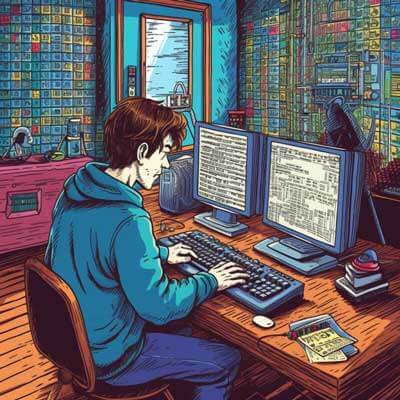
Table of Contents
Understanding Git Branches
Git branches are a fundamental concept in version control systems that allow you to work on different versions of your code simultaneously. Understanding how branches work in Git is essential for managing and organizing your codebase effectively.
Related Article: How To Fix Gitignore Not Working
What is a Git Branch?
In Git, a branch is a lightweight movable pointer to a commit. It represents an independent line of development within your repository. Each branch has a unique name and points to a specific commit. By default, when you create a new repository, a branch called "master" is created.
Why Use Git Branches?
Using branches in Git brings several benefits to your development workflow. Here are a few reasons why branches are essential:
1. Isolate Development: Branches allow you to work on new features or bug fixes without affecting the main codebase. This isolation ensures that your experimental changes won't impact the stability of the main branch until they are ready.
2. Collaboration: Branches enable multiple developers to work on different parts of a project simultaneously. Each developer can create their own branch, work independently, and merge their changes back into the main branch when they are ready.
3. Versioning: Branches provide a way to maintain different versions of your codebase. For example, you can have a branch for the current stable release, another for ongoing development, and another for experimental features.
Common Git Branch Operations
Now that we understand the importance of branches in Git, let's explore some common operations you can perform with branches.
Create a Branch: To create a new branch, you can use the git branch
command followed by the branch name. For example, to create a branch named "feature-branch", you would run:
$ git branch feature-branch
Switch to a Branch: To switch to a different branch, you can use the git checkout
command followed by the branch name. For example, to switch to the "feature-branch", you would run:
$ git checkout feature-branch
Merge Branches: To merge changes from one branch into another, you can use the git merge
command. For example, to merge changes from the "feature-branch" into the "master" branch, you would run:
$ git checkout master $ git merge feature-branch
Delete a Branch: To delete a branch, you can use the git branch -d
command followed by the branch name. For example, to delete the "feature-branch", you would run:
$ git branch -d feature-branch
Related Article: How To Cherry Pick A Commit With Git
Creating a New Branch
When working with Git, branches are a vital part of managing your project's codebase. They allow you to work on new features, bug fixes, or experiments without affecting the main codebase. We will learn how to create a new branch in Git.
To create a new branch, you can use the git branch
command followed by the branch name. For example, if you want to create a branch named "feature-branch", you can run the following command:
$ git branch feature-branch
This will create a new branch but won't switch you to that branch. To switch to the newly created branch, you can use the git checkout
command:
$ git checkout feature-branch
Alternatively, you can use a single command git checkout -b
to create and switch to the new branch in one step:
$ git checkout -b feature-branch
Once you have created and switched to the new branch, you can start making changes to your code. It's important to note that any changes you make will only affect the branch you are currently on, keeping your main codebase intact.
To push your new branch to a remote repository, you can use the git push
command with the --set-upstream
flag:
$ git push --set-upstream origin feature-branch
This will create the new branch on the remote repository and set the upstream branch, allowing you to easily push and pull changes from the remote branch.
To see a list of all branches in your repository, you can use the git branch
command without any arguments:
$ git branch
The current branch will be highlighted with an asterisk (*). This command will list both local and remote branches.
Creating and using branches is an essential part of Git workflow. It allows you to work on multiple features simultaneously, collaborate with other developers, and keep your codebase organized. By following the steps outlined here, you will be able to create a new branch in Git and start working on your next great feature.
Switching Between Branches
Switching between branches is a fundamental task in Git. It allows you to move between different branches within your repository, enabling you to work on different features or bug fixes simultaneously.
To switch to an existing branch, you can use the git checkout
command followed by the name of the branch you want to switch to. For example, to switch to a branch named feature/new-feature
, you would run the following command:
git checkout feature/new-feature
If you're currently in a branch with uncommitted changes, Git won't allow you to switch branches unless you either commit or stash those changes. You can use the git stash
command to save your changes temporarily and switch branches. After switching branches, you can apply the saved changes using the git stash apply
command.
To create and switch to a new branch in one step, you can use the -b
flag with the git checkout
command. For example, to create and switch to a branch named bugfix/issue-123
, you would run the following command:
git checkout -b bugfix/issue-123
To list all the branches in your repository, you can use the git branch
command. The branch you are currently on will be indicated with an asterisk (*) next to its name. For example:
$ git branch bugfix/issue-123 feature/new-feature * main
To switch back to the main branch, you would run:
git checkout main
If you want to switch to a specific commit or a remote branch, you can use the full commit hash or the remote branch name in the git checkout
command. For example:
git checkout 1a2b3c4d # Switch to a specific commit git checkout origin/branch-name # Switch to a remote branch
Switching between branches allows you to easily navigate and work on different parts of your project. It is a powerful feature of Git that enables efficient collaboration and development workflows.
Viewing Branches
To effectively manage your Git branches, it's essential to be able to view all the branches in your repository. Git provides several commands to help you with this.
To view all the branches in your local repository, you can use the following command:
git branch
This command will list all the branches in your repository. The currently active branch will be indicated with an asterisk (*) symbol.
If you want to see both local and remote branches, you can use the -a
flag:
git branch -a
By running this command, you will get a list of both local and remote branches. Remote branches are branches that exist on the remote repository but haven't been fetched or checked out locally.
If you want to see more details about each branch, you can use the --verbose
or -v
flag:
git branch --verbose
This command will show additional information about each branch, such as the commit message and the last commit's author.
Sometimes, you may only be interested in specific branches. Git provides options to filter the branch list based on certain criteria. For example, you can use the --merged
flag to list only the branches that have been merged into the current branch:
git branch --merged
Similarly, you can use the --no-merged
flag to list only the branches that have not been merged into the current branch:
git branch --no-merged
This can be useful when you want to clean up your repository and delete branches that have already been merged.
If you want to view the branches in a remote repository, you can use the git ls-remote
command followed by the URL of the remote repository:
git ls-remote <remote-url>
This command will display a list of all the branches in the remote repository along with the commit hash for each branch.
Being able to view and understand the branches in your Git repository is crucial for effective branch management. Whether you need to check the status of branches, locate merged or unmerged branches, or explore branches in a remote repository, Git provides powerful commands to assist you.
Deleting a Local Branch
In Git, a branch is a lightweight pointer to a specific commit. As your project evolves, you may find yourself needing to delete local branches that are no longer necessary. This helps keep your repository clean and organized.
To delete a local branch in Git, you can use the git branch
command with the -d
or -D
option, followed by the branch name. The difference between the two options is that -d
will only delete the branch if it has been fully merged, while -D
will delete it regardless of its merge status.
Here's an example of how to delete a local branch named "feature/new-feature" that has been fully merged into the main branch:
$ git branch -d feature/new-feature
If the branch has not been fully merged, Git will display an error message and prevent you from deleting it using the -d
option. In such cases, you can use the -D
option to force the deletion:
$ git branch -D feature/new-feature
It's important to note that deleting a branch will not delete the commits made on that branch. The commits will still be accessible through other branches or by using their commit hashes.
To verify that the branch has been successfully deleted, you can use the git branch
command with no arguments. This will list all the local branches in your repository:
$ git branch
The deleted branch should no longer be listed.
Deleting local branches that are no longer needed can help reduce clutter and improve the overall organization of your repository. However, be cautious when deleting branches, especially those that contain unmerged work. Always ensure that you have a backup or another branch that includes the commits you want to keep.
If you need to delete a branch on a remote repository, such as GitHub, you will need to use the git push
command with the --delete
option, followed by the remote name and the branch name. For example:
$ git push origin --delete feature/new-feature
This will delete the branch named "feature/new-feature" on the remote repository named "origin".
Remember, deleting branches should be done with care, and it's always a good practice to double-check your intentions before permanently deleting any branches.
Related Article: How to Use Git Fast Forwarding
Deleting a Remote Branch
In Git, a remote branch is a branch that exists on a remote repository, such as GitHub or Bitbucket. Deleting a remote branch is useful when you no longer need the branch or want to clean up your repository.
To delete a remote branch, you need to execute the appropriate command. Here are the essential commands and steps to delete a remote branch in Git.
1. First, make sure you are in the local repository where the branch is located. You can use the command cd <repository_directory>
to navigate to the repository directory.
2. Next, fetch the latest changes from the remote repository using the command git fetch
. This ensures that you have the most up-to-date information about the remote branches.
3. To delete the remote branch, use the command git push <remote_name> --delete branch_name
. Replace <remote_name>
with the name of the remote repository (e.g., origin) and branch_name
with the name of the branch you want to delete.
For example, to delete a branch named "feature-branch" on the remote repository "origin", you would run the following command:
git push origin --delete feature-branch
4. After executing the command, Git will remove the remote branch from the repository. You can verify this by running
git branch -r
to list all remote branches. The branch you deleted should no longer be listed.
$ git branch -r
origin/main
origin/feature-branch
Note that the branch may still be visible on remote platforms like GitHub until you refresh the page.
5. Finally, to ensure your local branch references are updated, run
git remote prune <remote_name>
to remove any remote tracking branches that no longer exist on the remote repository.
For example, if your remote repository is named "origin", you would use the following command:
git remote prune origin
That's it! You have successfully deleted a remote branch in Git. Deleting remote branches helps keep your repository clean and organized. Remember to use caution when deleting branches, as they cannot be easily recovered once deleted.
Now that you know how to delete a remote branch, you can efficiently manage your Git repositories and keep them tidy.
Deleting a Branch with Unmerged Changes
Sometimes, you may want to delete a branch in Git that has unmerged changes. This typically happens when you are working on a branch and decide that you no longer need it. However, Git won't allow you to delete a branch with unmerged changes by default, as it wants to ensure that you don't accidentally lose any work.
To delete a branch with unmerged changes, you have a few options. One approach is to use the
-D
option with thegit branch
command. This option forces Git to delete the branch, even if it has unmerged changes. Here's the command you can use:
$ git branch -D branch_name
Replace
branch_name
with the name of the branch you want to delete. Keep in mind that this command will permanently delete the branch and all the unmerged changes associated with it. Make sure you have a backup or have pushed any important changes to a remote repository before using this command.
Another option is to use the
--merged
or--no-merged
options with thegit branch
command to filter branches based on their merge status. This allows you to see which branches have unmerged changes before deciding to delete them. Here's how you can use these options:
$ git branch --no-merged
This command will list all the branches that have unmerged changes. You can then decide which branch to delete based on this information.
If you want to delete a branch with unmerged changes but keep the changes as separate commits, you can use the
git stash
command to temporarily save the changes. Here's how you can do it:
$ git stash
This command will stash the changes in the branch, allowing you to switch to another branch. Once you have switched to the desired branch, you can safely delete the original branch using the
git branch -D
command. Afterward, if you want to apply the stashed changes, you can use thegit stash apply
command.
Deleting a branch with unmerged changes can be a powerful tool to keep your Git repository clean and organized. Just make sure to double-check and backup any important changes before deleting a branch.
Deleting a Merged Branch
When working with Git, it's common to create branches to work on specific features or bug fixes. Once a branch has served its purpose and its changes have been merged into the main branch, it's a good practice to delete the branch to keep your repository clean and organized.
Deleting a merged branch is a straightforward process and can be done using either the command line or a Git client. In this section, we will explore both methods.
To delete a merged branch using the command line, follow these steps:
1. Open your terminal or command prompt.
2. Navigate to the repository directory using the
cd
command.3. Run the following command:
$ git branch -d branch_name
Replace
branch_name
with the name of the branch you want to delete. This command will delete the branch only if it has been fully merged into the current branch. If the branch has unmerged changes, Git will display an error message and prevent the deletion. To force the deletion of an unmerged branch, use the-D
option instead:
$ git branch -D branch_name
Using a Git client, such as GitKraken or SourceTree, the process is equally straightforward. Simply locate the branch you want to delete in the client's interface, right-click on it, and select the option to delete the branch. The client will handle the deletion and ensure that the branch has been merged before proceeding.
It's important to note that deleting a branch does not delete any commits made on that branch. If you want to permanently delete the commits associated with the branch, you can use the
git gc
command to perform garbage collection and clean up any unreachable objects.
Deleting merged branches is a crucial step in maintaining a clean and efficient Git repository. By removing unnecessary branches, you can reduce clutter and improve the overall workflow for you and your team.
In the next section, we will explore how to delete a branch that has not been merged yet. Stay tuned!
Deleting Multiple Branches at Once
In Git, you can delete multiple branches at once using the
git branch
command along with the-d
or--delete
option. This is particularly useful when you have several branches that you no longer need and want to remove them in one go.
To delete multiple branches at once, you need to specify the branch names separated by spaces after the
git branch -d
command. For example, let's say you have branches named "feature-1", "feature-2", and "feature-3" that you want to delete:
$ git branch -d feature-1 feature-2 feature-3
Keep in mind that Git will not delete branches that haven't been merged into the current branch. If you try to delete an unmerged branch, Git will display an error message and prevent the deletion. This is to ensure that you don't accidentally lose any work.
If you have a large number of branches to delete, manually typing all the branch names can become tedious and error-prone. In such cases, you can use wildcard patterns to delete multiple branches that match a specific pattern.
For example, let's say you have numerous branches starting with the prefix "feature/" that you want to delete. You can use the
git branch
command with the-d
option and a wildcard pattern like this:
$ git branch -d feature/*
This command will delete all branches that match the pattern "feature/*". The asterisk (*) acts as a placeholder for any characters.
It's important to note that when you use wildcard patterns to delete branches, Git will prompt you to confirm the deletion of each branch individually. This is a safety measure to prevent accidental deletion of branches.
If you want to delete branches without being prompted for confirmation, you can use the
-D
or--delete
option instead of-d
. This option forces Git to delete the branches without any confirmation prompts.
$ git branch -D feature/*
By using the
-D
option, Git will delete all branches that match the pattern "feature/*" without asking for confirmation.
Deleting multiple branches at once can help you keep your Git repository clean and organized. It allows you to remove branches that are no longer needed, making it easier to focus on the active development branches.
Remember to exercise caution when using wildcard patterns for branch deletion. Double-check the pattern to ensure that you're not accidentally deleting branches that you want to keep.
We will explore how to delete remote branches in Git.
Related Article: How to Git Pull from a Specific Branch
Recovering a Deleted Branch
Sometimes, we accidentally delete a branch in Git and later realize that we still need it. Fortunately, Git keeps a record of all the branches that have been deleted, allowing us to recover them easily. In this section, we will explore the steps to recover a deleted branch in Git.
To recover a deleted branch, we can use the
reflog
command in Git. Thereflog
command displays the reference log, which contains a detailed history of all the branch updates and movements in Git. By examining the reference log, we can find the commit hash of the branch before it was deleted and use it to restore the branch.
Here are the steps to recover a deleted branch in Git:
1. Open your terminal or command prompt and navigate to the repository where the branch was deleted.
2. Run the following command to view the reference log:
git reflog
This command will display a list of all the branch updates and movements, including the commits that were made before the branch was deleted.
3. Identify the commit hash of the branch before it was deleted. The commit hash is a unique identifier for each commit in Git.
4. Once you have identified the commit hash, run the following command to recreate the deleted branch:
git branch <branch-name> <commit-hash>
Replace
<branch-name>
with the name of the branch you want to recover and<commit-hash>
with the commit hash you identified in the previous step.
5. Verify that the branch has been successfully recovered by running the
git branch
command:
git branch
This command will display a list of all the branches in the repository, including the recovered branch.
That's it! You have successfully recovered a deleted branch in Git using the
reflog
command. Remember to be cautious when deleting branches in the future and always double-check before permanently removing any branches.
For more information about the
reflog
command, you can refer to the official Git documentation on https://git-scm.com/docs/git-reflog.
Deleting a Branch in Real World Scenarios
In real-world scenarios, deleting a branch in Git is a common task that developers often perform to keep their repository clean and organized. Whether you are working on a personal project or collaborating with a team, understanding how to delete a branch correctly is essential.
There are several scenarios in which you might need to delete a branch:
1. Merging a branch: After successfully merging a branch into the main codebase, it is recommended to delete the branch to avoid clutter and confusion. This ensures that only active and relevant branches are present in the repository.
To delete a branch after merging, use the following command:
$ git branch -d branch_name
2. Abandoned feature branch: Sometimes, you may start working on a feature branch but later decide not to continue with it. In such cases, deleting the branch is necessary to maintain a clean repository history.
To delete an abandoned feature branch, use the following command:
$ git branch -D branch_name
3. Hotfix branch: When a critical bug or issue arises in the production code, a hotfix branch is created to address the problem quickly. Once the hotfix is merged and deployed, the branch can be deleted.
To delete a hotfix branch, use the following command:
$ git branch -d branch_name
4. Obsolete branch: Over time, branches can become obsolete or irrelevant. It's important to regularly clean up these branches to minimize confusion and reduce repository clutter.
To delete an obsolete branch, use the following command:
$ git branch -D branch_name
5. Experimental branch: In some cases, developers create experimental branches to test new ideas or approaches. Once the experiments are complete, the branch can be removed from the repository.
To delete an experimental branch, use the following command:
$ git branch -d branch_name
Remember that the
-d
option stands for "delete" and is used for removing merged branches. The-D
option is used to force delete a branch, even if it contains unmerged changes.
It's worth mentioning that when deleting a branch, the branch's commit history is not lost. All the commits made on that branch are still accessible through other branches or by using the commit SHA.
Deleting branches regularly helps maintain a clean and manageable Git repository. By keeping only relevant and active branches, you create a more organized development environment for yourself and your team.
We will explore how to handle branch deletion conflicts and recover accidentally deleted branches. Stay tuned!
Advanced Techniques for Deleting Branches
Deleting branches is a common task when working with Git. In addition to the basic commands for deleting branches, there are some advanced techniques that can be useful in certain situations. We will explore these advanced techniques.
Deleting a Remote Branch
To delete a branch on a remote repository, you can use the
git push
command with the--delete
or-d
flag, followed by the remote name and the branch name. For example, to delete a branch named "feature" on the "origin" remote repository, you can use the following command:
git push origin --delete feature
This command will remove the branch from the remote repository, and it will no longer be available to other collaborators.
Deleting Multiple Branches
If you have multiple branches that you want to delete at once, you can use the
git branch
command with the-D
flag, followed by the branch names. For example, to delete branches named "feature1" and "feature2", you can use the following command:
git branch -D feature1 feature2
This command will delete both branches from your local repository. Make sure you have committed or saved any changes you want to keep before running this command, as it is irreversible.
Deleting Merged Branches
When working on a collaborative project, branches are often merged into the main branch (e.g., "master" or "main") once the changes are completed. In such cases, deleting the merged branches is a good practice to keep the repository clean.
To delete all merged branches, you can use the
git branch
command with the--merged
flag. This will list all the branches that have been merged into the current branch. You can then pipe this output to thexargs
command and use thegit branch -D
command to delete all the branches listed. Here's an example:
git branch --merged | grep -v "\*" | xargs -n 1 git branch -D
This command will delete all the branches that have been merged into the current branch, except the current branch itself. It is a good idea to run this command periodically to clean up your local repository.
Deleting Remote Tracking Branches
When you fetch changes from a remote repository, Git creates remote tracking branches to keep track of the remote branches. To delete these remote tracking branches, you can use the
git branch
command with the-r
flag and the-d
flag. For example, to delete a remote tracking branch named "origin/feature", you can use the following command:
git branch -d -r origin/feature
This command will remove the remote tracking branch from your local repository.
Deleting Branches with Force
Sometimes, you may encounter situations where Git prevents you from deleting a branch because it has unmerged changes or because it is the current branch. In such cases, you can use the force option to delete the branch.
To delete a branch with force, you can use the following command:
git branch -D branch_name
Replace
branch_name
with the name of the branch you want to delete. The-D
option is a shorthand for--delete --force
.
This command will delete the branch even if it has unmerged changes or if it is the current branch. However, be cautious when using the force option as it can result in the loss of unmerged changes.
Let's say you have a branch called
feature/new-feature
that you want to delete forcefully. You can execute the following command:
git branch -D feature/new-feature
If the branch exists and can be deleted forcefully, Git will remove it without any warnings or confirmation prompts.
It's important to note that the force option should be used with caution. Make sure you understand the consequences of deleting a branch forcefully, as it can lead to the loss of important changes. Always double-check before using this option.
Deleting branches with force can be useful in situations where you have already merged the changes from a branch and want to clean up your repository by removing the branch.
Related Article: Fixing the Git Error: "Git Not Recognized As A Command"
Deleting Branches with Wildcards
In Git, you can delete branches using wildcards to delete multiple branches at once. This can be especially useful when you have multiple branches that follow a similar naming pattern and you want to delete them all in one go.
To delete branches with wildcards, you can use the
git branch
command with the-D
flag followed by the wildcard expression. The-D
flag is used to force delete the branches, even if they have unmerged changes.
Here's an example of how you can delete branches with a wildcard expression:
$ git branch -D feature/*
In this example, the wildcard expression
feature/*
matches all branches that start with "feature/". This command will delete all branches that follow this naming pattern.
If you want to delete branches that match a specific pattern at any position in the branch name, you can use the
git branch
command along with the--list
flag and the wildcard expression.
For example, to delete all branches that contain the word "bugfix" in their name, you can use the following command:
$ git branch -D $(git branch --list '*bugfix*')
In this command, the
git branch --list '*bugfix*'
lists all branches that contain the word "bugfix" in their name. The output is then passed as an argument to thegit branch -D
command, which deletes all the listed branches.
It's important to note that the
git branch -D
command will permanently delete the branches, so make sure you have a backup or have pushed the branches to a remote repository if necessary.
If you want to delete branches that have already been merged into the current branch, you can use the
git branch
command with the--merged
flag and the wildcard expression.
For example, to delete all branches that have been merged into the current branch and start with "feature/", you can use the following command:
$ git branch -D $(git branch --merged | grep 'feature/')
In this command, the
git branch --merged
lists all branches that have been merged into the current branch. The output is then piped to thegrep
command, which filters the branches that start with "feature/". The filtered output is then passed as an argument to thegit branch -D
command, which deletes the branches.
Deleting branches with wildcards can be a powerful technique to clean up your repository and remove branches that are no longer needed. Just be cautious and double-check before deleting any branches to avoid losing important code or changes.