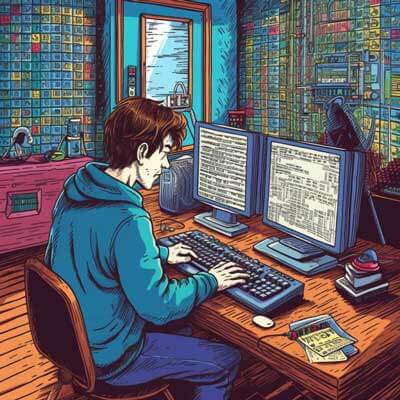
- Overview of Diphthong Detection Methods
- Python Libraries for Diphthong Detection
- Machine Learning Techniques for Diphthong Detection
- Challenges of Diphthong Detection
- Linguistic Analysis in Python for Diphthong Detection
- Pre-trained Models for Diphthong Detection
- Common Features for Diphthong Detection
- Additional Resources
Overview of Diphthong Detection Methods
Diphthongs, complex speech sounds beginning with one vowel sound and gliding into another within the same syllable, pose unique challenges in speech and linguistic analysis. The detection of these sounds is critical for various applications, including speech recognition, language learning apps, and linguistic research. Traditional methods rely heavily on acoustic analysis and phonetic algorithms, which analyze the sound frequencies and waveforms to identify the shift characteristic of diphthongs.
Related Article: 16 Amazing Python Libraries You Can Use Now
Python Libraries for Diphthong Detection
Python, being a versatile programming language, offers several libraries that facilitate the processing and analysis of audio data, useful in diphthong detection. Notably, librosa
and praat-parselmouth
are two libraries extensively used in this domain.
librosa
is primarily used for music and audio analysis, offering tools for feature extraction, such as Mel Frequency Cepstral Coefficients (MFCCs), which are beneficial for characterizing the unique properties of diphthongs.
import librosa y, sr = librosa.load('audio_file.wav') mfccs = librosa.feature.mfcc(y=y, sr=sr)
praat-parselmouth
integrates the functionality of Praat, a useful software for speech analysis, directly into Python. This integration allows for detailed acoustic analysis necessary for detecting diphthongs.
import parselmouth snd = parselmouth.Sound('audio_file.wav') formants = snd.to_formant_burg()
Machine Learning Techniques for Diphthong Detection
Machine Learning (ML) offers sophisticated approaches to diphthong detection, leveraging patterns in data to predict or classify speech sounds. Supervised learning models, such as Support Vector Machines (SVMs) and Neural Networks, have shown promise in this area. These models are trained on labeled datasets containing examples of diphthongs and their contexts, learning to generalize from these examples to detect diphthongs in unseen data.
Here is a simple example using SVM from the scikit-learn
library:
from sklearn import svm from sklearn.model_selection import train_test_split from sklearn.metrics import accuracy_score # X represents features extracted from audio, and y represents labels (0 for non-diphthong, 1 for diphthong) X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2) clf = svm.SVC() clf.fit(X_train, y_train) predictions = clf.predict(X_test) print("Accuracy:", accuracy_score(y_test, predictions))
Challenges of Diphthong Detection
Detecting diphthongs accurately involves overcoming several challenges. Variability in speech, including differences in accents, speech rate, and intonation, can significantly impact the acoustic features of diphthongs. Moreover, the quality of audio recordings and background noise can further complicate detection efforts. Developing robust methods that can generalize across these variations remains a significant hurdle in this field.
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
Linguistic Analysis in Python for Diphthong Detection
Linguistic analysis involves understanding the nuances of language sounds and structures. Python can be used to perform detailed linguistic analyses by combining libraries like NLTK
(Natural Language Toolkit) for processing text and speech analysis libraries for audio data. This combination allows for exploring the relationship between textual representations of speech and actual speech sounds, aiding in the detection and analysis of diphthongs.
For example, extracting phonetic transcriptions using NLTK
:
import nltk arpabet = nltk.corpus.cmudict.dict() word_phonemes = arpabet['word'][0] # Get the phonemes for 'word' print(word_phonemes)
Pre-trained Models for Diphthong Detection
Pre-trained models, which are trained on large datasets and can be used or fine-tuned for specific tasks, offer a shortcut to developing effective diphthong detection systems. Models trained on speech recognition tasks, such as those available through Hugging Face's
Transformers library, can be adapted for diphthong detection. These models have learned rich representations of speech sounds, including diphthongs, from extensive data, making them highly capable out of the box or with minimal additional training.
Example of loading a pre-trained speech recognition model:
from transformers import Wav2Vec2ForCTC, Wav2Vec2Tokenizer tokenizer = Wav2Vec2Tokenizer.from_pretrained("facebook/wav2vec2-base-960h") model = Wav2Vec2ForCTC.from_pretrained("facebook/wav2vec2-base-960h")
Common Features for Diphthong Detection
Effective diphthong detection hinges on identifying the right features in speech that signal the presence of a diphthong. Commonly used features include:
– Mel Frequency Cepstral Coefficients (MFCCs): Capture the short-term power spectrum of sound, useful for characterizing the unique sound of diphthongs.
– Formants: Peak frequencies in the sound spectrum that are crucial for identifying vowels and their movements, indicative of diphthongs.
– Duration: The length of the sound, as diphthongs tend to have distinctive durations compared to simple vowels or consonants.
– Pitch Contour: The change in pitch over the duration of the sound, which can help distinguish diphthongs from other vowel sounds.
Extracting these features and analyzing them correctly is key to accurately detecting and analyzing diphthongs in speech data.
Related Article: Django 4 Best Practices: Leveraging Asynchronous Handlers for Class-Based Views
Additional Resources
– Detecting Diphthongs in Python using Praat and Pysle
– Using Machine Learning for Diphthong Detection in Python