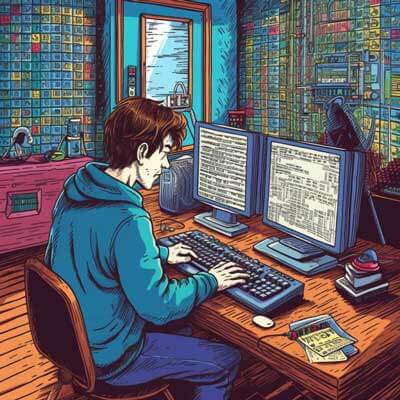
To horizontally center a <div>
element in CSS, you can use one of the following methods:
Method 1: Using margin: auto;
You can use the margin
property with the value auto
to horizontally center a <div>
. Here’s how you can do it:
<div style="margin: 0 auto">Centered div</div>
In the above example, we set the margin
property of the <div>
to 0 auto
. The 0
value for the top and bottom margins ensures that the <div>
doesn’t have any vertical spacing. The auto
value for the left and right margins automatically distributes the remaining space equally on both sides, effectively centering the <div>
horizontally.
Related Article: How to Center an Absolutely Positioned Element in a Div
Method 2: Using flexbox;
Another way to horizontally center a <div>
is by using flexbox. Here’s an example:
<div style="justify-content: center">Centered div</div>
In the above example, we set the display
property of the <div>
to flex
to create a flex container. The justify-content
property with the value center
aligns the flex items (in this case, the <div>
) along the horizontal axis, effectively centering it.
Best Practices:
– It’s recommended to use CSS classes instead of inline styles for better maintainability and reusability. For example:
.centered { margin: 0 auto; display: flex; justify-content: center; } <div class="centered">Centered div</div>
– If you want to center a <div>
both horizontally and vertically, you can use the align-items
property in combination with justify-content
. For example:
.centered { display: flex; justify-content: center; align-items: center; } <div class="centered">Centered div</div>
– If you need to support older browsers that don’t fully support flexbox, you can use a combination of display: table
and text-align: center
to achieve horizontal centering. However, this method is less flexible compared to flexbox. Here’s an example:
.centered { display: table; margin: 0 auto; text-align: center; } <div class="centered">Centered div</div>
Alternative Ideas:
– Another approach to horizontally center a <div>
is by using the position
property. You can set position: absolute
on the <div>
and then set left: 50%
and transform: translateX(-50%)
to center it. However, this method requires the parent container to have a non-static position (e.g., position: relative
).
.parent-container { position: relative; } .centered { position: absolute; left: 50%; transform: translateX(-50%); } <div class="parent-container"> <div class="centered">Centered div</div> </div>
– If you only need to center a single line of text within a <div>
, you can use the text-align
property instead. For example:
<div style="text-align: center"><a href="https://www.squash.io/centering-text-with-css-tricks-and-techniques/">Centered text</a></div>
Related Article: How to Vertically Align Text in a Div with CSS