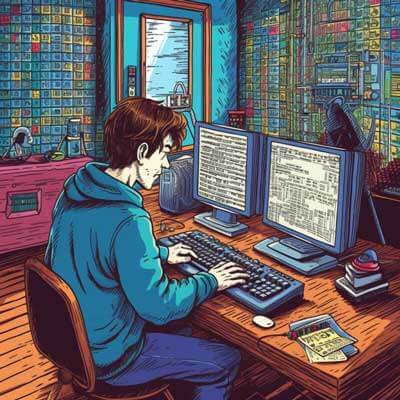
Table of Contents
Next.js dynamic pages
Next.js is a popular framework for building React applications. One of the key features of Next.js is its ability to create dynamic pages. Dynamic pages are pages that are generated on the server or at build time, and the content of the page can change based on user input or data from an external source.
To create dynamic pages in Next.js, you can use the dynamic routing feature. This allows you to define a page template and specify dynamic parameters in the URL. These parameters can then be used to fetch data from an API or a database and render the page with the dynamic content.
Here's an example of how you can create a dynamic page in Next.js:
1. Create a new file called pages/post/[id].js
. This will be the template for the dynamic page.
2. In the [id].js
file, import the useRouter
hook from the next/router
module.
3. Use the useRouter
hook to get the value of the id
parameter from the URL.
4. Fetch the data for the specific post using the id
parameter.
5. Render the content of the post on the page.
Here's an example code snippet:
import { useRouter } from 'next/router'; const Post = () => { const router = useRouter(); const { id } = router.query; // Fetch data for the specific post using the id parameter return ( <div> <h1>Post: {id}</h1> {/* Render the content of the post */} </div> ); }; export default Post;
With this setup, you can create dynamic pages in Next.js that can display different content based on the value of the id
parameter in the URL.
Related Article: How To Check If a Key Exists In a Javascript Object
Next.js static pages
In addition to dynamic pages, Next.js also allows you to create static pages. Static pages are pre-rendered at build time and served as HTML files to the client. This approach offers several benefits, such as improved performance and better SEO.
To create static pages in Next.js, you can use the getStaticProps
function. This function runs at build time and fetches the data needed to render the page. The fetched data is then passed as props to the page component, which can be used to render the content of the page.
Here's an example of how you can create a static page in Next.js:
1. Create a new file called pages/about.js
. This will be the static page.
2. In the about.js
file, export an async function called getStaticProps
.
3. Inside the getStaticProps
function, fetch the data needed to render the page.
4. Return the fetched data as props.
5. Create a React component called About
and use the fetched data to render the content of the page.
Here's an example code snippet:
export async function getStaticProps() { // Fetch the data needed to render the page return { props: { // Pass the fetched data as props }, }; } const About = ({ data }) => { // Render the content of the page using the data prop return ( <div> <h1>About</h1> {/* Render the content of the page */} </div> ); }; export default About;
With this setup, Next.js will pre-render the About
page at build time and serve it as an HTML file to the client. The fetched data will be available as props in the About
component, allowing you to render the content of the page.
React server-side rendering
Next.js leverages React's server-side rendering (SSR) capabilities to provide server-rendered React applications. SSR allows you to render React components on the server before sending them to the client, which improves performance and provides better SEO.
When a user requests a page in a Next.js application, the server executes the code for that page and generates the HTML for the initial render. This HTML, along with the necessary JavaScript and CSS files, is then sent to the client, where it is hydrated into a fully functioning React application.
React's SSR is achieved through the ReactDOMServer
module, which provides a method called renderToString
that converts a React component into its HTML representation. Next.js uses this method to generate the initial HTML for each page during server-side rendering.
Here's an example of how server-side rendering works in Next.js:
1. Create a React component that represents the page you want to render.
2. Use the ReactDOMServer.renderToString
method to convert the component into HTML.
3. Send the generated HTML to the client.
Here's an example code snippet:
import ReactDOMServer from 'react-dom/server'; const MyComponent = () => { return <h1>Hello, world!</h1>; }; const html = ReactDOMServer.renderToString(); console.log(html);
In this example, the MyComponent
component is rendered to HTML using the ReactDOMServer.renderToString
method. The generated HTML is then logged to the console.
Next.js SSR
Next.js provides built-in server-side rendering (SSR) capabilities, which allow you to render React components on the server and send the rendered HTML to the client. SSR improves performance by reducing the amount of JavaScript that needs to be executed on the client and provides better SEO by serving fully rendered HTML to search engines.
To enable SSR in Next.js, you can use the getServerSideProps
function. This function runs on the server for each incoming request and fetches the data needed to render the page. The fetched data is then passed as props to the page component, which can be used to render the content of the page.
Here's an example of how you can enable SSR in Next.js:
1. Create a new file called pages/post/[id].js
. This will be the template for the SSR page.
2. In the [id].js
file, export an async function called getServerSideProps
.
3. Inside the getServerSideProps
function, fetch the data needed to render the page.
4. Return the fetched data as props.
5. Create a React component called Post
and use the fetched data to render the content of the page.
Here's an example code snippet:
export async function getServerSideProps(context) { // Fetch the data needed to render the page return { props: { // Pass the fetched data as props }, }; } const Post = ({ data }) => { // Render the content of the page using the data prop return ( <div> <h1>Post</h1> {/* Render the content of the page */} </div> ); }; export default Post;
With this setup, Next.js will execute the getServerSideProps
function on the server for each incoming request and fetch the data needed to render the page. The fetched data will be available as props in the Post
component, allowing you to render the content of the page.
Related Article: How To Check Checkbox Status In jQuery
Next.js file-based routing
Next.js uses a file-based routing system, which means that each page in your application corresponds to a file in the pages
directory. The file name determines the URL of the page.
For example, if you have a file called pages/about.js
, the URL of the corresponding page will be /about
.
Next.js also supports nested routes by creating subdirectories within the pages
directory. For example, if you have a file called pages/products/index.js
, the URL of the corresponding page will be /products
.
Here's an example of how you can create a file-based route in Next.js:
1. Create a new file with the desired URL path in the pages
directory.
2. Export a React component from the file.
3. The file name and directory structure determine the URL path of the page.
Here's an example code snippet:
const About = () => { return ( <div> <h1>About</h1> {/* Render the content of the page */} </div> ); }; export default About;
In this example, the file pages/about.js
exports a React component called About
. This page will be accessible at the URL path /about
.
Next.js file-based routing provides a simple and intuitive way to define the structure of your application and create new pages.
Next.js getStaticProps
Next.js provides the getStaticProps
function, which allows you to fetch data at build time and pass it as props to your page components. This function is used for static site generation (SSG), where the HTML for each page is generated at build time and served as a static file.
To use getStaticProps
, you need to export it as an async function from your page component file. Inside the function, you can fetch data from an API or a database and return it as the props
object. The data will then be available as props in your page component.
Here's an example of how you can use getStaticProps
in Next.js:
1. Create a new file for your page component in the pages
directory.
2. Export an async function called getStaticProps
from the file.
3. Inside the getStaticProps
function, fetch the data needed for your page.
4. Return the fetched data as the props
object.
Here's an example code snippet:
export async function getStaticProps() { // Fetch the data needed for your page return { props: { // Pass the fetched data as props }, }; } const MyPage = ({ data }) => { // Render the content of your page using the data prop return ( <div> <h1>My Page</h1> {/* Render the content of your page */} </div> ); }; export default MyPage;
In this example, the getStaticProps
function fetches the data needed for the page and returns it as the props
object. The fetched data is then available as the data
prop in the MyPage
component, allowing you to render the content of the page.
Using getStaticProps
in Next.js enables static site generation and improves performance by pre-rendering pages at build time.
Next.js getServerSideProps
Next.js provides the getServerSideProps
function, which allows you to fetch data on the server for each request and pass it as props to your page components. This function is used for server-side rendering (SSR), where the HTML for each page is generated on the server and sent to the client.
To use getServerSideProps
, you need to export it as an async function from your page component file. Inside the function, you can fetch data from an API or a database and return it as the props
object. The data will then be available as props in your page component.
Here's an example of how you can use getServerSideProps
in Next.js:
1. Create a new file for your page component in the pages
directory.
2. Export an async function called getServerSideProps
from the file.
3. Inside the getServerSideProps
function, fetch the data needed for your page.
4. Return the fetched data as the props
object.
Here's an example code snippet:
export async function getServerSideProps() { // Fetch the data needed for your page return { props: { // Pass the fetched data as props }, }; } const MyPage = ({ data }) => { // Render the content of your page using the data prop return ( <div> <h1>My Page</h1> {/* Render the content of your page */} </div> ); }; export default MyPage;
In this example, the getServerSideProps
function fetches the data needed for the page on the server for each request and returns it as the props
object. The fetched data is then available as the data
prop in the MyPage
component, allowing you to render the content of the page.
Using getServerSideProps
in Next.js enables server-side rendering and allows you to fetch data at runtime for each request.
Next.js API routes
Next.js provides a built-in API routing system, which allows you to create serverless API endpoints within your Next.js application. API routes are defined as separate files in the pages/api
directory and can be used to handle HTTP requests and return JSON responses.
To create an API route in Next.js, you need to create a file in the pages/api
directory with the desired URL path. Inside the file, you export a default async function that handles the request and returns the response.
Here's an example of how you can create an API route in Next.js:
1. Create a new file in the pages/api
directory with the desired URL path, e.g., pages/api/hello.js
.
2. Export a default async function from the file.
3. Inside the function, handle the request and return the response.
Here's an example code snippet:
export default async function handler(req, res) { const { name } = req.query; // Handle the request and return the response res.status(200).json({ message: `Hello, ${name}!` }); }
In this example, the API route handles a GET request with a name
query parameter and returns a JSON response with a greeting message.
You can use API routes in Next.js to build serverless APIs that can be deployed alongside your Next.js application.
Related Article: How to Check for Null Values in Javascript
Next.js data fetching
Next.js provides multiple ways to fetch data for your pages, including static site generation (SSG), server-side rendering (SSR), and client-side rendering (CSR). The choice of data fetching method depends on your specific requirements and the type of data you need to fetch.
Here are the different data fetching methods available in Next.js:
1. Static site generation (SSG): Use the getStaticProps
function to fetch data at build time and pre-render the pages as static files. This method is suitable for data that doesn't change frequently and can be pre-rendered.
2. Server-side rendering (SSR): Use the getServerSideProps
function to fetch data on the server for each request and render the page with the fetched data. This method is suitable for data that needs to be fetched at runtime and can't be pre-rendered.
3. Client-side rendering (CSR): Use client-side data fetching techniques, such as the useEffect
hook or third-party libraries like axios
or fetch
, to fetch data on the client side. This method is suitable for data that needs to be fetched dynamically based on user interaction.
Here's an example of how you can fetch data using static site generation (SSG) in Next.js:
export async function getStaticProps() { // Fetch the data needed for your page return { props: { // Pass the fetched data as props }, }; }
And here's an example of how you can fetch data using server-side rendering (SSR) in Next.js:
export async function getServerSideProps() { // Fetch the data needed for your page return { props: { // Pass the fetched data as props }, }; }
In both examples, you can use any data fetching library or API to fetch the data you need. The fetched data will be available as props in your page component, allowing you to render the content of the page.
Next.js provides flexible data fetching options to cater to different use cases and requirements. You can choose the appropriate method based on your specific needs.
Next.js page generation
Next.js provides various methods for generating pages, including static site generation (SSG), server-side rendering (SSR), and client-side rendering (CSR). The choice of page generation method depends on your specific requirements and the type of content you want to display.
Static site generation (SSG) is the default page generation method in Next.js. With SSG, pages are generated at build time and served as static files. This approach offers improved performance and better SEO.
To use static site generation in Next.js, you can use the getStaticProps
function. This function runs at build time and fetches the data needed to render the page. The fetched data is then passed as props to the page component, which can be used to render the content of the page.
Here's an example of how you can generate a page using static site generation in Next.js:
1. Create a new file for your page component in the pages
directory.
2. Export an async function called getStaticProps
from the file.
3. Inside the getStaticProps
function, fetch the data needed for the page.
4. Return the fetched data as the props
object.
Here's an example code snippet:
export async function getStaticProps() { // Fetch the data needed for your page return { props: { // Pass the fetched data as props }, }; } const MyPage = ({ data }) => { // Render the content of your page using the data prop return ( <div> <h1>My Page</h1> {/* Render the content of your page */} </div> ); }; export default MyPage;
With this setup, Next.js will generate the HTML for the MyPage
component at build time and serve it as a static file. The fetched data will be available as props in the MyPage
component, allowing you to render the content of the page.
Next.js page generation provides a flexible and efficient way to generate pages with different content types.
What are the benefits of using static pages in Next.js?
Using static pages in Next.js offers several benefits, including improved performance, better SEO, and simpler deployment.
1. Improved performance: Static pages are pre-rendered at build time and served as HTML files to the client. This eliminates the need to generate the page on each request, resulting in faster page load times and a smoother user experience.
2. Better SEO: Search engines can easily crawl and index static HTML pages, which improves the visibility of your website in search engine results. Pre-rendered static pages also provide metadata that can be used by search engines to understand the content of your pages.
3. Simpler deployment: Static pages can be deployed to a variety of hosting platforms, including static site hosting services like Vercel and Netlify. Deploying static pages is often simpler and more cost-effective compared to deploying server-side rendered or client-side rendered applications.
4. Lower server load: Static pages do not require server-side processing for each request, which reduces the load on your server infrastructure. This is especially beneficial for high-traffic websites that need to handle a large number of concurrent requests.
5. Offline support: Static pages can be cached by content delivery networks (CDNs) and served to users even when they are offline. This provides a seamless browsing experience and ensures that your content is always accessible.
Using static pages in Next.js is a useful technique for optimizing the performance, SEO, and deployment of your web applications.
What is file-based routing in Next.js?
Next.js uses a file-based routing system, which means that each page in your application corresponds to a file in the pages
directory. The file name determines the URL of the page.
For example, if you have a file called pages/about.js
, the URL of the corresponding page will be /about
.
Next.js also supports nested routes by creating subdirectories within the pages
directory. For example, if you have a file called pages/products/index.js
, the URL of the corresponding page will be /products
.
File-based routing provides a simple and intuitive way to define the structure of your application and create new pages. It eliminates the need for manual routing configuration and makes it easy to organize your codebase.
Here's an example of how you can create a file-based route in Next.js:
1. Create a new file with the desired URL path in the pages
directory.
2. Export a React component from the file.
3. The file name and directory structure determine the URL path of the page.
Here's an example code snippet:
const About = () => { return ( <div> <h1>About</h1> {/* Render the content of the page */} </div> ); }; export default About;
In this example, the file pages/about.js
exports a React component called About
. This page will be accessible at the URL path /about
.
File-based routing in Next.js simplifies the process of creating and organizing pages in your application.
Related Article: How to Generate a GUID/UUID in JavaScript
How to fetch data in Next.js?
Next.js provides multiple ways to fetch data for your pages, including static site generation (SSG), server-side rendering (SSR), and client-side rendering (CSR). The choice of data fetching method depends on your specific requirements and the type of data you need to fetch.
To fetch data in Next.js, you can use the getStaticProps
, getServerSideProps
, or client-side data fetching techniques.
Here's an example of how you can fetch data using static site generation (SSG) in Next.js:
export async function getStaticProps() { // Fetch the data needed for your page return { props: { // Pass the fetched data as props }, }; }
And here's an example of how you can fetch data using server-side rendering (SSR) in Next.js:
export async function getServerSideProps() { // Fetch the data needed for your page return { props: { // Pass the fetched data as props }, }; }
In both examples, you can use any data fetching library or API to fetch the data you need. The fetched data will be available as props in your page component, allowing you to render the content of the page.
For client-side data fetching, you can use techniques like the useEffect
hook or third-party libraries like axios
or fetch
to fetch data on the client side.
Next.js provides flexible options for fetching data based on your specific needs and requirements.
What are the differences between getStaticProps and getServerSideProps in Next.js?
Next.js provides two methods for fetching data: getStaticProps
and getServerSideProps
. The main difference between these two methods lies in when and where the data fetching occurs.
getStaticProps
is used for static site generation (SSG) and fetches data at build time. The data is pre-rendered into HTML and served as static files. This method is suitable for data that doesn't change frequently and can be pre-rendered.
getServerSideProps
is used for server-side rendering (SSR) and fetches data on the server for each request. The data is fetched at runtime and the page is rendered on the server before being sent to the client. This method is suitable for data that needs to be fetched at runtime and can't be pre-rendered.
Here's a summary of the differences between getStaticProps
and getServerSideProps
:
- getStaticProps
:
- Fetches data at build time
- Pre-renders the page as static HTML
- Ideal for data that doesn't change frequently
- Provides improved performance and better SEO
- Data is fetched once during the build process
- getServerSideProps
:
- Fetches data on the server for each request
- Renders the page on the server before sending it to the client
- Ideal for data that needs to be fetched at runtime
- Provides dynamic content on each request
- Data is fetched on each request
To choose between getStaticProps
and getServerSideProps
, consider the nature of your data and the requirements of your application. If the data can be pre-rendered and doesn't change frequently, getStaticProps
is a good choice. If the data needs to be fetched at runtime or changes frequently, getServerSideProps
is more suitable.
How to create API routes in Next.js?
Next.js provides a built-in API routing system, which allows you to create serverless API endpoints within your Next.js application. API routes are defined as separate files in the pages/api
directory and can be used to handle HTTP requests and return JSON responses.
To create an API route in Next.js, you need to create a file in the pages/api
directory with the desired URL path. Inside the file, you export a default async function that handles the request and returns the response.
Here's a step-by-step guide on how to create API routes in Next.js:
1. Create a new file in the pages/api
directory with the desired URL path, e.g., pages/api/hello.js
.
2. Export a default async function from the file.
3. Inside the function, handle the request and return the response.
Here's an example code snippet:
export default async function handler(req, res) { const { name } = req.query; // Handle the request and return the response res.status(200).json({ message: `Hello, ${name}!` }); }
In this example, the API route handles a GET request with a name
query parameter and returns a JSON response with a greeting message.
You can use API routes in Next.js to build serverless APIs that can be deployed alongside your Next.js application.
Additional Resources
- Next.js - The React Framework