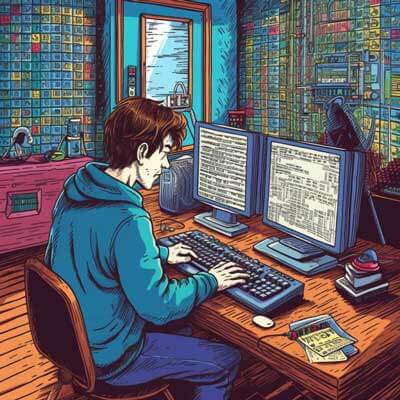
To center an absolutely positioned element in a div, you can use the following approaches:
Approach 1: Using Transform and Translate
One way to center an absolutely positioned element is by using the transform
property with the translate()
function. Here’s how you can do it:
.parent { position: relative; } .child { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); }
In this approach, we set the parent container to have a position
of relative
and the child element to have a position
of absolute
. Then, we use the top
and left
properties to position the child element at 50% from the top and left edges of the parent container.
The key to centering the element is the transform: translate(-50%, -50%);
property. This property moves the element 50% of its own width to the left and 50% of its own height up, effectively centering it horizontally and vertically within the parent container.
Related Article: How to Vertically Align Text in a Div with CSS
Approach 2: Using Flexbox
Another approach to center an absolutely positioned element is by using flexbox. Here’s an example:
.parent { display: flex; align-items: center; justify-content: center; height: 100vh; } .child { position: absolute; }
In this approach, we make the parent container a flex container by setting display: flex
. Then, we use the align-items
and justify-content
properties to center the child element both vertically and horizontally within the parent container.
This approach is particularly useful when you want to center an absolutely positioned element within the viewport. Setting height: 100vh
on the parent container ensures that it takes up the full height of the viewport.
Best Practices
Here are some best practices to consider when centering an absolutely positioned element in a div:
1. Use relative positioning: It’s a good practice to set the parent container to have a position
of relative
when centering an absolutely positioned element. This helps establish a containing block for the child element and ensures that it positions itself relative to the parent container.
2. Avoid using fixed dimensions: When centering elements, it’s generally better to use relative units like percentages or viewport units (vh
, vw
) instead of fixed pixel values. This ensures that the centering remains responsive and adapts to different screen sizes.
3. Consider browser compatibility: The approaches mentioned above, using transform
and flexbox, are well-supported by modern browsers. However, for older browsers, you may need to use alternative techniques like using negative margins or JavaScript-based solutions.
Alternative Ideas
While the approaches mentioned above are commonly used for centering absolutely positioned elements, there are alternative ideas you can explore:
1. Using grid: If you’re working with a grid layout, you can leverage CSS grid properties like justify-items
and align-items
to center an absolutely positioned element.
2. Using auto margins: In some cases, you can achieve centering by using auto margins. This technique works when the parent container has a fixed width and the child element has a specified width.
Here’s an example:
.parent { width: 300px; } .child { margin: 0 auto; }
In this example, the child element will be horizontally centered within the parent container by setting margin: 0 auto;
. The auto
value for the left and right margins distributes the available space equally, resulting in centering the child element.
Overall, the choice of technique depends on your specific requirements and the layout you’re working with. Flexbox and the transform
property are widely supported and offer a lot of flexibility for centering absolutely positioned elements.
Related Article: How to Horizontally Center a Div Element in CSS