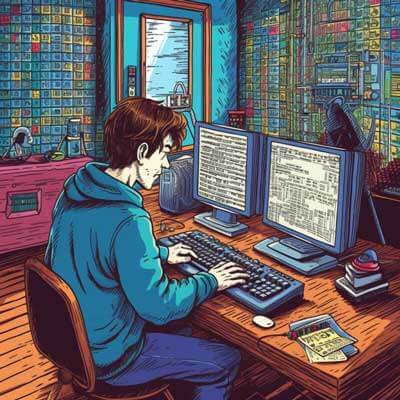
Table of Contents
Creating a Bash script in Ubuntu
To create a Bash script in Ubuntu, you can use any text editor of your choice, such as Nano or Vim. Here's an example of how to create a simple Bash script that prints "Hello, World!":
nano hello.sh
Inside the file, enter the following code:
#!/bin/bash echo "Hello, World!"
Save the file and exit the text editor.
Related Article: How to Compare Strings in Bash: A Simple Guide
Making a Bash script executable in Ubuntu
Before you can execute a Bash script, you need to make it executable. In Ubuntu, you can use the chmod
command to change the permissions of the file. Here's how you can make the hello.sh
script executable:
chmod +x hello.sh
Now, you can run the script by typing ./hello.sh
in the terminal:
./hello.sh
The output should be:
Hello, World!
Executing a Bash script at startup using systemd
Systemd is a system and service manager in Ubuntu that provides a standardized way of managing and controlling processes. You can use systemd to execute a Bash script at startup in Ubuntu.
1. Create a service unit file for your script. For example, create a file called myscript.service
in the /etc/systemd/system/
directory:
sudo nano /etc/systemd/system/myscript.service
2. Add the following content to the file:
[Unit] Description=My Script After=network.target [Service] ExecStart=/path/to/your/script.sh [Install] WantedBy=default.target
Replace /path/to/your/script.sh
with the actual path to your Bash script.
3. Save the file and exit the text editor.
4. Reload the systemd daemon to load the new service unit file:
sudo systemctl daemon-reload
5. Enable the service to start at boot:
sudo systemctl enable myscript.service
6. Start the service:
sudo systemctl start myscript.service
Now, your Bash script will be executed at startup.
Executing a Bash script at startup using rc.local
The rc.local
file is a script that is executed at the end of the system boot process in Ubuntu. You can use it to execute a Bash script at startup.
1. Open the rc.local
file in a text editor:
sudo nano /etc/rc.local
2. Add the following line before the exit 0
line:
/path/to/your/script.sh
Replace /path/to/your/script.sh
with the actual path to your Bash script.
3. Save the file and exit the text editor.
4. Make the rc.local
file executable:
sudo chmod +x /etc/rc.local
5. Reboot your system to execute the Bash script at startup.
Now, your Bash script will be executed at startup using the rc.local
file.
Related Article: Running a Script within a Bash Script in Linux
Executing a Bash script at startup using init.d
The init.d
directory is another method to execute a Bash script at startup in Ubuntu. It contains scripts that are executed during the boot process. To execute a Bash script at startup using init.d
, follow these steps:
1. Create an init script for your Bash script. For example, create a file called myscript
in the /etc/init.d/
directory:
sudo nano /etc/init.d/myscript
2. Add the following content to the file:
#!/bin/bash ### BEGIN INIT INFO # Provides: myscript # Required-Start: $remote_fs $syslog # Required-Stop: $remote_fs $syslog # Default-Start: 2 3 4 5 # Default-Stop: 0 1 6 # Short-Description: My Script # Description: Execute my script at startup ### END INIT INFO # Change to the directory where your script is located cd /path/to/your/script_directory # Execute your script ./your_script.sh
Replace /path/to/your/script_directory
with the actual path to the directory where your Bash script is located. Replace your_script.sh
with the actual name of your Bash script.
3. Save the file and exit the text editor.
4. Make the init script executable:
sudo chmod +x /etc/init.d/myscript
5. Update the init script to run at startup:
sudo update-rc.d myscript defaults
6. Reboot your system to execute the Bash script at startup.
Now, your Bash script will be executed at startup using the init.d
directory.
Understanding the purpose of a startup script
A startup script is a script that is executed automatically when a system boots up. Its purpose is to perform tasks or run commands that are necessary for the system to initialize and start running properly. Startup scripts can be used to configure network settings, mount filesystems, start services, or execute custom actions.
In Ubuntu Linux, startup scripts can be used to automate the execution of Bash scripts at system startup. This allows you to run custom commands or perform specific tasks automatically without manual intervention.
Scheduling a task to run automatically in Linux
In Linux, you can schedule tasks to run automatically using the cron
daemon. The cron
daemon is a time-based job scheduler that allows you to schedule commands or scripts to run at specific times, dates, or intervals.
To schedule a task to run automatically in Linux, you need to add an entry to the cron
table. You can do this by editing the crontab
file for the user or the system.
To edit the crontab
file for the current user, use the following command:
crontab -e
To edit the crontab
file for the system, use the following command:
sudo crontab -e
In the crontab
file, you can add entries in the following format:
* * * * * command_to_be_executed
Each field in the entry represents a time or date component, and the command_to_be_executed
is the command or script that you want to schedule.
For example, to schedule a Bash script to run every day at 9:00 AM, you can add the following entry to the crontab
file:
0 9 * * * /path/to/your/script.sh
Replace /path/to/your/script.sh
with the actual path to your Bash script.
Save the crontab
file and exit the text editor. The specified command or script will now be executed automatically at the scheduled time.
What is the shebang line in a Bash script?
The shebang line, also known as the hashbang or the interpreter directive, is a special line that is used at the beginning of a Bash script to specify the interpreter that should be used to execute the script.
The shebang line starts with a hash symbol (#
) followed by an exclamation mark (!
). After the exclamation mark, you specify the path to the interpreter that should be used.
For example, the following shebang line specifies that the script should be executed using the Bash interpreter:
#!/bin/bash
The shebang line is important because it allows you to write portable scripts that can be executed on different systems, even if the default shell interpreter is not Bash. By specifying the interpreter explicitly in the shebang line, you ensure that the script is executed correctly, regardless of the system configuration.
Related Article: Appending Dates to Filenames in Bash Scripts
Common Linux commands for executing Bash scripts
There are several common Linux commands that can be used to execute Bash scripts:
- bash
: This command is used to execute a Bash script explicitly. For example:
bash script.sh
- ./
: This command is used to execute a Bash script directly, without explicitly specifying the interpreter. The script must have the shebang line properly set. For example:
./script.sh
- source
or .
: These commands are used to execute a Bash script in the current shell environment, rather than in a subshell. This allows the script to modify environment variables or execute commands that affect the current shell session. For example:
source script.sh
or
. script.sh
These commands are useful when you want to execute a script that sets environment variables or defines functions that you want to use in your current shell session.
What is systemd and its relation to startup scripts?
Systemd is a system and service manager that is used in many modern Linux distributions, including Ubuntu. It is responsible for controlling the startup and management of system services, as well as other system-related tasks.
Systemd introduces a new approach to managing system services compared to traditional init systems. It uses a parallel and event-based activation system, which allows for faster and more efficient startup times. Systemd also provides features such as dependency management, process tracking, and service monitoring.
In relation to startup scripts, systemd provides a standardized way of executing scripts and managing services at system startup. It uses service unit files, which are configuration files that describe how a service should be started, stopped, and managed. These unit files can be used to execute Bash scripts at startup by defining a service unit that runs the script.
The purpose of the rc.local file in Linux
The rc.local
file is a script that is executed at the end of the system boot process in Linux. Its purpose is to allow system administrators to run custom commands or scripts at startup without modifying the system's default startup procedures.
The rc.local
file is located in the /etc
directory and is executed by the system's init process during the boot process. It is typically used to perform tasks that are specific to the local system or environment, such as configuring network settings, mounting filesystems, or starting custom services.
To use the rc.local
file, you simply add the commands or scripts that you want to run at startup to the file. Each command or script should be placed on a separate line, and the file should end with the line exit 0
.
It's important to note that the rc.local
file is executed as the root user, so you should exercise caution when adding commands or scripts to the file. It's recommended to use absolute paths for commands and scripts, and to include any necessary error handling or logging in your scripts to ensure that they run correctly.
The role of the init.d directory in Linux
The init.d
directory, short for initialization directory, is a directory in Linux that contains system startup scripts. It is located in the /etc
directory and is used by the system's init process to execute scripts during the boot process.
In Linux, the init process is responsible for starting and managing system services. When the system boots up, the init process reads the scripts in the init.d
directory and executes them in a specific order, as defined by the runlevel configuration.
The scripts in the init.d
directory are typically organized into different runlevels, which represent different system states or modes. Each runlevel has a specific set of scripts that are executed when the system enters that runlevel.
The scripts in the init.d
directory are executed by the init process using a standardized set of arguments, such as start
, stop
, restart
, and status
. These arguments determine the action that should be performed on the service or script.
The init.d
directory is a traditional method of managing system startup in Linux. However, modern Linux distributions, such as Ubuntu, have transitioned to using systemd as the default init system. While systemd still supports the execution of scripts in the init.d
directory, it encourages the use of service unit files for managing system services.
Related Article: How to Read Text Files in Linux with Bash Scripts
Additional Resources
- Difference between Local and Global Environment Variables in Linux