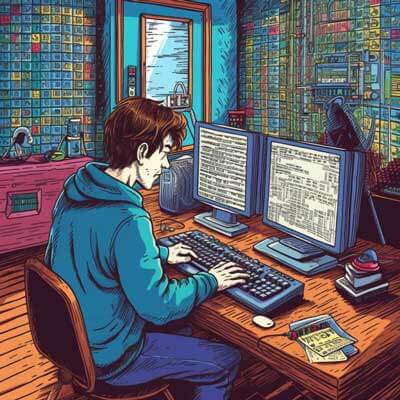
To implement an onclick effect in CSS, you can use the :active pseudo-class or JavaScript. Both methods can achieve the desired effect, but they offer different levels of flexibility and functionality. Let’s explore each approach in detail.
Using the :active Pseudo-class
The :active pseudo-class is a CSS selector that matches an element during the time it is being activated by the user. This can be when the user clicks on the element or presses it using a keyboard or touchscreen device. To implement an onclick effect using the :active pseudo-class, follow these steps:
1. Identify the element you want to apply the onclick effect to. This can be any HTML element, such as a button, link, or div.
2. Add the CSS property and value to the element’s style or CSS file. For example, if you want to change the background color of a button when it is clicked, you can use the following code:
button:active { background-color: red; }
3. Customize the CSS properties and values according to your desired onclick effect. You can change any CSS property, such as color, font-size, opacity, or transform. Experiment and combine different properties to achieve the desired visual effect.
Related Article: How to Hide Scroll Bar in CSS While Maintaining Scroll Functionality
Using JavaScript
If you require more complex interactions or want to manipulate the DOM dynamically based on user clicks, JavaScript is a useful tool. Here’s how you can implement an onclick effect using JavaScript:
1. Identify the element you want to apply the onclick effect to, just like in the previous method.
2. Add an onclick event handler to the element. This can be done inline in the HTML or through a separate JavaScript file. Here’s an example of an inline onclick event handler for a button:
<button>Click me</button>
3. Write the JavaScript function that will be triggered when the element is clicked. This function can manipulate the DOM, change styles, or perform any other desired actions. For example, to change the background color of the button when clicked, you can use the following JavaScript code:
function changeBackgroundColor() { var button = document.querySelector('button'); button.style.backgroundColor = 'red'; }
4. Customize the JavaScript function according to your desired onclick effect. You can access and manipulate any element or property within the DOM using JavaScript. Take advantage of JavaScript libraries and frameworks, such as jQuery or React, if they suit your project’s needs.
Best Practices and Suggestions
– Use the :active pseudo-class for simple onclick effects that only involve visual changes, such as changing colors or backgrounds.
– JavaScript should be used when you need more complex interactions, such as toggling classes, showing/hiding elements, or making API requests.
– When using JavaScript, consider using event delegation for better performance. Instead of attaching an onclick event handler to each element, you can attach a single event handler to a parent element and use event.target to identify the clicked element.
– Always test your onclick effects on different browsers and devices to ensure compatibility. Test for responsiveness and accessibility, keeping in mind that not all users interact with a mouse.
– Consider using CSS transitions or animations in combination with onclick effects to provide a smoother and more engaging user experience.
– Avoid relying solely on onclick effects for critical functionality. Always provide alternative ways for users to achieve the same outcome, such as keyboard shortcuts or accessible buttons.
Related Article: How to Fix CSS Text Overflow Ellipsis Issue