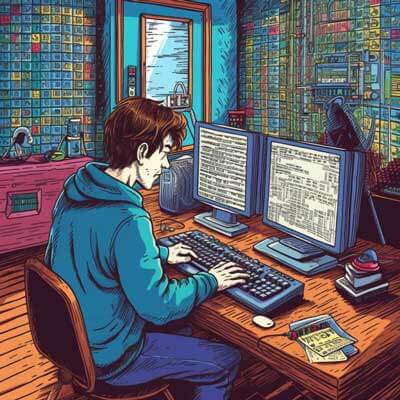
- What is Pair Programming?
- Benefits of Pair Programming
- Improving Collaboration
- Shared Understanding
- Instant Feedback
- Reduced Bugs and Improved Code Quality
- Enhanced Learning and Skill Development
- Enhancing Code Quality
- Practical Tips for Pair Programming
- Use Cases of Pair Programming
- Real World Examples
- Common Challenges and How to Overcome Them
- Communication and Collaboration
- Skill and Experience Disparities
- Time Management
- Code Ownership and Autonomy
- Advanced Techniques for Pair Programming
- Measuring the Success of Pair Programming
- Pair Programming in Agile Development
- Benefits of Pair Programming
- Implementing Pair Programming in Agile
- Pair Programming in Remote Teams
- The Benefits of Pair Programming in Remote Teams
- Tips for Successful Pair Programming in Remote Teams
- Pair Programming Best Practices
- Pair Programming Tools and Resources
- Integrated Development Environments (IDEs)
- Version Control Systems (VCS)
- Communication and Collaboration Tools
- Online Coding Platforms
What is Pair Programming?
Pair programming is an agile software development technique where two programmers work together at one workstation. In this collaborative approach, one person takes on the role of the driver, responsible for writing the code, while the other person acts as the observer or navigator, providing feedback, suggestions, and actively thinking about the big picture.
The idea behind pair programming is to leverage the strengths and perspectives of two individuals to produce higher quality code and enhance collaboration. By continuously reviewing each other’s work, pair programming helps catch errors and improves the overall code quality.
Pair programming can be used in various situations, such as during the initial development phase, when solving complex problems, or even during code reviews. It is not limited to a specific programming language or technology stack, making it applicable to a wide range of development teams.
Here’s an example of how pair programming can be beneficial. Consider a scenario where two developers, Alice and Bob, are working on a feature that requires integrating a payment gateway into an e-commerce application. Alice, the driver, starts writing the code to handle the payment gateway integration, while Bob, the navigator, actively reviews the code and suggests improvements.
// payment.js function handlePayment(paymentData) { // Code to handle payment gateway integration // ... }
As they work together, they can discuss potential edge cases, explore different implementation approaches, and catch any potential bugs or security vulnerabilities. This collaborative effort helps ensure that the code is well-designed, maintainable, and adheres to best practices.
Pair programming also has an important social aspect. It promotes knowledge sharing, reduces knowledge silos, and helps team members learn from each other. By actively discussing code and design decisions, pair programming helps spread domain knowledge across the team, reducing the risk of critical knowledge being lost if a team member leaves.
Overall, pair programming offers several benefits, including improved code quality, reduced bugs, enhanced collaboration, and increased team cohesion. It allows for better knowledge transfer and helps developers grow their skills by learning from one another. While it may take some time to adjust to this collaborative approach, the long-term benefits make it a valuable technique for any development team.
To learn more about the benefits of pair programming, check out this article by the Agile Alliance.
Related Article: 7 Shared Traits of Ineffective Engineering Teams
Benefits of Pair Programming
Pair programming is a software development technique where two programmers work together at one workstation. One person, known as the driver, writes the code while the other person, known as the navigator, reviews each line of code as it is typed. This collaborative approach offers numerous benefits that can significantly improve the quality of code and enhance collaboration among developers.
1. Improved Code Quality
One of the primary benefits of pair programming is the improved code quality it brings. With two sets of eyes constantly reviewing the code, errors and bugs can be caught and fixed more quickly. The navigator can spot logical flaws, syntax errors, and potential bugs in real-time, ensuring that problems are identified before they make their way into production. Additionally, pair programming promotes better code design and architecture, as both developers can contribute their ideas, resulting in better solutions.
Here’s an example of two developers working together to write a function in JavaScript:
// Function to calculate the factorial of a number function calculateFactorial(num) { let factorial = 1; for (let i = 1; i <= num; i++) { factorial *= i; } return factorial; }
2. Knowledge Sharing and Learning
Pair programming is an excellent way for developers to learn from each other and share knowledge. Junior developers can benefit from the expertise of more experienced programmers, who can guide and mentor them during the coding process. This collaborative environment allows for the transfer of skills, best practices, and problem-solving techniques, fostering continuous learning and growth within the team.
3. Increased Collaboration and Communication
Pair programming encourages constant communication between developers, leading to improved collaboration. By working together, developers can discuss ideas, brainstorm solutions, and make decisions collectively. This open line of communication helps in identifying potential issues early on and finding optimal solutions. Pair programming also fosters a positive team dynamic, as developers learn to trust and rely on each other, leading to a more cohesive and productive team.
4. Reduced Development Time
Though it may seem counterintuitive, pair programming can actually reduce development time in the long run. While it may take longer to write code initially, the code is generally of higher quality and requires less debugging and maintenance later on. Additionally, pair programming can help identify bottlenecks or areas where improvements can be made, resulting in more efficient code and faster development cycles.
5. Enhanced Problem Solving
Pair programming promotes effective problem-solving by leveraging the knowledge and perspectives of two developers. When faced with challenging tasks or complex problems, having two minds working together can lead to more innovative and creative solutions. Different approaches and ideas can be discussed and evaluated in real-time, allowing for more robust problem-solving strategies.
Pair programming offers several benefits that can greatly enhance collaboration and improve code quality. By leveraging the strengths of two developers working together, teams can build better software, share knowledge, and foster a more productive and efficient work environment.
Improving Collaboration
Collaboration is at the heart of pair programming. By working together, developers can share knowledge, exchange ideas, and find solutions more efficiently. This section explores how pair programming can enhance collaboration and improve code quality.
Shared Understanding
When two developers work together, they bring their unique perspectives and experiences to the table. This diversity of thought can lead to a more thorough examination of the problem at hand and a deeper understanding of the codebase.
Pair programming promotes shared understanding by encouraging constant communication and discussion. Developers can bounce ideas off each other, challenge assumptions, and collectively arrive at better solutions. This collaborative approach minimizes knowledge silos and ensures that multiple team members are familiar with different areas of the codebase.
In practical terms, this shared understanding translates into fewer misunderstandings and less time wasted on miscommunication. Pair programming allows for immediate clarification and correction, preventing issues from snowballing into larger problems.
Related Article: Top 6 AI Tools for Creating Multimedia in 2024
Instant Feedback
One of the immediate benefits of pair programming is the availability of instant feedback. Instead of waiting for code reviews or automated tests, developers can provide real-time feedback to each other.
For example, consider a scenario where a developer is writing a function that calculates the factorial of a number in Python:
def factorial(n): if n == 0: return 1 else: return n * factorial(n - 1)
While one developer writes the code, the other can review it simultaneously. They can catch syntax errors, suggest improvements, or discuss potential performance optimizations. This continuous feedback loop leads to higher-quality code and helps identify issues early in the development process.
Reduced Bugs and Improved Code Quality
Pair programming is known to result in fewer bugs and improved code quality. Two sets of eyes are better than one when it comes to catching errors and identifying potential issues.
By working together, developers can catch and fix bugs in real-time. This leads to faster bug resolution and reduces the likelihood of introducing new bugs later. Additionally, the collaborative nature of pair programming encourages the use of best practices and promotes code cleanliness.
For instance, in a web development scenario, consider the following HTML code snippet:
<div class="container"> <h1>Welcome to Pair Programming</h1> <p>Learn how to collaborate effectively and write better code.</p> </div>
While one developer focuses on the structure and semantics, the other can review the code for accessibility, proper indentation, and adherence to style guidelines. This collaborative effort helps produce higher-quality code that is more maintainable and less prone to errors.
Enhanced Learning and Skill Development
Pair programming is not just about solving problems; it is also a valuable learning opportunity. By working closely with a partner, developers can learn new techniques, gain insights into different programming paradigms, and improve their problem-solving skills.
Pair programming enables knowledge transfer in a natural and interactive manner. Less experienced developers can learn from more experienced ones, and vice versa. This collaborative learning environment fosters growth and helps the entire team improve their skills collectively.
Whether it’s learning a new algorithm, exploring a new programming language, or discovering a more efficient way to write code, pair programming provides an avenue for continuous learning and skill development.
In the next chapter, we will explore the role of pair programming in enhancing code reviews and ensuring code quality.
Enhancing Code Quality
Pair programming is an effective approach to enhance code quality. By having two developers working together on the same task, the likelihood of bugs and errors is greatly reduced. Let’s explore how pair programming can improve code quality.
1. Immediate Code Reviews: With pair programming, code reviews happen in real-time. As code is being written, the partner can provide immediate feedback, catching potential issues before they become problems. This immediate feedback loop helps to identify and fix bugs early in the development process.
Consider the following example in JavaScript:
// app.js function calculateSum(a, b) { return a + b; }
During pair programming, the partner may notice that the function calculateSum
does not handle non-numeric inputs correctly. They can immediately suggest improvements:
// app.js function calculateSum(a, b) { if (typeof a !== 'number' || typeof b !== 'number') { throw new Error('Both inputs must be numbers'); } return a + b; }
2. Increased Code Coverage: Pair programming encourages thorough testing of code. Both developers can collaborate on writing unit tests to cover different scenarios and edge cases. This helps to ensure that the code is robust and handles all possible scenarios.
For instance, in Python, consider the following function to calculate the factorial of a number:
# math_utils.py def factorial(n): if n == 0: return 1 else: return n * factorial(n-1)
While pair programming, the partners can discuss and write test cases to cover different scenarios such as negative numbers, zero, and large inputs:
# test_math_utils.py import math_utils def test_factorial(): assert math_utils.factorial(0) == 1 assert math_utils.factorial(5) == 120 assert math_utils.factorial(-1) == ValueError assert math_utils.factorial(10) == 3628800
3. Continuous Refactoring: Pair programming promotes continuous code refactoring. Both developers can collaborate on improving the design, structure, and readability of the code. By continuously reviewing and refactoring the code, technical debt is reduced, leading to cleaner and more maintainable code.
In this example, let’s consider a Java class for a simple calculator:
// Calculator.java public class Calculator { public int add(int a, int b) { return a + b; } }
During pair programming, the partners may identify the need for additional functionality and suggest refactoring the code to support subtraction:
// Calculator.java public class Calculator { public int add(int a, int b) { return a + b; } public int subtract(int a, int b) { return a - b; } }
Pair programming provides an opportunity for code quality to be continuously improved, resulting in better maintainability and fewer bugs.
Practical Tips for Pair Programming
Pair programming can be a highly effective practice when done right. Here are some practical tips to make the most out of your pair programming sessions:
1. Choose the Right Partner: Pair programming is all about collaboration, so it’s important to choose a partner who is compatible with your working style and has complementary skills. Look for someone who communicates well, is open to feedback, and shares a similar level of expertise.
2. Define Roles: Assigning roles can help streamline the pair programming process. One person can take the role of the “driver,” responsible for writing the code, while the other person takes the role of the “navigator,” responsible for reviewing the code and providing guidance. Switch roles frequently to ensure equal participation.
3. Set Clear Goals: Before starting a pair programming session, discuss and agree upon the specific goals and objectives. This will help keep the session focused and ensure that both programmers are working towards the same outcome.
4. Communicate Effectively: Communication is key in pair programming. Both programmers should actively discuss their ideas, ask questions, and provide constructive feedback. Use a combination of verbal communication, instant messaging, and screen sharing tools to facilitate effective communication.
5. Take Regular Breaks: Pair programming can be mentally exhausting, so it’s important to take regular breaks to recharge. Schedule short breaks every hour or so to get some fresh air, stretch your legs, or simply relax. This will help maintain focus and prevent burnout.
6. Practice Test-Driven Development (TDD): Test-driven development is a valuable practice to adopt during pair programming. Start by writing a failing test and then work together to write code that passes the test. This approach ensures that the code is thoroughly tested and helps uncover any potential issues early on.
// Example of test-driven development in Java public class CalculatorTest { @Test public void testAddition() { Calculator calculator = new Calculator(); int result = calculator.add(2, 2); assertEquals(4, result); } } public class Calculator { public int add(int a, int b) { return a + b; } }
7. Embrace Continuous Integration: Integrate your code frequently to catch any integration issues early. Use a version control system like Git and set up a continuous integration pipeline to automate the process. This will ensure that both programmers are always working with the most up-to-date codebase.
8. Provide Constructive Feedback: Pair programming is a learning opportunity, so be open to giving and receiving feedback. Focus on providing constructive criticism that helps improve the code quality and the partnership. Remember to always be respectful and considerate of each other’s ideas and perspectives.
By following these practical tips, you can maximize the benefits of pair programming, boost collaboration, and enhance the overall quality of your code. Happy coding!
Use Cases of Pair Programming
Pair programming is a versatile technique that can be applied in various scenarios to achieve better collaboration and improve code quality. Here are some common use cases where pair programming can be highly beneficial:
1. Onboarding New Team Members: When a new developer joins a team, pair programming can help them quickly get up to speed with the project and the codebase. By pairing with a more experienced team member, they can learn the best practices, coding conventions, and architecture of the system.
java
// Example of onboarding a new team member in Java
// OldTeamMember.java
public class OldTeamMember {
public void pairWithNewMember(NewTeamMember newMember) {
// Share knowledge and guide the new member
}
}
// NewTeamMember.java
public class NewTeamMember {
public void pairWithExperiencedMember(OldTeamMember experiencedMember) {
// Learn from the experienced member
}
}
2. Implementing Complex Features: Pair programming is particularly useful when working on complex features that require deep understanding and collaboration. By combining the knowledge and skills of two developers, complex problems can be tackled more efficiently and with higher quality.
python
# Example of implementing complex features in Python
def pair_programming(feature1, feature2):
# Collaboratively work on implementing the features
pass
feature1 = ...
feature2 = ...
pair_programming(feature1, feature2)
3. Resolving Critical Issues: When facing critical bugs or issues in production, pair programming can be an effective way to quickly identify and resolve the problem. Two minds working together can bring different perspectives and insights, leading to faster troubleshooting and resolution.
javascript
// Example of resolving critical issues in JavaScript
function pairProgramming(issue) {
// Collaborate to identify and fix the issue
}
const issue = ...
pairProgramming(issue);
4. Knowledge Sharing and Mentoring: Pair programming can be used as a mentoring tool to transfer knowledge and share expertise among team members. By pairing a senior developer with a junior developer, the senior can guide and mentor the junior, helping them learn new concepts and improve their skills.
csharp
// Example of knowledge sharing and mentoring in C#
public class SeniorDeveloper {
public void PairWithJunior(JuniorDeveloper junior) {
// Mentor the junior developer
}
}
public class JuniorDeveloper {
public void PairWithSenior(SeniorDeveloper senior) {
// Learn from the senior developer
}
}
5. Code Reviews: Pair programming can be used as an alternative or complement to traditional code reviews. By reviewing code together, developers can catch potential issues or improvements more effectively and in real-time. This leads to higher code quality and reduces the need for extensive code reviews later in the development process.
typescript
// Example of code reviews using pair programming in TypeScript
function pairReview(code) {
// Collaboratively review the code
}
const code = ...
pairReview(code);
Pair programming can be applied in various other scenarios depending on the needs and dynamics of the development team. Its flexibility and effectiveness in fostering collaboration and enhancing code quality make it a valuable technique in software development.
Real World Examples
Pair programming has been widely adopted by many software development teams, and numerous real-world examples demonstrate its benefits in boosting collaboration and enhancing code quality. Let’s explore a few of these examples.
1. Pivotal Labs:
Pivotal Labs, a renowned software development company, is well-known for its adoption of pair programming. They have found that pair programming significantly improves collaboration and knowledge sharing among team members. By working together, developers can quickly identify problems, brainstorm solutions, and learn from each other’s expertise.
In their book “Extreme Programming Explained,” Kent Beck and Cynthia Andres discuss how pair programming at Pivotal Labs helps in maintaining high code quality. By continuously reviewing code during development, pair programmers can catch bugs and design flaws early on, leading to cleaner and more maintainable code.
2. Google:
Google, one of the tech giants, also recognizes the benefits of pair programming. They encourage their developers to pair up and work together on critical projects. By doing so, Google aims to improve code quality, reduce bugs, and foster a collaborative work environment.
In a research paper published by Google, they found that pair programming at their organization led to higher-quality code, faster bug fixing, and increased knowledge sharing. Pair programmers were able to catch bugs more effectively, resulting in reduced debugging time and improved overall code stability.
3. Agile Development Teams:
Many agile development teams have embraced pair programming as a core practice. The Agile Manifesto emphasizes the importance of individuals and interactions over processes and tools. Pair programming aligns with this principle by promoting face-to-face collaboration and continuous learning.
For example, in a Scrum team, two developers may collaborate closely during a sprint to develop a user story. By working together, they can leverage their strengths and ensure that the code is of high quality. This practice also helps in spreading knowledge across the team, reducing knowledge silos, and enabling smoother project transitions.
Here’s an example of how pair programming can be implemented in a Java project:
// File: PairProgram.java public class PairProgram { public static void main(String[] args) { // Two developers pair up to write code together Developer developer1 = new Developer("Alice"); Developer developer2 = new Developer("Bob"); developer1.writeCode(); developer2.reviewCode(); } } class Developer { private String name; public Developer(String name) { this.name = name; } public void writeCode() { // Code implementation } public void reviewCode() { // Code review implementation } }
In this example, two developers, Alice and Bob, collaborate by pairing up to write and review code together.
Pair programming has proven to be a valuable practice in the software development industry, with various companies and agile teams reaping its benefits. By fostering collaboration, knowledge sharing, and code quality, pair programming can significantly improve the efficiency and effectiveness of software development projects.
Common Challenges and How to Overcome Them
Pair programming can bring numerous benefits to a development team, but like any collaboration method, it also has its challenges. In this chapter, we will discuss some of the common challenges that teams may face when implementing pair programming and provide strategies to overcome them.
Communication and Collaboration
Effective communication and collaboration are crucial for the success of pair programming. However, different communication styles and personalities can sometimes lead to misunderstandings or conflicts. Here are a few strategies to overcome these challenges:
– Establish clear communication guidelines: Define how team members should communicate during pair programming sessions. Encourage active listening, respect for differing opinions, and open dialogue.
– Set expectations for roles and responsibilities: Clearly define each team member’s role in the pair programming session. This will help avoid confusion and ensure that tasks are appropriately divided.
– Foster a positive team environment: Encourage team members to build trust and rapport. Foster an inclusive and supportive culture where everyone feels comfortable sharing their ideas and concerns.
Skill and Experience Disparities
Pair programming can become challenging when there are significant skill or experience disparities between team members. The following strategies can help address this issue:
– Rotate pairs regularly: Rotate team members frequently to ensure knowledge sharing. This will allow less experienced developers to learn from more experienced ones while mitigating the risk of knowledge silos.
– Encourage mentoring and coaching: Pairing experienced developers with less experienced ones can help bridge the skill gap. The experienced developer can guide and mentor the other team member, fostering their growth and development.
Time Management
Pair programming requires two developers to work together, which can impact productivity and time management. Here are a few strategies to overcome time-related challenges:
– Define a schedule: Set clear expectations for when pair programming sessions will occur. This will help team members plan their individual work and allocate time for collaboration.
– Use timeboxing techniques: Timeboxing involves allocating fixed time periods for specific tasks. This technique can help prevent sessions from running too long and ensure that progress is made within the allocated timeframe.
Code Ownership and Autonomy
Pair programming may lead to concerns about code ownership and individual autonomy. To address these challenges, consider the following strategies:
– Foster a shared code ownership mindset: Encourage team members to view the codebase as a collective responsibility. Emphasize the importance of collaboration and the benefits of shared knowledge and expertise.
– Encourage exploratory programming: Allow team members to explore new ideas and solutions individually or in smaller groups. This can help maintain a balance between individual autonomy and collaborative work.
Overall, while pair programming may present some challenges, they can be overcome with effective communication, collaboration, and a supportive team environment. By addressing these challenges head-on, teams can fully leverage the benefits of pair programming and enhance code quality in their projects.
Advanced Techniques for Pair Programming
Pair programming is a powerful technique that can greatly enhance collaboration and code quality. While the basic principles of pair programming are straightforward, there are some advanced techniques that can take your pair programming sessions to the next level. In this chapter, we will explore some of these techniques and how they can benefit your development process.
1. Ping-Pong Pairing:
Ping-pong pairing is a technique where one developer writes a failing test case and then hands off the keyboard to their partner, who implements the code to make the test pass. Once the test is passing, the roles are reversed, and the partner writes the next failing test case. This back-and-forth pattern continues throughout the session.
This technique not only promotes equal participation but also encourages the developers to think from both the test writer’s and test implementer’s perspectives. It helps catch edge cases and ensures that code is thoroughly tested. Here’s an example of how ping-pong pairing might look in a Python test file:
# test_foo.py def test_foo(): assert foo() == 42 def test_bar(): assert bar() == "hello"
2. Mob Programming:
Mob programming takes pair programming to a whole new level by involving the entire team in the process. Instead of just two developers working together, the entire team collaborates on a single task. One person sits at the keyboard, writing code based on the team’s discussions, while the others provide input, suggestions, and feedback.
This technique promotes knowledge sharing, fosters team cohesion, and can lead to faster problem-solving. It also helps identify potential design flaws and encourages everyone to contribute their expertise. Here’s an example of how mob programming might look in a JavaScript file:
// app.js function calculateSum(a, b) { return a + b; } function calculateProduct(a, b) { return a * b; } // ...more code
3. Remote Pair Programming:
With the rise of remote work, pair programming has also adapted to accommodate geographically distributed teams. Remote pair programming allows developers to collaborate effectively, regardless of their physical location. Tools like screen sharing, video conferencing, and collaborative code editors enable real-time collaboration between remote pair programmers.
To ensure smooth remote pair programming sessions, it is crucial to have a stable internet connection and use tools that facilitate seamless communication and code sharing. Here’s an example of how remote pair programming might look in a Ruby file:
# calculator.rb def add(a, b) return a + b end def subtract(a, b) return a - b end # ...more code
In this chapter, we have explored some advanced techniques for pair programming. Ping-pong pairing, mob programming, and remote pair programming all bring unique benefits to the table. Experiment with these techniques to find what works best for your team and enjoy the increased collaboration and enhanced code quality that pair programming can bring.
Measuring the Success of Pair Programming
Pair programming is a collaborative software development technique that involves two programmers working together on the same task. While the benefits of pair programming are well-known, it is important to measure its success to understand its impact on collaboration and code quality. In this chapter, we will explore various ways to measure the success of pair programming.
1. Code Review Metrics
One way to measure the success of pair programming is by analyzing code review metrics. Code reviews are an essential part of the software development process and can provide insights into the quality of the code produced through pair programming.
For example, you can track the number of code defects found during the review process. By comparing the defect density of code written by pairs versus code written individually, you can determine if pair programming leads to fewer defects.
Another metric to consider is the time it takes to complete a code review. If pair programming leads to better code quality, it is expected that the time spent on code reviews will be reduced as there will be fewer issues to address.
2. Productivity Metrics
Measuring the productivity of pair programming is another way to gauge its success. Although it may seem counterintuitive, pair programming has been shown to increase productivity in certain contexts.
One way to measure productivity is by tracking the number of story points or tasks completed by pairs versus individual programmers. By comparing these metrics, you can determine if pair programming leads to a higher throughput of work.
You can also measure the time it takes to complete a task or feature. If pair programming is effective, it should result in faster development cycles as two programmers can work on the problem simultaneously.
3. Collaboration Metrics
Pair programming is primarily a collaboration technique, so it is essential to measure its impact on collaboration within the development team.
One way to measure collaboration is by analyzing the number of code conflicts or merge issues encountered during development. If pair programming leads to better communication and coordination, it should result in fewer conflicts during integration.
Another metric to consider is the level of knowledge sharing within the team. Pair programming encourages knowledge transfer between team members, which can be measured by tracking the number of knowledge sharing sessions or the frequency of code reviews between pairs.
4. Developer Feedback
Finally, one of the most valuable ways to measure the success of pair programming is by gathering feedback from the developers themselves. Conducting surveys or interviews can provide insights into the perceived benefits and challenges of pair programming.
Ask developers about their experience with pair programming and whether they feel it has improved collaboration and code quality. Their feedback can help identify areas for improvement and provide a holistic view of the success of pair programming within the team.
Measuring the success of pair programming is crucial to understand its impact on collaboration and code quality. By analyzing code review metrics, productivity metrics, collaboration metrics, and gathering developer feedback, you can evaluate the effectiveness of pair programming and make informed decisions about its use in your development process.
Pair Programming in Agile Development
Pair programming is a software development technique that involves two programmers working together on the same task, at the same workstation. In the context of Agile development, pair programming is often used as a collaborative practice to boost collaboration and enhance code quality. This chapter explores the benefits of pair programming in Agile development and provides insights into how it can be effectively implemented.
Benefits of Pair Programming
Pair programming offers several benefits that can greatly contribute to the success of Agile development projects. Here are some key advantages:
1. Improved Code Quality: With two developers working together, the chances of catching errors and bugs are significantly higher. By continuously reviewing and discussing each line of code, pair programmers can identify potential issues early on, leading to more robust and reliable code.
2. Knowledge Sharing and Learning: Pair programming provides an excellent opportunity for knowledge transfer between team members. Junior developers can learn from more experienced programmers, while senior developers can gain fresh perspectives and insights from their peers. This knowledge sharing fosters a culture of continuous learning and growth within the team.
3. Reduced Risk and Increased Productivity: By having two sets of eyes on the code, pair programming helps to mitigate risks associated with individual developers making mistakes or overlooking important details. Moreover, the constant collaboration and communication between pair programmers can improve productivity by promoting focused and efficient work.
4. Enhanced Collaboration and Team Bonding: Pair programming promotes effective collaboration between team members, as it requires constant communication and synchronization. By working closely together, developers build a deeper understanding of each other’s strengths, weaknesses, and working styles, fostering a stronger sense of camaraderie and teamwork.
Implementing Pair Programming in Agile
To effectively implement pair programming in Agile development, consider the following best practices:
1. Rotate Pair Partners: Encourage team members to switch pairs regularly. By rotating pair partners, developers get the opportunity to work with different team members and gain diverse perspectives. This practice also helps to avoid dependency on a single pair and ensures knowledge sharing across the entire team.
2. Establish Clear Goals and Roles: Before starting a pairing session, clearly define the goals and roles of each pair programmer. This ensures that both developers have a shared understanding of the task at hand and their respective responsibilities. Clear communication and alignment on objectives will lead to more focused and productive pair programming sessions.
3. Provide the Right Tools and Environment: Pair programming requires a conducive environment and suitable tools that facilitate collaboration. Ensure that developers have access to dual monitors, comfortable workstations, and collaborative tools like screen sharing software or integrated development environments (IDEs) that support pair programming features.
4. Encourage Continuous Feedback: Regularly seek feedback from the pair programmers to identify areas for improvement and address any challenges they may be facing. Encouraging open communication and creating a safe space for constructive feedback will help to enhance the effectiveness of pair programming in Agile development.
# Example of Pair Programming in Python # Pair Programmer 1 def calculate_square(number): return number * number # Pair Programmer 2 def calculate_cube(number): return number * number * number # Pair Programming Session result = calculate_square(5) print("Square:", result) result = calculate_cube(5) print("Cube:", result)
In the above example, two pair programmers work together to implement two functions to calculate the square and cube of a number in Python. By collaborating and discussing their code, they can ensure that the functions are implemented correctly and produce the expected results.
Pair programming is a valuable technique in Agile development that fosters collaboration, enhances code quality, and promotes knowledge sharing. By implementing pair programming best practices, teams can leverage this technique to boost productivity and create high-quality software.
Pair Programming in Remote Teams
Working remotely has become increasingly common in today’s global workforce, with teams distributed across different time zones and geographical locations. While this brings many advantages, it also presents challenges when it comes to collaboration and communication. Pair programming, a practice where two programmers work together on the same code at the same time, can be particularly effective in remote teams. In this chapter, we will explore the benefits and strategies of pair programming in a remote setting.
The Benefits of Pair Programming in Remote Teams
Pair programming offers several advantages for remote teams:
1. Enhanced collaboration: Pair programming promotes real-time collaboration and knowledge sharing. Team members can bounce ideas off each other, discuss different approaches, and solve problems together. This leads to better decision-making and improved code quality.
2. Reduced isolation: Remote work can sometimes feel isolating. Pair programming provides an opportunity for remote team members to connect and build relationships with their colleagues. Regular pairing sessions foster a sense of camaraderie and teamwork.
3. Improved code quality: Two sets of eyes are better than one when it comes to code review. By working together, pair programmers catch more errors, identify potential bugs, and apply best practices. This results in cleaner, more maintainable code.
Tips for Successful Pair Programming in Remote Teams
To make the most of pair programming in a remote setting, consider the following tips:
1. Choose the right tools: Select collaborative tools that facilitate real-time coding and communication. For example, screen sharing applications like Zoom or TeamViewer allow remote team members to view and edit code simultaneously. Messaging platforms like Slack or Microsoft Teams can be used for quick discussions and sharing of resources.
2. Establish clear communication: Communication is key in remote pair programming. Both programmers should actively communicate their thoughts, ideas, and concerns. Use video or audio calls to mimic face-to-face interactions and ensure clarity.
3. Define roles and responsibilities: Assign roles to each programmer, such as a driver (the one writing code) and a navigator (the one reviewing and providing feedback). Rotate these roles regularly to keep both team members engaged and involved.
4. Set clear goals and objectives: Before starting a pairing session, define the goals and objectives. This helps maintain focus and ensures that both programmers are aligned on the desired outcome.
5. Take breaks: Remote work can be mentally taxing, so remember to take regular breaks during pair programming sessions. This allows for reflection and recharging, leading to increased productivity and creativity.
Pair Programming Best Practices
Pair programming can be a powerful technique for improving collaboration and code quality, but it requires following some best practices to be effective. Here are some tips for successful pair programming:
1. Choose the Right Partners: Pair programming works best when individuals with complementary skills and knowledge work together. Consider the expertise and experience of each programmer when forming pairs.
2. Rotate Roles: To ensure both programmers are engaged and learning, it’s important to rotate roles regularly. The two common roles in pair programming are the driver (the one who writes the code) and the navigator (the one who reviews the code and provides guidance). Switching roles helps prevent fatigue and ensures both partners contribute equally.
3. Communicate Effectively: Communication is key in pair programming. Encourage open and continuous communication between partners. Explain your thoughts and ideas clearly, ask questions, and actively listen to your partner’s input. Use tools like video conferencing, instant messaging, or collaborative coding platforms to facilitate communication if working remotely.
4. Take Breaks: Pair programming can be mentally exhausting, so it’s important to take breaks regularly. Short breaks can help maintain focus and prevent burnout. Use breaks to discuss the progress, review the code, or simply relax and refresh.
5. Use Test-Driven Development (TDD): Test-driven development is a natural fit for pair programming. Start by writing a failing test, then work together to make it pass. This iterative process helps ensure code quality and encourages collaboration.
6. Keep Code Reviews: While pair programming involves continuous code review, it’s still valuable to have a separate code review process. Once a task is complete, have another team member review the code to catch any potential issues or provide suggestions for improvement.
Here’s an example of a code snippet in Python demonstrating pair programming best practices:
def calculate_average(numbers): total = sum(numbers) average = total / len(numbers) return average # Pair programming example # Driver writes the code numbers = [1, 2, 3, 4, 5] average = calculate_average(numbers) # Navigator reviews the code print("The average is:", average)
In the example above, the driver writes the code to calculate the average of a list of numbers, while the navigator reviews the code for correctness and clarity. This collaborative approach ensures that both partners contribute to the codebase and enables knowledge sharing.
By following these best practices, you can maximize the benefits of pair programming, including improved collaboration, enhanced code quality, and accelerated learning and development.
Pair Programming Tools and Resources
Pair programming can be made even more effective with the use of various tools and resources that facilitate collaboration and code development. In this chapter, we will explore some popular pair programming tools and resources that can enhance communication, productivity, and code quality.
Integrated Development Environments (IDEs)
IDEs are essential tools for pair programming as they provide a collaborative coding environment with features designed to support teamwork. Some popular IDEs that offer pair programming capabilities include:
– Visual Studio Code (VS Code): A lightweight and extensible IDE that supports real-time collaboration through the “Live Share” extension. With Live Share, developers can share their coding session with others, allowing them to collaboratively edit, debug, and test code in real-time.
Here’s an example of how Live Share can be used in VS Code:
// File: index.ts function add(a: number, b: number): number { return a + b; } // Collaborator's cursor console.log(add(3, 4)); // Output: 7
– IntelliJ IDEA: A powerful Java IDE that offers a feature called “Code With Me,” which enables remote pair programming. Code With Me allows developers to share their IDE with others and collaborate on code editing, debugging, and navigation.
Version Control Systems (VCS)
Version control systems are critical for managing code changes and facilitating collaboration between developers. When pair programming, using a VCS allows both developers to work on the same codebase simultaneously and track changes. Some popular VCS tools include:
– Git: A distributed version control system widely used in the software development industry. Git allows developers to create branches, merge changes, and track code history. It also provides features like pull requests and code reviews, which can further enhance collaboration.
Here’s an example of how two developers can collaborate using Git:
# Developer 1 $ git clone <repository_url> $ git branch feature/awesome-feature $ git checkout feature/awesome-feature # Developer 2 $ git clone <repository_url> $ git branch feature/awesome-feature $ git checkout feature/awesome-feature # Both developers can now work on the same branch simultaneously
Communication and Collaboration Tools
To facilitate effective communication during pair programming sessions, it is essential to have reliable communication and collaboration tools. Some popular tools that can enhance communication include:
– Slack: A team communication platform that allows developers to chat, share files, and collaborate seamlessly. Slack provides features like channels, direct messaging, and integrations with other tools, making it ideal for real-time communication during pair programming.
– Zoom: A video conferencing tool that enables face-to-face communication between remote developers. Zoom offers features like screen sharing, remote control, and breakout rooms, which can enhance collaboration and pair programming experience.
Online Coding Platforms
Online coding platforms can be used for pair programming sessions, especially when working remotely with a distributed team. These platforms allow developers to write and test code collaboratively in a shared coding environment. Some popular online coding platforms include:
– CodePen: An online code editor that allows developers to write HTML, CSS, and JavaScript code in a collaborative environment. CodePen provides real-time synchronization, making it ideal for pair programming tasks that involve front-end web development.
– Repl.it: A web-based coding environment that supports multiple programming languages. Repl.it offers features like live collaboration, code sharing, and built-in development servers, enabling developers to work together on code in real-time.
Using the right pair programming tools and resources can significantly boost collaboration and enhance code quality. Integrated development environments, version control systems, communication tools, and online coding platforms provide the necessary infrastructure for effective pair programming. By leveraging these tools, developers can work together seamlessly, share knowledge, and produce high-quality code more efficiently.