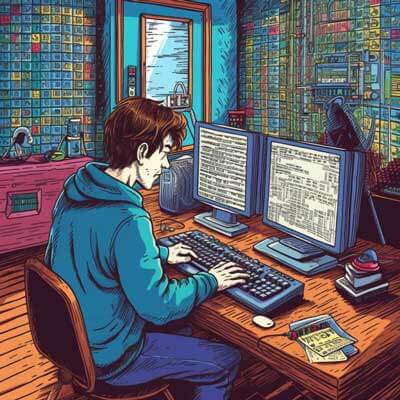
- What are database interactions and why are they important in Elixir?
- How does Ecto optimize database interactions in Elixir?
- What are some common query optimization techniques in Elixir?
- How does connection pooling improve performance in Elixir applications?
- What is the role of transactions in Elixir databases?
- How can load testing help identify performance bottlenecks in Phoenix applications?
- What tools can be used for profiling Phoenix applications in Elixir?
What are database interactions and why are they important in Elixir?
Database interactions are a critical part of most applications, as they allow the application to store and retrieve data. Efficient and optimized database interactions are important for several reasons:
1. Performance: Slow database interactions can significantly impact the performance of an application. If database queries take a long time to execute, it can lead to slow response times and a poor user experience. Optimizing database interactions can help improve the overall performance of an application.
2. Scalability: As an application grows in size and complexity, the number of database interactions also tends to increase. In order to handle a large number of concurrent users and requests, it is important to optimize database interactions to minimize bottlenecks and ensure scalability.
3. Consistency: Databases often enforce data consistency and integrity constraints, such as unique key constraints or foreign key relationships. Properly managing database interactions ensures that these constraints are maintained, preventing data corruption and ensuring the correctness of the application.
4. Security: Database interactions can also have security implications. By properly managing database interactions, such as using prepared statements or parameterized queries, it is possible to prevent common security vulnerabilities, such as SQL injection attacks.
Related Article: Integrating Phoenix Web Apps with Payment, Voice & Text
How does Ecto optimize database interactions in Elixir?
Ecto is a database wrapper and query language for Elixir that provides a set of abstractions and tools for working with databases. Ecto is designed to optimize database interactions in several ways:
1. Query composition: Ecto allows developers to build database queries using a functional and composable syntax. This allows for the construction of complex queries in a clear and concise manner. Ecto’s query composition also enables query reuse and abstraction, reducing the amount of duplicated code and improving maintainability.
2. Query optimization: Ecto’s query engine optimizes database queries by generating efficient SQL code. It performs query optimizations such as predicate pushdown, query rewriting, and intelligent join ordering. These optimizations help minimize the amount of data transferred between the application and the database, resulting in improved performance.
3. Connection pooling: Ecto provides connection pooling, which allows for efficient management and reuse of database connections. Connection pooling eliminates the overhead of establishing a new database connection for each query, improving performance and scalability. Ecto’s connection pooling also includes features such as connection monitoring and automatic reconnection, ensuring the reliability of the database connections.
4. Transaction management: Ecto provides a transaction API that simplifies the management of database transactions. Transactions allow for atomic and isolated database operations, ensuring data integrity and consistency. Ecto’s transaction management includes support for nested transactions, savepoints, and transaction retrying, providing flexibility and robustness in handling database interactions.
What are some common query optimization techniques in Elixir?
Optimizing database queries is crucial for improving the performance of Elixir applications. Here are some common query optimization techniques in Elixir:
1. Selecting the right indexes: Indexes are data structures that allow for efficient data retrieval based on specific columns. Choosing the right indexes for your queries can significantly improve query performance. You should analyze your query patterns and create indexes on columns that are frequently used for filtering or sorting.
Example:
# Creating an index on the "name" column of the "users" table create index(:users, [:name])
2. Limiting the number of rows returned: If your query only needs a subset of the data, you can use the limit
clause to restrict the number of rows returned. This can help reduce the amount of data transferred between the database and the application, improving performance.
Example:
# Query to retrieve the top 10 highest-rated movies Repo.all(from m in Movie, order_by: [desc: :rating], limit: 10)
3. Eager loading associations: When querying for records that have associations, Ecto’s preload
or join
functions can be used to eagerly load the associated data. This avoids the N+1 query problem, where each record triggers an additional query to fetch its associated data.
Example:
# Eager loading the comments for a post Repo.get(Post, post_id) |> Repo.preload(:comments)
4. Using database-specific functions and features: Different databases provide various functions and features that can optimize specific types of queries. Knowing the capabilities of your database and utilizing them appropriately can lead to significant performance improvements.
Example:
# Using PostgreSQL's full-text search capabilities Repo.all(from p in Product, where: fragment("to_tsvector('english', ?) @@ plainto_tsquery('english', ?)", p.name, "search term"))
5. Avoiding unnecessary data retrieval: Only retrieve the columns that are required for your query. Avoid selecting all columns using the select
function, as it can result in unnecessary data transfer and slower query execution.
Example:
# Selecting only the "name" and "email" columns of users Repo.all(from u in User, select: {u.name, u.email})
How does connection pooling improve performance in Elixir applications?
Connection pooling is a technique used to manage and reuse database connections in a more efficient way. It involves creating a pool of pre-established database connections that can be reused across multiple requests or processes instead of establishing a new connection for each request.
Connection pooling improves performance in Elixir applications in the following ways:
1. Reduced connection overhead: Establishing a new connection to a database can be an expensive operation in terms of time and resources. By reusing existing connections from the pool, the overhead of establishing a new connection for each request is eliminated, resulting in faster response times.
2. Increased scalability: Connection pooling allows for better utilization of database resources and improves the scalability of an application. With connection pooling, a limited number of connections can serve multiple requests concurrently, reducing the likelihood of exhausting the available resources and improving the overall throughput of the application.
3. Connection reuse: Reusing existing connections from the pool eliminates the need to negotiate the connection parameters and authentication with the database server for each request. This reduces the network roundtrip time and improves the overall efficiency of the application.
Ecto provides built-in connection pooling support through its database adapter. By configuring the pool size and other related parameters, developers can optimize the connection pooling behavior to match the specific requirements of their application.
Related Article: Phoenix Design Patterns: Actor Model, Repositories, and Events
What is the role of transactions in Elixir databases?
Transactions are a fundamental concept in database systems that allow for the atomic and isolated execution of multiple database operations. In Elixir databases, transactions play a crucial role in ensuring data integrity and consistency.
The key role of transactions in Elixir databases include:
1. Atomicity: Transactions ensure that a group of database operations either succeed as a whole or fail as a whole. This means that if any part of the transaction fails, all changes made within the transaction are rolled back, leaving the database in its original state. Atomicity guarantees that the database remains in a consistent state despite failures or errors during the transaction.
2. Isolation: Transactions provide isolation by ensuring that the changes made within a transaction are not visible to other concurrent transactions until the transaction is committed. This prevents conflicts and ensures that each transaction sees a consistent, isolated snapshot of the database. Isolation prevents issues such as dirty reads, non-repeatable reads, and phantom reads.
3. Consistency: Transactions help maintain data consistency by enforcing integrity constraints defined in the database schema. These constraints can include unique key constraints, foreign key relationships, and other business rules. By performing database operations within a transaction, developers can ensure that these constraints are maintained and that the data remains consistent.
4. Durability: Transactions provide durability by ensuring that once a transaction is committed, its changes are permanent and will survive system failures. This is achieved by ensuring that the changes made within a transaction are durably stored in the database’s storage medium, such as disk or solid-state drive. Durability guarantees that the data remains intact and recoverable in the event of a crash or hardware failure.
Ecto provides a transaction API that simplifies the management of database transactions in Elixir applications. Developers can use the Ecto.Multi
module to define a set of database operations that should be executed within a transaction. If any part of the transaction fails, the entire transaction is rolled back, ensuring the atomicity and consistency of the database operations.
How can load testing help identify performance bottlenecks in Phoenix applications?
Load testing is a technique used to simulate real-world user traffic and measure the performance of an application under different load conditions. Load testing can help identify performance bottlenecks in Phoenix applications by putting the application through a series of stress tests and analyzing its behavior under high load.
Here are some ways load testing can help identify performance bottlenecks in Phoenix applications:
1. Stress testing: Load testing can simulate a high number of concurrent users and requests to stress the application. By gradually increasing the load and monitoring the application’s response time, developers can identify the point at which the application starts to degrade in performance. This can help identify areas of the application that are not properly optimized or that have scalability issues.
2. Scalability analysis: Load testing can provide insights into the scalability of a Phoenix application. By analyzing the application’s response time and throughput under different load conditions, developers can determine if the application scales linearly with the number of concurrent users or if there are bottlenecks that limit its scalability. This information can guide optimizations and infrastructure planning.
3. Resource utilization analysis: Load testing can help identify resource utilization patterns of a Phoenix application under different load conditions. By monitoring system metrics such as CPU usage, memory consumption, and database connection usage, developers can identify resource-intensive operations or bottlenecks that impact the application’s performance. This information can guide optimizations and infrastructure provisioning.
4. Performance profiling: Load testing can be combined with performance profiling tools to analyze the performance of specific components or functions within a Phoenix application. By profiling the application during load tests, developers can identify hotspots, slow database queries, or inefficient code paths that contribute to performance bottlenecks. This information can guide code optimizations and architectural improvements.
To perform load testing on a Phoenix application, developers can use tools such as Apache JMeter, Gatling, or Locust. These tools allow for the simulation of concurrent user traffic and provide detailed reports and metrics that can be used to analyze the application’s performance under different load conditions.
What tools can be used for profiling Phoenix applications in Elixir?
Profiling is the process of measuring and analyzing the performance characteristics of a software application. Profiling can help identify performance bottlenecks, memory leaks, and other issues that affect the application’s performance. In the context of Phoenix applications in Elixir, there are several tools available for profiling and performance analysis.
Here are some tools that can be used for profiling Phoenix applications in Elixir:
1. Erlang/OTP Profiler: The Erlang/OTP Profiler is a built-in tool that comes with the Erlang/OTP distribution. It provides low-level profiling capabilities for Elixir and Erlang applications. The profiler can be used to measure CPU usage, memory usage, and function call statistics. By analyzing the profiler’s output, developers can identify performance bottlenecks and optimize critical sections of the code.
Example usage:
:eprof.start() :eprof.run(:my_module, :my_function, []) :eprof.stop()
2. Elixir’s :timer.tc/1
: Elixir’s :timer.tc/1
function can be used to measure the execution time of a specific block of code. It returns the time taken in microseconds and can be used to profile specific functions or sections of code.
Example usage:
{time, _result} = :timer.tc(fn -> my_function() end) IO.puts("Execution time: #{time} microseconds")
3. Erlang’s fprof
: fprof
is a profiling tool that comes with the Erlang/OTP distribution. It provides detailed function call statistics, memory usage, and execution time information. fprof
can be used to profile specific modules or functions and generates reports that can be analyzed to identify performance bottlenecks.
Example usage:
fprof:start() fprof:apply(my_module, my_function, []) fprof:stop() fprof:analyse()
4. Phoenix.LiveDashboard: Phoenix.LiveDashboard is a Phoenix package that provides a real-time dashboard for monitoring and profiling Phoenix applications. It includes features such as request metrics, process information, database queries, and live code introspection. Developers can use Phoenix.LiveDashboard to monitor the performance of their application in real-time and identify bottlenecks.
To use Phoenix.LiveDashboard, you need to add it as a dependency in your mix.exs file and configure it in your application’s endpoint module. Once configured, you can access the dashboard by visiting the specified URL in your web browser.
# mix.exs defp deps do [ {:phoenix_live_dashboard, "~> 0.5", only: :dev} ] end # lib/my_app/endpoint.ex defmodule MyApp.Endpoint do use Phoenix.Endpoint, otp_app: :my_app if Mix.env() == :dev do plug Phoenix.LiveDashboard end # ... end
These tools provide valuable insights into the performance characteristics of Phoenix applications in Elixir. By profiling and analyzing the application’s performance, developers can identify bottlenecks and areas for optimization, leading to improved application performance and user experience.