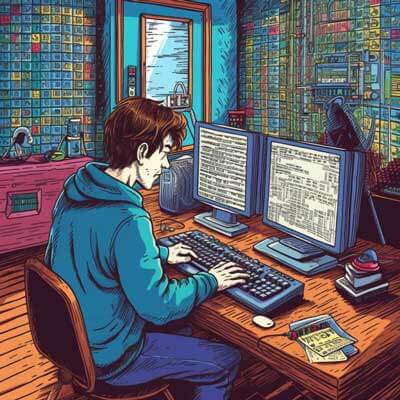
To get a timestamp in JavaScript, you can use the Date.now()
method or the getTime()
method of the Date
object. These methods return the number of milliseconds elapsed since January 1, 1970, 00:00:00 UTC (Coordinated Universal Time). This value is often referred to as a Unix timestamp.
Here are two possible ways to get a timestamp in JavaScript:
Using the Date.now() Method
The Date.now()
method returns the current timestamp in milliseconds. It is a static method, so you can call it directly on the Date
object.
const timestamp = Date.now(); console.log(timestamp);
This will output the current timestamp in milliseconds.
Related Article: How To Check If a Key Exists In a Javascript Object
Using the getTime() Method
The getTime()
method returns the timestamp in milliseconds for a specific Date
object. You can create a new Date
object and then call the getTime()
method on it.
const date = new Date(); const timestamp = date.getTime(); console.log(timestamp);
This will output the timestamp in milliseconds for the current date and time.
The question of how to get a timestamp in JavaScript may arise for various reasons. Here are some potential reasons why someone may need to get a timestamp:
– Recording events: Timestamps are often used to record the time at which specific events occur. For example, in a chat application, each message may be associated with a timestamp to indicate when it was sent.
– Comparing dates: When working with dates, it is often necessary to compare them. By converting dates to timestamps, you can easily compare them using simple numerical comparisons.
– Measuring elapsed time: By calculating the difference between two timestamps, you can measure the elapsed time between two events. This can be useful for performance monitoring or determining the duration of a process.
When working with timestamps in JavaScript, consider the following suggestions and alternative ideas:
– Be aware of the units: The timestamps returned by Date.now()
and getTime()
are in milliseconds. If you need the timestamp in seconds, you can divide it by 1000.
– Consider using a library: If you need to perform complex date and time calculations or handle time zones, consider using a library like Moment.js or Luxon. These libraries provide additional functionalities and make working with dates and timestamps easier.
– Use UTC methods: When working with timestamps, consider using the UTC versions of the Date
methods, such as getUTCFullYear()
, getUTCMonth()
, getUTCDate()
, etc. These methods return values in UTC instead of local time, which can help avoid issues related to time zones and daylight saving time.
Here is an example of how to convert a timestamp to a human-readable date and time using the Date
object:
const timestamp = 1632184260000; // Example timestamp const date = new Date(timestamp); const year = date.getFullYear(); const month = date.getMonth() + 1; // Months are zero-based const day = date.getDate(); const hours = date.getHours(); const minutes = date.getMinutes(); const seconds = date.getSeconds(); console.log(`${year}-${month}-${day} ${hours}:${minutes}:${seconds}`);
This code snippet converts the timestamp 1632184260000
to the format YYYY-MM-DD HH:mm:ss
.
In summary, getting a timestamp in JavaScript can be done using the Date.now()
method or the getTime()
method of the Date
object. These methods return the number of milliseconds since January 1, 1970, and can be used for various purposes such as recording events, comparing dates, or measuring elapsed time. Remember to consider units, use libraries for complex operations, and be aware of time zone differences when working with timestamps.
Related Article: How To Use the Javascript Void(0) Operator