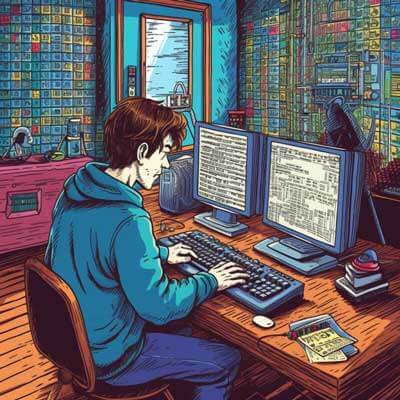
Table of Contents
Setting a time delay in JavaScript is a common requirement when working with web applications or scripts. Whether you need to delay the execution of a specific function, create a timed event, or add a delay between actions, JavaScript provides various methods to achieve this.
Using setTimeout()
One of the most commonly used methods to set a time delay in JavaScript is the setTimeout()
function. This function allows you to execute a specific piece of code after a specified delay, in milliseconds.
Here's the syntax for using setTimeout()
:
setTimeout(function, delay);
The function
parameter represents the code that you want to execute after the delay, and the delay
parameter specifies the time delay in milliseconds.
For example, let's say you want to display an alert message after a delay of 3 seconds. You can achieve this using the setTimeout()
function:
setTimeout(function() { alert("Delayed message"); }, 3000);
In the above code, the anonymous function inside setTimeout()
will be executed after a delay of 3000 milliseconds (3 seconds), resulting in an alert message being displayed.
Related Article: How to Retrieve Remote URLs with Next.js
Using setInterval()
Another method to set a time delay in JavaScript is the setInterval()
function. This function repeatedly calls a specified function or piece of code at a given interval.
Here's the syntax for using setInterval()
:
setInterval(function, delay);
The function
parameter represents the code that you want to execute repeatedly, and the delay
parameter specifies the time interval in milliseconds between each execution.
For example, let's say you want to log a message to the console every second. You can achieve this using the setInterval()
function:
setInterval(function() { console.log("Message logged every second"); }, 1000);
In the above code, the anonymous function inside setInterval()
will be executed every 1000 milliseconds (1 second), resulting in a message being logged to the console.
It's important to note that setInterval()
will continue to execute the specified function indefinitely at the given interval until you explicitly stop it using the clearInterval()
function.
Why is the question asked?
The question of how to set a time delay in JavaScript is often asked for various reasons. Some potential reasons include:
1. Animation Effects: JavaScript is frequently used to create animation effects on web pages. Setting a time delay allows you to control the timing of animations, such as fading in or out, sliding elements, or transitioning between states.
2. Event Timing: Delaying the execution of code can be useful when you want to synchronize certain events or actions. For example, you might want to display a message after a user clicks a button, but only after a short delay to provide a smoother user experience.
3. Asynchronous Operations: JavaScript is often used to handle asynchronous operations, such as making API calls or performing AJAX requests. Setting a time delay can help manage the timing of these operations, ensuring that they occur at the desired moments.
Suggestions and Alternative Ideas
While setTimeout()
and setInterval()
are commonly used for setting time delays in JavaScript, there are alternative approaches and suggestions worth considering:
1. Promises: JavaScript Promises provide a more structured and modern way to handle asynchronous operations. Instead of relying on timers, you can use Promise-based functions and the setTimeout()
function to introduce time delays within a Promise chain.
2. Event-Based Approaches: In some scenarios, it may be more appropriate to use event-based approaches, such as the setTimeout()
method of the window
object or the requestAnimationFrame()
function, to achieve time delays. These methods are particularly useful for animation purposes.
3. Libraries and Frameworks: Depending on your project's requirements, you might consider utilizing JavaScript libraries or frameworks that provide built-in functionality for handling time delays. For example, popular libraries like jQuery or frameworks like React have their own mechanisms for managing timed events.
Related Article: How to Distinguish Between Let and Var in Javascript
Best Practices
When setting time delays in JavaScript, it's important to follow some best practices to ensure efficient and reliable code:
1. Use Clearing Functions: If you use setTimeout()
or setInterval()
, make sure to clear them when they are no longer needed using the clearTimeout()
or clearInterval()
functions, respectively. This prevents memory leaks and unnecessary resource consumption.
2. Avoid Excessive Delays: Be cautious when setting long time delays, as it can negatively impact the user experience. Long delays may cause the web page to become unresponsive or give the impression of slow loading times.
3. Consider Performance: JavaScript timers can impact the overall performance of your application, especially if you have multiple timers running simultaneously. Minimize the number of active timers and optimize your code to reduce unnecessary delays.
4. Use the Appropriate Time Unit: When specifying the delay, use the appropriate time unit to make your code more readable and avoid confusion. For example, use 1000
for 1 second rather than 60000
for 60 seconds.
5. Test and Debug: Always test your code thoroughly to ensure that the time delays are working as expected. Debug any issues that arise and consider using browser developer tools or logging techniques to help identify and fix problems.