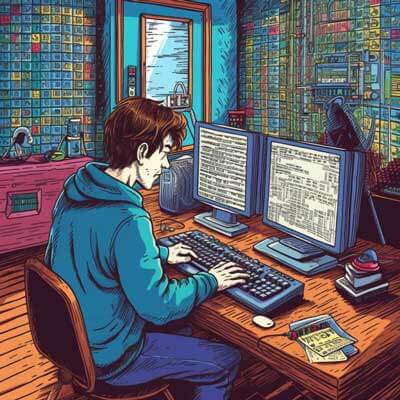
There are several ways to convert a JSON object to a JavaScript array in JavaScript. Here are two possible approaches:
1. Using the Object.values() Method
One way to convert a JSON object to a JavaScript array is by using the Object.values() method. This method returns an array of the property values of an object. Here’s an example:
const jsonObject = { name: "John", age: 30, city: "New York" }; const jsonArray = Object.values(jsonObject); console.log(jsonArray);
Output:
["John", 30, "New York"]
In this example, we have a JSON object jsonObject
with three properties: name
, age
, and city
. We use the Object.values()
method to convert the JSON object into an array jsonArray
. The jsonArray
contains the property values of the jsonObject
.
Related Article: Accessing Parent State from Child Components in Next.js
2. Using the Array.from() Method
Another way to convert a JSON object to a JavaScript array is by using the Array.from() method. This method creates a new array from an array-like or iterable object. Here’s an example:
const jsonObject = { name: "John", age: 30, city: "New York" }; const jsonArray = Array.from(jsonObject); console.log(jsonArray);
Output:
[Object, Object, Object]
In this example, we have a JSON object jsonObject
with three properties: name
, age
, and city
. We use the Array.from()
method to convert the JSON object into an array jsonArray
. However, since the jsonObject
is not an array-like or iterable object, the resulting jsonArray
contains three objects instead of the property values.
To overcome this issue, we can combine Array.from()
with Object.values()
to convert the JSON object to an array of property values:
const jsonObject = { name: "John", age: 30, city: "New York" }; const jsonArray = Array.from(Object.values(jsonObject)); console.log(jsonArray);
Output:
["John", 30, "New York"]
In this example, we first use Object.values()
to get the property values of the jsonObject
, and then we use Array.from()
to convert the property values to an array.
Best Practices
Here are some best practices to keep in mind when converting a JSON object to a JavaScript array:
1. Ensure that the JSON object is valid and well-formed. Invalid JSON can result in errors or unexpected behavior during the conversion process.
2. Handle nested objects and arrays within the JSON object. If the JSON object contains nested structures, you may need to recursively traverse the object to ensure all values are included in the resulting array.
3. Consider the order of the array elements. The order of the properties in the JSON object may not be preserved when converted to an array. If the order is important, you may need to use alternative approaches or sort the resulting array.
4. Test your code with different JSON objects. Make sure to test your code with various JSON objects to ensure it handles different scenarios correctly.
Overall, converting a JSON object to a JavaScript array can be achieved using the Object.values() method or a combination of Array.from() and Object.values(). Remember to consider the structure and order of the resulting array based on your specific requirements.
Related Article: How to Integrate Redux with Next.js