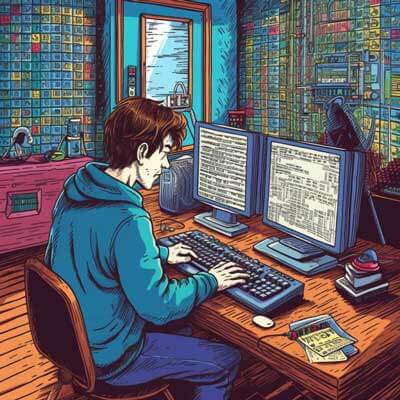
Validating email addresses is a common task in software development. Regular expressions, or regex, provide a powerful tool for pattern matching and can be used to validate email addresses. In this guide, we will discuss how to validate an email address using regex and provide some best practices to consider.
Why Validate Email Addresses?
Validating email addresses is important because it ensures that the email address provided by a user or entered into a system is in a valid format. This helps to prevent errors and reduce the risk of issues related to email functionality, such as failed deliveries or security vulnerabilities.
There are several reasons why you might want to validate email addresses:
1. User Registration: When users sign up for an account on a website or application, validating their email address ensures that they have provided a valid email address that can be used for communication or account verification.
2. Form Submissions: If you have a form that collects email addresses, validating them can help ensure that the data submitted is in the correct format before processing it further.
3. Data Quality: Validating email addresses can help maintain the quality of your data by filtering out invalid or incorrectly formatted email addresses.
Related Article: How to Ignore Case Sensitivity with Regex (Case Insensitive)
Validating Email Addresses with Regex
To validate email addresses using regex, we can define a pattern that matches the structure of a valid email address. Here is a simple regex pattern that can be used for basic email address validation:
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
Let’s break down this regex pattern:
– ^
: Matches the start of the string.
– [a-zA-Z0-9._%+-]+
: Matches one or more alphanumeric characters, dots, underscores, percent signs, plus signs, or hyphens.
– @
: Matches the “@” symbol.
– [a-zA-Z0-9.-]+
: Matches one or more alphanumeric characters, dots, or hyphens.
– \.
: Matches a dot (escape character is used because a dot is a special character in regex).
– [a-zA-Z]{2,}
: Matches two or more alphabetic characters.
– $
: Matches the end of the string.
This pattern ensures that an email address consists of at least one character before the “@” symbol, followed by a domain name with at least two characters.
Here is an example of how you can use this regex pattern in JavaScript to validate an email address:
function validateEmail(email) { const regex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/; return regex.test(email); } const email = "test@example.com"; console.log(validateEmail(email)); // Output: true
In this example, the validateEmail
function takes an email address as input and uses the test
method of the regex object to check if the email address matches the pattern. The function returns true
if the email address is valid and false
otherwise.
Best Practices for Email Address Validation
While the above regex pattern provides a basic validation for email addresses, it’s important to note that it may not catch all edge cases or account for all valid email address formats. Email address validation can be a complex task due to the variety of valid formats and the potential for internationalization.
Here are some best practices to consider when validating email addresses:
1. Use a Well-Tested Regex Pattern: While the above regex pattern is a good starting point, there are more comprehensive patterns available that can handle a wider range of valid email address formats. Consider using a well-tested and widely-used regex pattern that has been proven to be effective.
2. Consider Internationalization: Email addresses can contain non-ASCII characters, such as accented letters or characters from non-Latin scripts. To account for international email addresses, consider using regex patterns that support Unicode characters.
3. Allow for Subdomains: Some email addresses may include subdomains, such as name@subdomain.example.com
. Ensure that your regex pattern allows for valid subdomain structures.
4. Consider Length Limitations: Email addresses have a maximum length limit defined by the email service provider. Be aware of the maximum length and validate that the email address does not exceed it.
5. Test Edge Cases: Test your email address validation with a wide range of test cases, including valid and invalid email addresses, to ensure that your regex pattern covers all scenarios.
Alternative Approaches
While regex is a commonly used approach for email address validation, there are alternative methods that you can consider:
1. Use a Library or Framework: Many programming languages have libraries or frameworks that provide built-in email address validation functionality. These libraries often have more comprehensive validation rules and handle edge cases that may not be covered by a simple regex pattern. Consider using these libraries to simplify your validation code.
2. Send Verification Email: Instead of relying solely on regex validation, you can also send a verification email to the provided address with a unique link or verification code. This approach ensures that the email address is valid and belongs to the user who provided it.
Related Article: How To Match Standard 10 Digit Phone Numbers With Regex