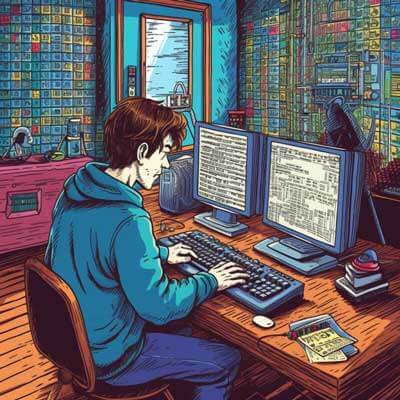
Table of Contents
Media queries are a useful tool in CSS that allow you to apply different styles to elements based on the characteristics of the device or screen size. This enables you to create responsive designs that adapt seamlessly to different devices, such as desktops, tablets, and mobile phones. In this answer, I will explain how to use media queries to target specific devices and provide examples of best practices.
Step 1: Understanding Media Queries
Media queries are CSS rules that apply different styles based on the characteristics of the device or viewport. You can specify conditions such as the width, height, aspect ratio, or orientation of the device. Media queries use the @media
rule followed by the condition in parentheses. Here's an example of a media query that targets devices with a maximum width of 600 pixels:
@media (max-width: 600px) { /* CSS rules for devices with a maximum width of 600px */ }
Related Article: How To Set the CSS Background Opacity Property
Step 2: Targeting Desktop Devices
To target desktop devices, you can use media queries that specify a minimum width. Here's an example of a media query that targets devices with a minimum width of 1024 pixels:
@media (min-width: 1024px) { /* CSS rules for devices with a minimum width of 1024px (desktop devices) */ }
Within this media query, you can define styles specific to desktop devices. For example, you can increase the font size, adjust the layout, or add additional elements. It's important to consider the user experience on larger screens and make use of the available space effectively.
Step 3: Targeting Tablet Devices
To target tablet devices, you can use media queries that specify a width range that is typically associated with tablets. The exact width range may vary depending on your design and target audience. Here's an example of a media query that targets devices with a width between 601 pixels and 1023 pixels:
@media (min-width: 601px) and (max-width: 1023px) { /* CSS rules for devices with a width between 601px and 1023px (tablet devices) */ }
Within this media query, you can define styles specific to tablet devices. Consider the touch interface and adjust the layout and interactions accordingly. It's also important to test your design on different tablet devices to ensure optimal user experience.
Step 4: Targeting Mobile Devices
To target mobile devices, you can use media queries that specify a maximum width. Here's an example of a media query that targets devices with a maximum width of 600 pixels:
@media (max-width: 600px) { /* CSS rules for devices with a maximum width of 600px (mobile devices) */ }
Within this media query, you can define styles specific to mobile devices. Consider the smaller screen size and optimize the layout, font size, and interactions for mobile users. It's also important to test your design on different mobile devices and orientations to ensure a consistent and user-friendly experience.
Related Article: How to Implement an Onclick Effect in CSS
Step 5: Best Practices
Here are some best practices to consider when using media queries for desktop, tablet, and mobile:
- Use a mobile-first approach: Start with the styles for mobile devices and then use media queries to add styles for larger screens. This ensures a graceful degradation of styles and a better user experience on smaller devices.
- Test on real devices: Use actual devices or device emulators to test your responsive design. This helps you identify any issues and ensure that your styles are applied correctly across different devices.
- Use relative units: Instead of using fixed pixel values, consider using relative units such as percentages or rem
to create a flexible and scalable design. This allows your design to adapt to different screen sizes more effectively.
- Consider touch interactions: Mobile and tablet devices often rely on touch interactions. Make sure your design accommodates touch gestures and provides enough spacing between interactive elements to prevent accidental taps.
- Optimize performance: In responsive designs, it's common to hide or show certain elements based on the screen size. However, be mindful of performance implications. Avoid loading unnecessary resources or executing heavy JavaScript on devices where they are not needed.
Step 6: Example Code
Here's an example of how you can use media queries to create a responsive layout that adapts to different devices:
.container { width: 100%; max-width: 800px; margin: 0 auto; } @media (min-width: 601px) { .container { padding: 20px; } } @media (min-width: 1024px) { .container { border: 1px solid #ccc; border-radius: 5px; } } <div class="container"> <h1>Responsive Layout Example</h1> <p>This is a responsive layout that adapts to different devices.</p> </div>
In this example, the .container
element has different styles based on the screen size. On mobile devices, it has a full-width layout. On tablet devices, it adds some padding. On desktop devices, it adds a border and border-radius.
Step 7: Alternative Ideas
While media queries are a popular approach for creating responsive designs, there are alternative approaches that you can consider:
- CSS frameworks: Many CSS frameworks, such as Bootstrap and Foundation, provide responsive grid systems and pre-defined classes that make it easier to create responsive designs without writing custom media queries.
- CSS-in-JS: If you are using a CSS-in-JS solution, such as styled-components or Emotion, you can use JavaScript to conditionally apply styles based on the device characteristics. This allows for more dynamic and flexible styling options.
- Server-side detection: In some cases, it may be more appropriate to use server-side detection to serve different stylesheets or markup based on the device accessing the website. This approach can be useful when you have significant differences in content or layout between different devices.