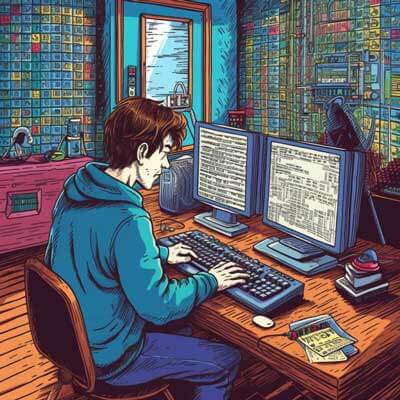
- What is React?
- How does component state work?
- What are some popular global state management solutions?
- What is Redux and how does it manage state?
- What is the Context API in React and how does it help with state management?
- What is the useState hook in React and how does it manage state?
- What is the useEffect hook in React and how does it manage state?
- What is the useReducer hook in React and how does it manage state?
- What is immutable state and why is it important in state management?
- What are some popular state management libraries for React?
What is React?
React is a popular JavaScript library for building user interfaces. It was developed by Facebook and is widely used by developers to create interactive and dynamic web applications. React follows a component-based architecture where the UI is broken down into reusable components. These components can be composed together to create complex user interfaces.
React makes use of a virtual DOM (Document Object Model) which allows it to efficiently update and render the UI. When the state of a component changes, React compares the virtual DOM with the actual DOM and only updates the necessary parts, resulting in a more efficient and performant application.
Related Article: Accessing Parent State from Child Components in Next.js
How does component state work?
In React, component state is an object that holds data related to a particular component. It represents the mutable values of a component and can be updated using the setState
method. When the state of a component changes, React automatically re-renders the component and updates the UI to reflect the new state.
Component state is typically used to store and manage data that can change over time, such as user input or data fetched from an API. It is a local state that belongs to a specific component and is not shared with other components.
Here’s an example of a simple React component that uses state:
import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button>Increment</button> </div> ); }; export default Counter;
In this example, the Counter
component has a state variable called count
initialized to 0 using the useState
hook. The increment
function updates the count
state by incrementing it by 1 when the button is clicked. The count
state is then rendered in the UI.
What are some popular global state management solutions?
In React, managing global state can become challenging as the application grows in complexity. There are several popular state management solutions available that can help handle global state more effectively. Some of these solutions include:
– Redux: Redux is a predictable state container for JavaScript apps. It provides a centralized store to manage the state of an application, making it easier to access and update the state from any component.
– MobX: MobX is a simple and scalable state management library. It uses observables and reactions to automatically track and update state changes, making it easy to keep the UI in sync with the underlying data.
– Zustand: Zustand is a lightweight state management library that uses a hook-based API. It provides a simple and intuitive way to manage state without the need for complex setup or boilerplate code.
– Recoil: Recoil is a state management library developed by Facebook. It uses atoms and selectors to manage state and provides an efficient way to handle complex state dependencies.
These are just a few examples of popular global state management solutions in React. The choice of which one to use depends on the specific needs and requirements of your application.
What is Redux and how does it manage state?
Redux is a popular JavaScript library for managing the state of an application. It follows the principles of Flux architecture and provides a predictable state container that can be used with any JavaScript framework, including React.
At the core of Redux is the concept of a single immutable state tree. The state of an application is stored in a single JavaScript object called the store. Actions are dispatched to update the state, and reducers are used to handle these actions and update the state accordingly.
Here’s an example of how Redux manages state in a React application:
// store.js import { createStore } from 'redux'; const initialState = { count: 0 }; const reducer = (state = initialState, action) => { switch (action.type) { case 'INCREMENT': return { ...state, count: state.count + 1 }; case 'DECREMENT': return { ...state, count: state.count - 1 }; default: return state; } }; const store = createStore(reducer); export default store;
// Counter.js import React from 'react'; import { useSelector, useDispatch } from 'react-redux'; const Counter = () => { const count = useSelector(state => state.count); const dispatch = useDispatch(); const increment = () => { dispatch({ type: 'INCREMENT' }); }; const decrement = () => { dispatch({ type: 'DECREMENT' }); }; return ( <div> <p>Count: {count}</p> <button>Increment</button> <button>Decrement</button> </div> ); }; export default Counter;
In this example, the Redux store is created using the createStore
function from the Redux library. The initial state is defined as an object with a count
property set to 0. The reducer function handles the INCREMENT
and DECREMENT
actions and updates the state accordingly.
The Counter
component uses the useSelector
hook to access the count
state from the Redux store, and the useDispatch
hook to dispatch actions. When the increment or decrement buttons are clicked, the corresponding action is dispatched, and the state is updated accordingly.
Related Article: How to Integrate Redux with Next.js
What is the Context API in React and how does it help with state management?
The Context API is a built-in feature in React that allows for global state management. It provides a way to pass data through the component tree without having to pass props manually at every level.
The Context API consists of two main components: the provider and the consumer. The provider component is responsible for defining the data that needs to be shared, while the consumer component is used to access the shared data.
Here’s an example of how the Context API can be used for state management:
// ThemeContext.js import React, { createContext, useState } from 'react'; export const ThemeContext = createContext(); export const ThemeProvider = ({ children }) => { const [theme, setTheme] = useState('light'); const toggleTheme = () => { setTheme(theme === 'light' ? 'dark' : 'light'); }; return ( {children} ); };
// App.js import React from 'react'; import { ThemeProvider } from './ThemeContext'; import Header from './Header'; import Content from './Content'; const App = () => { return ( <div> <Header /> </div> ); }; export default App;
// Header.js import React, { useContext } from 'react'; import { ThemeContext } from './ThemeContext'; const Header = () => { const { theme, toggleTheme } = useContext(ThemeContext); return ( <header> <h1>My App</h1> <button> {theme === 'light' ? 'Switch to Dark Theme' : 'Switch to Light Theme'} </button> </header> ); }; export default Header;
In this example, the ThemeProvider
component defines the theme state and the toggleTheme
function using the useState
hook. It provides this state and function to its child components using the ThemeContext.Provider
component.
The Header
component consumes the theme
and toggleTheme
values from the ThemeContext
using the useContext
hook. When the button is clicked, the toggleTheme
function is called to update the theme state.
The Context API simplifies the process of sharing state between components and eliminates the need for prop drilling. It is particularly useful for managing global state in larger applications.
What is the useState hook in React and how does it manage state?
The useState
hook is a built-in hook in React that allows functional components to manage state. It provides a way to add stateful behavior to functional components without the need for a class component.
The useState
hook takes an initial value as its argument and returns an array with two elements: the current state value and a function to update the state. By calling this update function, React will re-render the component and update the UI to reflect the new state.
Here’s an example of how the useState
hook can be used to manage state in a functional component:
import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button>Increment</button> </div> ); }; export default Counter;
In this example, the Counter
component uses the useState
hook to initialize a state variable called count
with an initial value of 0. The increment
function updates the count
state by incrementing it by 1 when the button is clicked. The count
state is then rendered in the UI.
The useState
hook simplifies state management in functional components and provides a more concise and readable syntax compared to class components.
What is the useEffect hook in React and how does it manage state?
The useEffect
hook is a built-in hook in React that allows functional components to perform side effects. Side effects include data fetching, subscriptions, or manually changing the DOM, among others.
The useEffect
hook takes two arguments: a function that contains the side effect code, and an optional array of dependencies. The side effect code is executed after the component has rendered, and subsequent re-renders if the dependencies have changed.
Here’s an example of how the useEffect
hook can be used to manage state and perform side effects in a functional component:
import React, { useState, useEffect } from 'react'; const Counter = () => { const [count, setCount] = useState(0); useEffect(() => { document.title = `Count: ${count}`; }, [count]); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button>Increment</button> </div> ); }; export default Counter;
In this example, the Counter
component uses the useEffect
hook to update the document title whenever the count
state changes. The useEffect
hook is called after the component has rendered, and every time the count
state changes.
The useEffect
hook allows functional components to perform side effects in a declarative way, without the need for class components and lifecycle methods.
Related Article: Next.js Bundlers Quick Intro
What is the useReducer hook in React and how does it manage state?
The useReducer
hook is a built-in hook in React that allows functional components to manage state using a reducer function. It provides an alternative to the useState
hook for managing more complex state logic.
The useReducer
hook takes a reducer function and an initial state as its arguments, and returns an array with two elements: the current state value and a dispatch function to update the state. The reducer function takes the current state and an action as its arguments, and returns the new state.
Here’s an example of how the useReducer
hook can be used to manage state in a functional component:
import React, { useReducer } from 'react'; const initialState = { count: 0 }; const reducer = (state, action) => { switch (action.type) { case 'INCREMENT': return { count: state.count + 1 }; case 'DECREMENT': return { count: state.count - 1 }; default: return state; } }; const Counter = () => { const [state, dispatch] = useReducer(reducer, initialState); const increment = () => { dispatch({ type: 'INCREMENT' }); }; const decrement = () => { dispatch({ type: 'DECREMENT' }); }; return ( <div> <p>Count: {state.count}</p> <button>Increment</button> <button>Decrement</button> </div> ); }; export default Counter;
In this example, the Counter
component uses the useReducer
hook to manage the state of the count
property. The reducer
function handles the INCREMENT
and DECREMENT
actions and returns the new state accordingly.
The useReducer
hook provides a way to manage more complex state logic and state transitions in a more organized and maintainable manner.
What is immutable state and why is it important in state management?
Immutable state refers to state values that cannot be modified once they are created. Instead of directly modifying the state, immutable state encourages creating new state objects with the desired changes.
Immutable state is important in state management for several reasons:
– Predictability: Immutable state ensures that state changes are predictable and easy to reason about. Since the state cannot be modified directly, it prevents unexpected side effects and makes it easier to track state changes.
– Performance: Immutable state can improve performance by allowing efficient equality checks. Since the state objects are immutable, it is possible to compare them by reference instead of performing deep equality checks, which can be more expensive.
– Time-travel debugging: Immutable state is crucial for implementing time-travel debugging, a useful debugging technique that allows developers to step back and forth through the application’s state history. With immutable state, it is easier to track and store the previous states of the application.
– Component reusability: Immutable state makes it easier to reuse components in different contexts without worrying about unintended state modifications. Components that rely on immutable state can be safely used in different parts of the application without causing unexpected side effects.
To achieve immutable state in JavaScript, developers often make use of libraries such as Immutable.js, Immer, or follow certain patterns and best practices to ensure immutability.
What are some popular state management libraries for React?
React has a vibrant ecosystem of state management libraries that provide different approaches and solutions for managing state in React applications. Some of the popular state management libraries for React include:
– Redux: Redux is one of the most widely used state management libraries in the React ecosystem. It follows the principles of Flux architecture and provides a predictable state container that can be used with any JavaScript framework. Redux offers a centralized store and a unidirectional data flow, making it suitable for managing complex and global state.
– MobX: MobX is a simple and scalable state management library. It uses observables and reactions to automatically track and update state changes, making it easy to keep the UI in sync with the underlying data. MobX provides a more flexible and intuitive approach to state management compared to Redux.
– Zustand: Zustand is a lightweight state management library that uses a hook-based API. It provides a simple and intuitive way to manage state without the need for complex setup or boilerplate code. Zustand leverages the power of React hooks and offers a minimalistic approach to state management.
– Recoil: Recoil is a state management library developed by Facebook. It uses atoms and selectors to manage state and provides an efficient way to handle complex state dependencies. Recoil offers a flexible and scalable approach to state management, making it suitable for large-scale applications.
– Context API: The Context API is a built-in feature in React that allows for global state management. It provides a way to pass data through the component tree without having to pass props manually at every level. While the Context API is not as feature-rich as other state management libraries, it can be a good choice for simpler applications or when a lightweight solution is needed.
These are just a few examples of popular state management libraries for React. The choice of which library to use depends on the specific needs and requirements of the application. It is important to consider factors such as complexity, performance, and developer experience when selecting a state management library.