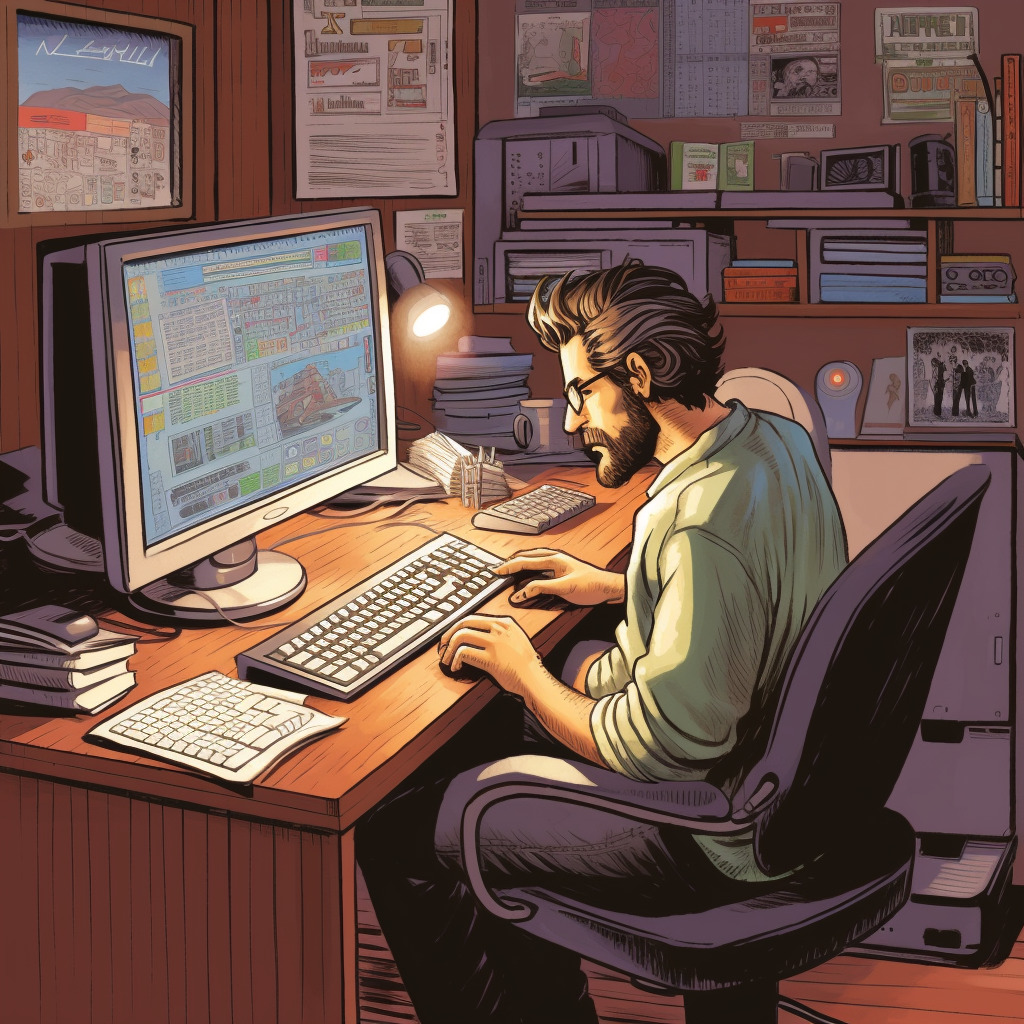
- The Fundamentals of Git
- Initializing a Git Repository
- Adding Files to the Repository
- Committing Changes
- Viewing the Commit History
- Creating Branches
- Merging Branches
- Remote Repositories
- Cloning a Repository
- Setting Up Git on Your Local Machine
- Installing Git
- Configuring Git
- Checking Git Version
- Initializing a Git Repository
- Cloning a Git Repository
- Creating and Cloning Repositories
- Creating a Repository
- Cloning a Repository
- Working with Remote Repositories
- Initializing an Existing Repository
- Understanding Branches and Merging
- Creating and Switching Branches
- Merging Branches
- Resolving Merge Conflicts
- Deleting Branches
- Branching Strategies
- Mainline Branching
- Feature Branching
- Gitflow Workflow
- Collaborating with Remote Repositories
- Cloning a Repository
- Fetching and Pulling Changes
- Pushing Changes
- Resolving Conflicts in Git
- Managing Large Repositories
- 1. Cloning Partial History
- 2. Sparse Checkout
- 3. Shallow Clone Specific Branch
- 4. Git Reflog
- 5. Git LFS (Large File Storage)
- 6. Git Garbage Collection
- Using Git for Deployment
- 1. Git Hooks
- 2. Git Submodules
- 3. Git Worktrees
- 4. Git Deployment Tools
- Git Hooks: Automating Workflow
- Understanding Git Hooks
- Types of Git Hooks
- Creating a Git Hook
- Using Git Hooks in a Team
- Git Alias
- Creating a Git Alias
- Customizing Aliases
- Using Shell Commands in Aliases
- Listing and Editing Aliases
- Git Reset: Undoing Changes
- Git Reflog
- Recovering Lost Commits with Git Reflog
- Undoing Actions with Git Reflog
- Git Stash: Temporarily Saving Changes
- Git Rebase
- Common Use Cases for Git Rebase
- Performing a Git Rebase
- Advanced Git Rebase Techniques
- Git Submodules
- Adding a Submodule
- Updating Submodules
- Removing Submodules
- Working with Submodules
- Git Bisect: Finding the Cause of Issues
- Git Worktree
- Creating a New Worktree
- Working with Multiple Worktrees
- Listing Worktrees
- Removing Worktrees
- The .gitignore File
- Pattern Matching
- Ignoring Files Already Tracked by Git
- Ignoring Files Globally
- Git Cherry-pick
- Conflict Resolution
- Cherry-picking a Range of Commits
- Cherry-picking from a Different Branch
- Git Amend: Modifying the Last Commit
- Git Blame
- Viewing Changes in Detail
- Ignoring Whitespace Changes
- Limiting the Output
- Git Revert
- Using Git Revert
- Reverting Multiple Commits
- Reverting Merge Commits
- Git LFS
- Installing Git LFS
- Configuring Git LFS
- Tracking Large Files
- Committing and Pushing Large Files
- Cloning a Repository with Git LFS
- Working with Git LFS
- Git Secrets: Preventing Sensitive Information
- 1. Gitignore
- 2. Remove Sensitive Data from Git History
- 3. Git Secrets
- 4. Encryption and Secrets Management
- 5. Continuous Integration and Code Analysis
- Git Bisect: Debugging with Binary Search
- What is Git Bisect?
- How to Use Git Bisect
- Practical Example
- Subtree
- Adding a Subtree
- Updating a Subtree
- Pushing Changes to a Subtree
- Splitting a Subtree
- Removing a Subtree
- Git Archive: Exporting Repository Snapshots
- Git Clean: Removing Untracked Files
- Git Rerere: Resolving Repeated Conflicts
- Forking Workflow
- Feature Branch Workflow
- Git Hooks for CI/CD
- Integrating Git with CI/CD Tools
The Fundamentals of Git
Git is a distributed version control system that allows multiple people to collaborate on a project. Whether you are a beginner or an experienced developer, understanding the fundamentals of Git is crucial for effectively using this powerful tool. This chapter will cover the basic concepts and commands that every Git user should know.
Related Article: 6 Essential software testing tools to add to your arsenal (2023 updated)
Initializing a Git Repository
To start using Git, you first need to initialize a Git repository in your project directory. Open your terminal and navigate to the desired directory. Then, run the following command:
git init
This command creates a new Git repository in your current directory. Git will create a hidden .git
directory that stores all the necessary files and metadata.
Adding Files to the Repository
Once you have initialized a Git repository, you can start tracking files. To add a file to the repository, use the git add
command followed by the file name. For example:
git add file.txt
This command stages the file.txt
for the next commit. Staging is the process of preparing files to be committed to the repository.
Committing Changes
After staging the changes, you need to commit them to the repository. A commit is a snapshot of your project at a specific point in time. To commit the changes, use the git commit
command with a descriptive message:
git commit -m "Add file.txt"
The -m
flag allows you to provide a commit message directly in the command. It is recommended to write meaningful and concise commit messages that describe the changes made.
Related Article: The very best software testing tools
Viewing the Commit History
Git maintains a complete history of all commits made in the repository. You can view the commit history using the git log
command:
git log
This command displays a list of commits in reverse chronological order. Each commit is identified by a unique hash, and it includes details such as the author, date, and commit message.
Creating Branches
Branching is a fundamental concept in Git that allows you to work on different features or versions of a project simultaneously. To create a new branch, use the git branch
command followed by the branch name:
git branch feature-branch
This command creates a new branch named feature-branch
based on the current commit. You can then switch to the new branch using the git checkout
command:
git checkout feature-branch
Now you can make changes and commit them to the feature-branch
without affecting the main branch.
Merging Branches
Once you have finished working on a feature branch, you can merge it back into the main branch. Switch to the main branch using the git checkout
command:
git checkout main
Then, use the git merge
command to merge the feature branch into the main branch:
git merge feature-branch
Git will automatically merge the changes from the feature branch into the main branch, combining the commit history of both branches.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Remote Repositories
Git allows you to collaborate with others by using remote repositories. To add a remote repository, use the git remote add
command:
git remote add origin https://github.com/username/repo.git
This command adds a remote named origin
with the URL of your remote repository. You can then push your local commits to the remote repository using the git push
command:
git push origin main
This command pushes the local main
branch to the origin
remote repository.
Cloning a Repository
To work on an existing Git repository, you can clone it to your local machine. Use the git clone
command followed by the repository URL:
git clone https://github.com/username/repo.git
This command creates a local copy of the remote repository on your machine.
Setting Up Git on Your Local Machine
Git is a widely used version control system that allows developers to track changes in their codebase efficiently. Whether you are starting a new project or joining an existing one, setting up Git on your local machine is the first step towards utilizing its full potential. In this chapter, we will guide you through the process of installing and configuring Git on different operating systems.
Related Article: Installing Docker on Ubuntu in No Time: a Step-by-Step Guide
Installing Git
Before you can start using Git, you need to install it on your local machine. Git is available for Windows, macOS, and Linux, and can be downloaded from the official Git website at https://git-scm.com/downloads.
Configuring Git
After successfully installing Git, the next step is to configure it with your personal information. This is important as Git uses this information to identify the author of each commit. You can configure Git using the following commands in your terminal:
$ git config --global user.name "Your Name" $ git config --global user.email "youremail@example.com"
Replace “Your Name” with your actual name and “youremail@example.com” with your email address. The --global
flag ensures that these configurations are applied globally to all your Git repositories.
Checking Git Version
To verify that Git has been successfully installed and configured, you can check its version using the following command:
$ git --version
This will display the installed Git version on your machine. It is always a good practice to keep Git updated to the latest version to benefit from the latest features and bug fixes.
Related Article: Docker How-To: Workdir, Run Command, Env Variables
Initializing a Git Repository
Once Git is set up, you can start using it by initializing a Git repository in your project directory. Navigate to your project folder in the terminal and run the following command:
$ git init
This will create a new, empty Git repository in your project directory. You can now start tracking changes in your codebase and making commits.
Cloning a Git Repository
If you are joining an existing project, you can clone the Git repository to your local machine using the git clone
command. This will create a copy of the repository on your machine. In your terminal, navigate to the directory where you want to clone the repository and run the following command:
$ git clone https://github.com/username/repository.git
Replace https://github.com/username/repository.git
with the URL of the repository you want to clone.
Congratulations! You have successfully set up Git on your local machine.
Creating and Cloning Repositories
Git is a powerful version control system that allows developers to track changes in their codebase effectively. In this chapter, we will explore the process of creating and cloning repositories, which is the first step in leveraging the full potential of Git.
Related Article: How to Push Changes to a Remote Repository with Git Push
Creating a Repository
To create a new Git repository, navigate to the desired directory in your terminal and execute the following command:
$ git init
This command initializes an empty Git repository in the current directory. Git will create a hidden directory named .git
, which contains all the necessary files to manage the repository.
Cloning a Repository
Cloning a repository allows you to create a local copy of an existing repository. To clone a repository, use the following command:
$ git clone <repository_url>
Replace <repository_url>
with the URL of the repository you want to clone. For example, to clone a repository hosted on GitHub:
$ git clone https://github.com/user/repo.git
Git will create a new directory with the same name as the repository and download all the repository’s files and history into it.
Working with Remote Repositories
Once you have cloned a repository, you can interact with the remote repository using various Git commands. The remote repository is typically where the main development of a project occurs.
To view the existing remote repositories associated with your local repository, use the following command:
$ git remote -v
This command will display a list of remote repositories and their corresponding URLs.
To add a new remote repository, use the following command:
$ git remote add <name> <repository_url>
Replace <name>
with a convenient name for the remote repository, and <repository_url>
with the URL of the repository. For example:
$ git remote add origin https://github.com/user/repo.git
The name origin
is commonly used to refer to the main remote repository.
Related Article: Using Stored Procedures in MySQL
Initializing an Existing Repository
If you have an existing project that is not yet under version control, you can initialize a new Git repository to start tracking changes. Navigate to the project’s directory and execute the following command:
$ git init
Git will initialize the repository and start tracking changes from that point forward. You can then use Git commands to manage the project’s versions and collaborate with others.
Understanding Branches and Merging
Git’s branch and merge capabilities are powerful tools that allow developers to work on multiple versions of a project simultaneously and seamlessly integrate changes from different branches. Understanding how branches work and how to effectively merge them is essential for working with Git.
Creating and Switching Branches
In Git, a branch is a lightweight movable pointer to a commit. It allows you to isolate development work without affecting other branches. Creating a new branch is as simple as running the following command:
$ git branch <branch-name>
To switch to a different branch, you can use the checkout
command:
$ git checkout <branch-name>
Alternatively, you can combine the creation and switching of branches with the checkout
command by specifying the -b
flag:
$ git checkout -b <branch-name>
Related Article: How to Use Nested Queries in Databases
Merging Branches
Merging branches in Git combines changes from one branch into another. The most common merge is the fast-forward merge, which occurs when the branch being merged into has no new commits since the branch to be merged was created.
To perform a fast-forward merge, switch to the branch you want to merge into and run the merge command with the branch to be merged:
$ git checkout <branch-to-merge-into> $ git merge <branch-to-merge>
In some cases, Git cannot perform a fast-forward merge due to diverging commit histories. In such cases, a three-way merge is required. Git will automatically create a new commit that combines the changes from both branches.
Resolving Merge Conflicts
During a merge, conflicts can occur when Git cannot automatically determine how to combine the changes from different branches. Git will mark the conflicting sections in the affected files, allowing you to manually resolve the conflicts.
To resolve a merge conflict, open the conflicted file(s) in a text editor and look for the conflict markers. Edit the file to keep the desired changes and remove the markers. After resolving the conflicts, stage the changes and commit:
$ git add <file1> <file2> ... $ git commit -m "Resolved merge conflicts"
Deleting Branches
Once you have merged a branch into another, you might want to delete the merged branch to keep your repository clean. To delete a branch, use the branch
command with the -d
flag:
$ git branch -d <branch-name>
If the branch has not been fully merged and you want to delete it anyway, use the -D
flag instead:
$ git branch -D <branch-name>
Related Article: Tutorial on Database Sharding in MySQL
Branching Strategies
Branching is a core feature of Git that enables parallel development and collaboration. There are various branching strategies you can adopt based on the needs of your team and project. Let’s explore a few commonly used strategies:
Mainline Branching
In this strategy, the main branch serves as the integration point for all features and bug fixes. Developers create feature branches off the main branch, work on their changes, and merge them back into the main branch once they are ready. This approach ensures a stable mainline and facilitates continuous integration.
Feature Branching
With this strategy, each new feature or task is developed in a dedicated branch. Developers can work independently on their respective features without interfering with each other. Once a feature is complete, it can be merged back into the main branch. Feature branching enhances collaboration and allows for easy feature isolation and testing.
Related Article: How to Implement Database Sharding in PostgreSQL
Gitflow Workflow
Gitflow is a popular branching model that provides a structured approach to collaboration. It defines two main branches: master
and develop
. The master
branch represents the production-ready code, while the develop
branch serves as the integration branch for ongoing development. Feature branches are created off the develop
branch, and once completed, they are merged back into develop
. Release branches and hotfix branches are also used to manage releases and urgent bug fixes.
Collaborating with Remote Repositories
Git allows you to collaborate with team members by leveraging remote repositories. Here are some commands and workflows to facilitate collaboration:
Cloning a Repository
To start collaborating with an existing Git repository, you first need to clone it to your local machine using the git clone
command. This creates a complete copy of the repository, including all branches and commit history.
git clone https://github.com/example/repository.git
Related Article: How to Implement Database Sharding in MongoDB
Fetching and Pulling Changes
To keep your local copy up to date with the remote repository, you can use the git fetch
and git pull
commands. git fetch
retrieves the latest changes from the remote repository, while git pull
fetches and merges the changes into your local branch.
git fetch git pull origin main
Pushing Changes
When you have made changes to your local branch and want to share them with others, you can use the git push
command to push your commits to the remote repository.
git push origin my-feature-branch
Resolving Conflicts in Git
Git is a powerful version control system that allows multiple developers to work on the same project simultaneously. However, this can sometimes lead to conflicts when two or more developers make changes to the same file. Resolving these conflicts is an essential skill for any Git user. In this chapter, we will explore various techniques to resolve conflicts in Git.
When conflicts occur, Git provides a set of tools to help you identify and resolve them. The most common scenario is when two branches have diverged and changes have been made to the same lines of code. Git uses markers to highlight the conflicting sections within the file.
To resolve conflicts, follow these steps:
1. Identify the conflicting files: Git will inform you about the files that have conflicts. You can use the command git status
to see the list of files with conflicts.
2. Open the conflicting file(s) in your text editor: Use your preferred text editor to open the files with conflicts. Look for the markers added by Git to identify the conflicting sections.
3. Resolve the conflicts: Review the conflicting sections and make the necessary changes to resolve the conflicts. Remove the Git markers once the conflicts are resolved.
4. Save the changes: Save the file(s) after resolving the conflicts.
5. Add the changes: Use the command git add <file>
to stage the resolved file(s) for the next commit. Alternatively, you can use git add .
to stage all changes.
6. Commit the changes: Use the command git commit -m "Resolved conflicts"
to commit the changes with a descriptive message.
Git also provides advanced tools to help automate conflict resolution. The git mergetool
command launches a visual merge tool, such as KDiff3 or Beyond Compare, to resolve conflicts. This tool provides a side-by-side comparison of the conflicting sections and allows you to choose which changes to keep.
Here’s an example of using git mergetool
:
$ git mergetool
This command will launch the configured merge tool and guide you through the conflict resolution process.
In addition to resolving conflicts locally, Git also allows you to fetch changes from a remote repository and merge them with your local branch. The git pull
command is used to fetch and merge changes from a remote branch.
$ git pull origin <branch>
By default, Git will attempt to automatically merge the changes. However, if conflicts occur, you will need to resolve them manually using the steps mentioned earlier.
Resolving conflicts in Git can be challenging, but with practice and the right tools, it becomes easier. Understanding the conflict resolution process and using Git’s built-in tools will help you effectively collaborate with other developers and maintain a clean and functional codebase.
Related Article: Tutorial on Installing and Using redis-cli with Redis
Managing Large Repositories
Managing large repositories can be a challenge, especially when it comes to performance and storage. In this chapter, we will explore some advanced hacks and commands to help you effectively manage large repositories in Git.
1. Cloning Partial History
When cloning a large repository, you may not always need the entire history. Git allows you to clone only a specific branch or a limited number of commits using the --depth
option. For example, to clone only the last 10 commits of a branch:
git clone --depth 10 <repository>
This can significantly reduce the clone time and disk space usage, especially for repositories with a long history.
2. Sparse Checkout
If you only need a subset of files from a large repository, you can use the sparse checkout feature. Sparse checkout allows you to specify the files or directories you want to include in your working directory, excluding the rest.
To enable sparse checkout, first, initialize it:
git init
Then, enable the sparse checkout configuration:
git config core.sparseCheckout true
Next, specify the files or directories you want to include by adding their paths to the .git/info/sparse-checkout
file:
echo "path/to/file" >> .git/info/sparse-checkout
Finally, update the working directory to reflect the changes:
git read-tree -mu HEAD
Now, your working directory will only contain the files or directories you specified.
3. Shallow Clone Specific Branch
In some cases, you may only want to clone a specific branch from a large repository. Git provides the --single-branch
option to perform a shallow clone of only that branch:
git clone --depth 1 --single-branch --branch <branch> <repository>
This can be useful when you are only interested in a specific branch’s history and want to save disk space and cloning time.
4. Git Reflog
The reflog is a powerful tool that records all the changes to Git references in a repository, including commits, branches, and tags. It can be particularly useful for managing large repositories.
To view the reflog, use the following command:
git reflog
The reflog displays a chronological list of all the reference updates, along with their corresponding commit messages and hashes. You can use this information to recover or undo changes that may have been lost or overwritten.
5. Git LFS (Large File Storage)
Git LFS is an extension that allows you to handle large files more efficiently in Git repositories. It replaces large files with text pointers, storing the actual file content on a remote server. This reduces the repository size and improves performance.
To start using Git LFS, you need to install it and initialize it in your repository:
git lfs install git lfs track "*.bin"
The git lfs track
command specifies the file patterns to track with Git LFS. In this example, we are tracking all files with a .bin
extension.
After committing and pushing the changes, Git LFS will take care of uploading and downloading the large files, while Git handles the rest of the repository.
6. Git Garbage Collection
Over time, Git repositories may accumulate unnecessary objects, which can impact performance and disk space. Git’s garbage collection helps optimize and clean up the repository by removing these unnecessary objects.
To perform garbage collection, use the following command:
git gc
By default, Git automatically runs garbage collection when necessary, but you can manually trigger it when needed.
It’s worth noting that running garbage collection can take some time, especially for large repositories, so it’s recommended to run it during off-peak usage periods.
Using Git for Deployment
Git, being a powerful version control system, can also be used for deploying your applications or websites. By utilizing Git for deployment, you can easily automate the process, track changes, and roll back if necessary. In this chapter, we will explore different techniques and commands to effectively use Git for deployment.
1. Git Hooks
Git hooks are scripts that Git executes before or after certain events occur, such as committing or pushing code. By utilizing Git hooks, you can trigger custom actions during the deployment process. For example, you can automatically update your production server whenever a new commit is pushed to the master branch.
To create a Git hook, navigate to your Git repository’s .git/hooks
directory. Here, you will find various sample hook scripts that can be renamed and customized to suit your needs. Hooks are written in any scripting language, such as Bash or Python.
Let’s say you want to automatically build your project whenever code is pushed. You can create a post-receive
hook with the following content (Bash script):
#!/bin/bash while read oldrev newrev refname; do if [[ $refname == "refs/heads/master" ]]; then # Build your project npm run build fi done
2. Git Submodules
Git submodules allow you to include another Git repository as a subdirectory within your own repository. This is useful when you want to include a dependency or library that is separately maintained. By utilizing submodules, you can easily manage and update the included repository.
To add a submodule to your repository, use the git submodule add
command followed by the URL of the repository:
git submodule add https://github.com/example/repo.git
This command will clone the repository and add it as a submodule in your project. The submodule will be added as a reference, and its contents will be stored in a separate .gitmodules
file.
When deploying your project, make sure to initialize and update the submodules using the following commands:
git submodule init git submodule update
These commands will ensure that the submodules are properly set up and updated in your deployment environment.
3. Git Worktrees
Git worktrees allow you to have multiple working trees linked to a single repository. This is useful when you need to deploy your project to multiple servers or environments.
To create a new worktree, use the git worktree add
command followed by the path where you want to create the worktree:
git worktree add /path/to/deployment/folder
This command will create a new working tree in the specified folder, allowing you to deploy your project separately. Each worktree has its own separate HEAD, index, and working directory.
When deploying to a worktree, you can use standard Git commands such as git pull
or git checkout
to update the code. This allows you to easily manage and deploy your project to multiple environments using a single repository.
4. Git Deployment Tools
In addition to the built-in features provided by Git, several third-party deployment tools can further simplify the deployment process. These tools often provide additional features such as continuous integration, automated testing, and rollback capabilities.
Some popular Git deployment tools include:
– Capistrano: A Ruby-based deployment script that automates the deployment process for web applications.
– Buddy: A CI/CD platform that integrates with Git to automate the deployment workflow.
– DeployHQ: A hosted deployment service that allows you to easily deploy your Git repositories.
These tools provide a higher level of automation and customization for your deployment process, making it easier to manage and deploy your projects efficiently.
Using Git for deployment not only streamlines the process but also allows you to take advantage of Git’s powerful version control capabilities. By incorporating Git hooks, submodules, worktrees, and deployment tools, you can ensure a smooth and efficient deployment workflow.
Git Hooks: Automating Workflow
Git hooks are scripts that Git executes before or after certain events occur, such as committing changes, pushing to a remote repository, or merging branches. They allow you to automate and customize your Git workflow, ensuring consistency and enforcing best practices within your team. In this chapter, we will explore the power of Git hooks and how they can be used to enhance your development process.
Understanding Git Hooks
Git hooks are stored in the .git/hooks
directory of your Git repository. Each hook is simply an executable script, written in any scripting language you prefer, such as shell, Python, or Ruby. Git provides a set of predefined hooks that you can use, and you can also create custom hooks to suit your specific needs.
When a hook is triggered by an event, Git passes certain information as command-line arguments to the hook script. For example, when the pre-commit hook is executed, Git passes the commit message file path as an argument. This allows you to access and manipulate the relevant data within your hook script.
Types of Git Hooks
Git provides several types of hooks that can be used to automate different stages of your workflow. Some commonly used hooks include:
– pre-commit
: Triggered before a commit is created. You can use this hook to enforce code formatting rules, run static code analysis, or perform any other checks before allowing a commit to proceed.
– pre-push
: Triggered before a push operation is performed. You can use this hook to run tests, validate code, or perform other checks to ensure the quality of the pushed changes.
– post-merge
: Triggered after a successful merge operation. You can use this hook to update dependencies, rebuild your project, or perform any other necessary tasks after merging branches.
– post-checkout
: Triggered after a checkout operation is performed. You can use this hook to set up the development environment, install dependencies, or perform any other actions required when switching branches.
Creating a Git Hook
To create a Git hook, you simply need to add an executable script with the appropriate name to the .git/hooks
directory of your repository. For example, to create a pre-commit hook, you would add a script named pre-commit
to the .git/hooks
directory.
Here’s an example of a pre-commit hook script written in a shell script:
#!/bin/sh # Check for trailing whitespace if git diff --check --cached | grep -q "^+.*[[:blank:]]$" ; then echo "Error: Trailing whitespace found, please remove before committing." exit 1 fi # Run code formatting make format
This hook checks for trailing whitespace in the changes being committed and displays an error message if any is found. It then runs a code formatting command using make
.
Using Git Hooks in a Team
Git hooks can be shared among team members by including them in the repository and ensuring they are executable. However, it’s important to note that hooks are not version-controlled, so each team member needs to manually set up the hooks in their local repositories.
To simplify hook setup, you can use tools like Husky or Git templates to automatically install hooks for new team members or when cloning the repository.
Git Alias
Git aliases are a powerful feature that allows you to create shortcuts for your most frequently used Git commands. With aliases, you can save time and effort by avoiding lengthy and repetitive commands. In this chapter, we will explore how to customize your Git commands using aliases.
Creating a Git Alias
To create a Git alias, you can use the git config
command with the --global
flag to set the alias in your global Git configuration file. Here’s an example of creating an alias for the git status
command:
git config --global alias.st status
In this example, we set the alias st
for status
. Now, instead of typing git status
, you can simply run git st
to get the same result.
Customizing Aliases
You can customize your aliases to suit your preferences and workflow. Aliases can be as simple as mapping a command to a shorter name, or they can include additional options and parameters.
Let’s say you frequently use the git log
command with specific options. You can create an alias that includes these options by running the following command:
git config --global alias.lg "log --pretty=format:'%h - %an, %ar : %s'"
Now, running git lg
will display the commit log with the specified format.
Using Shell Commands in Aliases
Git aliases are not limited to Git commands only; you can also include shell commands in your aliases. This allows you to perform more complex operations or combine multiple commands into a single alias.
For example, let’s say you want to create an alias that shows the number of commits you have made in a repository. You can achieve this by including a shell command in your alias:
git config --global alias.num-commits "rev-list --count HEAD"
Now, running git num-commits
will display the total number of commits in your repository.
Listing and Editing Aliases
To list all your existing aliases, you can run the following command:
git config --global --get-regexp "^alias\."
This will display a list of all your aliases and their corresponding commands.
To edit an existing alias, you can use the git config
command with the --global
flag and provide the alias name and its new command. For example, to change the command associated with the lg
alias, you can run:
git config --global alias.lg "log --pretty=format:'%h - %an, %ar : %s' --graph"
Now, running git lg
will display the commit log with the updated format and graph.
Git Reset: Undoing Changes
One of the most useful features of Git is the ability to undo changes that have been made. Git provides several commands to help us revert back to a previous state, and one of the most commonly used commands for this purpose is git reset
.
When we make changes to our codebase, it is not uncommon to realize later that we need to undo some of those changes. This could be due to a mistake or an experimental change that did not work as expected. Git provides the git reset
command to help us undo these changes and revert back to a previous commit.
There are three main options when using git reset
: --soft
, --mixed
, and --hard
. Each option has a different level of undoing changes.
– git reset --soft
: This option only resets the commit history, leaving the changes in the working directory and the staging area untouched. This is useful when we want to undo the last commit but keep the changes for further modification or commit.
Here is an example of how to use git reset --soft
:
$ git reset --soft HEAD~1
– git reset --mixed
: This option resets the commit history and the staging area, but keeps the changes in the working directory. It unstages the changes, allowing us to modify them before committing again.
Here is an example of how to use git reset --mixed
:
$ git reset --mixed HEAD~1
– git reset --hard
: This option resets everything, including the commit history, the staging area, and the working directory. It discards all the changes and reverts back to the state of the specified commit.
Here is an example of how to use git reset --hard
:
$ git reset --hard HEAD~1
It is important to note that git reset
rewrites the commit history. If the changes we want to undo have already been pushed to a remote repository, extreme caution should be exercised. Rewriting commit history can cause conflicts and disrupt the work of other team members. In such cases, it is recommended to use git revert
instead, which creates a new commit that undoes the changes made in a previous commit.
The git reset
command is a handy tool for undoing changes in Git. It provides different options to reset the commit history, staging area, and working directory, allowing developers to selectively undo changes based on their needs. However, it is important to use this command with caution, especially when working with a shared remote repository.
Git Reflog
In the previous chapters, we have explored various Git commands and techniques to manage our repositories effectively. However, there may be instances where we accidentally delete or lose commits, leading to frustration and potential loss of work. In this chapter, we will learn about Git’s reflog feature, which allows us to recover lost commits and undo certain actions.
Git reflog, short for “reference logs,” is a powerful tool that records the movement of Git references, such as branches and tags, in your repository. It keeps a log of all the actions performed on your local repository, including commits, branch creations and deletions, merges, rebases, and more.
The reflog is stored locally and is not shared when you push or pull changes from a remote repository. Its purpose is to help you recover lost commits or undo certain actions, providing a safety net for your work.
Recovering Lost Commits with Git Reflog
When we accidentally delete or lose commits, Git reflog comes to the rescue. Let’s walk through the process of recovering lost commits using Git reflog.
First, let’s display the reflog for your current branch by running the following command in your Git repository:
git reflog
This command will display a list of all the actions that have been performed on your repository, along with the corresponding commit hashes and messages. Each entry in the reflog has a unique index number, which we will use to restore lost commits.
To recover a lost commit, identify the corresponding entry in the reflog and note its index number. Then, use the following command:
git cherry-pick <commit-hash>
Replace <commit-hash>
with the commit hash of the lost commit you want to recover. Git will apply the changes from that commit to your current branch, effectively restoring the lost commit.
Undoing Actions with Git Reflog
Apart from recovering lost commits, Git reflog can also be used to undo actions such as branch deletions, merges, and rebases. To undo an action, follow these steps:
1. Display the reflog using the command git reflog
.
2. Identify the entry in the reflog corresponding to the action you want to undo.
3. Note the index number of the entry.
4. Use the following command to revert the action:
git reset --hard HEAD@{<index>}
Replace <index>
with the index number of the reflog entry you want to revert. This command will reset your repository’s state to the state it was in at that particular reflog entry.
Git Stash: Temporarily Saving Changes
When working on a project with Git, there may be times when you need to switch branches or work on a different task while leaving your current changes behind. Git stash is a powerful command that allows you to save your modifications temporarily without committing them.
Git stash is particularly useful when you’re in the middle of working on a feature or fixing a bug, and you need to switch to another branch or address an urgent issue. Instead of committing your changes, which could affect the stability of the project in progress, you can stash your changes and return to them later.
To stash your changes, you can use the following command:
git stash
This command will save all your modifications, including untracked files, into a new stash. Git will revert your working directory to the last commit, allowing you to switch branches or perform other tasks.
Once you’re ready to come back to your stashed changes, you can apply the stash using the following command:
git stash apply
By default, git stash apply
will apply the latest stash. However, if you have multiple stashes, you can specify which stash to apply by providing its reference. For example:
git stash apply stash@{2}
In addition to applying the stash, it is retained in the stash list. If you want to remove the stash after applying it, you can use the git stash drop
command:
git stash drop
You can also create a new branch from a stash and switch to it directly using the git stash branch
command. This command applies the latest stash and creates a new branch with the specified name:
git stash branch <branch-name>
Git stash also allows you to inspect the contents of a stash without applying it. You can use the git stash show
command to see the changes made in a specific stash:
git stash show stash@{1}
If you want to see the full diff of the stash, including all file changes, you can use the -p
flag:
git stash show -p stash@{3}
In some cases, you may need to stash only a specific set of changes instead of all modifications. Git provides the git stash save
command, which allows you to give a descriptive message and selectively stash changes. For example:
git stash save "WIP: Refactoring the authentication module"
With the git stash save
command, you can include only the relevant changes in your stash, making it easier to manage and apply later.
Remember that stashing is not a substitute for committing your changes. Stashes are temporary and should not be relied upon for long-term storage. Always commit your changes when they are ready and stable.
With the ability to temporarily save your changes using Git stash, you can confidently switch between branches or address urgent tasks without the fear of losing your progress.
Git Rebase
Git rebase is a command that enables you to combine, edit, or remove commits from a branch’s history. Unlike Git merge, which creates a new commit to merge changes from one branch into another, rebase rewrites the commit history of a branch by moving, modifying, or discarding commits.
The basic syntax for performing a rebase is as follows:
git rebase <base_branch>
Here, <base_branch>
refers to the branch you want to rebase onto. By default, Git rebase will apply the commits from the current branch on top of the latest commit of the <base_branch>
.
Common Use Cases for Git Rebase
Git rebase can be useful in various scenarios, including:
1. Cleaning up your commit history: You can use rebase to squash multiple small commits into a single, more meaningful commit. This helps keep your commit history clean and concise.
2. Reordering commits: With rebase, you can rearrange commits in your branch’s history. This can be handy when you want to present your commits in a logical order or group related changes together.
3. Resolving conflicts: When you rebase your branch onto another branch, conflicts may arise if both branches have made changes to the same code. Git provides tools to help you resolve these conflicts during the rebase process.
Performing a Git Rebase
To perform a basic Git rebase, follow these steps:
1. Switch to the branch you want to rebase:
git checkout <branch_name>
2. Run the rebase command, specifying the base branch:
git rebase <base_branch>
3. Resolve any conflicts that may occur during the rebase process. Git will pause the rebase and prompt you to resolve conflicts manually.
4. After resolving conflicts, continue the rebase by running:
git rebase --continue
Advanced Git Rebase Techniques
Git rebase offers several advanced techniques to further enhance your commit history:
1. Interactive rebase: Git provides an interactive mode for rebase which allows you to cherry-pick, edit, squash, or drop individual commits. This is useful for fine-grained control over your commit history.
2. Rebasing on top of a specific commit: Instead of rebasing on top of a branch, you can rebase onto a specific commit hash. This allows you to incorporate changes from another commit without merging the entire branch.
3. Using Git rebase to split commits: Rebase can also be used to split a commit into multiple smaller commits. This can be helpful when you realize a commit contains unrelated changes or if you want to separate a large commit into smaller logical units.
Git Submodules
Git submodules are a way to include one Git repository as a subdirectory of another Git repository. They allow you to manage external dependencies by linking to a specific commit in another repository. This can be useful when you want to include a library or framework in your project without actually copying its code into your repository.
Adding a Submodule
To add a submodule, you can use the git submodule add
command followed by the URL of the repository you want to include and the path where you want it to be placed. For example, to add a submodule from the repository at https://github.com/example/repo.git
and place it in the vendor/repo
directory, you would run:
git submodule add https://github.com/example/repo.git vendor/repo
Git will clone the repository and add it as a submodule in your project. It will also create a .gitmodules
file that contains information about the submodule.
Updating Submodules
When you clone a repository with submodules, you need to initialize and update the submodules separately. To initialize and update all submodules in a repository, you can use the git submodule update --init --recursive
command. This will clone all the submodules and checkout the specific commit they are linked to.
If you have already cloned a repository with submodules, you can update them using the git submodule update
command with the --remote
flag. For example:
git submodule update --remote
This command will fetch the latest changes from the remote repository and update the submodules to the latest commit.
Removing Submodules
To remove a submodule from your project, you need to follow a few steps. First, you need to remove the submodule’s entry from the .gitmodules
file. Then, you need to remove the submodule’s reference from the Git index by running the following command:
git rm --cached path/to/submodule
Finally, you can remove the actual submodule files by running:
rm -rf path/to/submodule
Working with Submodules
When working with submodules, it’s important to remember that they are separate Git repositories. This means that each submodule has its own commit history and branch structure. To make changes to a submodule, you need to navigate to its directory and treat it as a standalone repository.
To update a submodule to a specific commit, you can navigate to the submodule directory and use the git checkout
command. For example:
cd path/to/submodule git checkout <commit>
You can then commit the updated submodule reference in the main repository to track the new commit of the submodule.
Git Bisect: Finding the Cause of Issues
Git is a powerful version control system that allows developers to track and manage changes to their codebase. However, when bugs or issues arise, it can sometimes be challenging to determine the exact commit that introduced the problem. This is where Git bisect comes in handy.
Git bisect is a command that helps you find the commit that introduced a bug by performing a binary search through your commit history. It automates the process of checking out different commits and allows you to narrow down the problematic commit quickly.
## How Git Bisect Works
The Git bisect command uses a binary search algorithm to pinpoint the commit that introduced the issue. It starts by marking a “good” commit and a “bad” commit. The “good” commit is a commit where the issue is not present, while the “bad” commit is a commit where the issue is present.
Git bisect then checks out the commit in the middle of the range defined by the “good” and “bad” commits. It prompts you to test whether the commit is “good” or “bad.” Based on your response, it selects the next commit to check out, effectively halving the range each time. This process continues until Git bisect identifies the problematic commit.
## Using Git Bisect
To use Git bisect, follow these steps:
1. Start the bisect process by running the following command:
git bisect start
2. Mark a “good” commit using its commit hash:
git bisect good <commit>
3. Mark a “bad” commit using its commit hash:
git bisect bad <commit>
4. Git bisect will automatically check out a commit in the middle of the range and prompt you to test it. Based on the result, run one of the following commands:
– If the commit is “good,” use:
git bisect good
– If the commit is “bad,” use:
git bisect bad
5. Repeat step 4 until Git bisect identifies the problematic commit. Once it does, it will display the commit hash and additional information.
6. To exit the bisect process, use:
git bisect reset
## Practical Example
Let’s assume you have a project with a bug that was introduced at some point in the commit history. You want to find the commit that caused the issue using Git bisect.
1. Start the bisect process:
git bisect start
2. Mark a “good” commit:
git bisect good v1.0
3. Mark a “bad” commit:
git bisect bad v1.2
4. Git bisect checks out a commit in the middle and prompts you to test it. After testing, you determine it is a “bad” commit. Run:
git bisect bad
5. Repeat step 4 multiple times until Git bisect identifies the problematic commit.
6. Once Git bisect identifies the problematic commit, it will display the commit hash and additional information.
7. Exit the bisect process:
git bisect reset
Git bisect is a valuable tool for finding the cause of issues in your codebase. It automates the process of narrowing down the problematic commit and saves you valuable time in debugging. By using Git bisect, you can quickly identify the exact commit that introduced a bug and take the necessary steps to fix it.
For more information on Git bisect, refer to the official Git documentation: https://git-scm.com/docs/git-bisect.
Git Worktree
As developers, we often find ourselves working on multiple features or bug fixes simultaneously. Switching between different branches can become cumbersome and time-consuming. Git Worktree is a powerful tool that allows us to manage multiple workspaces, enabling us to work on different branches simultaneously without the need to switch back and forth.
Git Worktree is a feature introduced in Git version 2.5. It allows us to have multiple working trees associated with a single repository. Each working tree has its own separate working directory, index, and HEAD, allowing us to work on different branches or commits simultaneously. This feature is particularly useful when we want to test different branches or work on multiple features in parallel.
Creating a New Worktree
Creating a new worktree is simple using the git worktree
command. To create a new worktree, we need to specify the destination directory and the branch or commit we want to work on. For example, to create a new worktree and switch to the feature/new-feature
branch, we can use the following command:
$ git worktree add ../new-worktree feature/new-feature
This command creates a new worktree in the ../new-worktree
directory and checks out the feature/new-feature
branch.
Working with Multiple Worktrees
Once we have multiple worktrees, we can easily switch between them using the git worktree
command. To switch to a specific worktree, we can use the git worktree checkout
command followed by the path to the worktree. For example:
$ git worktree checkout ../new-worktree
This command switches to the new-worktree
worktree.
Listing Worktrees
We can list all the available worktrees using the git worktree list
command. This command displays information about each worktree, including the associated branch or commit, and the path to the worktree. For example:
$ git worktree list
This command lists all the available worktrees.
Removing Worktrees
To remove a worktree, we can use the git worktree remove
command followed by the path to the worktree. For example:
$ git worktree remove ../new-worktree
This command removes the new-worktree
worktree.
The .gitignore File
In Git, the .gitignore
file plays a crucial role in managing which files and directories should be ignored by Git. By specifying patterns in this file, you can prevent certain files from being tracked or included in your Git repository. This chapter will explore the power of the .gitignore
file and how to make the most of it.
To start ignoring files and directories, you need to create a .gitignore
file in your repository’s root directory. This file should be committed to your repository to ensure that everyone working on the project follows the same ignore patterns.
Here’s a basic example of a .gitignore
file that ignores a few common files and directories:
# Ignore compiled binaries *.exe *.dll # Ignore logs *.log # Ignore temporary files *.tmp # Ignore a specific directory /docs/
With this .gitignore
file, any files with the extensions .exe
or .dll
will be ignored, as well as any files with the extension .log
or .tmp
. Additionally, the entire docs
directory will be ignored.
Pattern Matching
The patterns in a .gitignore
file use simple pattern matching rules to determine which files and directories should be ignored. Here are a few important rules to keep in mind:
– Use *
to match zero or more characters.
– Use ?
to match a single character.
– Use [abc]
to match any single character within the brackets.
– Use [^abc]
to match any single character not within the brackets.
– Use **/
to match directories recursively.
For example, the pattern *.txt
will match any file with the .txt
extension, while the pattern temp?
will match files like temp1
or temp2
. The pattern [abc].txt
will match files like a.txt
, b.txt
, or c.txt
, and the pattern [^abc].txt
will match any file with the .txt
extension that does not start with a
, b
, or c
.
Ignoring Files Already Tracked by Git
Sometimes you may want to ignore files that are already being tracked by Git. To do this, you need to untrack the file first and then add it to your .gitignore
file. Here’s an example of how to achieve this:
# Untrack the file $ git rm --cached path/to/file # Add the file to .gitignore $ echo "path/to/file" >> .gitignore
The git rm --cached
command removes the file from Git’s index without deleting it from the file system. The echo
command appends the file path to the .gitignore
file.
Ignoring Files Globally
If you have files or directories that should be ignored across all your Git repositories, you can set up a global .gitignore
file. To do this, open your terminal and run the following command:
$ git config --global core.excludesfile ~/.gitignore_global
This command tells Git to use the .gitignore_global
file located in your home directory as the global ignore file. You can edit this file with any text editor to add patterns that should be ignored globally.
The .gitignore
file is a powerful tool for managing which files and directories should be ignored by Git. By understanding pattern matching and utilizing this file effectively, you can keep your repositories clean and avoid including unnecessary or sensitive files. So next time you find yourself needing to ignore certain files or directories, remember the power of the .gitignore
file.
Git Cherry-pick
In Git, cherry-picking refers to the process of selecting specific commits from one branch and applying them to another. It allows you to take a commit from one branch and apply it to another branch, regardless of their relationship or chronological order. This can be extremely useful when you want to selectively apply changes without merging entire branches.
To cherry-pick a commit, you need to provide the commit hash or a branch name and a commit range. Here’s the basic syntax:
git cherry-pick <commit>
For example, to cherry-pick a commit with hash abc123
:
git cherry-pick abc123
If you want to cherry-pick a range of commits, you can specify the commit range using the double-dot notation:
git cherry-pick <start-commit>..<end-commit>
For example, to cherry-pick all the commits between abc123
and def456
:
git cherry-pick abc123..def456
Conflict Resolution
In some cases, cherry-picking may result in conflicts if the changes being cherry-picked conflict with the current state of the branch. Git will pause the cherry-pick process and indicate the conflicting files.
To resolve conflicts during cherry-picking, you can use the same conflict resolution techniques used during a regular merge. After resolving the conflicts, you can use the git cherry-pick --continue
command to continue the cherry-pick process.
If you decide to abort the cherry-pick operation due to conflicts or any other reason, you can use the git cherry-pick --abort
command to revert back to the original state of the branch.
Cherry-picking a Range of Commits
The git cherry-pick
command also allows you to cherry-pick a range of commits. This can be useful when you want to apply a series of related commits to another branch. To cherry-pick a range of commits, specify the commit range using the double-dot notation:
git cherry-pick <start-commit>..<end-commit>
For example, to cherry-pick commits abc123
, def456
, and ghi789
:
git cherry-pick abc123..ghi789
Cherry-picking from a Different Branch
By default, the git cherry-pick
command applies commits from the current branch. However, you can cherry-pick commits from a different branch by specifying the branch name along with the commit hash or commit range. Here’s the syntax:
git cherry-pick <branch-name> <commit>
For example, to cherry-pick a commit with hash abc123
from a branch named feature
:
git cherry-pick feature abc123
This allows you to cherry-pick changes from branches that are not currently checked out.
Git Amend: Modifying the Last Commit
When working with Git, it’s common to realize that the last commit you made needs some changes. Whether you forgot to include a file, made a typo in the commit message, or simply need to update some code, Git provides the amend
command to help you modify the last commit.
To amend the last commit, you can use the following command:
git commit --amend
Executing this command will open your default text editor, displaying the commit message of the last commit. You can make any necessary changes to the message and save the file. Git will then update the last commit with your modifications.
Additionally, if you want to add changes to the last commit, you can stage the modifications using the git add
command before executing git commit --amend
. This allows you to include any files or changes you may have forgotten in the previous commit.
It’s worth mentioning that amending a commit creates a new commit object and replaces the previous one. Consequently, if you have already pushed the previous commit to a remote repository, amending it can cause issues for others who have based their work on the previous commit.
To push the amended commit to a remote repository, you’ll need to force push using the -f
or --force
flag:
git push -f origin branch-name
However, before force pushing, it’s important to communicate with your team and ensure that everyone is aware of the changes you made. Force pushing can overwrite other developers’ work if they have based their work on the old commit.
In addition to amending the commit message and adding changes, you can also use the amend
command to split a commit into multiple commits. This can be useful when you realize that a single commit contains changes that should have been separate.
To split a commit, you can use the interactive rebase feature of Git. First, find the commit hash of the commit you want to split using the git log
command. Then, execute the following command:
git rebase -i commit-hash^
This command will open an interactive rebase window where you can choose to edit, squash, or split commits. To split a commit, replace the pick
keyword with edit
in the rebase window for the commit you want to split. Save the file and exit the editor.
Git will then stop at the commit you want to split. You can use the git reset
command to unstage the changes from the commit, make the necessary changes, and create new commits as desired. Once you have finished splitting the commit, you can continue the rebase process by executing:
git rebase --continue
This will apply the remaining commits and complete the rebase operation.
In this chapter, we explored the git amend
command, which allows you to modify the last commit. We learned how to amend the commit message, add changes to the last commit, and even split a commit into multiple commits. However, it’s important to use caution when amending commits, as it can affect the work of other team members. Always communicate with your team before force pushing any amended commits to a remote repository.
Git Blame
In Git, the git blame
command is a powerful tool that allows you to investigate the changes made to a file. It provides valuable information about who made each change, when the change was made, and the commit that introduced the change. This chapter will explore how to use git blame
effectively to understand the history of a file.
To use the git blame
command, simply navigate to the repository’s root directory in your terminal and run the following command:
git blame <file>
Replace <file> with the path to the file you want to investigate. For example, to investigate the changes made to a file named app.js
, you would run:
git blame app.js
The output of the git blame
command will display information about each line in the file. Each line will be prefixed with the commit hash, the author’s name, the date of the change, and the line content. This information can help you understand who made each change and when it was made.
Viewing Changes in Detail
If you want to view the changes made in a specific commit, you can use the git show
command along with the commit hash. For example, to view the changes in the commit with the hash abc123
, you would run:
git show abc123
This will display detailed information about the commit, including the changes made to the file. It can be helpful to use git blame
in conjunction with git show
to investigate specific changes in more detail.
Ignoring Whitespace Changes
Sometimes, you may want to ignore whitespace changes when using git blame
. This can be useful if you’re only interested in substantive changes to the code. To ignore whitespace changes, you can use the -w
flag with the git blame
command. For example:
git blame -w app.js
This will show the blame information without considering whitespace changes, making it easier to focus on the relevant code changes.
Limiting the Output
If you want to limit the output of git blame
to a specific range of lines, you can use the -L
flag followed by the range. For example, to only show the blame information for lines 10 to 20 of a file, you would run:
git blame -L 10,20 app.js
This can be helpful when you’re only interested in a specific section of the file and want to narrow down the blame information.
Git Revert
Git revert is a command that allows you to undo changes made in a commit by creating a new commit that undoes those changes. Unlike other commands like git reset, which can alter the commit history, git revert maintains a clear and accurate history by creating new commits.
When you revert a commit, Git analyzes the changes made in that commit and creates a new commit that undoes those changes. This means that the original commit is still in the history, but its changes are effectively removed.
Using Git Revert
To revert a commit, you need to provide the commit hash or a reference to the commit you want to revert. The command syntax is as follows:
git revert <commit>
For example, to revert the last commit, you can use:
git revert HEAD
Or to revert a specific commit with the hash “abc123”, you can use:
git revert abc123
After running the command, Git will create a new commit that undoes the changes made in the specified commit.
Reverting Multiple Commits
In some cases, you may want to revert multiple commits at once. Git provides the ability to revert a range of commits using the following syntax:
git revert <start-commit>..<end-commit>
For example, to revert the last three commits, you can use:
git revert HEAD~2..HEAD
This command will create three new commits, each reverting one of the specified commits.
Reverting Merge Commits
Reverting merge commits can be a bit more complex because each merge commit has multiple parents. When you revert a merge commit, Git needs to know which parent’s changes to undo. By default, Git reverts the changes made in the merge commit itself, effectively undoing the merge.
If you want to revert the changes made in one of the merged branches, you can specify the parent commit using the -m
option followed by the parent number. For example, to revert the changes made in the branch merged with parent 2, you can use:
git revert -m 2 <merge-commit>
Git revert allows you to undo changes made in a commit while maintaining a clear and accurate commit history. Whether you need to revert a single commit or a range of commits, Git revert provides a reliable and straightforward solution. Mastering the Git revert command will empower you to confidently manage your project’s history and easily undo any unwanted changes.
Git LFS
Git LFS is an extension to Git that allows you to manage large files more effectively. Instead of storing the actual file content in your Git repository, Git LFS stores a pointer file in Git, while the actual file is stored in a separate storage system. This approach helps to keep your repository size small and improves the performance of Git operations.
Installing Git LFS
Before you can start using Git LFS, you need to install it on your system. The installation process varies depending on your operating system. Visit the Git LFS website for detailed instructions on how to install it.
Configuring Git LFS
Once Git LFS is installed, you need to configure it for your repository. Navigate to your repository’s directory using the command line interface and run the following command:
git lfs install
This command sets up Git LFS for your repository by configuring hooks and filters.
Tracking Large Files
To start managing large files with Git LFS, you need to specify which files should be tracked. You can either explicitly specify the file extensions or use a pattern to match multiple files. For example, to track all PNG images, you can run the following command:
git lfs track "*.png"
This command adds a configuration entry to your repository’s .gitattributes
file, instructing Git LFS to track any file with the .png
extension.
Committing and Pushing Large Files
After tracking the large files, you can commit and push them to your Git repository. Git LFS automatically replaces the large file with a pointer file during the commit process. To commit the changes, use the standard Git commands:
git add . git commit -m "Add large files"
To push the changes, use the git push
command:
git push
Git LFS will handle the large files and upload them to the appropriate storage system, while the pointer files will be stored in your Git repository.
Cloning a Repository with Git LFS
When cloning a repository that uses Git LFS, you need to ensure that Git LFS is installed on your system. After cloning, you can pull the large files using the git lfs pull
command:
git lfs pull
This command downloads the actual file content from the storage system and replaces the pointer files in your local repository.
Working with Git LFS
Git LFS provides additional commands to manage large files in your repository. Here are some commonly used commands:
– git lfs ls-files
: Lists the large files tracked by Git LFS.
– git lfs status
: Shows the status of large files in your repository.
– git lfs logs
: Displays the Git LFS server logs.
– git lfs locks
: Shows the locked large files and their owners.
These commands help you monitor and manage large files efficiently.
Git LFS is a valuable extension to Git that enables efficient management of large binary files. By storing the actual files in a separate storage system and using pointer files in Git, Git LFS helps to keep your repository size small and improves performance.
Git Secrets: Preventing Sensitive Information
Git is a powerful version control system that allows developers to track changes in their codebase and collaborate efficiently. However, when working with Git, it’s important to be cautious about storing sensitive information in your repositories. This chapter will explore various techniques and best practices to prevent sensitive information from being accidentally committed and shared.
1. Gitignore
One of the simplest ways to prevent sensitive information from being added to a Git repository is by using a .gitignore
file. This file specifies intentionally untracked files that Git should ignore when making commits. By adding sensitive files, such as configuration files or API keys, to the .gitignore
file, you can ensure they are not accidentally committed.
Here’s an example of a .gitignore
file that ignores files with .env
extension, which often contains sensitive information:
.env
2. Remove Sensitive Data from Git History
If sensitive information has already been committed to your Git repository, you can remove it from the entire history using the git filter-branch
command. This command allows you to rewrite the entire history of a branch, including all commits and tags.
To remove a specific file containing sensitive information, you can use the following command:
git filter-branch --force --index-filter 'git rm --cached --ignore-unmatch path/to/sensitive-file' --prune-empty --tag-name-filter cat -- --all
It’s important to note that using the git filter-branch
command can alter the repository history and should be used with caution.
3. Git Secrets
Git Secrets is a tool that helps prevent developers from committing sensitive information, such as passwords or API keys, to Git repositories. It scans commits, commit messages, and other Git objects for potential secrets and alerts developers to remove them before making a commit.
To use Git Secrets, you first need to install it by following the instructions provided in the official Git Secrets repository: [https://github.com/awslabs/git-secrets](https://github.com/awslabs/git-secrets)
Once installed, you can initialize Git Secrets in your Git repository by running the following command:
git secrets --install
After initialization, Git Secrets will start scanning your repository for potential secrets every time you make a commit.
4. Encryption and Secrets Management
For additional security, you can encrypt sensitive files and use a secrets management system to store and retrieve decryption keys or other sensitive information at runtime.
One popular approach is to use tools like Ansible Vault or HashiCorp Vault to encrypt sensitive files, such as configuration files or certificates. These tools allow you to securely store encryption keys and secrets, ensuring they are not exposed in your Git repository.
By encrypting sensitive files and using a secrets management system, you can prevent unauthorized access to sensitive information even if your Git repository is compromised.
5. Continuous Integration and Code Analysis
Integrating Git with a continuous integration (CI) system can also help prevent sensitive information from being committed. CI systems can run pre-commit hooks or perform code analysis to check for potential secrets before allowing a commit.
Popular CI systems like Jenkins, GitLab CI/CD, or CircleCI provide options to run pre-commit hooks or perform code analysis using tools like SonarCloud or Snyk. These tools can scan your codebase for potential sensitive information and prevent it from being committed.
By integrating Git with a CI system and using code analysis tools, you can add an extra layer of security to your development workflow.
Preventing sensitive information from being committed to Git repositories is crucial for maintaining the security of your codebase and protecting sensitive data. By utilizing techniques such as .gitignore
, git filter-branch
, Git Secrets, encryption, secrets management, and continuous integration, you can significantly reduce the risk of exposing sensitive information in your Git repositories. Remember to follow best practices and remain vigilant when working with sensitive data in your Git workflow.
Git Bisect: Debugging with Binary Search
In the world of software development, debugging is an essential skill. It involves identifying and fixing issues in code. While there are many debugging techniques available, one powerful tool in the Git arsenal is the “git bisect” command. This command allows developers to perform a binary search through the commit history of a project to identify the exact commit that introduced a bug or regression.
What is Git Bisect?
Git bisect is a command-line tool that helps developers track down bugs by performing a binary search on the commit history. It automates the process of checking out different commits and allows developers to quickly narrow down the commit that introduced the bug.
The binary search algorithm used by git bisect is efficient because it halves the number of possible commits to check with each iteration. By checking out a commit in the middle of the range, git bisect can determine if the bug is present or not. Based on the result, it then halves the range of commits and repeats the process until it identifies the exact commit that introduced the bug.
How to Use Git Bisect
Using git bisect involves three steps: starting the bisect session, marking commits as good or bad, and identifying the problematic commit.
To start a bisect session, you need to specify two commits: a known good commit and a known bad commit. These commits should represent the range within which the bug was introduced. For example:
$ git bisect start $ git bisect good v1.0 $ git bisect bad v1.1
Git bisect will then automatically check out a commit between the good and bad commits. Your task is to test that commit and determine if it is good or bad. If it is good, you mark it as such using:
$ git bisect good
If the commit is bad, you mark it as such using:
$ git bisect bad
Git bisect will then repeat the process, checking out another commit between the remaining range. You continue marking commits as good or bad until git bisect identifies the problematic commit.
Once the problematic commit is identified, you can end the bisect session using:
$ git bisect reset
Practical Example
Let’s say you are working on a project and a bug has been reported. You know that the bug was not present in version 1.0, but it appeared in version 1.1. To identify the problematic commit, you can use git bisect.
$ git bisect start $ git bisect good v1.0 $ git bisect bad v1.1
Git bisect will automatically check out a commit between v1.0 and v1.1. Let’s say it checks out commit “abc123”. You test the code and find that the bug is present. You mark the commit as bad:
$ git bisect bad
Git bisect will then check out another commit between v1.0 and “abc123”. Let’s say it checks out commit “def456”. You test the code and find that the bug is not present. You mark the commit as good:
$ git bisect good
Git bisect will continue this process, automatically checking out commits and narrowing down the range, until it identifies the exact commit that introduced the bug.
Subtree
In Git, a subtree is a way to include the contents of one repository into another repository as a subdirectory. This can be useful when you want to incorporate external code or libraries into your project without having to maintain a separate repository.
Adding a Subtree
To add a subtree to your Git repository, you can use the git subtree add
command followed by the URL of the external repository and the path where you want the subtree to be added. For example, if you want to add the example-repo
as a subtree inside the subdir
directory:
$ git subtree add --prefix=subdir https://github.com/example/example-repo.git master
This command will add the contents of the example-repo
repository’s master
branch to the subdir
directory in your repository. Git will create a commit that adds the subtree and records the commit ID of the external repository.
Updating a Subtree
To update a subtree with the latest changes from the external repository, you can use the git subtree pull
command. This command will fetch the latest changes from the remote repository and merge them into your subtree.
$ git subtree pull --prefix=subdir https://github.com/example/example-repo.git master
If there are any conflicts during the merge, Git will prompt you to resolve them manually.
Pushing Changes to a Subtree
If you want to contribute changes to the external repository from your subtree, you can use the git subtree push
command. This command will push the changes made in the subtree to the remote repository.
$ git subtree push --prefix=subdir https://github.com/example/example-repo.git branch-name
Make sure to replace branch-name
with the branch name in the external repository you want to push the changes to.
Splitting a Subtree
Sometimes you may want to split a subtree into a separate repository. This can be useful when you want to extract a specific part of your project into its own repository. Git provides the git subtree split
command for this purpose.
$ git subtree split --prefix=subdir --branch=new-branch
This command will create a new branch new-branch
containing the commits that belong to the subtree located in the subdir
directory. You can then push this branch to a separate repository if needed.
Removing a Subtree
To remove a subtree from your repository, you can use the git subtree remove
command followed by the path of the subtree.
$ git subtree remove --prefix=subdir
This command will remove the subtree from your repository’s history, but it will not delete the files in the subtree from your local file system.
Git Archive: Exporting Repository Snapshots
Git Archive is a powerful feature that allows you to export a snapshot of your repository without including the entire Git history. This can be useful when you want to share a specific version of your code with someone who doesn’t have access to your Git repository.
To create an archive of your repository, you can use the git archive
command followed by the desired branch or commit. For example, to export the latest version of your code on the master branch, you can run the following command:
$ git archive --format=zip --output=archive.zip master
In this example, the --format
option specifies the format of the archive, which in this case is a zip file. You can also choose other formats such as tar or tar.gz. The --output
option specifies the name of the output file.
If you want to export a specific commit instead of a branch, you can provide the commit hash instead. For example:
$ git archive --format=zip --output=archive.zip 3a2c8d1
This will create an archive containing the state of the repository at the specified commit.
By default, the git archive
command exports the entire repository, including all files and directories. However, you can also specify a subdirectory or a set of files that you want to include in the archive. For example, to export only the files within a src
directory, you can use the --prefix
option:
$ git archive --format=zip --output=archive.zip master --prefix=src/
This will create an archive that contains only the files within the src
directory, preserving the directory structure.
It’s worth noting that the git archive
command does not include any Git-specific metadata in the exported archive. This means that the recipient of the archive will not be able to perform Git operations on it. However, they will have access to the code and can use it as a standalone snapshot.
Git Archive is a handy tool for sharing specific versions of your code, creating release packages, or generating code snapshots for deployment. It allows you to extract a clean and self-contained version of your repository without the entire Git history, making it easier to distribute and use.
To learn more about the git archive
command and its options, you can refer to the official Git documentation: https://git-scm.com/docs/git-archive.
Git Clean: Removing Untracked Files
Git is a powerful version control system that allows you to track changes, collaborate with others, and manage your codebase efficiently. One of the many useful features of Git is the ability to remove untracked files from your repository using the git clean
command.
When you work on a project, you may create temporary files, backups, or other artifacts that are not intended to be part of your version-controlled codebase. These untracked files can clutter your project directory, making it harder to navigate and understand your code. The git clean
command provides an easy and efficient way to remove these untracked files.
To use git clean
, navigate to your project directory using the command line or terminal. Then, run the following command:
git clean [options]
The [options]
parameter is optional and allows you to customize the behavior of the git clean
command. Here are some commonly used options:
– -n
or --dry-run
: This option displays a list of untracked files that would be removed without actually deleting them. It is useful when you want to preview the files that will be deleted before running the command.
– -f
or --force
: This option forces the removal of untracked files without prompting for confirmation. Use this option with caution, as it can delete files permanently.
– -d
or --directory
: This option tells git clean
to also remove untracked directories. By default, git clean
only removes untracked files.
By default, git clean
only removes files and directories that are not being ignored by Git. If you want to remove ignored files as well, you can use the -x
or --ignored
option.
Here are some examples of how to use the git clean
command:
# List untracked files that would be removed git clean -n # Remove untracked files without prompting for confirmation git clean -f # Remove untracked files and directories git clean -fd # Remove ignored files and directories git clean -fxd
It’s important to note that git clean
permanently deletes files from your project directory. Make sure to double-check the list of files that will be removed using the -n
option before running the command with the -f
option.
In some cases, you may want to exclude certain files or directories from being removed by git clean
. You can do this by specifying patterns in a .gitignore
file. Any files or directories that match the patterns in the .gitignore
file will be ignored by the git clean
command.
Git Rerere: Resolving Repeated Conflicts
Git is a powerful version control system that allows multiple developers to work on a project simultaneously. However, conflicts can often arise when merging branches, and resolving these conflicts can be time-consuming and error-prone. Git Rerere (Reuse Recorded Resolution) is a useful feature that can help automate the resolution of repeated conflicts, saving time and effort.
When Git encounters a conflict during a merge or rebase operation, it records the conflict resolution in a special file inside the .git
directory. This recorded resolution can then be reused automatically for future conflicts in the same location. Git Rerere works by identifying the context of the conflict and applying the recorded resolution accordingly.
To enable Git Rerere, you need to run the following command:
$ git config --global rerere.enabled true
Once enabled, Git will start recording conflict resolutions, and you can reuse them later on. Let’s see an example to understand how Git Rerere works.
Imagine you are working on a project with your team, and you encounter a conflict while merging a feature branch. You resolve the conflict manually and complete the merge. Git Rerere will automatically record this resolution for future use.
The next time you encounter a similar conflict in the same location, Git Rerere will apply the recorded resolution automatically. This not only saves time but also ensures consistent conflict resolutions across the team.
Git Rerere also allows you to manually apply a recorded resolution if needed. You can do this by running the following command:
$ git rerere
This command applies the recorded resolution to the current conflict, resolving it automatically. If multiple resolutions are available, Git Rerere will prompt you to choose the appropriate one.
You can also view the recorded resolutions by running the following command:
$ git rerere status
This command displays the status of the recorded resolutions, including the number of recorded resolutions and the paths where they are applicable.
Git Rerere is a powerful feature that can significantly improve the efficiency of conflict resolution in Git. By reusing recorded resolutions, you can save time and reduce the chance of errors when resolving conflicts.
To learn more about Git Rerere and how to use it effectively, you can refer to the official Git documentation on Git Rerere.
Now that you understand how Git Rerere works, let’s move on to the next chapter and explore another advanced hack in Git.
Forking Workflow
Continuous Integration (CI) and Continuous Delivery (CD) are essential practices in modern software development. These workflows help teams deliver high-quality code more efficiently by automating the build, test, and deployment processes. Git, with its powerful branching and merging capabilities, is a perfect fit for implementing CI/CD workflows. In this chapter, we will explore different Git workflows that support CI/CD and discuss best practices for integrating Git with popular CI/CD tools.
The Forking Workflow is a popular Git workflow for open-source projects and large development teams. It allows contributors to clone the main repository, make changes in their own forks, and submit pull requests to merge their changes. This workflow fits well with CI/CD because pull requests can trigger automated tests and deployments.
Here’s an example of how the Forking Workflow works:
1. Fork the main repository on the Git hosting platform (e.g., GitHub, GitLab).
2. Clone your fork to your local machine:
git clone https://github.com/your-username/repository.git
3. Create a new branch for your feature or bug fix:
git checkout -b my-feature
4. Make your changes, commit them, and push to your fork:
git add .
git commit -m "Implement my feature"
git push origin my-feature
5. Open a pull request on the main repository to merge your changes.
Feature Branch Workflow
The Feature Branch Workflow is another popular Git workflow that works well with CI/CD. It encourages developers to create a new branch for each new feature or bug fix. This workflow allows for parallel development and easy integration of changes using pull requests or merge requests.
Here’s an example of how the Feature Branch Workflow works:
1. Clone the main repository to your local machine:
git clone https://github.com/repository.git
2. Create a new branch for your feature or bug fix:
git checkout -b my-feature
3. Make your changes, commit them, and push to the remote branch:
git add .
git commit -m "Implement my feature"
git push origin my-feature
4. Open a pull request or merge request to merge your changes into the main branch.
Git Hooks for CI/CD
Git hooks are scripts that can be executed before or after specific Git operations. They provide a way to automate tasks in Git workflows, including running tests, linting code, and triggering deployments. By leveraging Git hooks, you can seamlessly integrate CI/CD processes into your development workflow.
Here’s an example of a Git hook that runs tests before allowing a commit:
1. Create a file named pre-commit
in the .git/hooks/
directory of your repository.
2. Add the following code to the pre-commit
file:
bash
#!/bin/sh
echo "Running tests..."
npm test
3. Make the pre-commit
file executable:
chmod +x .git/hooks/pre-commit
4. Now, whenever you try to make a commit, the tests will be executed automatically.
Integrating Git with CI/CD Tools
Many popular CI/CD tools provide native integration with Git, making it easy to automate your development workflow. Here are a few examples:
– Jenkins: Jenkins offers extensive Git integration through plugins, allowing you to trigger builds, tests, and deployments based on Git events.
– CircleCI: CircleCI provides built-in support for Git and allows you to configure workflows that automatically build and test your code on every push.
– Travis CI: Travis CI offers seamless integration with Git and lets you define build and deployment steps using a .travis.yml
configuration file in your repository.
These are just a few examples, and there are many other CI/CD tools available that integrate well with Git.