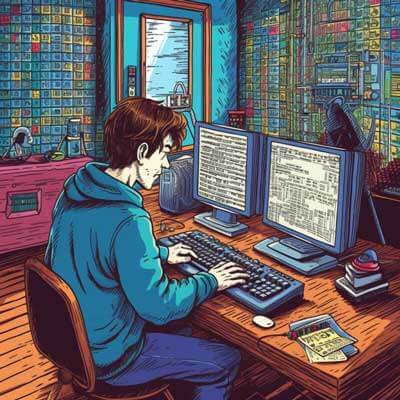
Table of Contents
The integration between Spark and Elasticsearch allows users to perform distributed data processing and analytics on data stored in Elasticsearch. Spark provides a high-level API for interacting with Elasticsearch, making it easy to read and write data from and to Elasticsearch.
When executing a query in Spark that involves Elasticsearch, Spark sends the query to Elasticsearch for processing. Elasticsearch then retrieves the relevant data and returns the results to Spark. This process is known as query passing.
To pass a query from Spark to Elasticsearch, you need to create an instance of the Elasticsearch connector provided by the Elasticsearch-Hadoop project. This connector allows you to read and write data between Spark and Elasticsearch.
Here's an example of how to pass a query to Elasticsearch using Spark:
import org.elasticsearch.spark._ val sparkConf = new SparkConf().setAppName("Spark Elasticsearch") sparkConf.set("es.nodes", "") sparkConf.set("es.port", "") sparkConf.set("es.query", "{\"query\":{\"match\":{\"field\":\"value\"}}}") val sparkContext = new SparkContext(sparkConf) val rdd = sparkContext.esRDD("/")
In this example, we set the Elasticsearch node and port using the es.nodes
and es.port
configuration properties. We also specify the query using the es.query
property. Finally, we read the data from Elasticsearch using the esRDD
method.
Data analysis in Spark Elasticsearch integration
One of the key use cases of Spark Elasticsearch integration is data analysis. Spark provides useful tools and libraries for performing data analysis tasks, such as querying, filtering, aggregating, and visualizing data.
With Spark Elasticsearch integration, you can leverage the querying capabilities of Elasticsearch to retrieve relevant data for analysis. Spark allows you to perform complex data transformations and aggregations on the retrieved data using its DataFrame API or RDD API.
Here's an example of how to perform data analysis using Spark Elasticsearch integration:
import org.elasticsearch.spark._ val sparkConf = new SparkConf().setAppName("Spark Elasticsearch") sparkConf.set("es.nodes", "") sparkConf.set("es.port", "") val sparkSession = SparkSession.builder().config(sparkConf).getOrCreate() val df = sparkSession.read.format("org.elasticsearch.spark.sql").load("/") val filteredData = df.filter("age > 30") val aggregatedData = filteredData.groupBy("gender").avg("salary") aggregatedData.show()
In this example, we read the data from Elasticsearch using the org.elasticsearch.spark.sql
data source format. We then filter the data to only include records where the age is greater than 30. Finally, we group the data by gender and calculate the average salary for each gender.
Related Article: What is Test-Driven Development? (And How To Get It Right)
Elasticsearch as a search engine
Elasticsearch is widely used as a search engine due to its useful search capabilities and near real-time performance. It allows you to index and search structured and unstructured data efficiently.
Elasticsearch uses inverted indexes to store and retrieve data quickly. It supports various types of queries, including full-text search, term queries, phrase queries, and fuzzy queries. It also provides advanced features, such as relevance scoring, highlighting, and suggestions.
Here's an example of performing a full-text search in Elasticsearch:
GET /my-index/_search { "query": { "match": { "message": "quick brown fox" } } }
This query searches for documents in the "my-index" index that contain the phrase "quick brown fox" in the "message" field.
Importance of data indexing in Elasticsearch
Data indexing is a crucial step in Elasticsearch as it determines how efficiently data can be searched and retrieved. Elasticsearch uses inverted indexes to index data, which allows for fast and efficient full-text search.
When indexing data in Elasticsearch, you define the mapping, which determines the structure and type of the data. The mapping includes information about the fields, their data types, and any specific settings or analyzers to be applied.
Here's an example of defining a mapping in Elasticsearch:
PUT /my-index { "mappings": { "properties": { "title": { "type": "text" }, "author": { "type": "keyword" }, "publish_date": { "type": "date" } } } }
In this example, we define a mapping for the "my-index" index with three fields: "title" of type "text", "author" of type "keyword", and "publish_date" of type "date". The mapping specifies the data type of each field, which affects how the data is indexed and searched.
Real-time analytics in Spark Elasticsearch integration
Spark Elasticsearch integration enables real-time analytics by allowing you to process and analyze data as it is ingested into Elasticsearch. This is particularly useful for scenarios where you need to monitor and analyze streaming data in real-time.
Spark provides a streaming API that allows you to consume data from various sources, such as Kafka, Flume, and Elasticsearch. You can use this API to process data in real-time and perform analytics on the incoming data.
Here's an example of performing real-time analytics using Spark Elasticsearch integration:
import org.apache.spark.streaming._ import org.elasticsearch.spark.streaming._ val sparkConf = new SparkConf().setAppName("Spark Elasticsearch Streaming") sparkConf.set("es.nodes", "") sparkConf.set("es.port", "") val streamingContext = new StreamingContext(sparkConf, Seconds(1)) val stream = streamingContext.receiverStream(new ElasticsearchReceiver(Map("es.resource" -> "/"))) val wordCounts = stream.flatMap(_.split(" ")).map((_, 1)).reduceByKey(_ + _) wordCounts.print() streamingContext.start() streamingContext.awaitTermination()
In this example, we create a streaming context with a batch interval of 1 second. We then create a receiver stream from Elasticsearch using the ElasticsearchReceiver
class. We split the data into words, count the occurrences of each word, and print the results in real-time.
Related Article: How To Use Enctype Multipart Form Data
Achieving data visualization in Spark Elasticsearch integration
Data visualization is an essential part of data analysis and allows you to gain insights from your data more effectively. Spark Elasticsearch integration provides various options for visualizing data, including integration with popular visualization libraries, such as Matplotlib and Plotly.
You can use Spark to retrieve and process the data from Elasticsearch and then pass the processed data to a visualization library for creating charts, graphs, and other visual representations.
Here's an example of visualizing data using Spark Elasticsearch integration and Matplotlib:
import org.elasticsearch.spark._ val sparkConf = new SparkConf().setAppName("Spark Elasticsearch") sparkConf.set("es.nodes", "") sparkConf.set("es.port", "") val sparkSession = SparkSession.builder().config(sparkConf).getOrCreate() val df = sparkSession.read.format("org.elasticsearch.spark.sql").load("/") val filteredData = df.filter("age > 30") val aggregatedData = filteredData.groupBy("gender").count() val matplotlibData = aggregatedData.collect().map(row => (row.getString(0), row.getLong(1))) import matplotlib.pyplot as plt val genders = matplotlibData.map(_._1) val counts = matplotlibData.map(_._2) plt.bar(genders, counts) plt.show()
In this example, we read the data from Elasticsearch, filter it to only include records where the age is greater than 30, and group it by gender. We then collect the results and pass them to Matplotlib for creating a bar chart.
Handling large datasets in Spark Elasticsearch integration
Spark Elasticsearch integration is designed to handle large datasets efficiently by leveraging the distributed computing capabilities of Spark and the scalability of Elasticsearch.
When working with large datasets, it is important to consider the following best practices:
1. Partitioning: Partition your data in Elasticsearch to distribute it across multiple nodes. This allows Spark to process the data in parallel, improving performance. You can use the Elasticsearch API or the Elasticsearch-Hadoop connector to partition your data.
2. Caching: If you need to access the same data multiple times, consider caching it in Spark's memory. This avoids the need to fetch the data from Elasticsearch each time it is accessed, resulting in significant performance improvements.
3. Filtering: Use filtering to reduce the amount of data processed by Spark. Elasticsearch supports various types of filters, such as term filters, range filters, and bool filters. By filtering the data before retrieving it, you can reduce the amount of network traffic and improve performance.
4. Aggregation: Use aggregations in Elasticsearch to pre-aggregate data before retrieving it with Spark. This can significantly reduce the amount of data transferred from Elasticsearch to Spark, improving performance.
5. Schema optimization: Optimize the schema of your data in Elasticsearch to reduce the storage space and improve query performance. This includes choosing appropriate data types, defining mappings, and using appropriate analyzers.
Advantages of distributed computing for big data processing
Distributed computing offers several advantages for big data processing:
1. Scalability: Distributed computing allows you to scale your data processing tasks horizontally by adding more machines to your cluster. This enables you to process large datasets efficiently and handle increasing workloads.
2. Fault tolerance: Distributed computing systems, such as Apache Spark, are designed to handle failures gracefully. They automatically recover from failures and continue processing without data loss. This ensures the reliability of your data processing tasks.
3. Parallel processing: Distributed computing systems divide data into partitions and process them in parallel across multiple machines. This allows you to process large datasets faster by utilizing the computing power of multiple machines simultaneously.
4. Flexibility: Distributed computing systems provide a flexible programming model that allows you to express complex data processing tasks easily. They provide high-level APIs and libraries for various data processing tasks, such as batch processing, stream processing, and machine learning.
Additional Resources
- Integrating Elasticsearch with Apache Spark