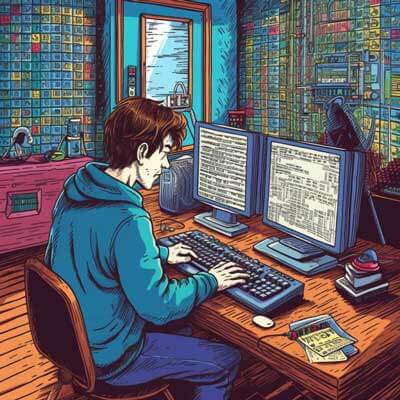
To get the current year in JavaScript, you can use the built-in Date
object. Below are two possible approaches to retrieve the current year:
1. Using the getFullYear
method
The Date
object in JavaScript has a method called getFullYear
that returns the current year as a four-digit number. Here’s an example:
const currentYear = new Date().getFullYear(); console.log(currentYear);
This will output the current year in the console.
Related Article: How to Format JavaScript Dates as YYYY-MM-DD
2. Using the getUTCFullYear
method
Similar to the getFullYear
method, the Date
object also provides a getUTCFullYear
method that returns the current year in UTC. Here’s an example:
const currentYear = new Date().getUTCFullYear(); console.log(currentYear);
This will output the current year in UTC in the console.
Best Practices and Suggestions
Here are some best practices and suggestions when working with the current year in JavaScript:
– Remember that the Date
object represents the current date and time. If you only need the current year, you can create a new instance of the Date
object without passing any arguments.
– Be aware that JavaScript’s Date
object returns the current year based on the user’s system clock. If the user’s system clock is incorrect, the returned year will also be incorrect.
– If you need to display the current year on a webpage, you can directly set the value using JavaScript. For example, to display the current year in an HTML element with the id “year”:
const currentYear = new Date().getFullYear(); document.getElementById("year").textContent = currentYear; <p>© <span id="year"></span> My Website. All rights reserved.</p>
This will dynamically update the year whenever the page is loaded.
– To manipulate or perform calculations with the current year, it’s recommended to store it in a variable. This avoids calling the Date
object multiple times and improves code readability.
const currentYear = new Date().getFullYear(); // Example: Checking if a year is a leap year function isLeapYear(year) { // implementation } if (isLeapYear(currentYear)) { console.log("The current year is a leap year!"); } else { console.log("The current year is not a leap year."); }
– If you’re working with date and time in general, consider using a library like Moment.js. Moment.js provides a comprehensive set of utilities for parsing, validating, manipulating, and formatting dates and times.
const currentYear = moment().year(); console.log(currentYear);
This example assumes you have imported the Moment.js library into your project.
Related Article: Tutorial: JavaScript in AJAX Technology