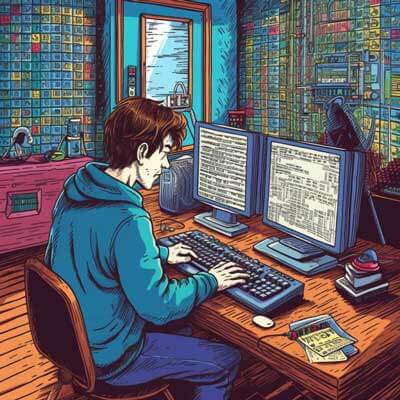
- How does PostgreSQL handle executing 100 queries at once?
- What is the impact of executing 100 queries concurrently in PostgreSQL?
- How can I optimize the execution of 100 queries in PostgreSQL?
- Is it recommended to execute 100 queries at once in PostgreSQL?
- What are the best practices for executing multiple queries in node.js with PostgreSQL?
- Additional Resources
How does PostgreSQL handle executing 100 queries at once?
PostgreSQL is a useful and feature-rich open-source relational database management system (RDBMS) that is capable of handling multiple queries simultaneously. When executing 100 queries at once in PostgreSQL, the database engine employs various mechanisms to efficiently process and manage the workload.
One key aspect of PostgreSQL’s query execution is its use of a multi-process architecture. PostgreSQL utilizes multiple worker processes, each capable of handling a separate query, to execute queries concurrently. These worker processes are managed by the database engine and are responsible for executing the SQL statements and returning the results.
To handle the execution of 100 queries at once, PostgreSQL dynamically allocates resources such as CPU and memory to the worker processes. The database engine intelligently schedules and assigns the queries to the available worker processes, ensuring optimal utilization of system resources.
Additionally, PostgreSQL employs sophisticated query optimization techniques to improve the performance of executing multiple queries concurrently. The query optimizer analyzes the queries and generates an optimized execution plan, taking into account factors such as indexes, statistics, and table relationships. This optimization process helps PostgreSQL to efficiently execute the queries, minimizing the overall execution time.
Let’s consider an example where we want to execute 100 queries simultaneously in PostgreSQL. We can achieve this by utilizing the pg
package, which is a popular Node.js module for interacting with PostgreSQL databases. Here’s an example code snippet that demonstrates the execution of 100 queries concurrently using the pg
package:
const { Pool } = require('pg'); // Create a pool of database connections const pool = new Pool({ user: 'your_username', host: 'your_host', database: 'your_database', password: 'your_password', port: 5432, }); // Asynchronously execute 100 queries async function executeQueries() { const queries = []; for (let i = 1; i <= 100; i++) { queries.push(pool.query(`SELECT * FROM users WHERE id = ${i}`)); } const results = await Promise.all(queries); console.log(results); } executeQueries();
In this example, we create a connection pool using the pg
package, which allows us to establish multiple concurrent connections to the PostgreSQL database. We then use a loop to generate 100 queries, each fetching a user record by their ID. These queries are executed concurrently using the pool.query()
method and wrapped in a Promise.all()
to await their completion. Finally, the results of all the queries are logged to the console.
Related Article: How To Fix 'Maximum Call Stack Size Exceeded' Error
What is the impact of executing 100 queries concurrently in PostgreSQL?
Executing 100 queries concurrently in PostgreSQL can have both positive and negative impacts on the database performance and overall system resources. It is important to consider these impacts when designing and executing such a workload.
Positive impacts:
– Improved throughput: By executing multiple queries concurrently, PostgreSQL can process a higher number of queries within a given time frame. This can result in improved overall system throughput, allowing more work to be completed in a shorter amount of time.
– Efficient resource utilization: PostgreSQL’s multi-process architecture allows for efficient utilization of system resources such as CPU and memory. By distributing the workload across multiple worker processes, the database engine can make better use of available resources, potentially leading to improved performance.
– Reduced latency: Concurrent execution of queries can help reduce the overall latency of query processing. As the queries are executed simultaneously, the total time taken to complete the workload can be significantly reduced compared to executing the queries sequentially.
Negative impacts:
– Increased resource consumption: Executing 100 queries concurrently can put a higher load on system resources such as CPU, memory, and disk I/O. This increased resource consumption can lead to higher system overhead and may impact the performance of other concurrent processes running on the same system.
– Contentions and locks: Concurrent execution of queries can result in contentions and locks on database objects such as tables and rows. This can occur when multiple queries are trying to access or modify the same data simultaneously, leading to potential conflicts and performance degradation.
– Decreased individual query performance: When executing a large number of queries concurrently, the individual query performance may be impacted. This can occur due to resource contention or the need for the database engine to allocate resources across multiple queries, resulting in longer query execution times.
To mitigate the potential negative impacts of executing 100 queries concurrently in PostgreSQL, it is important to optimize the database schema, query design, and system resources. This includes techniques such as proper indexing, query tuning, and resource allocation. Additionally, thorough testing and benchmarking should be performed to ensure that the system can handle the desired workload without significant degradation in performance.
How can I optimize the execution of 100 queries in PostgreSQL?
To optimize the execution of 100 queries in PostgreSQL, several strategies can be employed. These strategies focus on improving query performance, reducing resource consumption, and maximizing the efficiency of query execution.
1. Use parameterized queries: Parameterized queries help prevent SQL injection attacks and improve query performance by allowing PostgreSQL to reuse query plans. Instead of concatenating values directly into the query string, use placeholders and pass the values as separate parameters.
Example:
const query = 'SELECT * FROM users WHERE id = $1'; const values = [userId]; pool.query(query, values);
2. Optimize indexes: Properly indexing the tables can significantly improve query performance. Analyze the query patterns and identify the columns frequently used in the WHERE, JOIN, and ORDER BY clauses. Create indexes on these columns to speed up the query execution.
Example:
CREATE INDEX idx_users_email ON users (email);
3. Use appropriate data types: Choosing the right data types for columns can improve query performance and reduce storage requirements. Use the most efficient data types that accurately represent the data being stored.
Example:
CREATE TABLE users ( id SERIAL PRIMARY KEY, name TEXT, email TEXT UNIQUE );
4. Optimize query logic: Review the query logic to ensure that it is efficient and optimized. Avoid unnecessary subqueries or complex joins that can slow down query execution. Simplify the query logic wherever possible.
Example:
-- Avoid unnecessary subquery SELECT * FROM users WHERE id IN (SELECT user_id FROM orders); -- Equivalent optimized query using JOIN SELECT u.* FROM users u JOIN orders o ON u.id = o.user_id;
5. Tune PostgreSQL configuration: Adjusting the PostgreSQL configuration parameters can have a significant impact on query performance. Tune parameters such as shared_buffers
, work_mem
, and max_connections
based on the available system resources and workload requirements.
Example:
# PostgreSQL configuration file (postgresql.conf) shared_buffers = 4GB work_mem = 64MB max_connections = 200
6. Monitor database performance: Regularly monitor the performance of the database using tools like pg_stat_statements, pg_stat_activity, and EXPLAIN. Identify slow queries and bottlenecks, and make necessary optimizations based on the findings.
Example:
-- Enable pg_stat_statements extension CREATE EXTENSION IF NOT EXISTS pg_stat_statements; -- View top 10 slowest queries SELECT query, total_time, calls FROM pg_stat_statements ORDER BY total_time DESC LIMIT 10;
Is it recommended to execute 100 queries at once in PostgreSQL?
The recommendation to execute 100 queries at once in PostgreSQL depends on several factors, including the nature of the application, the database schema, and the available system resources. While PostgreSQL is capable of handling concurrent query execution, it is important to consider the potential impact on performance, resource utilization, and data consistency.
Executing 100 queries at once can be beneficial in certain scenarios, such as when there is a need to process a large amount of data or when there is a requirement for high throughput. Concurrent execution of queries can help improve overall system performance and reduce the time required to complete a workload.
However, there are also potential drawbacks to executing 100 queries concurrently. The increased resource consumption and contention for system resources can lead to degraded performance and increased latency. Additionally, executing a large number of queries concurrently may introduce the risk of data inconsistencies and conflicts if proper transaction management is not in place.
To determine whether executing 100 queries at once is recommended in your specific scenario, consider the following:
1. Workload characteristics: Evaluate the nature of the workload and the requirements of the application. If the workload involves processing a large amount of data or requires high throughput, executing 100 queries concurrently may be beneficial. On the other hand, if the workload is small or the requirements can be met with sequential execution, executing the queries concurrently may not be necessary.
2. System resources: Consider the available system resources, including CPU, memory, and disk I/O. Executing 100 queries concurrently can put a significant load on these resources, potentially impacting the performance of other processes running on the same system. Ensure that the system has sufficient resources to handle the concurrent workload without degradation in performance.
3. Transaction management: If the queries involve data modifications or require transactional consistency, careful consideration should be given to transaction management. PostgreSQL provides transaction isolation levels that determine the level of concurrency and data consistency. Ensure that proper transaction boundaries are defined and that data integrity is maintained during concurrent execution.
4. Benchmarking and testing: Before executing 100 queries concurrently in a production environment, perform thorough testing and benchmarking. Evaluate the performance impact, resource utilization, and data consistency under various load conditions. Adjust the configuration and optimization techniques as necessary to achieve the desired results.
Related Article: How To Download a File With jQuery
What are the best practices for executing multiple queries in node.js with PostgreSQL?
When executing multiple queries in Node.js with PostgreSQL, it is important to follow best practices to ensure efficient and secure execution. Here are some best practices to consider:
1. Use connection pooling: Establishing a connection to the PostgreSQL database can be an expensive operation. To avoid the overhead of establishing a new connection for each query, use a connection pooling library like pg-pool
or pgBouncer
. Connection pooling allows for reusing connections and improves the performance of query execution.
Example using pg-pool
:
const { Pool } = require('pg'); // Create a pool of database connections const pool = new Pool({ user: 'your_username', host: 'your_host', database: 'your_database', password: 'your_password', port: 5432, max: 10, // Maximum number of connections in the pool }); // Execute a query using the pool pool.query('SELECT * FROM users', (err, res) => { if (err) { console.error(err); } else { console.log(res.rows); } });
2. Use parameterized queries: Avoid constructing SQL queries by concatenating user input directly into the query string. Instead, use parameterized queries to prevent SQL injection attacks and improve query performance. Parameterized queries use placeholders for dynamic values and separate values from the query logic.
Example using pg
package:
const { Pool } = require('pg'); const pool = new Pool({ user: 'your_username', host: 'your_host', database: 'your_database', password: 'your_password', port: 5432, }); const name = 'John Doe'; // Use a parameterized query const query = { text: 'SELECT * FROM users WHERE name = $1', values: [name], }; pool.query(query, (err, res) => { if (err) { console.error(err); } else { console.log(res.rows); } });
3. Optimize query execution: Follow the optimization techniques mentioned earlier in this article to improve query performance. Proper indexing, data type selection, and query logic optimization can significantly enhance the execution speed of multiple queries.
4. Use asynchronous execution: Node.js is well-suited for asynchronous programming. Leverage the asynchronous nature of Node.js to execute multiple queries concurrently and improve the overall performance of your application. Use Promises, async/await, or callback functions to handle the asynchronous execution of queries.
Example using Promises:
const { Pool } = require('pg'); const pool = new Pool({ user: 'your_username', host: 'your_host', database: 'your_database', password: 'your_password', port: 5432, }); async function executeQueries() { const query1 = 'SELECT * FROM users'; const query2 = 'SELECT * FROM products'; const result1 = await pool.query(query1); const result2 = await pool.query(query2); console.log(result1.rows); console.log(result2.rows); } executeQueries();
5. Implement error handling: Proper error handling is crucial when executing multiple queries. Handle errors gracefully and ensure that the application can recover from any unexpected issues. Use try/catch blocks or error handling middleware to catch and handle errors in a consistent manner.
Example using try/catch:
const { Pool } = require('pg'); const pool = new Pool({ user: 'your_username', host: 'your_host', database: 'your_database', password: 'your_password', port: 5432, }); async function executeQuery() { try { const query = 'SELECT * FROM users'; const result = await pool.query(query); console.log(result.rows); } catch (err) { console.error(err); } } executeQuery();