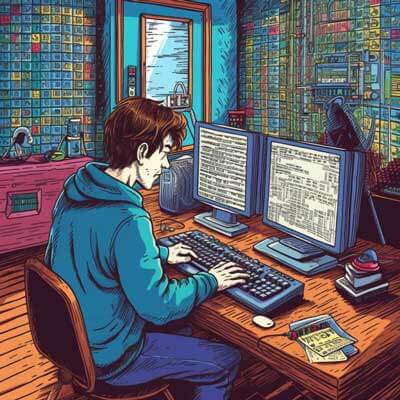
- Creating a Bash Script in Linux – Step-by-Step Guide
- Common Commands Used in Bash Scripting
- Examples of Bash Scripts for Various Use Cases
- Benefits of Writing Bash Scripts
- Debugging Bash Scripts – Techniques and Tools
- Best Practices for Writing Bash Scripts
- Difference Between Bash Script and Shell Script
- Running a Bash Script on Windows – Compatibility and Limitations
- Additional Resources
Creating a Bash Script in Linux – Step-by-Step Guide
Writing a bash script in Linux allows you to automate tasks, perform system administration tasks, and execute commands with ease. Here is a step-by-step guide to help you create a bash script in Linux:
Step 1: Open a text editor
Open your preferred text editor, such as Vim, Nano, or Sublime Text.
Step 2: Start with a shebang
The shebang is the first line of the script and tells the system which interpreter to use. In the case of bash scripts, the shebang is #!/bin/bash.
Example:
#!/bin/bash
Step 3: Add comments and description
Add comments to explain the purpose of the script and any important details. This helps other users understand the script and makes it easier for you to maintain it in the future.
Example:
#!/bin/bash # This is a simple bash script to greet the user
Step 4: Define variables (optional)
If your script requires any variables, you can define them at the beginning of the script. Variables are placeholders for values that can be used throughout the script.
Example:
#!/bin/bash # This is a simple bash script to greet the user name="John"
Step 5: Write the script logic
Now, you can write the actual logic of the script. This can include executing commands, performing calculations, or running conditional statements.
Example:
#!/bin/bash # This is a simple bash script to greet the user name="John" echo "Hello, $name! Welcome to the Linux world!"
Step 6: Save the script
Save the script with a .sh extension, such as myscript.sh, in a convenient location on your Linux system.
Step 7: Make the script executable
To run the script, you need to make it executable. Use the chmod command to set the executable permission.
Example:
chmod +x myscript.sh
Step 8: Run the script
Finally, you can run the script by executing the following command:
Example:
./myscript.sh
Congratulations! You have successfully created and executed your first bash script in Linux. Now, let’s explore some common commands used in bash scripting.
Related Article: How To Echo a Newline In Bash
Common Commands Used in Bash Scripting
Bash scripting offers a wide range of commands that can be used to automate tasks, manipulate files, and interact with the system. Here are some common commands used in bash scripting:
1. echo: The echo command is used to display messages or variables on the terminal.
Example:
#!/bin/bash message="Hello, World!" echo $message
2. read: The read command is used to read input from the user and store it in a variable.
Example:
#!/bin/bash echo "Enter your name:" read name echo "Hello, $name!"
3. if: The if command is used to perform conditional execution based on the result of a condition.
Example:
#!/bin/bash number=10 if [ $number -gt 5 ]; then echo "The number is greater than 5." fi
4. for: The for command is used to iterate over a list of items and perform a set of actions.
Example:
#!/bin/bash fruits=("Apple" "Banana" "Orange") for fruit in "${fruits[@]}"; do echo "I like $fruit." done
5. while: The while command is used to repeatedly execute a set of actions as long as a condition is true.
Example:
#!/bin/bash counter=1 while [ $counter -le 5 ]; do echo "Count: $counter" counter=$((counter+1)) done
These are just a few examples of the common commands used in bash scripting. The bash scripting language provides a rich set of commands and features that can be combined to create useful scripts for various use cases. Speaking of use cases, let’s explore some examples of bash scripts next.
Examples of Bash Scripts for Various Use Cases
Bash scripts can be used for a wide range of purposes, from automating repetitive tasks to managing system configurations. Here are some examples of bash scripts for various use cases:
1. Backup Script:
A backup script can be used to automate the backup process of important files or directories. It can create compressed archives and store them in a specified location.
Example:
#!/bin/bash # Backup script backup_dir="/path/to/backup" source_dir="/path/to/source" date=$(date +"%Y%m%d") filename="backup_$date.tar.gz" tar -czf "$backup_dir/$filename" "$source_dir"
2. Log Analyzer:
A log analyzer script can parse log files and extract useful information, such as error messages or specific patterns. It can generate reports or trigger alerts based on the analysis.
Example:
#!/bin/bash # Log analyzer script log_file="/path/to/logfile" grep "ERROR" "$log_file" > error.log grep "WARNING" "$log_file" > warning.log # Perform further analysis on the generated logs
3. System Monitoring:
A system monitoring script can collect and display system information, such as CPU usage, memory usage, and disk usage. It can help in identifying performance bottlenecks or resource-intensive processes.
Example:
#!/bin/bash # System monitoring script cpu_usage=$(top -bn1 | grep "Cpu(s)" | awk '{print $2 + $4}') memory_usage=$(free -m | awk '/Mem/{print $3}') echo "CPU Usage: $cpu_usage%" echo "Memory Usage: $memory_usage MB"
4. Deployment Script:
A deployment script can automate the deployment process of a web application or service. It can pull the latest code from a version control system, build the application, and restart the necessary services.
Example:
#!/bin/bash # Deployment script app_dir="/path/to/app" repo_url="https://github.com/user/repo.git" cd "$app_dir" git pull "$repo_url" npm install pm2 restart app.js
These are just a few examples of the many use cases where bash scripts can be handy. The flexibility and power of bash scripting make it a valuable tool for automating tasks and managing systems. Next, let’s explore the benefits of writing bash scripts.
Benefits of Writing Bash Scripts
Writing bash scripts can bring several benefits to developers and system administrators. Here are some of the advantages of using bash scripts:
1. Automation: Bash scripts allow you to automate repetitive tasks, reducing manual effort and saving time. You can write scripts to perform tasks such as file backups, log analysis, or system configurations, and schedule them to run at specific intervals or events.
2. Simplified Task Execution: With a bash script, you can execute a sequence of commands or actions with a single command. This makes it easier to perform complex tasks that involve multiple steps or require specific configurations.
3. Improved Productivity: By automating tasks and simplifying task execution, bash scripts can significantly improve productivity. Developers and system administrators can focus on more critical and creative work, while routine tasks are handled by scripts.
4. Consistency and Reliability: Bash scripts ensure consistency in task execution by following predefined steps and configurations. This reduces the chances of human error and improves the reliability of tasks performed by scripts.
5. Customization and Flexibility: Bash scripts provide a high level of customization and flexibility. You can tailor scripts to meet specific requirements, adapt them to different environments, and extend their functionality by including conditional statements, loops, and variables.
6. Portability: Bash scripts can run on any Linux distribution, which makes them highly portable. You can write a script on one Linux system and run it on another without modifications, as long as the required dependencies are available.
7. Scalability: Bash scripts can scale to handle complex tasks and large-scale deployments. With proper design and organization, you can develop modular scripts that are easy to maintain and extend as your needs grow.
These are just a few of the benefits that come with writing bash scripts. Next, let’s explore techniques and tools for debugging bash scripts.
Related Article: How to Use If-Else Statements in Shell Scripts
Debugging Bash Scripts – Techniques and Tools
Debugging bash scripts is an essential skill for scriptwriters. It helps identify and fix errors, understand the flow of execution, and optimize script performance. Here are some techniques and tools you can use to debug bash scripts:
1. Echo Statements:
Adding echo statements to your script can help you trace the execution flow and identify potential issues. By displaying the values of variables or the output of commands at various stages, you can pinpoint where things might be going wrong.
Example:
#!/bin/bash # Debugging with echo statements echo "Starting script..." echo "Variables: foo=$foo, bar=$bar" # Rest of the script
2. Error Handling:
Implementing proper error handling in your script can provide useful information when something goes wrong. Use the set -e option at the beginning of your script to exit immediately if any command fails. Additionally, you can use the trap command to catch and handle errors.
Example:
#!/bin/bash # Error handling with set -e and trap set -e trap 'echo "Error: command failed with exit code $?"' ERR # Rest of the script
3. Debugging Mode:
You can enable debugging mode by using the set -x option at the beginning of your script. This will display each command executed, along with its output and any relevant variable values. It helps you understand the flow of execution and identify issues.
Example:
#!/bin/bash # Debugging mode with set -x set -x # Rest of the script
4. Shellcheck:
Shellcheck is a static analysis tool for shell scripts, including bash scripts. It helps identify common issues and potential problems in your script. You can run Shellcheck on your script to get suggestions for improvements and ensure best practices.
Example:
shellcheck myscript.sh
5. Debugging Utilities:
There are several debugging utilities available for bash scripts, such as bashdb and Bash Debugger. These tools provide a more interactive debugging experience with features like breakpoints, stepping through code, and inspecting variables.
Example:
bashdb myscript.sh
These are some of the techniques and tools you can use to debug bash scripts effectively. Debugging is an iterative process, so don’t hesitate to experiment with different approaches and tools to find what works best for you. Next, let’s explore some best practices for writing bash scripts.
Best Practices for Writing Bash Scripts
Writing bash scripts involves following best practices to ensure readability, maintainability, and reliability. Here are some best practices to consider when writing bash scripts:
1. Use Meaningful Variable Names:
Choose descriptive variable names that reflect their purpose and content. This improves code readability and makes it easier to understand and maintain the script.
# Bad x=10 # Good count=10
2. Indentation and Formatting:
Maintain consistent indentation and formatting throughout your script. Use tabs or spaces to align code blocks and separate logical sections. This enhances readability and makes the script easier to navigate.
# Bad if [ $x -gt 5 ]; then echo "Greater than 5." fi # Good if [ $x -gt 5 ]; then echo "Greater than 5." fi
3. Handle Errors and Edge Cases:
Implement proper error handling to handle unexpected conditions and edge cases. Handle errors gracefully by displaying meaningful error messages and exiting the script when necessary.
# Bad command && echo "Success" || echo "Failure" # Good if command; then echo "Success" else echo "Failure" exit 1 fi
4. Use Quotes for Strings:
Enclose strings in quotes to prevent word splitting and preserve whitespace. This ensures that strings with special characters or spaces are treated as a single entity.
# Bad greeting=Hello, World! # Good greeting="Hello, World!"
5. Comment Your Code:
Add comments to explain the purpose, logic, and any important details of your script. Comments improve code understandability and make it easier for others (including your future self) to maintain the script.
# Bad x=10 # assign 10 to x # Good # Assign 10 to x x=10
6. Test Your Scripts:
Before deploying or using your script in a production environment, test it thoroughly. Test different scenarios, edge cases, and inputs to ensure that the script behaves as expected and handles errors appropriately.
7. Use Version Control:
Version control your bash scripts to track changes, collaborate with others, and revert to previous versions if needed. Use a version control system like Git to manage your script’s codebase.
These best practices will help you write clean, maintainable, and reliable bash scripts. Remember to continuously review and improve your scripts based on feedback and evolving requirements. Next, let’s clarify the difference between a bash script and a shell script.
Difference Between Bash Script and Shell Script
The terms “bash script” and “shell script” are often used interchangeably, but they do have a subtle difference. Let’s clarify the distinction between these two terms:
Bash Script:
A bash script specifically refers to a script written in the Bash (Bourne Again SHell) scripting language. Bash is a popular shell and scripting language for Unix-like systems, including Linux. It is an enhanced version of the original Bourne shell (sh) and provides additional features and capabilities.
Shell Script:
A shell script, on the other hand, is a broader term that encompasses scripts written in various shell languages, including Bash. Shell scripts can be written in different shell languages, such as Bash, sh, csh, ksh, and more. The choice of the shell language depends on the specific requirements and the target system.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Running a Bash Script on Windows – Compatibility and Limitations
While bash scripts are primarily associated with Unix-like systems, it is possible to run them on Windows using various methods. Let’s explore the compatibility and limitations of running bash scripts on Windows:
Compatibility:
1. Windows Subsystem for Linux (WSL): WSL allows you to run a Linux environment directly on Windows. It provides compatibility for running bash scripts, as it includes a full-fledged Linux kernel. You can install a Linux distribution, such as Ubuntu, within WSL and run bash scripts seamlessly.
2. Cygwin: Cygwin is a large collection of GNU and Open Source tools that provide functionality similar to a Linux distribution on Windows. It includes a bash shell, allowing you to run bash scripts on Windows. However, some scripts may require modifications due to differences between the Windows and Linux environments.
Limitations:
1. Platform-Specific Commands: Bash scripts that rely on platform-specific commands or utilities may not work as expected on Windows. Windows and Linux have different sets of commands and utilities, so scripts that use Linux-specific tools may need to be modified or replaced with equivalent Windows commands.
2. File Path Differences: Windows and Linux have different file path conventions. Bash scripts that reference file paths using Linux-style paths (e.g., /path/to/file) may encounter issues on Windows. Windows uses backslashes (\) as path separators and drive letters (e.g., C:\) to specify disk drives.
3. Line Endings: Windows and Linux use different line ending characters in text files. Windows uses carriage return (CR) and line feed (LF) characters (\r\n), while Linux uses only the LF character (\n). Bash scripts written on Linux may have issues with line endings when run on Windows, and vice versa. This can be resolved by ensuring consistent line endings or using tools to convert line endings.
4. Environment Variables: Environment variables may have different names or behaviors on Windows compared to Linux. Bash scripts that rely heavily on environment variables may need to be updated to work correctly on Windows.
To overcome these limitations, it’s recommended to write platform-agnostic bash scripts whenever possible. This involves using portable commands, avoiding platform-specific tools, and considering the differences between Windows and Linux environments.
Now that you have a better understanding of running bash scripts on Windows, let’s explore some resources for learning bash scripting.
Additional Resources
– Writing a Basic Bash Script
– Common Commands in Bash Scripting
– Difference Between Bash Scripting and Shell Scripting