performance, but it may not be as efficient as MySQL in certain scenarios. PostgreSQL uses a different caching mechanism called the shared buffer cache, which stores recently accessed data in memory. While this helps improve read performance, it may not be as effective as MySQL's query cache in certain situations.
2. Write Performance:
When it comes to write performance, PostgreSQL generally outperforms MySQL. PostgreSQL is known for its robust transaction management capabilities and its support for advanced features such as ACID (Atomicity, Consistency, Isolation, Durability) compliance. These features ensure data integrity and provide better support for write-intensive workloads.
MySQL, on the other hand, sacrifices some write performance for better read performance. It uses different storage engines, such as InnoDB and MyISAM, which have different characteristics when it comes to write operations. InnoDB, which is the default storage engine for MySQL, provides better support for transactional operations and ensures data consistency at the cost of slightly lower write performance compared to MyISAM.
3. Scalability:
Both MySQL and PostgreSQL offer scalability options to handle growing workloads. MySQL provides tools like replication and sharding to distribute the load across multiple servers. Replication allows you to replicate data across multiple MySQL servers, while sharding allows you to partition data across multiple servers based on a specific criterion.
PostgreSQL also provides similar scalability options, such as streaming replication and logical replication. Streaming replication allows you to replicate data from a primary PostgreSQL server to one or more standby servers. Logical replication, on the other hand, allows you to replicate specific tables or databases to different servers.
4. Complex Queries:
When it comes to complex queries involving joins and subqueries, PostgreSQL often performs better than MySQL. PostgreSQL's query optimizer is known for its advanced optimization techniques, such as cost-based query optimization and query rewriting. These techniques help PostgreSQL generate more efficient query plans, resulting in faster query execution times.
MySQL, on the other hand, has a simpler query optimizer that may not be as effective in optimizing complex queries. However, MySQL's query optimizer has improved significantly in recent versions, and it can still handle most common query scenarios efficiently.
It's important to note that performance can vary depending on the specific workload and the tuning parameters used. It's recommended to benchmark both MySQL and PostgreSQL in your specific environment to determine which one performs better for your use case.
Related Article: Examining Query Execution Speed on Dates in PostgreSQL
Key Features of PostgreSQL compared to MySQL
PostgreSQL offers several key features that set it apart from MySQL. These features make PostgreSQL a popular choice for applications that require advanced functionality and robustness. Let's explore some of the key features of PostgreSQL compared to MySQL.
1. Advanced Data Types:
PostgreSQL provides a wide range of advanced data types that are not available in MySQL. These data types include arrays, JSON, hstore, UUID, and geometric types. These data types allow developers to store and manipulate complex data structures more efficiently.
2. Extensibility:
PostgreSQL is highly extensible, allowing developers to define their own data types, operators, and functions. This extensibility allows you to customize PostgreSQL to meet the specific requirements of your application.
3. Concurrency Control:
PostgreSQL provides advanced concurrency control mechanisms, such as multi-version concurrency control (MVCC). MVCC allows multiple transactions to access the database simultaneously without blocking each other, improving concurrency and scalability.
4. Full Text Search:
PostgreSQL has built-in support for full-text search, allowing you to perform advanced text search operations on large volumes of text data efficiently. This feature is not available in MySQL by default.
5. Geospatial Capabilities:
PostgreSQL has extensive support for geospatial data and provides a wide range of functions and operators for working with spatial data. This makes PostgreSQL a popular choice for applications that deal with location-based data.
6. Stored Procedures and Triggers:
PostgreSQL supports stored procedures and triggers, allowing you to define custom business logic directly in the database. This can help improve performance by reducing the amount of data transferred between the application and the database.
Limitations of Querying in MySQL and PostgreSQL
While both MySQL and PostgreSQL are useful relational database management systems, they have their own limitations when it comes to querying data. Let's explore some of the limitations of querying in MySQL and PostgreSQL.
1. MySQL Limitations:
- Lack of support for advanced SQL features: MySQL has traditionally lagged behind PostgreSQL in terms of SQL features and support for advanced functionality. While MySQL has made significant improvements in recent versions, it may still lack certain advanced features that are available in PostgreSQL.
- Limited subquery support: MySQL's subquery support is not as extensive as PostgreSQL's. It may not be able to handle complex subqueries efficiently, which can impact query performance.
- Lack of transactional support in certain storage engines: MySQL offers different storage engines, such as InnoDB and MyISAM. However, not all storage engines support transactions. For example, MyISAM does not support transactions, which can be a limitation in certain scenarios.
2. PostgreSQL Limitations:
- Higher memory requirements: PostgreSQL generally requires more memory compared to MySQL, especially when dealing with large datasets. This can be a limitation in environments with limited resources.
- Slower performance for certain types of queries: While PostgreSQL's query optimizer is known for its advanced optimization techniques, it may not perform as well as MySQL's optimizer for certain types of queries. This can impact query performance in specific scenarios.
- Complexity for beginners: PostgreSQL has a steeper learning curve compared to MySQL, especially for beginners. Its advanced features and extensive configuration options can be overwhelming for new users.
It's important to consider these limitations when choosing between MySQL and PostgreSQL for your querying needs. It's recommended to evaluate your specific requirements and use cases to determine which database system is the best fit for your application.
Migrating Queries from MySQL to PostgreSQL
If you are considering migrating your queries from MySQL to PostgreSQL, it's important to understand the differences in syntax and functionality between the two database systems. Here are some steps to help you migrate queries from MySQL to PostgreSQL.
1. Analyze your MySQL queries:
Start by analyzing your existing MySQL queries to understand their structure and functionality. Identify any MySQL-specific syntax or features used in the queries.
2. Study PostgreSQL documentation:
Familiarize yourself with the PostgreSQL documentation, specifically the sections related to SQL syntax and functionality. Understand the differences between MySQL and PostgreSQL in terms of querying methods and syntax.
3. Convert MySQL-specific syntax to PostgreSQL syntax:
Identify any MySQL-specific syntax used in your queries and convert them to equivalent PostgreSQL syntax. For example, if your MySQL query uses the LIMIT
clause, you will need to convert it to the equivalent FETCH FIRST
clause in PostgreSQL.
4. Test and validate the converted queries:
Once you have converted your queries to PostgreSQL syntax, test and validate them against your PostgreSQL database. Ensure that the queries produce the expected results and that any MySQL-specific functionality is replicated correctly in PostgreSQL.
5. Optimize the converted queries:
After migrating your queries, you may need to optimize them for performance in the PostgreSQL environment. Analyze the query execution plans and consider indexing strategies, query rewriting, and other optimization techniques to improve query performance.
6. Test and validate the optimized queries:
Test and validate the optimized queries to ensure that they perform well in the PostgreSQL environment. Monitor query execution times and compare them to the original MySQL queries to ensure that the performance has improved.
7. Fine-tune the queries:
Continuously monitor and fine-tune the migrated queries in the PostgreSQL environment. Keep an eye on query performance and make adjustments as needed to optimize performance.
Optimizing Query Performance in MySQL and PostgreSQL
Optimizing query performance is crucial for ensuring that your database performs efficiently and meets the demands of your application. Both MySQL and PostgreSQL provide various techniques and strategies to optimize query performance. Let's explore some of these techniques for both database systems.
1. MySQL Performance Optimization:
- Indexing: Proper indexing can significantly improve query performance in MySQL. Identify the columns frequently used in the WHERE, JOIN, and ORDER BY clauses, and create indexes on those columns. However, be cautious not to over-index, as it can impact write performance.
- Query optimization: Use the EXPLAIN statement to analyze the query execution plan and identify any potential bottlenecks. Use appropriate JOIN techniques, such as INNER JOIN, LEFT JOIN, and RIGHT JOIN, based on the relationships between the tables. Rewrite queries, if necessary, to simplify the logic and improve performance.
- Query caching: Enable the query cache in MySQL to cache the results of frequently executed queries. This can help improve performance by reducing the need to execute the same query repeatedly.
- Configuration tuning: Adjust the MySQL configuration parameters, such as the buffer sizes, thread pool size, and query cache size, based on your specific workload and hardware resources. Monitor performance metrics and make adjustments as needed.
2. PostgreSQL Performance Optimization:
- Indexing: Similar to MySQL, proper indexing is important for query performance in PostgreSQL. Analyze the query execution plans using the EXPLAIN statement and create indexes on the frequently accessed columns. Consider using partial indexes and expression indexes for more complex scenarios.
- Query optimization: Use the EXPLAIN statement in PostgreSQL to analyze the query execution plans and identify any performance issues. Rewrite queries, if necessary, to simplify the logic and improve performance. Consider using techniques like CTEs (Common Table Expressions) and window functions to optimize complex queries.
- Configuration tuning: Adjust the PostgreSQL configuration parameters, such as the shared buffers, work_mem, and max_connections, based on your specific workload and hardware resources. Monitor performance metrics and make adjustments as needed.
- Table partitioning: If you have large tables, consider partitioning them based on a specific criterion, such as date or range. This can improve query performance by reducing the amount of data that needs to be scanned.
It's important to note that query performance optimization is an ongoing process. Continuously monitor your queries, analyze their execution plans, and make adjustments as needed to ensure optimal performance.
Related Article: How to Set Timestamps With & Without Time Zone in PostgreSQL
Handling Relational Databases in MySQL and PostgreSQL
Both MySQL and PostgreSQL are relational database management systems (RDBMS) that provide useful features for handling relational databases. Let's explore some of the key features and techniques for handling relational databases in MySQL and PostgreSQL.
1. Table Creation:
In both MySQL and PostgreSQL, you can create tables to store structured data. Define the table schema by specifying the column names, data types, and any constraints, such as primary keys or foreign keys. Here's an example of creating a table in MySQL:
CREATE TABLE employees ( id INT PRIMARY KEY, name VARCHAR(100), department_id INT, FOREIGN KEY (department_id) REFERENCES departments(id) );
And here's an example of creating the same table in PostgreSQL:
CREATE TABLE employees ( id INT PRIMARY KEY, name VARCHAR(100), department_id INT, CONSTRAINT fk_department_id FOREIGN KEY (department_id) REFERENCES departments(id) );
2. Data Insertion:
Both MySQL and PostgreSQL allow you to insert data into tables using the INSERT statement. Specify the column names and their corresponding values. Here's an example of inserting data into the employees table in MySQL:
INSERT INTO employees (id, name, department_id) VALUES (1, 'John Doe', 1), (2, 'Jane Smith', 2);
And here's an example of inserting the same data into the employees table in PostgreSQL:
INSERT INTO employees (id, name, department_id) VALUES (1, 'John Doe', 1), (2, 'Jane Smith', 2);
3. Querying Related Data:
Both MySQL and PostgreSQL support querying related data using JOINs. JOINs allow you to combine rows from two or more tables based on a related column between them. Here's an example of querying related data in MySQL:
SELECT employees.name, departments.name FROM employees JOIN departments ON employees.department_id = departments.id;
And here's an example of querying the same related data in PostgreSQL:
SELECT employees.name, departments.name FROM employees JOIN departments ON employees.department_id = departments.id;
4. Data Modification:
Both MySQL and PostgreSQL allow you to modify existing data in tables using the UPDATE statement. Specify the columns to update and their new values. Here's an example of updating data in the employees table in MySQL:
UPDATE employees SET name = 'John Smith' WHERE id = 1;
And here's an example of updating the same data in the employees table in PostgreSQL:
UPDATE employees SET name = 'John Smith' WHERE id = 1;
5. Data Deletion:
Both MySQL and PostgreSQL allow you to delete data from tables using the DELETE statement. Specify the conditions for deleting rows. Here's an example of deleting data from the employees table in MySQL:
DELETE FROM employees WHERE id = 1;
And here's an example of deleting the same data from the employees table in PostgreSQL:
DELETE FROM employees WHERE id = 1;
Handling relational databases in MySQL and PostgreSQL involves creating tables, inserting data, querying related data, and modifying or deleting data as needed. Both database systems provide similar capabilities for handling relational databases, making it easy to work with structured data.
Performance Differences in Querying Large Datasets in MySQL and PostgreSQL
Querying large datasets can be a challenging task, especially when it comes to performance. Both MySQL and PostgreSQL offer features and techniques to handle large datasets efficiently. Let's explore some of the performance differences in querying large datasets in MySQL and PostgreSQL.
1. Indexing:
Proper indexing is crucial for efficient querying of large datasets in both MySQL and PostgreSQL. Indexes allow the database to locate and retrieve data more quickly, reducing the need for full-table scans. However, the indexing strategies and mechanisms differ between the two databases.
MySQL uses different storage engines, such as InnoDB and MyISAM, which have different indexing mechanisms. InnoDB uses a clustered index to store data physically in the order of the index, which can improve performance for large datasets. MyISAM, on the other hand, uses a separate index file and data file, which can be less efficient for large datasets.
PostgreSQL uses a B-tree index structure by default, which is designed to handle large datasets efficiently. It also provides other indexing options, such as hash indexes and GiST (Generalized Search Tree) indexes, which can be useful for specific scenarios.
2. Query Optimization:
Both MySQL and PostgreSQL have query optimization mechanisms to improve performance when querying large datasets. These mechanisms involve generating optimal query execution plans based on the query structure and available indexes.
MySQL's query optimizer has improved significantly in recent versions, but it may not be as effective as PostgreSQL's optimizer for complex queries involving large datasets. PostgreSQL's query optimizer uses advanced optimization techniques, such as cost-based query optimization, to generate efficient query plans. This can result in faster query execution times for large datasets.
3. Partitioning:
Partitioning is a technique used to divide large datasets into smaller, more manageable pieces. Both MySQL and PostgreSQL provide partitioning options to improve performance when querying large datasets.
MySQL supports horizontal partitioning, which involves dividing a table into multiple smaller tables based on a specific criterion, such as range or list. Each smaller table is called a partition and can be queried independently, improving performance for large datasets.
PostgreSQL supports both horizontal and vertical partitioning. Horizontal partitioning in PostgreSQL is similar to MySQL, where a table is divided into smaller partitions based on a specific criterion. Vertical partitioning, on the other hand, involves dividing a table into multiple smaller tables based on the columns, improving performance by reducing the amount of data that needs to be scanned.
4. Caching:
Caching can significantly improve query performance when querying large datasets. Both MySQL and PostgreSQL provide caching mechanisms to reduce disk I/O and improve response times for frequently accessed data.
MySQL uses a query cache mechanism that caches the results of frequently executed queries. This can be useful for read-heavy workloads where the same query is executed multiple times. However, the query cache may not be as effective for large datasets or write-intensive workloads.
PostgreSQL, on the other hand, uses a shared buffer cache to store recently accessed data in memory. This cache can improve performance by reducing the need to read data from disk. PostgreSQL also provides options for fine-tuning the cache size and other caching parameters to optimize performance for large datasets.
Overall, both MySQL and PostgreSQL provide features and techniques to handle large datasets efficiently. However, PostgreSQL's advanced query optimizer and indexing mechanisms make it a popular choice for querying large datasets. It's recommended to benchmark both databases in your specific environment to determine which one performs better for your use case.
Additional Resources
- PostgreSQL Data Types