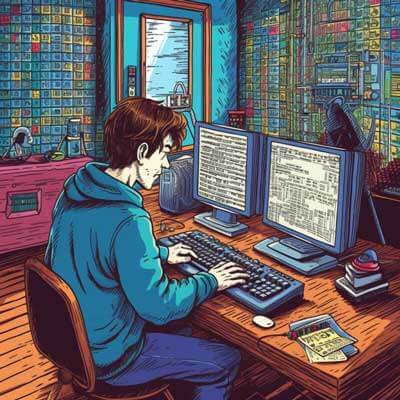
Table of Contents
Introduction to Hex Code Colors
Hex code colors are a widely used method of representing colors in web development. Instead of using traditional color names or RGB values, hex code colors utilize a combination of six alphanumeric characters to define a specific color. Each character represents a different component of the color, including red, green, and blue.
To understand how hex code colors work, let's take a look at an example:
body { background-color: #FF0000; }
In this example, the hex code color #FF0000
represents the color red. The first two characters, FF
, represent the intensity of the red component. The remaining four characters, 0000
, are used to define the green and blue components, which are set to zero in this case.
Related Article: How to Style CSS Scrollbars
Introduction to Alpha Values
Alpha values, also known as opacity values, are used to control the transparency of an element or a color. They allow you to make elements partially or completely transparent, giving you more flexibility in designing your web pages.
In CSS, alpha values can be specified using the rgba
or hsla
notation. The rgba
notation stands for "red, green, blue, alpha," while the hsla
notation stands for "hue, saturation, lightness, alpha."
Let's take a look at an example of using alpha values with the rgba
notation:
body { background-color: rgba(255, 0, 0, 0.5); }
In this example, the alpha value is set to 0.5
, making the background color of the body
element partially transparent. The higher the alpha value, the more opaque the element becomes.
How Hex Code Colors Work
Hex code colors work by combining different levels of red, green, and blue components to create a specific color. Each character in the hex code represents a 4-bit value, ranging from 0 to 15. These values are then converted to an 8-bit value, ranging from 0 to 255, which represents the intensity of each color component.
For example, the hex code color #FF0000
represents full intensity red, while #00FF00
represents full intensity green, and #0000FF
represents full intensity blue. By combining different levels of red, green, and blue, you can create a wide range of colors.
How Alpha Values Impact Colors
Alpha values have a significant impact on the transparency of colors. By adjusting the alpha value, you can make colors partially or completely transparent. This allows you to create effects such as overlays, gradients, and fading animations.
Let's take a look at an example of how alpha values impact colors:
div { background-color: rgba(255, 0, 0, 0.5); }
In this example, the div
element has a background color with an alpha value of 0.5
, making it partially transparent. As a result, the content behind the div
element will be partially visible, creating an overlay effect.
Related Article: How to Style a Disabled Button with CSS
Hex Code Colors with Alpha Values: A Comprehensive Guide
Using hex code colors with alpha values provides a comprehensive solution for controlling the transparency of colors in web development. By combining the power of hex code colors and alpha values, you can create visually appealing designs and effects.
Let's explore the various use cases, best practices, real-world examples, performance considerations, advanced techniques, and error handling related to hex code colors with alpha values.
Use Cases: Designing Transparent Div Elements
One common use case for hex code colors with alpha values is designing transparent div elements. By applying a background color with an alpha value to a div element, you can create overlays, tooltips, or highlight specific areas of your web page.
<div style="width: 200px;height: 200px"></div>
In this example, the div element has a background color with an alpha value of 0.5
, making it partially transparent. You can adjust the alpha value to achieve the desired level of transparency.
Use Cases: Creating a Color Gradient
Another use case for hex code colors with alpha values is creating color gradients. By defining multiple colors with different alpha values, you can achieve smooth transitions between colors, resulting in visually pleasing gradients.
div { background-image: linear-gradient(rgba(255, 0, 0, 0.5), rgba(0, 0, 255, 0.5)); }
In this example, the div element has a background image defined using the linear-gradient function. The gradient starts with a red color with an alpha value of 0.5
and transitions to a blue color with the same alpha value.
Use Case: Adding Alpha Values to Background Colors
Adding alpha values to background colors allows you to control the transparency of elements on your web page. This can be useful when you want to make certain elements stand out while still allowing the underlying content to be visible.
<div style="background-color: #FF000080;width: 200px;height: 200px"></div>
In this example, the div element has a background color of #FF000080
, where 80
represents the alpha value in hex code format. This makes the div element partially transparent, allowing the content behind it to show through.
Related Article: CSS Position Relative: A Complete Guide
Best Practices: Consistent Use of Hex Code Colors
To maintain a consistent design and ensure readability of your code, it's important to use hex code colors consistently throughout your web development projects. Here are some best practices to consider:
- Use a color palette to define a set of colors that align with your design theme.
- Document the hex code colors you use in your project for easy reference.
- Use meaningful variable names when defining colors in CSS to improve code readability.
:root { --primary-color: #FF0000; --secondary-color: #00FF00; --background-color: #000000; } h1 { color: var(--primary-color); } p { color: var(--secondary-color); } body { background-color: var(--background-color); }
In this example, we define three hex code colors using CSS variables. By using variables, we can easily update the colors throughout our project by modifying the variable values in one place.
Best Practices: Using Alpha Values Sparingly
While alpha values provide flexibility in controlling the transparency of colors, it's important to use them sparingly to avoid compromising readability and accessibility. Here are some best practices to follow:
- Use alpha values only when necessary, such as for overlays or specific design effects.
- Consider the readability of text or other content when applying alpha values to background colors.
- Test the accessibility of your design by ensuring that text and interactive elements are still easily visible with the applied alpha values.
Real World Example: Transparent Navigation Menus
Transparent navigation menus can add a modern and visually appealing touch to your website. By applying a background color with an alpha value to the navigation menu, you can create a subtle overlay effect that allows the background image or content to show through.
<nav> <ul> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Services</a></li> </ul> </nav>
In this example, the navigation menu has a background color with an alpha value of 0.5
, making it partially transparent. This creates a subtle overlay effect on top of the content behind the navigation menu.
Real World Example: Semi-Transparent Overlays
Semi-transparent overlays can be used to draw attention to specific sections or elements on your web page. By applying a background color with an alpha value to an overlay element, you can create a visually striking effect.
<div class="overlay"></div> .overlay { position: fixed; top: 0; left: 0; width: 100%; height: 100%; background-color: rgba(0, 0, 0, 0.3); }
In this example, the overlay element has a background color with an alpha value of 0.3
, making it semi-transparent. The overlay covers the entire viewport and draws attention to the content below it.
Related Article: How to Append New Colors in Tailwind CSS Without Removing Originals
Performance Considerations: Impact of Alpha Values on Rendering Time
Using alpha values can have an impact on the rendering time of your web pages. The more complex the transparency effects, the more processing power is required by the browser to render the page.
To minimize the impact on performance, consider the following tips:
- Avoid applying alpha values to elements that don't require transparency.
- Limit the number of elements with complex transparency effects on a single page.
- Optimize your code and assets to improve overall page load time.
Performance Considerations: Hex Code Colors and Browser Compatibility
While hex code colors with alpha values are widely supported by modern browsers, it's important to consider browser compatibility. Older browsers may not fully support alpha values, resulting in unexpected rendering or ignoring the transparency effects altogether.
To ensure broad compatibility, consider the following:
- Test your web pages on multiple browsers and versions.
- Provide fallback options for browsers that don't support alpha values.
- Use CSS preprocessors or postprocessors to automatically generate fallback code when necessary.
Advanced Technique: Creating Complex Color Gradients with Alpha Values
Advanced techniques involving hex code colors with alpha values include creating complex color gradients. By combining multiple colors with different alpha values, you can achieve intricate and visually captivating gradient effects.
div { background-image: linear-gradient(rgba(255, 0, 0, 0.5), rgba(0, 255, 0, 0.5), rgba(0, 0, 255, 0.5)); }
In this example, the div element has a background image defined using the linear-gradient function. The gradient transitions from red to green to blue, with each color having the same alpha value of 0.5
.
Advanced Technique: Using Alpha Values for Animation Effects
Alpha values can also be used to create animation effects. By animating the alpha value of an element's background color, you can achieve fading, pulsating, or other visually dynamic effects.
@keyframes pulsate { 0% { background-color: rgba(255, 0, 0, 0.5); } 50% { background-color: rgba(255, 0, 0, 1); } 100% { background-color: rgba(255, 0, 0, 0.5); } } div { animation: pulsate 2s infinite; }
In this example, we define a keyframe animation called pulsate
that animates the alpha value of the div element's background color. The animation cycles between an alpha value of 0.5
and 1
, creating a pulsating effect.
Related Article: How to Center a Position Absolute Element in CSS
Code Snippet: Basic Hex Code with Alpha Value
p { color: #FF000080; }
In this code snippet, the paragraph element has a text color defined using a hex code with an alpha value of 80
. The alpha value makes the text partially transparent, allowing the underlying content to show through.
Code Snippet: Transparent Div Element
<div style="width: 200px;height: 200px"></div>
In this code snippet, the div element has a background color with an alpha value of 0.5
, making it partially transparent. The div element has a width and height of 200px
, but you can adjust these values to fit your specific needs.
Code Snippet: Background Color with Alpha Value
<div style="background-color: #00000080;width: 200px;height: 200px"></div>
In this code snippet, the div element has a background color of #00000080
, where 80
represents the alpha value in hex code format. This makes the div element partially transparent, allowing the content behind it to show through.
Code Snippet: Color Gradient with Alpha Value
div { background-image: linear-gradient(rgba(255, 0, 0, 0.5), rgba(0, 0, 255, 0.5)); }
In this code snippet, the div element has a background image defined using the linear-gradient function. The gradient starts with a red color with an alpha value of 0.5
and transitions to a blue color with the same alpha value.
Related Article: How to Implement an Onclick Effect in CSS
Code Snippet: Animation Effect with Alpha Value
@keyframes fade-in-out { 0% { opacity: 1; } 50% { opacity: 0.5; } 100% { opacity: 1; } } div { animation: fade-in-out 2s infinite; }
In this code snippet, we define a keyframe animation called fade-in-out
that animates the opacity of the div element. The animation cycles between an opacity value of 1
and 0.5
, creating a fading in and out effect.
Error Handling: Debugging Hex Code Colors
When working with hex code colors, it's important to ensure that you're using the correct format and values. Here are some common issues and how to debug them:
- Double-check that the hex code is preceded by a #
symbol.
- Verify that the hex code has exactly six characters after the #
symbol.
- Use a color picker tool or a browser extension to visually inspect the colors on your web page.
Error Handling: Dealing with Unsupported Alpha Values
In some cases, older browsers or certain CSS properties may not fully support alpha values. When encountering unsupported alpha values, consider the following options:
- Provide fallback options by using solid colors instead of transparent colors.
- Use feature detection techniques to apply alternative styles or effects for unsupported browsers.
- Educate your users about the limitations of certain browsers and encourage them to use modern, up-to-date browsers.