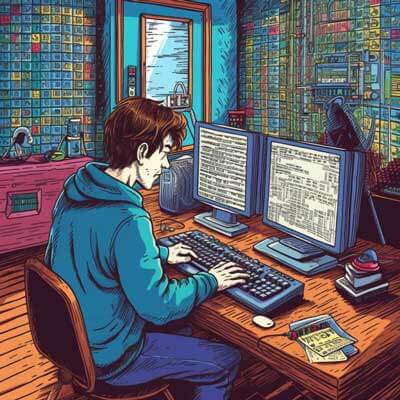
- What is schema stitching in GraphQL?
- How does Relay work with GraphQL?
- What are GraphQL subscriptions?
- What is introspection in GraphQL?
- How do directives work in GraphQL?
- How can caching be implemented in GraphQL?
- What are the best practices for pagination in GraphQL?
- What are GraphQL fragments and how are they used?
- What is the role of the type system in GraphQL?
- How do resolvers work in GraphQL?
- Additional Resources
In today’s data-driven world, organizations are constantly searching for ways to efficiently manage and query their data. GraphQL, a query language for APIs, has gained popularity due to its ability to provide a flexible and efficient approach to data fetching and manipulation.
One useful feature of GraphQL is its ability to integrate with various data sources, including Snowflake. Snowflake is a cloud-based data warehousing solution that allows organizations to store and analyze large amounts of data. In this article, we will explore how GraphQL can be integrated with Snowflake, enabling efficient querying and manipulation of data.
What is schema stitching in GraphQL?
Schema stitching is a technique in GraphQL that allows multiple GraphQL schemas to be combined into a single schema. This enables developers to create a unified API that can fetch data from multiple sources.
To illustrate this concept, let’s consider a scenario where we have two GraphQL schemas: one that provides information about users and another that provides information about products. We can use schema stitching to combine these schemas into a single schema, allowing us to query for both user and product data in a single GraphQL query.
Here’s an example of how schema stitching can be implemented in GraphQL:
import { makeExecutableSchema } from 'graphql-tools'; const userSchema = ` type User { id: ID! name: String email: String } type Query { user(id: ID!): User } `; const productSchema = ` type Product { id: ID! name: String price: Float } type Query { product(id: ID!): Product } `; const schema = makeExecutableSchema({ typeDefs: [userSchema, productSchema], });
In the above example, we define two separate schemas for users and products. We then use the makeExecutableSchema
function from the graphql-tools
library to combine these schemas into a single schema.
Once the schemas are stitched together, we can query for user and product data using a single GraphQL query. For example, we can fetch a user and their associated products like this:
query { user(id: "1") { name email products { name price } } }
This query will return the user’s name and email, as well as the name and price of the products associated with that user.
Related Article: Exploring GraphQL Playground Query Variables
How does Relay work with GraphQL?
Relay is a useful JavaScript framework developed by Facebook for building data-driven React applications. It provides a set of tools and conventions that make it easier to fetch and manage data from a GraphQL API.
One of the key features of Relay is its ability to perform efficient data fetching by batching and caching GraphQL queries. Relay accomplishes this by leveraging GraphQL’s introspection capabilities to analyze the query and optimize the data fetching process.
To understand how Relay works with GraphQL, let’s consider an example where we have a React component that needs to fetch and display a list of users from a GraphQL API using Relay.
First, we define a GraphQL query using the Relay-specific @relay
directive:
query UserListQuery { users { edges { node { id name email } } } }
In the above query, we request the id
, name
, and email
fields for each user. The @relay
directive tells Relay that this query should be processed using its optimizations.
Next, we define a Relay container component that wraps our React component:
import { graphql, createFragmentContainer } from 'react-relay'; const UserList = ({ data }) => { const { users } = data; return ( <ul> {users.edges.map(({ node }) => ( <li key={node.id}> <div>Name: {node.name}</div> <div>Email: {node.email}</div> </li> ))} </ul> ); }; export default createFragmentContainer(UserList, { data: graphql` fragment UserList_data on Query { users { edges { node { id name email } } } } `, });
In the container component, we define a fragment that specifies the data requirements for our component. This fragment matches the structure of the GraphQL query we defined earlier.
Finally, we use the createFragmentContainer
function from the react-relay
library to create a container component that fetches the required data and passes it to our React component as props.
With Relay, the data fetching process is automatically optimized based on the query structure. Relay will batch multiple queries together and cache the results, reducing the number of network requests and improving performance.
What are GraphQL subscriptions?
GraphQL subscriptions are a feature of the GraphQL specification that allows clients to receive real-time updates from a server. Subscriptions enable applications to maintain a persistent connection with a server and receive data as it changes.
To illustrate how GraphQL subscriptions work, let’s consider a chat application where users can send messages to each other in real-time. We can use GraphQL subscriptions to notify clients whenever a new message is sent.
First, we define a GraphQL subscription that represents a new message:
subscription NewMessage { newMessage { id text createdAt sender { id name } } }
In the above subscription, we request the id
, text
, createdAt
, and sender
fields for each new message.
On the server side, we implement the resolver for the newMessage
subscription to handle the logic for sending new messages to clients. This resolver can be implemented using various technologies, depending on the GraphQL server implementation.
Once the server is set up to handle subscriptions, clients can subscribe to the newMessage
subscription to receive real-time updates. For example, a client can use the Apollo Client library to subscribe to the newMessage
subscription:
import { gql } from '@apollo/client'; const NEW_MESSAGE_SUBSCRIPTION = gql` subscription NewMessage { newMessage { id text createdAt sender { id name } } } `; const subscription = client.subscribe({ query: NEW_MESSAGE_SUBSCRIPTION }); subscription.subscribe({ next: ({ data }) => { // Handle new message }, error: (error) => { // Handle subscription error }, });
In the above example, we use Apollo Client to subscribe to the newMessage
subscription. When a new message is sent, the next
callback will be called with the new message data. We can then update the UI to display the new message.
GraphQL subscriptions provide a useful mechanism for building real-time applications and keeping clients in sync with server-side updates.
What is introspection in GraphQL?
Introspection is a feature of the GraphQL specification that allows clients to query the schema of a GraphQL API. It provides a way for clients to discover and understand the available types, fields, and directives of the API.
Introspection is especially useful during development and debugging, as it allows clients to dynamically explore the schema of a GraphQL API without prior knowledge of its structure.
To perform introspection in GraphQL, we can use the __schema
field, which is automatically added to the root query type of a GraphQL schema. This field returns an object that represents the schema of the API.
Here’s an example of how to perform introspection using GraphQL:
query IntrospectionQuery { __schema { types { name kind description fields { name type { name kind } } } } }
In the above query, we request the name
, kind
, description
, and fields
fields for each type in the schema. The __schema
field represents the root of the schema.
The result of the introspection query will be a JSON object that represents the schema of the GraphQL API. Clients can use this information to dynamically generate queries, validate input, or build tools that interact with the API.
Introspection is a useful feature that enables clients to interact with GraphQL APIs in a flexible and dynamic manner.
Related Article: Step by Step Process: Passing Enum in GraphQL Query
How do directives work in GraphQL?
Directives are a feature of the GraphQL specification that allows clients to provide additional instructions to the server at runtime. Directives are used to modify the behavior of GraphQL operations and types based on certain conditions or requirements.
In GraphQL, directives are defined using the directive
keyword and can be applied to fields, arguments, or fragments. Directives can be used to control field visibility, provide default values for arguments, or apply custom logic to fields.
To illustrate how directives work in GraphQL, let’s consider an example where we have a GraphQL schema that defines a User
type with a isAdmin
field. We can use a directive to restrict access to the isAdmin
field based on the user’s role.
First, we define a directive called isAdmin
that can be applied to fields:
directive @isAdmin on FIELD_DEFINITION
Next, we apply the @isAdmin
directive to the isAdmin
field of the User
type:
type User { id: ID! name: String email: String isAdmin: Boolean @isAdmin }
In the above example, the @isAdmin
directive is applied to the isAdmin
field. This directive can be used to implement custom logic that determines whether a user has access to this field.
On the server side, we implement the resolver for the isAdmin
directive to handle the logic for determining whether a user is an admin. This resolver can be implemented using various technologies, depending on the GraphQL server implementation.
When a client queries the isAdmin
field, the server will execute the resolver for the isAdmin
directive and determine whether the user has access to the field based on the custom logic.
Directives provide a flexible and useful way to modify the behavior of GraphQL operations and types based on various conditions or requirements.
How can caching be implemented in GraphQL?
Caching is an important technique for improving the performance and efficiency of data fetching in GraphQL. By caching the results of GraphQL queries, we can avoid unnecessary network requests and reduce the load on the server.
There are several ways to implement caching in GraphQL, depending on the specific requirements and constraints of the application.
One common approach is to use a caching layer between the client and the server. This caching layer can be implemented using technologies such as Redis or Memcached. When a client sends a GraphQL query, the caching layer checks if the result is already cached. If the result is found in the cache, it is returned to the client without hitting the server. If the result is not found in the cache, the caching layer forwards the query to the server, caches the result, and returns it to the client.
Another approach is to implement caching at the field level within the GraphQL server. This can be done by adding a caching layer to the resolver functions that fetch data for each field. When a resolver function is called, it first checks if the result is already cached. If the result is found in the cache, it is returned immediately. If the result is not found in the cache, the resolver function fetches the data from the data source, caches the result, and returns it.
Here’s an example of how caching can be implemented in a GraphQL server using the dataloader
library:
import DataLoader from 'dataloader'; const userLoader = new DataLoader(async (ids) => { // Fetch users from the data source const users = await fetchUsers(ids); // Cache the fetched users users.forEach((user) => userLoader.prime(user.id, user)); // Return the fetched users return users; }); const resolvers = { Query: { user: (_, { id }) => userLoader.load(id), }, };
In the above example, we use the dataloader
library to implement caching at the field level. The userLoader
instance is responsible for fetching and caching user data. When the user
field is queried, the userLoader.load
function is called, which either fetches the user from the data source or returns it from the cache.
Caching is an essential technique for optimizing the performance of GraphQL APIs and reducing the load on the server. The specific implementation of caching will depend on the requirements and constraints of the application.
What are the best practices for pagination in GraphQL?
Pagination is a common requirement in GraphQL APIs, especially when dealing with large datasets. GraphQL provides various techniques and best practices for implementing pagination in a flexible and efficient manner.
One common approach to pagination in GraphQL is to use the Relay specification, which provides a set of conventions and best practices for building pagination in GraphQL APIs. Using Relay-style pagination allows clients to efficiently fetch paginated data using standardized cursors.
To implement Relay-style pagination, we can use the Connection
pattern, which consists of defining a connection type that contains the paginated data along with additional metadata.
Here’s an example of how Relay-style pagination can be implemented in a GraphQL schema:
type User { id: ID! name: String } type UserConnection { edges: [UserEdge!]! pageInfo: PageInfo! } type UserEdge { node: User! cursor: String! } type PageInfo { hasNextPage: Boolean! hasPreviousPage: Boolean! startCursor: String endCursor: String } type Query { users(first: Int, after: String): UserConnection! }
In the above example, we define a UserConnection
type that contains the paginated users, along with the pageInfo
field that provides metadata about the pagination.
The UserConnection
type consists of an array of UserEdge
objects, where each UserEdge
contains a node
field that represents a user and a cursor
field that represents a unique identifier for the user.
The pageInfo
field contains information about the pagination, such as whether there is a next or previous page, as well as the cursors of the first and last items in the paginated list.
The Query
type includes a users
field that accepts optional first
and after
arguments for pagination. The first
argument specifies the maximum number of users to return, and the after
argument specifies the cursor of the last item in the previous page.
Related Article: Achieving Production-Ready GraphQL
What are GraphQL fragments and how are they used?
GraphQL fragments are reusable units of fields that can be included in multiple GraphQL queries. Fragments allow us to define a set of fields once and then reference them in multiple places, reducing duplication and improving maintainability.
To define a GraphQL fragment, we use the fragment
keyword followed by the name of the fragment and the type it applies to. We then specify the fields we want to include in the fragment.
Here’s an example of how to define a GraphQL fragment:
fragment UserFields on User { id name email }
In the above example, we define a fragment called UserFields
that applies to the User
type. The fragment includes the id
, name
, and email
fields.
To use a fragment in a GraphQL query, we use the ...
syntax followed by the name of the fragment. This allows us to include the fields defined in the fragment in the query.
Here’s an example of how to use a fragment in a GraphQL query:
query { user(id: "1") { ...UserFields } }
In the above query, we use the UserFields
fragment to include the id
, name
, and email
fields in the response for the user
query.
Fragments can also be used with interfaces and unions to specify a set of fields that are common to multiple types.
GraphQL fragments provide a useful way to define reusable units of fields and improve the maintainability of GraphQL queries.
What is the role of the type system in GraphQL?
The type system is a fundamental component of GraphQL that defines the structure and capabilities of a GraphQL API. The type system allows us to define the available types, fields, and operations in the API, as well as the relationships between them.
In GraphQL, types are used to define the shape of the data that can be queried. Each field in a GraphQL schema has a specific type, which determines the kind of data that can be fetched for that field.
The GraphQL type system provides a set of built-in scalar types, such as String
, Int
, Float
, Boolean
, and ID
, which represent common data types. It also allows us to define custom object types, interface types, union types, enumeration types, and input types.
Here’s an example of how to define custom types in a GraphQL schema:
type User { id: ID! name: String email: String } type Query { user(id: ID!): User }
In the above example, we define a custom type called User
with fields for id
, name
, and email
. We also define a root Query
type with a field for fetching a user by their id
.
The type system also allows us to define relationships between types using fields and arguments. For example, we can define a field that returns a list of users, or a field that accepts arguments for filtering or sorting the data.
How do resolvers work in GraphQL?
Resolvers are functions that are responsible for fetching the data for a specific field in a GraphQL schema. They are a key component of the GraphQL execution process, as they determine how the data is resolved and returned to the client.
In GraphQL, each field in a schema must have a corresponding resolver function. The resolver function is responsible for fetching the data for that field from the appropriate data source, such as a database, an API, or an in-memory cache.
Resolvers can be implemented using various technologies and programming languages, depending on the GraphQL server implementation. They can be synchronous or asynchronous functions, and they have access to the parent object, arguments, context, and other information related to the execution of the query.
Here’s an example of how to implement resolvers in a JavaScript-based GraphQL server using the graphql-js
library:
const resolvers = { Query: { user: (_, { id }) => { // Fetch user data from the data source return fetchUser(id); }, }, User: { name: (user) => { // Resolve the name field of the User type return user.firstName + ' ' + user.lastName; }, }, };
In the above example, we define resolvers for the user
query and the name
field of the User
type. The resolver for the user
query fetches the user data from the data source, while the resolver for the name
field resolves the name
field based on the firstName
and lastName
fields of the user object.
When a client queries the user
query, the resolver for the user
query is called, and the result is returned to the client. If the client also requests the name
field of the user, the resolver for the name
field is called, and the result is added to the response.
Related Article: Implementing TypeORM with GraphQL and NestJS
Additional Resources
– What is GraphQL and how does it work?
– What are the benefits of using GraphQL in programming?
– Building a GraphQL API with Snowflake