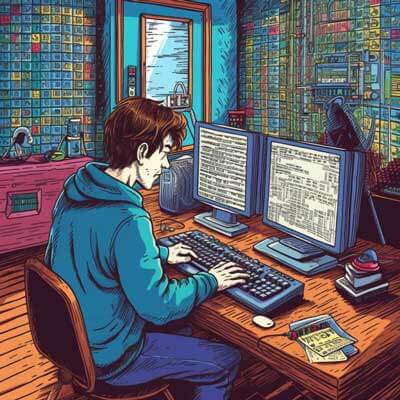
There are several methods you can use to vertically center text with CSS. In this answer, we will explore two common techniques: using flexbox and using CSS grid.
Using Flexbox
Flexbox is a useful CSS layout module that provides a simple way to vertically center elements. Here’s how you can use flexbox to vertically center text:
1. Create a container element that wraps the text you want to center. For example, let’s say you have the following HTML structure:
<div class="container"> <p class="centered-text">This is vertically centered text.</p> </div>
2. Apply the following CSS styles to the container element:
.container { display: flex; justify-content: center; align-items: center; }
The display: flex;
property makes the container a flex container, and the justify-content: center;
and align-items: center;
properties horizontally and vertically center the child elements within the container, respectively.
3. Optionally, you can add additional styles to the .centered-text
class to adjust the appearance of the text:
.centered-text { font-size: 24px; font-weight: bold; }
That’s it! The text will now be vertically centered within the container. You can adjust the styles and dimensions according to your needs.
Related Article: How to Center an Absolutely Positioned Element in a Div
Using CSS Grid
CSS Grid is another useful CSS layout module that provides a flexible way to create grid-based layouts. Here’s how you can use CSS grid to vertically center text:
1. Create a container element that wraps the text you want to center. For example:
<div class="container"> <p class="centered-text">This is vertically centered text.</p> </div>
2. Apply the following CSS styles to the container element:
.container { display: grid; place-items: center; }
The display: grid;
property makes the container a grid container, and the place-items: center;
property centers the child elements both horizontally and vertically within the container.
3. Optionally, you can add additional styles to the .centered-text
class to customize the appearance of the text:
.centered-text { font-size: 24px; font-weight: bold; }
That’s it! The text will now be vertically centered within the container using CSS grid. You can adjust the styles and dimensions as needed.
Alternative Methods
In addition to flexbox and CSS grid, there are a few other techniques you can use to vertically center text with CSS:
– Using the line-height
property: You can vertically center a single line of text by setting the line-height
property of the text element to be equal to the height of its container. For example:
.container { height: 200px; } .centered-text { line-height: 200px; }
– Using absolute positioning: If you know the height of the container element and the height of the text element, you can use absolute positioning to center the text vertically. For example:
.container { position: relative; height: 200px; } .centered-text { position: absolute; top: 50%; transform: translateY(-50%); }
These are just a few alternative methods you can use to vertically center text with CSS. Choose the technique that best suits your specific requirements and layout.
Best Practices
When vertically centering text with CSS, keep the following best practices in mind:
– Use appropriate container elements: Wrap the text you want to center in a container element to ensure that the centering styles are applied correctly.
– Consider responsive design: If your layout needs to be responsive, make sure to use media queries or other responsive techniques to adjust the centering styles based on different screen sizes.
– Test across browsers: Different browsers may interpret CSS rules differently, so it’s important to test your vertically centering styles across multiple browsers to ensure consistent behavior.
– Avoid excessive nesting: Try to keep your HTML structure clean and avoid excessive nesting of container elements, as it can make your code harder to maintain and understand.
Related Article: How to Vertically Align Text in a Div with CSS