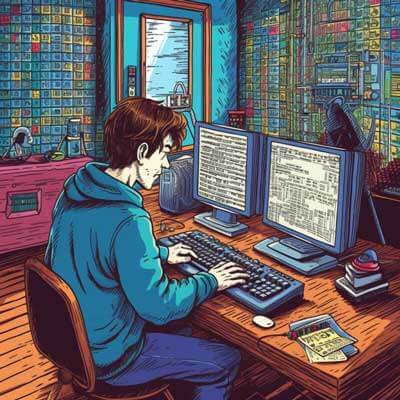
Table of Contents
Project Requirements and Tools
To build an interactive language learning game, we will be using a combination of technologies and tools that are well-suited for the task. These include:
- GraphQL: A query language for APIs that allows us to define the data requirements of our game and retrieve them efficiently.
- Node.js: A JavaScript runtime that allows us to run JavaScript code on the server side. We will be using Node.js to build the backend logic of our game.
- React.js: A JavaScript library for building user interfaces. We will be using React.js to create the game interface.
- MongoDB: A NoSQL database that provides a flexible and scalable solution for storing language-related data.
- Docker: A platform that allows us to package our application and its dependencies into containers, making it easier to deploy and manage.
Related Article: Fix The Engine Node Is Incompatible With This Module Nodejs
Building the Backend with Node.js
The backend of our language learning game will be responsible for handling requests from the frontend, managing user profiles and progress, and implementing the game logic. We can achieve this using Node.js, a useful and efficient runtime for building server-side applications.
To get started, we need to set up a new Node.js project. Open your terminal and run the following command:
mkdir language-learning-game-backend cd language-learning-game-backend npm init -y
This will create a new directory for our project and initialize a new Node.js project with default settings. Next, we need to install some dependencies. Run the following command to install the required packages:
npm install express graphql express-graphql mongoose
We are installing the following packages:
- express: A fast and minimalist web framework for Node.js, which we will use to handle HTTP requests.
- graphql: The core package for implementing a GraphQL server.
- express-graphql: A package that allows us to easily integrate GraphQL with Express.
- mongoose: A MongoDB object modeling tool that provides a straightforward way to interact with the database.
Once the installation is complete, we can start building our backend logic. Create a new file called server.js
in the root directory of our project and add the following code:
const express = require('express'); const { graphqlHTTP } = require('express-graphql'); const { buildSchema } = require('graphql'); // Define our GraphQL schema const schema = buildSchema(` type Query { hello: String } `); // Define our resolvers const root = { hello: () => 'Hello, world!', }; // Create an Express server const app = express(); // Configure the GraphQL endpoint app.use('/graphql', graphqlHTTP({ schema: schema, rootValue: root, graphiql: true, })); // Start the server app.listen(3000, () => { console.log('Server started on port 3000'); });
In this code, we are defining a simple GraphQL schema with a single query field called "hello" that returns a string. We also define a resolver function that returns the string "Hello, world!" when the "hello" query is executed.
To run the server, use the following command:
node server.js
Now, if you open your browser and navigate to http://localhost:3000/graphql
, you should see a GraphQL playground where you can execute the "hello" query and see the result.
Implementing GraphQL for User Profiles and Progress
In our language learning game, we need to manage user profiles and track their progress. GraphQL provides a flexible and efficient way to handle these requirements.
To implement user profiles, we can define a new type in our GraphQL schema called "User" with fields for the user's name, email, and language proficiency. We can also define a mutation field for creating a new user. Here's an example schema:
type User { id: ID! name: String! email: String! proficiency: String } type Query { user(id: ID!): User users: [User] } input CreateUserInput { name: String! email: String! proficiency: String } type Mutation { createUser(input: CreateUserInput!): User }
In this schema, we define a "User" type with fields for the user's id, name, email, and proficiency. We also define query fields for retrieving a specific user by id and retrieving all users. Additionally, we define a mutation field for creating a new user, which takes an input object of type "CreateUserInput".
To implement the resolvers for these schema fields, we can use the mongoose package to interact with our MongoDB database. Here's an example implementation:
const mongoose = require('mongoose'); // Connect to MongoDB mongoose.connect('mongodb://localhost/language-learning-game', { useNewUrlParser: true, useUnifiedTopology: true, }); // Define the User model const User = mongoose.model('User', { name: String, email: String, proficiency: String, }); // Define the resolvers const root = { user: async ({ id }) => { return await User.findById(id); }, users: async () => { return await User.find(); }, createUser: async ({ input }) => { const user = new User(input); await user.save(); return user; }, };
In this code, we connect to our MongoDB database using the mongoose package. We define a User model that maps to a collection in the database. We then define the resolvers for the user and users queries, as well as the createUser mutation.
With this implementation in place, we can now create and retrieve user profiles using GraphQL.
Storing Language Data with MongoDB
To store language-related data in our language learning game, we can use MongoDB, a NoSQL database that provides a flexible and scalable solution for storing and retrieving data.
First, we need to install the mongoose package, which provides a straightforward way to interact with MongoDB from our Node.js application. Run the following command to install mongoose:
npm install mongoose
Next, we need to define a schema for our language data. Create a new file called language.js
in a new directory called models
, and add the following code:
const mongoose = require('mongoose'); // Define the Language schema const LanguageSchema = new mongoose.Schema({ name: String, phrases: [{ text: String, translation: String, }], }); // Create the Language model const Language = mongoose.model('Language', LanguageSchema); module.exports = Language;
In this code, we define a schema for our language data, which consists of a name field and an array of phrases. Each phrase has a text field for the original phrase and a translation field for the translated phrase. We then create a mongoose model for the Language schema and export it.
To use this model in our application, we need to modify our server.js
file. Add the following code at the top of the file, before the definition of the root resolver:
const Language = require('./models/language');
Then, update the root resolver to include a query field for retrieving languages and a mutation field for creating a new language:
const root = { hello: () => 'Hello, world!', language: async ({ id }) => { return await Language.findById(id); }, languages: async () => { return await Language.find(); }, createLanguage: async ({ input }) => { const language = new Language(input); await language.save(); return language; }, };
Now, we can use GraphQL to create and retrieve language data from our MongoDB database.
Related Article: How to Fix the “getaddrinfo ENOTFOUND” Error in Node.js
Creating the Game Logic with Node.js
The game logic of our language learning game will be responsible for generating game sessions, managing user progress, and handling game events. We can implement this logic using Node.js, a useful and efficient runtime for building server-side applications.
To get started, we need to define the game rules and mechanics. For example, our game could involve matching foreign language phrases with their translations, completing sentence fragments, or answering multiple-choice questions.
Once we have defined the game rules, we can create a new file called gameLogic.js
and implement the necessary functions and classes. Here's an example implementation:
class GameSession { constructor(user, language) { this.user = user; this.language = language; this.score = 0; this.currentQuestion = null; } start() { // Generate a new question this.currentQuestion = this.generateQuestion(); // Update the user's progress this.user.progress.push(this.currentQuestion.id); } answerQuestion(answer) { // Check if the answer is correct if (answer === this.currentQuestion.answer) { this.score++; } // Generate a new question this.currentQuestion = this.generateQuestion(); // Update the user's progress this.user.progress.push(this.currentQuestion.id); } generateQuestion() { // Generate a new question based on the game rules and mechanics } } module.exports = GameSession;
In this code, we define a GameSession class that represents a single game session. It has properties for the user, language, score, and current question. The start() method is called when a new game session is started and generates the first question. The answerQuestion() method is called when the user answers a question and updates the score and generates a new question. The generateQuestion() method is responsible for generating a new question based on the game rules and mechanics.
To use the game logic in our application, we need to modify our server.js
file. Add the following code at the top of the file, before the definition of the root resolver:
const GameSession = require('./gameLogic');
Then, update the root resolver to include a mutation field for starting a new game session and answering a question:
const root = { hello: () => 'Hello, world!', user: async ({ id }) => { return await User.findById(id); }, users: async () => { return await User.find(); }, createUser: async ({ input }) => { const user = new User(input); await user.save(); return user; }, startGame: async ({ userId, languageId }) => { const user = await User.findById(userId); const language = await Language.findById(languageId); const gameSession = new GameSession(user, language); gameSession.start(); return gameSession; }, answerQuestion: async ({ sessionId, answer }) => { const gameSession = await GameSession.findById(sessionId); gameSession.answerQuestion(answer); return gameSession; }, };
With this implementation, we can now start game sessions and answer questions using GraphQL.
Designing the Game Interface with React.js
The game interface of our language learning game will be responsible for displaying game content, capturing user input, and providing feedback to the user. We can implement this interface using React.js, a JavaScript library for building user interfaces.
To get started, we need to set up a new React.js project. Open your terminal and run the following command:
npx create-react-app language-learning-game-frontend
This will create a new directory for our project and set up a new React.js project with default settings. Next, we need to install some dependencies. Run the following command to install the required packages:
cd language-learning-game-frontend npm install react-apollo graphql apollo-boost
We are installing the following packages:
- react-apollo: A React.js integration for Apollo Client, which allows us to easily fetch and manage GraphQL data in our React components.
- graphql: The core package for implementing a GraphQL client.
- apollo-boost: A package that includes everything we need to get started with Apollo Client.
Once the installation is complete, we can start building our game interface. Open the src/App.js
file and replace its contents with the following code:
import React, { useState } from 'react'; import { useQuery, useMutation, gql } from '@apollo/client'; const GET_RANDOM_QUESTION = gql` query GetRandomQuestion { randomQuestion { id text translation } } `; const ANSWER_QUESTION = gql` mutation AnswerQuestion($input: AnswerQuestionInput!) { answerQuestion(input: $input) { id score } } `; function App() { const [answer, setAnswer] = useState(''); const { loading, data, refetch } = useQuery(GET_RANDOM_QUESTION); const [answerQuestion] = useMutation(ANSWER_QUESTION); const handleSubmit = async (event) => { event.preventDefault(); await answerQuestion({ variables: { input: { answer: answer, }, }, }); setAnswer(''); refetch(); }; if (loading) { return <div>Loading...</div>; } return ( <div> <h2>Language Learning Game</h2> <form onSubmit={handleSubmit}> <p>{data.randomQuestion.text}</p> <input type="text" value={answer} onChange={(event) => setAnswer(event.target.value)} required /> <button type="submit">Submit</button> </form> </div> ); } export default App;
In this code, we define two GraphQL operations: GET_RANDOM_QUESTION
and ANSWER_QUESTION
. The GET_RANDOM_QUESTION
query fetches a random question from the server, and the ANSWER_QUESTION
mutation submits the user's answer to the server.
We use the useQuery
hook to fetch the random question and the useMutation
hook to handle the answer submission. We use the refetch
function to fetch a new random question after the user submits their answer.
The handleSubmit
function is called when the user submits their answer. It calls the answerQuestion
mutation with the user's answer and then resets the answer input and refetches a new random question.
Finally, we render the game interface with a title, the current question text, an input field for the user's answer, and a submit button.
To start the game interface, open the src/index.js
file and replace its contents with the following code:
import React from 'react'; import ReactDOM from 'react-dom'; import { ApolloProvider, ApolloClient, InMemoryCache } from '@apollo/client'; import App from './App'; const client = new ApolloClient({ uri: 'http://localhost:3000/graphql', cache: new InMemoryCache(), }); ReactDOM.render( <ApolloProvider client={client}> <App /> </ApolloProvider>, document.getElementById('root') );
In this code, we create a new Apollo Client instance with the URL of our GraphQL server and an in-memory cache. We then wrap the game interface component with the ApolloProvider
component, passing the client instance as a prop.
To run the game interface, use the following command:
npm start
Now, if you open your browser and navigate to http://localhost:3000
, you should see the game interface with a random question and an input field for answering the question.
Deploying the Game with Docker
To simplify the deployment of our language learning game, we can use Docker, a platform that allows us to package our application and its dependencies into containers. This makes it easier to deploy and manage our game on different environments.
To get started, we need to create a Dockerfile that specifies the steps for building our Docker image. Create a new file called Dockerfile
in the root directory of our project and add the following code:
# Use the official Node.js 14 image as the base image FROM node:14 # Set the working directory in the container WORKDIR /app # Copy the package.json and package-lock.json files to the container COPY package*.json ./ # Install the dependencies RUN npm install # Copy the rest of the application files to the container COPY . . # Expose port 3000 EXPOSE 3000 # Start the server CMD ["node", "server.js"]
In this Dockerfile, we start with the official Node.js 14 image as the base image. We set the working directory in the container to /app
and copy the package.json and package-lock.json files to the container. We then install the dependencies using npm install
. Finally, we copy the rest of the application files to the container, expose port 3000, and start the server.
To build the Docker image, open your terminal and run the following command:
docker build -t language-learning-game .
This will build a Docker image with the tag language-learning-game
based on the Dockerfile in the current directory.
Once the image is built, we can run a container from the image. Run the following command to start a new container:
docker run -p 3000:3000 language-learning-game
This will start a new container from the language-learning-game
image and map port 3000 of the container to port 3000 on the host machine.
Now, if you open your browser and navigate to http://localhost:3000
, you should be able to access and play the language learning game.
Strategies for Practicing Vocabulary and Phrases
When building an interactive language learning game, it's important to consider effective strategies for practicing vocabulary and phrases. Here are a few strategies that can be implemented in our game:
1. Flashcards: Implement a flashcard-style game where users are presented with a word or phrase in the target language and have to choose the correct translation from a list of options. This helps users practice recognition and recall.
2. Listening Exercises: Include audio clips of native speakers pronouncing words or phrases, and ask users to select the corresponding written translation. This helps users improve their listening comprehension skills.
3. Sentence Completion: Provide incomplete sentences or phrases in the target language, and ask users to complete them with the correct word or phrase. This helps users practice grammar and sentence structure.
4. Multiple-Choice Questions: Present users with multiple-choice questions that test their knowledge of vocabulary, grammar, and cultural aspects of the target language. This helps users reinforce their understanding of the language.
5. Spelling Challenges: Ask users to spell words or phrases in the target language correctly. This helps users improve their spelling and reinforces their understanding of the language's writing system.
Related Article: How to Write an Nvmrc File for Automatic Node Version Change
Improving Language Learning through the Game
An interactive language learning game can be a valuable tool for improving language learning outcomes. Here are a few ways in which our game can enhance the language learning experience:
1. Engagement: By making language learning fun and interactive, our game can engage users and motivate them to practice more frequently. The game mechanics, rewards, and progression system can create a sense of achievement and encourage users to continue learning.
2. Contextual Learning: By presenting language content in the context of a game, our game can help users understand the practical usage of vocabulary and phrases. This can improve their comprehension and retention of language skills.
3. Repetition and Reinforcement: Our game can provide repeated exposure to vocabulary and phrases through gameplay, which helps reinforce learning and improve memorization. By encountering words and phrases in different contexts and exercises, users can develop a deeper understanding of the language.
4. Interactive Feedback: Our game can provide immediate feedback to users, allowing them to learn from their mistakes and correct their understanding. By highlighting correct and incorrect answers, explaining the correct answers, and providing hints or explanations, our game can help users learn from their errors and improve their language skills.
5. Personalized Learning: By using GraphQL to manage user profiles and progress, our game can track individual users' strengths and weaknesses and adapt the gameplay and exercises accordingly. This personalized learning approach can optimize the learning experience for each user and provide targeted practice in areas that need improvement.
Key Features of an Interactive Language Learning Game
An interactive language learning game should include key features that enhance the learning experience and provide a comprehensive language learning tool. Here are some important features to consider:
1. User Profiles: Allow users to create and manage their profiles, including personal information, language preferences, and learning goals. This allows for personalized learning experiences and progress tracking.
2. Progress Tracking: Track and display users' progress, including their scores, completed exercises, and areas for improvement. This helps users monitor their progress and motivates them to continue learning.
3. Game Mechanics: Implement game mechanics such as levels, achievements, rewards, and leaderboards to make the learning experience more engaging and motivating. This provides a sense of accomplishment and encourages users to continue practicing.
4. Varied Exercises: Include a variety of exercises and activities that cover different aspects of language learning, such as vocabulary, grammar, listening comprehension, and cultural knowledge. This ensures a well-rounded learning experience and caters to different learning styles.
5. Feedback and Correction: Provide immediate feedback to users on their answers, highlighting correct and incorrect responses. Offer explanations or hints to help users understand their mistakes and improve their understanding of the language.
6. Interactive Interface: Design an intuitive and user-friendly interface that is easy to navigate and interact with. Use visuals, animations, and audio to enhance the learning experience and make it more engaging.
7. Customization Options: Allow users to customize their learning experience by selecting specific topics, difficulty levels, or exercise types. This gives users more control over their learning journey and allows them to focus on areas of interest or challenge.
8. Social Features: Incorporate social features such as the ability to compete with friends, share achievements, or join language learning communities. This adds a social aspect to the game and enables users to learn from and support each other.
Benefits of GraphQL, Node.js, React.js, MongoDB, and Docker
Using GraphQL, Node.js, React.js, MongoDB, and Docker in our language learning game provides several benefits:
1. GraphQL:
- Efficient Data Fetching: GraphQL allows us to define the data requirements of our game and retrieve them efficiently with a single request. This reduces over-fetching and under-fetching of data, improving performance and reducing network overhead.
- Flexible API Development: GraphQL provides a flexible and intuitive way to define APIs, allowing us to easily evolve and iterate on our game's data requirements without breaking existing clients.
- Strong Typing System: GraphQL's type system provides a clear and self-documenting contract between the client and server, reducing the risk of miscommunication and improving the development process.
- Tooling and Ecosystem: GraphQL has a vibrant ecosystem with tools, libraries, and community support that make it easier to develop, test, and maintain our game's API.
2. Node.js:
- JavaScript Everywhere: Node.js allows us to write server-side code in JavaScript, which provides a consistent and familiar development experience for full-stack developers.
- Scalability: Node.js is designed to handle a large number of concurrent requests efficiently, making it well-suited for building high-performance and scalable server-side applications.
- Rich Ecosystem: Node.js has a rich ecosystem of modules and libraries that can accelerate development and provide solutions for common challenges.
- Asynchronous Programming: Node.js's non-blocking I/O model and event-driven architecture enable us to write highly performant and responsive server-side code.
3. React.js:
- Component-Based Architecture: React.js's component-based architecture promotes reusability, modularity, and maintainability of our game's user interface code.
- Virtual DOM: React.js's virtual DOM allows for efficient updates and rendering of UI components, resulting in improved performance and a smoother user experience.
- Declarative Syntax: React.js's declarative syntax makes it easier to reason about and debug our game's user interface code, reducing complexity and improving developer productivity.
- Large and Active Community: React.js has a large and active community that provides support, resources, and community-driven tools and libraries.
4. MongoDB:
- Schema Flexibility: MongoDB's flexible schema allows us to store language-related data with varying structures and easily adapt to changing requirements.
- Scalability and Performance: MongoDB's horizontal scaling capabilities and efficient indexing mechanisms provide high performance and scalability for our game's data storage needs.
- Document-Oriented Model: MongoDB's document-oriented model aligns well with the structure of language-related data, making it a natural fit for our game's requirements.
- Rich Query Language: MongoDB's query language provides useful and expressive querying capabilities, allowing us to retrieve and manipulate language-related data efficiently.
5. Docker:
- Reproducible Builds: Docker enables us to create reproducible build environments, ensuring that our language learning game runs consistently across different environments.
- Dependency Management: Docker allows us to package our game and its dependencies into containers, making it easier to manage and isolate dependencies and reducing the risk of conflicts.
- Portability: Docker containers are portable and can be easily deployed on different environments, reducing deployment and configuration issues.
- Scalability: Docker's containerization technology allows us to scale our game horizontally by running multiple instances of the same container, providing scalability and load balancing capabilities.
Using these technologies and tools in our language learning game enables us to develop a scalable, efficient, and maintainable application with an engaging and interactive user interface.
Accessing and Playing the Game
To access and play the language learning game, follow these steps:
1. Open your web browser and navigate to the URL where the game is deployed. For example, if the game is deployed locally, navigate to http://localhost:3000
.
2. If you are a new user, click on the "Sign up" or "Create an account" button to create a new user profile. Provide the required information, such as your name, email, and language proficiency.
3. Once you have created a user profile, you can log in using your credentials.
4. After logging in, you will be presented with the game interface. Follow the instructions and complete the exercises to practice vocabulary and phrases in the target language.
5. As you progress through the game, your score and achievements will be tracked and displayed in your user profile.
6. You can continue playing the game, track your progress, and improve your language skills by regularly accessing the game and completing the exercises.