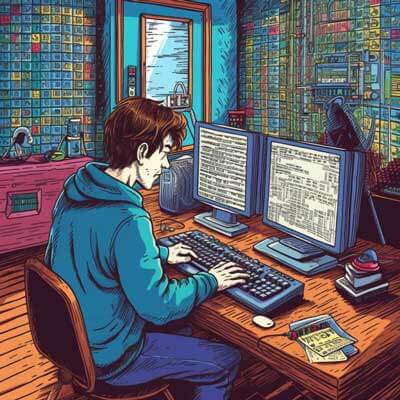
Moving recent commits to a new branch in Git can be done in a few simple steps. This process is particularly useful when you realize that certain commits should have been made on a separate branch instead of the current branch. It allows you to maintain a clean commit history and organize your code more effectively. Here are two possible ways to achieve this:
Method 1: Using the git branch and git cherry-pick commands
1. First, create a new branch at the current commit using the following command:
git branch <new-branch-name>
For example, if you want to create a new branch named “feature-branch” at the current commit, you would run:
git branch feature-branch
2. Switch to the new branch using the git checkout command:
git checkout <new-branch-name>
For example, to switch to the “feature-branch”, you would run:
git checkout feature-branch
3. Identify the commit(s) you want to move to the new branch. You can use the git log command to view the commit history and find the commit hashes. Take note of the commit hash(es) of the recent commits you want to move.
4. Cherry-pick the commit(s) from the previous branch to the new branch using the git cherry-pick command:
git cherry-pick <commit-hash>
Replace <commit-hash>
with the actual commit hash of the commit you want to move. You can repeat this command for each commit you want to move to the new branch.
For example, to cherry-pick a commit with the hash “abc123” to the current branch, you would run:
git cherry-pick abc123
If you have multiple commits to move, you can specify multiple commit hashes in a single command, separated by spaces.
5. After cherry-picking all the necessary commits, you can push the changes to the remote repository if needed using the git push command:
git push origin <new-branch-name>
This will create the new branch on the remote repository and push the commits to it.
Related Article: How to Discard All Local Changes in a Git Project
Method 2: Using the git rebase command
1. Start by creating a new branch at the current commit, similar to Method 1.
2. Switch to the new branch using the git checkout command.
3. Run the following command to initiate an interactive rebase session:
git rebase -i <commit-hash>
Replace <commit-hash>
with the commit hash of the commit just before the first commit you want to move. This will open a text editor with a list of commits.
4. In the text editor, find the line(s) corresponding to the commits you want to move and change the word “pick” to “edit” or “e” for each of those lines.
5. Save and close the text editor. Git will automatically stop at each commit you marked for editing.
6. For each commit you want to move, run the following command to amend the commit to the new branch:
git commit --amend
This will open a text editor where you can modify the commit message if needed. Save and close the text editor.
7. After amending the commit, you can continue the rebase process by running:
git rebase --continue
Git will apply the remaining commits and stop again if there are more commits to move.
8. Once the rebase is complete, you can push the changes to the remote repository if needed.
Best Practices and Considerations
– Before moving commits to a new branch, it’s important to ensure that you have a clean working directory and that there are no uncommitted changes. Use the git status command to check the status of your repository before starting the process.
– It’s generally recommended to create a new branch at the current commit before attempting to move commits. This allows you to keep the original branch intact in case you need to revert any changes.
– When cherry-picking commits, be aware that the new branch will have different commit hashes compared to the original branch. This can cause conflicts if the original branch has already been merged into other branches. It’s important to communicate and coordinate with other team members to avoid potential conflicts.
– If you’re unsure about which method to use or want to experiment with moving commits without modifying your repository, you can create a new local repository and test the process there. This can help you gain confidence before applying the changes to your main repository.
– Remember to communicate any changes made to the commit history, especially if you’re working in a team. Clear and concise commit messages can help others understand the changes and make collaboration smoother.
For more information on Git and its various commands, you can refer to the official Git documentation: https://git-scm.com/doc.
Related Article: How to Stash Untracked Files in Git