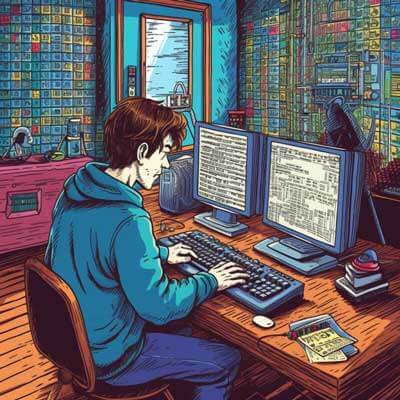
Table of Contents
Getting Started with CSS
CSS, or Cascading Style Sheets, is a powerful tool for controlling the visual appearance of HTML elements. It allows you to define styles such as colors, fonts, and layouts for your web page. We will cover the basics of CSS and how to use it to center an image on a web page.
To get started, you need to have a basic understanding of HTML. If you are new to HTML, we recommend checking out the W3Schools HTML tutorial.
CSS can be applied in three different ways: inline, embedded, or external. Inline styles are applied directly to individual HTML elements using the "style" attribute. Embedded styles are defined within the "style" tags in the "head" section of an HTML document. External styles are stored in a separate CSS file and linked to the HTML document using the "link" tag.
Let's take a look at an example of an embedded style:
img { display: block; margin-left: auto; margin-right: auto; } <img src="image.jpg" alt="Centered Image">
In this example, we have defined a CSS rule for the "img" element. The "display: block;" property ensures that the image is displayed as a block-level element, which allows us to apply margins. The "margin-left: auto;" and "margin-right: auto;" properties set the left and right margins to automatic, which centers the image horizontally within its container.
By setting the "margin-top" and "margin-bottom" properties to "auto" as well, you can center the image both horizontally and vertically within its container.
To apply an external style sheet to your HTML document, you need to create a separate CSS file with the ".css" extension. Let's say we have created a file called "styles.css" with the following content:
img { display: block; margin-left: auto; margin-right: auto; }
To link this external CSS file to your HTML document, you can use the following code within the "head" section:
Now, the styles defined in the "styles.css" file will be applied to the HTML document.
We have covered the basics of CSS and how to use it to center an image on a web page. We have learned about inline, embedded, and external styles, and how to define CSS rules to center an image horizontally and vertically. We will explore more advanced techniques for centering images using CSS.
Related Article: CSS Position Relative: A Complete Guide
Understanding the Box Model
The box model is a fundamental concept in CSS that defines how elements are rendered on a web page. It consists of four components: content, padding, border, and margin. Each of these components contributes to the total width and height of an element.
By understanding the box model, you'll be able to effectively center an image or any other element on a web page.
The Box Model Components
1. Content: The content area of an element is where the actual content, such as text or an image, is displayed. The width and height of this area can be explicitly set using CSS properties like width
and height
.
2. Padding: The padding area surrounds the content and provides space between the content and the border. It can be set using the padding
property and its shorthand variations like padding-top
, padding-right
, padding-bottom
, and padding-left
.
3. Border: The border area surrounds the padding and content and provides a visible boundary for the element. It can be set using the border
property and its shorthand variations like border-width
, border-style
, and border-color
.
4. Margin: The margin area surrounds the border and provides space between the element and other elements on the page. It can be set using the margin
property and its shorthand variations like margin-top
, margin-right
, margin-bottom
, and margin-left
.
Centering an Image Using the Box Model
To center an image using the box model, you can make use of the margin
property. By setting the left and right margins to auto
and the display property of the image to block
, the image will be horizontally centered within its parent container.
Here's an example of how you can center an image using CSS:
img { display: block; margin-left: auto; margin-right: auto; }
In the example above, the display: block
property ensures that the image is treated as a block-level element, allowing us to set the left and right margins to auto
. The margin-left: auto
and margin-right: auto
properties then center the image horizontally within its parent container.
Keep in mind that this method will only center the image horizontally. If you also want to center it vertically, you can use additional CSS techniques like flexbox or CSS Grid.
Related Article: How To Add a Border Inside a Div: CSS Border Inside
Using Margin and Padding
Margin and padding are two CSS properties that can be used to center an image within its container.
Margin
The margin property allows you to control the space around an element. By setting the left and right margins of an image to "auto" and giving it a specific width, you can center the image horizontally within its container.
Here's an example:
img { width: 300px; margin-left: auto; margin-right: auto; }
In this example, the image has a width of 300 pixels. By setting the left and right margins to "auto", the browser will automatically calculate and distribute the remaining space equally on each side, effectively centering the image.
Padding
The padding property controls the space between the content of an element and its border. By applying equal padding values to the top and bottom, and setting the left and right padding values to "auto", you can center the image horizontally and vertically within its container.
Here's an example:
.container { display: flex; justify-content: center; align-items: center; height: 400px; padding: 50px auto; } img { max-width: 100%; max-height: 100%; }
In this example, the container element has a fixed height of 400 pixels. By setting the padding to "50px auto", the browser will automatically calculate and distribute the remaining vertical space equally on the top and bottom, effectively centering the image vertically. The display: flex;
property and the justify-content: center;
and align-items: center;
properties are used to center the image horizontally within the container.
Using margin and padding properties provides flexible options for centering images within their containers. Experiment with different values to achieve the desired centering effect.
Centering an Image Horizontally
To center an image horizontally on a webpage, you can use CSS along with a few different techniques. Here are two commonly used methods:
1. Using margin: auto;
One way to center an image horizontally is by using the margin: auto;
property. This method works by setting the left and right margins of the image to "auto", which causes the image to be centered within its parent container.
img { display: block; margin-left: auto; margin-right: auto; }
In the above example, we set the display
property to block
to ensure that the image is treated as a block-level element. Then, we set both the left and right margins to auto
, which centers the image horizontally.
2. Using flexbox:
Another method to center an image horizontally is by using flexbox. Flexbox is a powerful CSS layout model that provides a flexible way to distribute space among elements.
.container { display: flex; justify-content: center; } img { align-self: center; }
In the above example, we first create a container element with the class name "container". We set the display
property to flex
to enable flexbox on the container. Then, we use the justify-content
property with a value of center
to horizontally center the contents of the container.
To center the image specifically, we set the align-self
property on the image to center
. This aligns the image vertically within the container.
These methods will center an image horizontally on a webpage. You can choose the technique that best suits your needs and the overall layout of your website. Experiment with these methods to find the one that provides the desired result.
Remember to apply these CSS styles to the appropriate selectors in your HTML markup. You can target an image directly by its element name, class, or ID, depending on your specific requirements.
Now that you know how to center an image horizontally using CSS, we'll explore how to center an image vertically.
# Centering an Image Vertically
In addition to centering an image horizontally, you may also want to center it vertically within its container. This can be useful when you have a container with a fixed height and want the image to be perfectly centered vertically within that container.
To center an image vertically, we can use a combination of CSS positioning properties and techniques. Here are a few methods you can try:
## Method 1: Flexbox
One of the easiest ways to center an image vertically is by using flexbox. Flexbox provides a simple and flexible way to align items within a container.
Here's an example of how to center an image vertically using flexbox:
.container { display: flex; justify-content: center; align-items: center; height: 300px; /* adjust the height to your needs */ } .container img { max-width: 100%; max-height: 100%; }
In this example, we set the container's display property to flex
and use justify-content: center
and align-items: center
to horizontally and vertically center the image within the container. Adjust the height
property of the container to suit your needs.
## Method 2: Position and Transform
Another method to center an image vertically is by using absolute positioning and the transform
property. This method works well when you know the height of the container.
Here's an example of how to center an image vertically using position and transform:
.container { position: relative; height: 300px; /* adjust the height to your needs */ } .container img { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); max-width: 100%; max-height: 100%; }
In this example, we set the container's position property to relative
and set the image's position to absolute
. By using top: 50%
and left: 50%
, we position the image at the center of the container. The transform: translate(-50%, -50%)
property then moves the image back by half of its width and height, effectively centering it vertically.
## Method 3: Table Display
If you need to support older browsers, you can also center an image vertically using table display properties.
Here's an example of how to center an image vertically using table display:
.container { display: table; width: 100%; height: 300px; /* adjust the height to your needs */ } .container img { display: table-cell; vertical-align: middle; max-width: 100%; max-height: 100%; }
In this example, we set the container's display property to table
and give it a width of 100%
. By setting the image's display property to table-cell
and using vertical-align: middle
, we can center the image vertically within the container.
Related Article: How to Make a Div Vertically Scrollable Using CSS
Centering an Image Horizontally and Vertically
Centering an image horizontally and vertically on a web page can be achieved using CSS. There are a few different techniques you can use depending on your specific requirements.
1. Using Flexbox:
Flexbox is a powerful CSS layout module that makes it easy to center elements both horizontally and vertically. To center an image using flexbox, you can wrap it in a container element and apply the following CSS:
.container { display: flex; justify-content: center; align-items: center; }
By setting the display
property to flex
, the container becomes a flex container. The justify-content: center
property centers the image horizontally, while the align-items: center
property centers it vertically.
2. Using Absolute Positioning and Transform:
Another way to center an image is by using absolute positioning and the transform
property. This technique is useful when you want to center an image within a specific container. Apply the following CSS to the container:
.container { position: relative; } .img-center { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); }
In this example, the container element is set to position: relative
to establish it as the reference point for the absolute positioning of the image. The top: 50%
and left: 50%
properties position the image at the center of the container. The transform: translate(-50%, -50%)
property then adjusts the image's position so that it is perfectly centered both horizontally and vertically.
3. Using Grid:
If you are using CSS Grid layout, you can center an image by creating a grid and placing the image in the middle cell. Here's an example:
.container { display: grid; place-items: center; }
By setting the display
property to grid
, the container becomes a grid container. The place-items: center
property centers the image both horizontally and vertically within the grid.
These are just a few techniques you can use to center an image both horizontally and vertically using CSS. Choose the one that best fits your needs and give it a try!
To learn more about CSS positioning and layout techniques, check out the MDN web docs.
Using Flexbox for Image Centering
Flexbox is a powerful CSS layout module that provides a flexible way to align and distribute space among elements in a container. It can also be used to center an image both horizontally and vertically within its container.
To use Flexbox for image centering, you need to create a container element and apply the appropriate Flexbox properties to it. Here's an example:
.container { display: flex; justify-content: center; align-items: center; }
In the above code, we have a container with the class name "container". The display: flex;
property makes the container a flex container, enabling the use of Flexbox properties. The justify-content: center;
property horizontally centers the image within the container, while the align-items: center;
property vertically centers the image.
Now, let's apply this container to an image element:
<div class="container"> <img src="image.jpg" alt="Centered Image"> </div>
By wrapping the image element with the container, the image will be centered both horizontally and vertically within the container. You can adjust the size of the container and the image as needed.
Flexbox also provides additional properties that allow you to control the behavior of the elements within the container. For example, you can use the flex-direction
property to change the direction of the flex container, or the flex-wrap
property to control whether the elements should wrap or not.
.container { display: flex; justify-content: center; align-items: center; flex-direction: column; flex-wrap: wrap; }
In the above code, the flex-direction: column;
property changes the direction of the flex container to vertical, and the flex-wrap: wrap;
property allows the elements to wrap onto multiple lines if needed.
Flexbox provides a flexible and powerful way to center an image both horizontally and vertically within its container. It is widely supported by modern browsers, making it a reliable and convenient method for image centering in CSS.
To learn more about Flexbox and its various properties, you can refer to the MDN documentation or other online resources.
Using Grid for Image Centering
One of the most flexible and powerful ways to center an image in CSS is by using the CSS Grid layout. CSS Grid allows you to create a grid of columns and rows, and then place elements within those grid areas. By utilizing Grid, you can easily center an image both horizontally and vertically.
To center an image using Grid, follow these steps:
1. Create a container element and apply the display: grid;
property to it.
.container { display: grid; }
2. Specify the number of columns and rows you want in your grid. In this example, we'll use a single row and a single column.
.container { display: grid; grid-template-columns: 1fr; grid-template-rows: 1fr; }
3. Place the image element within the grid area using the grid-column
and grid-row
properties. Set both properties to 1
to ensure the image occupies the entire grid.
.container { display: grid; grid-template-columns: 1fr; grid-template-rows: 1fr; } .image { grid-column: 1; grid-row: 1; }
4. Finally, use the justify-self
and align-self
properties to center the image horizontally and vertically within the grid area.
.container { display: grid; grid-template-columns: 1fr; grid-template-rows: 1fr; } .image { grid-column: 1; grid-row: 1; justify-self: center; align-self: center; }
And that's it! Your image will now be centered within the Grid container both horizontally and vertically. Adjust the number of columns and rows in the grid as needed, depending on your layout requirements.
By using Grid, you have the flexibility to create more complex layouts and easily center images within them. Experiment with different grid configurations to achieve the desired positioning for your images.
In the next section, we'll explore another technique for centering images in CSS.
Using Positioning for Image Centering
When it comes to centering an image using CSS, one popular method is to use positioning. By setting the left and right margins of an image to "auto" and applying a positioning method, we can easily achieve a centered image.
To begin, let's take a look at the HTML structure for our image:
<div class="container"> <img src="path/to/image.jpg" alt="Centered Image"> </div>
In this example, we have wrapped our image inside a container div, which will help with the positioning. Now let's move on to the CSS.
.container { position: relative; } .container img { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); }
In the CSS snippet above, we first set the position of the container div to "relative". This is necessary to establish a positioning context for the child image.
Next, we target the image within the container using the .container img
selector. We set its position to "absolute", which allows us to manipulate its position freely. By setting the top and left properties to 50%, we move the image to the center of the container.
To fine-tune the centering, we use the transform
property with the translate
function. This function allows us to shift the image by a specified percentage of its own size. By translating the image 50% to the left and 50% to the top, we effectively center it within the container.
Once you apply these CSS rules, you should see your image perfectly centered within its container. Remember to adjust the dimensions of the container div and the image itself to suit your specific design requirements.
Using positioning for image centering provides a reliable and flexible method that works well across different browsers and screen sizes. It is a useful technique to have in your CSS toolbox when you need to center an image in your web projects.
Related Article: How to Implement an Onclick Effect in CSS
Using Transform for Image Centering
Sometimes, you may need to center an image both horizontally and vertically on a webpage. While there are multiple ways to achieve this, one popular method is to use the CSS transform
property.
The transform
property allows you to apply various transformations to an element, such as scaling, rotating, and translating. In this case, we can use the translate
transformation to center an image.
To center an image horizontally, you can use the following CSS code:
.image-container { position: relative; } .image { position: absolute; left: 50%; transform: translateX(-50%); }
In this example, we first set the parent container's position to relative
. This is important because the child image will be positioned relative to its parent container.
Next, we set the child image's position to absolute
. This removes the image from the normal document flow, allowing us to position it freely.
By setting the left
property to 50%
, we move the image halfway across the parent container horizontally. However, the image is not perfectly centered yet.
To center the image completely, we use the transform
property with the translateX(-50%)
value. This moves the image back by 50% of its own width, effectively centering it horizontally.
To center an image both horizontally and vertically, you can use the following CSS code:
.image-container { display: flex; justify-content: center; align-items: center; }
In this example, we utilize the power of flexbox to easily center the image both horizontally and vertically. By setting the parent container's display
property to flex
, we create a flex container.
The justify-content: center;
property centers the items along the main axis (horizontally in this case), and the align-items: center;
property centers the items along the cross axis (vertically in this case). This effectively centers the image both horizontally and vertically within the container.
It's worth noting that the second method is more straightforward and generally recommended for centering images. However, the first method using the transform
property can be useful in situations where you need more control over the positioning or want to center only horizontally.
Now that you know how to center an image using the transform
property, you can easily achieve the desired layout for your webpage.
Handling Responsive Image Centering
Centering an image is a common requirement when designing responsive web pages. In this section, we will discuss different methods for handling responsive image centering using CSS.
Method 1: Margin Auto
One straightforward way to center an image horizontally is by using the margin
property with the value auto
. This method works well when the image has a fixed width.
img { display: block; margin-left: auto; margin-right: auto; }
In the above example, we set the display
property to block
to ensure that the image is treated as a block-level element. Then, we set both left and right margins to auto
, which automatically distributes the available space equally on both sides, effectively centering the image.
Method 2: Flexbox
Flexbox is a powerful CSS layout module that provides a flexible way to handle centering elements. To center an image using flexbox, we need to apply flexbox properties to the parent container.
.container { display: flex; justify-content: center; align-items: center; }
In the above example, we create a container with the class name .container
and set its display
property to flex
. Then, we use justify-content: center
to horizontally center the image and align-items: center
to vertically center it within the container.
Related Article: How to Right Align Div Elements in CSS
Method 3: Grid
CSS Grid is another modern layout module that can be used to center images. Similar to flexbox, we apply grid properties to the parent container.
.container { display: grid; place-items: center; }
In the above example, we set the display
property of the container to grid
. Then, we use place-items: center
to center the image both horizontally and vertically within the container.
Method 4: Absolute Positioning
If you need to center an image within a specific container or element, you can use absolute positioning.
.container { position: relative; } img { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); }
In the above example, we set the position
property of the container to relative
to establish it as the reference point for absolute positioning. Then, we apply absolute positioning to the image by setting its position
property to absolute
. We use the top: 50%
and left: 50%
properties to position the image's top left corner at the center of the container. Finally, we use the transform
property with translate(-50%, -50%)
to shift the image back by 50% of its own width and height, effectively centering it.
These are just a few methods you can use to center images in CSS for responsive web design. Depending on your specific requirements and the layout of your page, you can choose the most appropriate method. Experiment with these techniques to achieve the desired image centering effect on your website.
Common Issues and Troubleshooting
When centering an image in CSS, you may encounter some common issues. In this section, we will discuss these issues and provide troubleshooting tips to help you overcome them.
1. Image not centered horizontally:
If your image is not centered horizontally, there are a few possible reasons for this. One common mistake is forgetting to set the parent container's positioning to either "relative" or "absolute". Without a proper positioning, the image will not be able to center itself within the container. To fix this, ensure that the parent container has one of these positioning values:
.container { position: relative; /* or absolute */ }
Another reason for the image not being centered horizontally could be due to a width constraint on the parent container. Ensure that the container has enough width to accommodate the image. If necessary, adjust the container's width or use the max-width
property to allow the image to scale down if needed:
.container { max-width: 100%; /* or a specific value */ }
2. Image not centered vertically:
Centering an image vertically can be a bit trickier. One common mistake is using the vertical-align
property to center the image. However, this property only works on inline or table-cell elements, and not on block-level elements like <div>
. To center an image vertically, you can use the following CSS:
.container { display: flex; align-items: center; }
This code snippet uses flexbox to vertically center the image within the container. The align-items: center
property aligns the items vertically in the center of the container.
3. Image overflowing or not resizing properly:
Sometimes, when centering an image, it may overflow or not resize properly. This can occur when the image's dimensions are larger than the container's dimensions. To ensure that the image resizes properly, you can use the max-width
and max-height
properties to set a maximum size for the image:
.container img { max-width: 100%; max-height: 100%; }
By setting both properties to 100%, the image will resize proportionally to fit within the container, preventing any overflow.
4. Image not centered on mobile devices:
If your image is centered on desktop but not on mobile devices, it could be due to the parent container's width being set to a fixed value. To ensure that the image stays centered on mobile devices, you can use media queries to adjust the container's width based on the device's screen size:
@media (max-width: 768px) { .container { width: 100%; } }
In this example, the container's width is set to 100% when the device's screen size is 768 pixels or less, ensuring that the image remains centered on smaller screens.
5. Browser compatibility issues:
Lastly, it's important to consider browser compatibility when centering an image using CSS. While most modern browsers support the techniques discussed in this guide, older versions of Internet Explorer may require different approaches. If you need to support older browsers, you can use CSS vendor prefixes or consider using a CSS framework or library that provides cross-browser compatibility.
We covered some common issues and troubleshooting tips when centering an image in CSS. By applying these solutions, you'll be able to overcome any challenges you may encounter while centering images on your web pages.
Real World Examples of Image Centering
In the previous section, we discussed the different CSS techniques for centering images. Now, let's take a look at some real-world examples that demonstrate how to center an image using CSS.
Example 1: Centering an Image Using the Flexbox Layout
The flexbox layout is a powerful CSS feature that makes it easy to center elements, including images. To center an image using flexbox, you can use the following CSS code:
.container { display: flex; justify-content: center; align-items: center; }
In this example, we define a container element with the class "container". By setting the display property to flex, we activate the flexbox layout. The justify-content property is set to center, which horizontally centers the image within the container. The align-items property is also set to center, which vertically centers the image within the container.
Example 2: Centering an Image Using the Grid Layout
Another modern CSS feature for centering images is the grid layout. With the grid layout, you can create complex grid structures and easily center elements within them. Here's an example of how to center an image using the grid layout:
.container { display: grid; place-items: center; }
In this example, we define a container element with the class "container". By setting the display property to grid, we activate the grid layout. The place-items property is set to center, which horizontally and vertically centers the image within the container.
Example 3: Centering an Image Using Absolute Positioning
If you need to center an image within a specific container, you can use absolute positioning. Here's an example of how to center an image using absolute positioning:
.container { position: relative; } .image { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); }
In this example, we define a container element with the class "container" and set its position property to relative. This allows us to position the image within the container using absolute positioning.
The image itself has the class "image" and its position is set to absolute. The top and left properties are set to 50%, which positions the image at the center of the container horizontally and vertically. The transform property with the translate(-50%, -50%) value further adjusts the position of the image to ensure it is perfectly centered.
These are just a few examples of how to center an image using CSS. Depending on the specific requirements of your project, you may need to adjust the CSS properties or use alternative techniques. However, these examples should give you a good starting point for centering images in your own web development projects.
Now that you have learned different techniques for centering images in CSS, you can choose the one that best suits your needs and create visually appealing designs for your websites or web applications.
Related Article: How to Make the First Character Uppercase in CSS
Advanced Techniques for Image Centering
When it comes to centering images in CSS, there are a few more advanced techniques you can use to achieve different effects and improve the overall presentation of your website. Let's explore some of these techniques in detail.
Flexbox Centering
Flexbox is a powerful layout model in CSS that allows you to easily center elements. To center an image using flexbox, you can create a flex container and use the justify-content
and align-items
properties to center the image both horizontally and vertically.
.container { display: flex; justify-content: center; align-items: center; }
This will center the image within the container, regardless of its size. You can also adjust the alignment as needed, such as using justify-content: flex-start
to align the image to the left.
Grid Centering
Similar to flexbox, CSS Grid provides a powerful layout system that can be used to center images. Using the place-items
property on a grid container, you can easily center the image horizontally and vertically.
.container { display: grid; place-items: center; }
This will center the image within the container, regardless of its size. You can also adjust the alignment as needed, such as using align-items: start
to align the image to the top.
Transform Centering
Another technique to center an image is by using the transform
property. By applying a combination of translate
and left: 50%
, you can center the image horizontally.
.container { position: relative; } .image { position: absolute; left: 50%; transform: translateX(-50%); }
This technique is particularly useful when you need to center an image within a parent container that has a fixed width.
Related Article: How to Vertically Center Text with CSS
Centering with Margin
Alternatively, you can center an image using margin auto. This technique is commonly used when you have a block-level element.
.container { text-align: center; } .image { display: block; margin-left: auto; margin-right: auto; }
By setting the left and right margins to auto, the image will be centered within the container.
Centering Using CSS Pseudo-elements
You can also center an image using CSS pseudo-elements. By applying ::before
and ::after
pseudo-elements to the container, you can create invisible elements that help center the image.
.container::before { content: ''; display: inline-block; height: 100%; vertical-align: middle; } .container::after { content: ''; display: inline-block; height: 100%; vertical-align: middle; } .image { display: inline-block; vertical-align: middle; }
By vertically aligning the pseudo-elements and the image using vertical-align: middle
, the image will be centered both horizontally and vertically within the container.
These advanced techniques give you more flexibility and control over how your images are centered in CSS. Experiment with these methods to achieve the desired centering effect for your website.