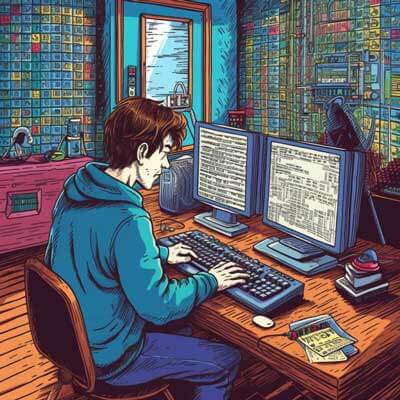
The ValueError: Invalid literal for int with base 10
error is a common issue that occurs in Python when trying to convert a string to an integer using the int()
function, but the string does not represent a valid integer in base 10. This error typically arises when there are non-numeric characters or invalid formatting in the string.
To fix this error, consider the following solutions:
1. Check the input string
The first step in troubleshooting this error is to examine the input string that is causing the issue. Look for any non-numeric characters, leading or trailing whitespace, or other invalid formatting. It’s important to ensure that the string only contains numeric characters and is properly formatted.
Here’s an example that demonstrates how to check and clean the input string:
def convert_to_integer(input_string): cleaned_string = input_string.strip() # Remove leading/trailing whitespace if not cleaned_string.isdigit(): raise ValueError("Invalid input: string contains non-numeric characters") return int(cleaned_string) try: input_string = "123abc" result = convert_to_integer(input_string) print(f"Converted value: {result}") except ValueError as e: print(f"Error: {str(e)}")
In this example, the convert_to_integer()
function first removes any leading or trailing whitespace from the input string using the strip()
method. Then, it checks if the cleaned string consists only of digits using the isdigit()
method. If any non-numeric characters are found, a ValueError
is raised with an appropriate error message.
Related Article: Fixing "ValueError: Setting Array with a Sequence" In Python
2. Handle exceptions using try-except block
Another approach to handling the ValueError
is to use a try-except block. This allows you to catch the exception and handle it gracefully, providing a fallback value or displaying an error message to the user.
Here’s an example that demonstrates using a try-except block to handle the ValueError
:
input_string = "123abc" try: result = int(input_string) print(f"Converted value: {result}") except ValueError: print("Error: Invalid input string")
In this example, the int()
function is called directly on the input string. If the string cannot be converted to an integer, a ValueError
is raised. The except block catches the exception and prints an appropriate error message.
Why is the question asked?
The question “How to fix ValueError: Invalid literal for int with base 10?” is often asked by Python developers who encounter this error while attempting to convert a string to an integer. This error can occur due to various reasons, such as input validation issues, incorrect data formatting, or improper handling of user input.
By understanding the possible causes and solutions for this error, developers can troubleshoot and resolve the issue more effectively.
Potential reasons for the error:
– Non-numeric characters in the string: The presence of characters other than digits in the input string can cause the ValueError
to be raised.
– Leading or trailing whitespace: Whitespace at the beginning or end of the string can also trigger the error.
– Incorrect data formatting: If the string is not properly formatted as a valid integer, the int()
function will fail to convert it.
Suggestions and alternative ideas:
– Validate user input: Before attempting to convert a string to an integer, it’s important to validate the input and ensure it meets the expected format. This can be done using regular expressions or custom validation logic.
– Handle exceptions gracefully: Instead of allowing the ValueError
to propagate and potentially crash the program, it’s recommended to handle the exception using a try-except block. This allows for better error handling and can provide a more user-friendly experience.
– Use alternative conversion methods: If the input string may contain non-numeric characters or you need more flexibility in handling non-base 10 integers, consider using alternative conversion methods. For example, the float()
function can be used to convert strings to floating-point numbers.
Best practices:
– Validate user input before attempting conversion to avoid potential errors. Use regex or custom validation logic to ensure the input string only contains valid characters.
– When handling exceptions, provide informative error messages to aid in troubleshooting and debugging.
– Use try-except blocks to catch and handle exceptions instead of allowing them to crash the program.
– Follow proper coding conventions and consistently use error handling techniques to improve code readability and maintainability.