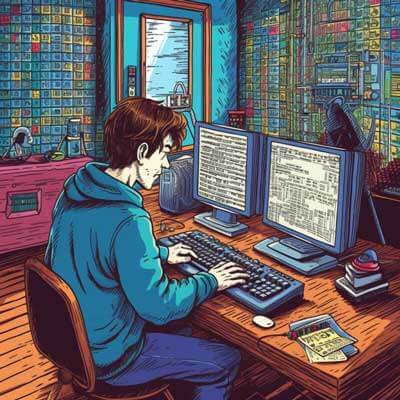
Table of Contents
In Bash, the if
statement allows you to perform conditional execution based on the evaluation of a condition. The and
operator (&&
) is one of the logical operators that can be used in an if
statement to combine multiple conditions. When the and
operator is used, the subsequent commands or actions will only be executed if all the conditions evaluate to true.
Here are two possible ways to use the and
operator in an if
statement in Bash:
Method 1: Using the &&
Operator
The &&
operator can be used to combine multiple conditions in an if
statement. Here's the syntax:
if [ condition1 ] && [ condition2 ] then # commands to be executed if both conditions are true fi
The conditions within the square brackets ([ ]
) can be any valid test conditions in Bash. For example, you can use comparison operators (-eq
, -ne
, -lt
, -gt
, -le
, -ge
) to compare numbers, or string comparison operators (==
, !=
, <
, >
, -z
, -n
) to compare strings.
Here's an example that demonstrates the usage of the &&
operator in an if
statement:
#!/bin/bash # Check if the file exists and is readable if [ -f "myfile.txt" ] && [ -r "myfile.txt" ] then echo "The file exists and is readable." # Add more commands here if needed else echo "The file does not exist or is not readable." fi
In this example, the if
statement checks if the file "myfile.txt" exists and is readable. If both conditions are true, it prints a message indicating that the file exists and is readable. Otherwise, it prints a message indicating that the file does not exist or is not readable.
Related Article: How to Choose the Preferred Bash Shebang in Linux
Method 2: Using Multiple Conditions Separated by Spaces
Alternatively, you can also use multiple conditions separated by spaces in an if
statement. In this case, the subsequent commands or actions will only be executed if all the conditions evaluate to true. Here's the syntax:
if [ condition1 ] && [ condition2 ] then # commands to be executed if both conditions are true fi
Here's an example that demonstrates this approach:
#!/bin/bash # Check if the current user is root and the system is running on Linux if [ "$(whoami)" == "root" ] && [ "$(uname)" == "Linux" ] then echo "The current user is root and the system is running on Linux." # Add more commands here if needed else echo "Either the current user is not root or the system is not running on Linux." fi
In this example, the if
statement checks if the current user is root
and if the system is running on Linux. If both conditions are true, it prints a message indicating that the current user is root
and the system is running on Linux. Otherwise, it prints a message indicating that either the current user is not root
or the system is not running on Linux.
Best Practices
Related Article: How to Detect if Your Bash Script is Running
When using the and
operator in an if
statement in Bash, consider the following best practices:
1. Use meaningful condition checks: Ensure that the conditions you use in the if
statement accurately reflect the logic you want to implement. Using descriptive condition checks can make your code more readable and maintainable.
2. Enclose conditions in double quotes: It is a good practice to enclose string conditions in double quotes ("
) to handle cases where the condition might contain special characters or spaces. For example: if [ "$var" == "value" ] && [ "$var2" == "another value" ]
.
3. Use indentation for readability: Properly indenting the commands inside the if
statement improves code readability. It helps to clearly identify the block of code that will be executed when the conditions are true.
4. Test your conditions: Before using the and
operator in an if
statement, test each condition separately to ensure that they evaluate as expected. This can help you identify any issues or unexpected behavior.
5. Comment your code: If your conditions are complex or require explanation, consider adding comments to clarify the logic and make it easier for others (and yourself) to understand the code in the future.
Overall, using the and
operator in an if
statement in Bash allows you to combine multiple conditions to perform conditional execution. By following best practices and writing clear, readable code, you can ensure that your Bash scripts are robust and maintainable.