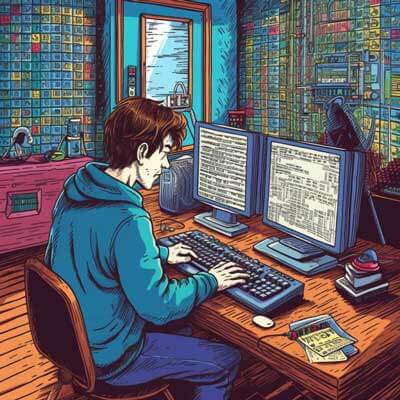
- Integrating Phoenix with Payment Platforms
- Libraries for Phoenix and Stripe Integration
- Using ExStripe to Integrate Phoenix with Stripe
- Setting up Voice Functionalities in Phoenix Apps
- Using Twilio for Voice Functionalities in Phoenix Apps
- Setting up SMS Functionalities in Phoenix Apps
- Using Twilio for SMS Functionalities in Phoenix Apps
- Building Chatbots with Phoenix
- Example: Building a Simple Chatbot
- Creating Other Voice Interfaces with Phoenix
- Example: Creating a Simple Voice Interface
- Popular Payment Platforms for Phoenix Integration
- 1. Stripe
- 2. PayPal
- 3. Braintree
- 4. Square
- Additional Resources
Integrating Phoenix with Payment Platforms
Integrating payment platforms with web applications is crucial for businesses that rely on online transactions. Phoenix, a web framework built with Elixir, provides a robust platform for developing scalable and high-performance applications. In this section, we will explore how to integrate Phoenix with popular payment platforms like Stripe.
Related Article: Phoenix with Bootstrap, Elasticsearch & Databases
Libraries for Phoenix and Stripe Integration
When it comes to integrating Phoenix with Stripe, there are several libraries available that simplify the process. These libraries provide an abstraction layer that handles the communication with the Stripe API, making it easier to implement payment functionality in your Phoenix application. Let’s take a look at one of the popular libraries for Phoenix and Stripe integration: ExStripe.
Using ExStripe to Integrate Phoenix with Stripe
ExStripe is a useful library that allows developers to seamlessly integrate Phoenix with the Stripe payment platform. With ExStripe, you can easily create customers, charge credit cards, manage subscriptions, and handle other payment-related tasks.
To get started with ExStripe, you need to add it as a dependency in your Phoenix project’s mix.exs
file:
defp deps do [ {:ex_stripe, "~> 2.0"} ] end
After adding the dependency, run mix deps.get
to fetch and compile the library.
Next, you need to configure ExStripe with your Stripe API keys. In your Phoenix application’s config/config.exs
file, add the following configuration:
config :ex_stripe, secret_key: "YOUR_STRIPE_SECRET_KEY", publishable_key: "YOUR_STRIPE_PUBLISHABLE_KEY"
Replace YOUR_STRIPE_SECRET_KEY
and YOUR_STRIPE_PUBLISHABLE_KEY
with your actual Stripe API keys.
With ExStripe configured, you can now start using its features in your Phoenix application. Let’s take a look at a couple of examples to see how ExStripe can be used to handle payments.
Example 1: Creating a Customer and Charging a Credit Card
def create_customer_and_charge_credit_card(user, card_token, amount) do customer = ExStripe.Customer.create(%{ email: user.email, source: card_token }) ExStripe.Charge.create(%{ amount: amount, currency: "usd", customer: customer.id }) end
In this example, we define a function create_customer_and_charge_credit_card
that takes a user object, a card token (obtained from Stripe.js or Elements), and an amount. The function creates a customer in Stripe using the provided email and card token, and then charges the customer’s credit card with the specified amount.
Example 2: Handling Subscriptions
def create_subscription(user, plan_id) do customer = ExStripe.Customer.create(%{ email: user.email, source: user.card_token }) ExStripe.Subscription.create(%{ customer: customer.id, items: [ %{plan: plan_id} ] }) end
In this example, we define a function create_subscription
that takes a user object and a plan ID. The function creates a customer in Stripe using the provided email and card token, and then creates a subscription for the customer with the specified plan.
These are just a few examples of what you can do with ExStripe. The library provides a comprehensive set of functions and options for interacting with the Stripe API. Refer to the ExStripe documentation for more details and examples.
Setting up Voice Functionalities in Phoenix Apps
Adding voice functionalities to Phoenix applications can enhance user experiences and enable interactive communication. In this section, we will explore how to set up voice functionalities in Phoenix apps using services like Twilio.
Related Article: Phoenix Design Patterns: Actor Model, Repositories, and Events
Using Twilio for Voice Functionalities in Phoenix Apps
Twilio is a cloud communications platform that provides APIs for building voice and messaging applications. By integrating Twilio with your Phoenix app, you can easily add voice functionalities such as making and receiving phone calls, sending and receiving SMS messages, and more.
To get started with Twilio in your Phoenix app, you need to add the ex_twilio
library as a dependency in your mix.exs
file:
defp deps do [ {:ex_twilio, "~> 0.8"} ] end
After adding the dependency, run mix deps.get
to fetch and compile the library.
Next, you need to configure ex_twilio
with your Twilio account credentials. In your Phoenix application’s config/config.exs
file, add the following configuration:
config :ex_twilio, account_sid: "YOUR_TWILIO_ACCOUNT_SID", auth_token: "YOUR_TWILIO_AUTH_TOKEN"
Replace YOUR_TWILIO_ACCOUNT_SID
and YOUR_TWILIO_AUTH_TOKEN
with your actual Twilio account credentials.
With ex_twilio
configured, you can now start using its features in your Phoenix application. Let’s take a look at a couple of examples to see how Twilio can be used to add voice functionalities.
Example 1: Making a Phone Call
def make_phone_call(to, from, url) do ExTwilio.Call.create( to: to, from: from, url: url ) end
In this example, we define a function make_phone_call
that takes a to
phone number, a from
phone number, and a URL. The function uses ExTwilio.Call.create
to initiate a phone call from the from
number to the to
number. The call will follow the instructions specified in the provided URL.
Example 2: Sending an SMS Message
def send_sms(to, from, body) do ExTwilio.Message.create( to: to, from: from, body: body ) end
In this example, we define a function send_sms
that takes a to
phone number, a from
phone number, and a message body. The function uses ExTwilio.Message.create
to send an SMS message from the from
number to the to
number with the specified body.
These examples demonstrate how easy it is to add voice functionalities to your Phoenix app using Twilio and the ex_twilio
library. Twilio provides a wide range of features and options for building voice and messaging applications. Refer to the ex_twilio
documentation for more details and examples.
Setting up SMS Functionalities in Phoenix Apps
Adding SMS functionalities to Phoenix applications can enable real-time communication and enhance user experiences. In this section, we will explore how to set up SMS functionalities in Phoenix apps using services like Twilio.
Using Twilio for SMS Functionalities in Phoenix Apps
Twilio, as mentioned earlier, is a cloud communications platform that provides APIs for building voice and messaging applications. By integrating Twilio with your Phoenix app, you can easily add SMS functionalities such as sending and receiving text messages.
To set up SMS functionalities in your Phoenix app using Twilio, you need to follow the same steps as mentioned in the previous section for voice functionalities. This includes adding the ex_twilio
library as a dependency and configuring it with your Twilio account credentials.
Once you have ex_twilio
configured, you can start using its features to send and receive SMS messages in your Phoenix application. Let’s take a look at a couple of examples.
Example 1: Sending an SMS Message
def send_sms(to, from, body) do ExTwilio.Message.create( to: to, from: from, body: body ) end
This example is the same as the one mentioned earlier in the voice functionalities section. The send_sms
function takes a to
phone number, a from
phone number, and a message body. It uses ExTwilio.Message.create
to send an SMS message from the from
number to the to
number with the specified body.
Example 2: Receiving an SMS Message
To receive SMS messages in your Phoenix app, you need to set up a webhook URL in your Twilio account. This URL will be called by Twilio whenever an SMS message is received. You can define a controller action in your Phoenix app to handle the incoming SMS messages.
defmodule MyApp.TwilioController do use MyApp.Web, :controller def create(conn, %{"From" => from, "Body" => body}) do # Handle the received SMS message IO.puts("Received SMS from #{from}: #{body}") # Return a valid TwiML response conn |> put_status(200) |> put_resp_header("content-type", "text/xml") |> send_resp(200, "") end end
In this example, we define a create
action in the MyApp.TwilioController
module. The action handles the incoming SMS message by printing the sender’s phone number and the message body to the console. It then returns a valid TwiML response to acknowledge the receipt of the message.
These examples demonstrate how you can set up SMS functionalities in your Phoenix app using Twilio and the ex_twilio
library. Twilio provides a comprehensive set of features for building SMS applications. Refer to the ex_twilio
documentation for more details and examples.
Related Article: Phoenix Core Advanced: Contexts, Plugs & Telemetry
Building Chatbots with Phoenix
Chatbots have become increasingly popular in recent years, as they provide a convenient and efficient way for businesses to interact with their customers. Phoenix, with its real-time capabilities and Elixir’s concurrency model, is a great platform for building chatbots. In this section, we will explore how to build chatbots with Phoenix.
Building a chatbot with Phoenix involves several components:
1. User Interface: The user interface is responsible for displaying the chat interface and capturing user input. This can be implemented using HTML, CSS, and JavaScript.
2. Chatbot Engine: The chatbot engine is responsible for processing user input, generating appropriate responses, and maintaining the conversation state. This can be implemented using Elixir’s pattern matching capabilities and the Phoenix framework.
3. Integration with Messaging Platforms: To make the chatbot accessible to users, it needs to be integrated with messaging platforms like Facebook Messenger, Slack, or Telegram. These platforms provide APIs for sending and receiving messages.
Let’s take a look at an example of how to build a simple chatbot with Phoenix.
Example: Building a Simple Chatbot
To build a simple chatbot with Phoenix, we can start by creating a new Phoenix project:
$ mix phx.new chatbot
Next, we need to set up the user interface for the chatbot. This typically involves creating an HTML page with a form for capturing user input and displaying the chat conversation. You can use HTML, CSS, and JavaScript to implement the user interface according to your requirements.
Once the user interface is set up, we can move on to implementing the chatbot engine. In Phoenix, we can define a controller action to handle the user input and generate responses. Here’s an example of how the controller action can be implemented:
defmodule ChatbotWeb.ChatController do use ChatbotWeb, :controller def index(conn, _params) do render(conn, "index.html") end def handle_message(conn, %{"message" => message}) do response = generate_response(message) render(conn, "response.json", response: response) end defp generate_response(message) do # Process the user message and generate a response # This can involve pattern matching, calling external APIs, or other logic case message do "Hello" -> "Hi there!" "How are you?" -> "I'm good, thank you!" _ -> "I'm sorry, I don't understand." end end end
In this example, the index
action renders the HTML page with the chat interface. The handle_message
action handles the user input by calling the generate_response
function, which processes the message and generates an appropriate response.
To integrate the chatbot with a messaging platform, you need to set up a webhook URL that the platform can use to send user messages. You can use the handle_message
action as the endpoint for receiving messages. The exact setup depends on the messaging platform you are using, so refer to the documentation of the specific platform for instructions.
This is just a basic example of building a chatbot with Phoenix. Depending on your requirements, you can add more advanced features such as natural language processing, external API calls, or integration with databases.
Creating Other Voice Interfaces with Phoenix
In addition to traditional phone calls, Phoenix can also be used to create other voice interfaces such as interactive voice response (IVR) systems, voice-controlled applications, and voice assistants. These voice interfaces can provide users with a hands-free and convenient way to interact with applications. In this section, we will explore how to create other voice interfaces with Phoenix.
Creating voice interfaces with Phoenix involves several components:
1. Speech Recognition: The speech recognition component is responsible for converting spoken words into text. This can be achieved using speech recognition APIs provided by platforms like Google Cloud Speech-to-Text or Microsoft Azure Speech Services.
2. Natural Language Understanding: The natural language understanding component is responsible for extracting meaning and intent from the user’s input. This can involve techniques such as natural language processing (NLP) and machine learning (ML).
3. Voice Response: The voice response component is responsible for generating a voice response based on the user’s input and the application’s logic. This can involve text-to-speech synthesis using platforms like Google Cloud Text-to-Speech or Amazon Polly.
Let’s take a look at an example of how to create a simple voice interface with Phoenix.
Related Article: Optimizing Database Queries with Elixir & Phoenix
Example: Creating a Simple Voice Interface
To create a simple voice interface with Phoenix, we can start by setting up the speech recognition component. There are several speech recognition APIs available, such as Google Cloud Speech-to-Text and Microsoft Azure Speech Services. Choose the one that best fits your requirements and follow the documentation to set it up.
Once the speech recognition component is set up, we can move on to implementing the natural language understanding component. This can involve using NLP libraries like Natural or ML frameworks like TensorFlow to extract meaning and intent from the user’s input. The exact implementation depends on the specific library or framework you choose.
Finally, we need to implement the voice response component. This involves generating a voice response based on the user’s input and the application’s logic. There are platforms like Google Cloud Text-to-Speech and Amazon Polly that provide APIs for text-to-speech synthesis. Choose the one that best fits your requirements and follow the documentation to set it up.
With the components in place, we can now create a Phoenix controller to handle the voice interface. Here’s an example of how the controller action can be implemented:
defmodule VoiceInterfaceWeb.VoiceController do use VoiceInterfaceWeb, :controller def handle_voice(conn, %{"audio" => audio}) do text = recognize_speech(audio) intent = extract_intent(text) response = generate_response(intent) audio_response = synthesize_speech(response) render(conn, "response.json", audio_response: audio_response) end defp recognize_speech(audio) do # Use the speech recognition API to convert audio to text # This can involve making an HTTP request to the API endpoint # and processing the response # Refer to the documentation of the specific API for instructions # and code examples end defp extract_intent(text) do # Use NLP or ML techniques to extract intent from the text # This can involve pattern matching, classification models, or other logic end defp generate_response(intent) do # Generate a response based on the intent and the application's logic # This can involve calling external APIs, accessing databases, or other logic end defp synthesize_speech(text) do # Use the text-to-speech synthesis API to convert text to audio # This can involve making an HTTP request to the API endpoint # and processing the response # Refer to the documentation of the specific API for instructions # and code examples end end
In this example, the handle_voice
action takes an audio input, recognizes the speech using the speech recognition component, extracts the intent using the natural language understanding component, generates a response based on the intent and the application’s logic, and finally synthesizes the response into audio using the text-to-speech synthesis component.
Again, this is just a basic example of creating a voice interface with Phoenix. Depending on your requirements, you can add more advanced features such as context-aware conversations, multi-language support, or integration with other APIs.
Popular Payment Platforms for Phoenix Integration
Phoenix provides the flexibility to integrate with various payment platforms, giving developers the freedom to choose the best option for their specific needs. While Stripe is a popular choice, there are other payment platforms that offer similar features and functionalities. In this section, we will explore some popular payment platforms for Phoenix integration.
1. Stripe
Stripe is a leading payment platform that provides a suite of APIs for accepting payments, managing subscriptions, and handling other payment-related tasks. It offers a developer-friendly interface and supports a wide range of payment methods, currencies, and business models. Integrating Phoenix with Stripe using libraries like ExStripe simplifies the process of implementing payment functionalities in your applications.
Related Article: Internationalization & Encoding in Elixir Phoenix
2. PayPal
PayPal is another popular payment platform that enables businesses to accept online payments securely. It offers a range of payment options, including credit cards, debit cards, and PayPal accounts. Phoenix can be integrated with PayPal using libraries like ExPaypal, which provide an abstraction layer for interacting with the PayPal API.
3. Braintree
Braintree, a subsidiary of PayPal, is a payment platform that focuses on providing smooth and seamless payment experiences. It offers a range of payment solutions, including credit cards, PayPal, and digital wallets like Apple Pay and Google Pay. Phoenix can be integrated with Braintree using libraries like ExBraintree, which provide a convenient way to interact with the Braintree API.
4. Square
Square is a popular payment platform that provides solutions for accepting payments in-person, online, and on mobile devices. It offers a range of payment options, including credit cards, debit cards, and digital wallets. Phoenix can be integrated with Square using libraries like ExSquare, which simplify the process of interacting with the Square API.
These are just a few examples of popular payment platforms for Phoenix integration. Depending on your specific requirements, you may choose other platforms that offer features and functionalities that align with your business needs.
Related Article: Implementing Enterprise Features with Phoenix & Elixir
Additional Resources
– Setting Up Voice Functionality in Phoenix Apps with Twilio
– Enabling SMS Functionality in Phoenix Apps with Twilio