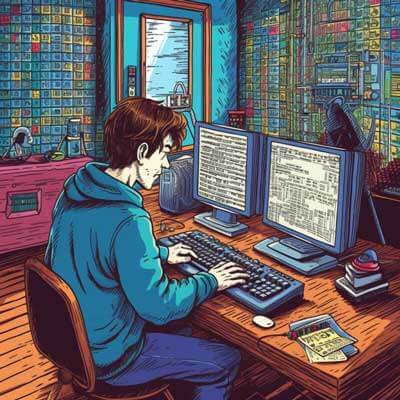
Table of Contents
To discard all local changes in a Git project, you can use the git checkout
command with the -- .
option. This will revert all files in the current directory to their last committed state. Here are the steps to follow:
Step 1: Open the Terminal or Command Prompt
Open your preferred terminal or command prompt application. This could be the built-in terminal in your code editor or a separate terminal window.
Related Article: How to Undo Last Commits in Git
Step 2: Navigate to the Git Project Directory
Use the cd
command to navigate to the directory of your Git project. For example, if your project is located in the Documents
folder, you can use the following command:
cd Documents/my-git-project
Make sure you replace my-git-project
with the actual name of your Git project.
Step 3: Verify Local Changes
Before discarding all local changes, it's a good practice to verify the changes you have made. You can use the git status
command to see the current status of your project. This will display a list of modified files, untracked files, and other relevant information.
git status
Step 4: Discard All Local Changes
To discard all local changes, use the following command:
git checkout -- .
This command will recursively revert all files in the current directory and its subdirectories to their last committed state. The -- .
part instructs Git to apply the checkout operation to the entire directory.
Related Article: How To Combine My Last N Commits In Git
Step 5: Verify Discarded Changes
After executing the git checkout -- .
command, you can use the git status
command again to verify that all local changes have been discarded. The output should indicate that there are no modifications.
git status
Alternative Approach: Stashing Local Changes
If you want to preserve your local changes for future use but temporarily discard them, you can use Git's stash feature. Stashing allows you to save your changes on a stack and revert back to the last committed state. Here's how you can do it:
Step 1: Verify Local Changes
Before stashing your changes, verify the modifications you have made using the git status
command.
git status
Step 2: Create a New Stash
To create a new stash, use the following command:
git stash save "My changes"
Replace "My changes"
with a descriptive message that represents the changes you are stashing.
Step 3: Verify Stash
You can use the git stash list
command to view the list of stashes you have created.
git stash list
Step 4: Apply Stashed Changes
If you want to reapply the stashed changes later, you can use the following command:
git stash apply stash@{0}
Replace stash@{0}
with the appropriate stash reference from the git stash list
command output.
Step 5: Discard Stashed Changes
If you decide that you no longer need the stashed changes, you can use the git stash drop
command to discard them.
git stash drop stash@{0}
Replace stash@{0}
with the appropriate stash reference from the git stash list
command output.
Best Practices
- Before discarding all local changes, make sure you have a backup of any important modifications. Double-check that you don't permanently lose any work.
- It's a good practice to commit your changes regularly instead of relying on discarding local changes. Committing allows you to have a history of your modifications and makes it easier to track and revert changes if needed.
- Use the stash feature if you want to temporarily discard changes but keep them for future use. Stashing allows you to switch between different branches or tasks without losing your modifications.
- If you accidentally discard changes and want to recover them, you can use the git reflog
command to view the history of your branch and find the commit where the changes were discarded. From there, you can create a new branch or cherry-pick the changes back into your current branch.