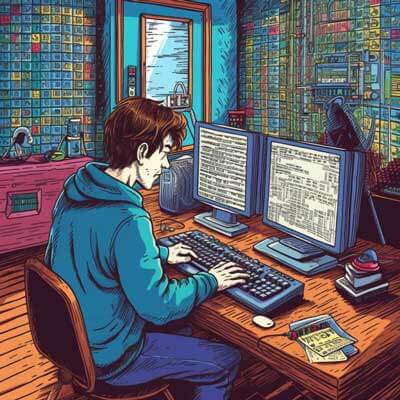
To center a div in a Bootstrap responsive page, there are several approaches you can take. Below are two possible solutions that you can use:
Method 1: Using Bootstrap Flexbox
One way to center a div in a Bootstrap responsive page is by using Bootstrap’s Flexbox utility classes. Flexbox provides a flexible way to align and distribute space among elements in a container.
Here’s an example of how you can center a div horizontally and vertically using Flexbox:
<div class="d-flex justify-content-center align-items-center" style="height: 100vh"> <div class="text-center"> <!-- Your content here --> </div> </div>
In the above example, the outer div with the classes d-flex justify-content-center align-items-center
creates a flex container and aligns its children both horizontally and vertically. The justify-content-center
class horizontally centers the content, and the align-items-center
class vertically centers the content. The height: 100vh
CSS property ensures that the outer div takes up the full height of the viewport.
Related Article: How to Center an Absolutely Positioned Element in a Div
Method 2: Using CSS Margin Auto
Another method to center a div in a Bootstrap responsive page is by using CSS margin: auto
. This approach is particularly useful when you want to center a div horizontally.
Here’s an example of how you can center a div horizontally using margin: auto
:
<div class="container"> <div class="row"> <div class="col-12"> <div class="text-center"> <!-- Your content here --> </div> </div> </div> </div>
In the above example, the outermost container
class provides a fixed-width container for your content. The row
class is used to create a horizontal row, and the col-12
class makes the column take up the full width of the container. Finally, the inner text-center
class centers the content horizontally within the column.
Alternative Approaches
Aside from the methods mentioned above, there are other ways to center a div in a Bootstrap responsive page. Here are a few alternative approaches you can consider:
1. Using CSS Flexbox: You can use pure CSS Flexbox properties to center a div. This approach gives you more flexibility and control over the alignment of elements. You can achieve horizontal and vertical centering using the display: flex
property on the container and various alignment properties.
2. Using CSS Grid: CSS Grid is another useful layout system that can be used to center a div in a Bootstrap responsive page. By defining grid areas and using the place-items: center
property on the container, you can achieve both horizontal and vertical centering.
3. Using JavaScript: If you need more advanced centering techniques or dynamic centering based on the viewport size, you can use JavaScript to calculate and set the proper positioning of the div. You can listen for window resize events and update the position accordingly.
Best Practices
When centering a div in a Bootstrap responsive page, it’s important to consider the following best practices:
1. Use responsive design: Ensure that your centered div adapts well to different screen sizes and orientations. Bootstrap provides a responsive grid system that can help you achieve this.
2. Avoid using fixed dimensions: When centering a div, it’s generally better to use relative units like percentages or viewport units (vh
, vw
, etc.) instead of fixed pixel values. This allows the div to scale properly on different devices.
3. Test on multiple devices: Always test your centered div on different devices and screen sizes to ensure it appears correctly centered across various platforms.
4. Keep accessibility in mind: Ensure that your centered div remains accessible to all users, including those with disabilities. Use proper semantic markup and provide alternative text for images and other media.
Related Article: How to Vertically Align Text in a Div with CSS