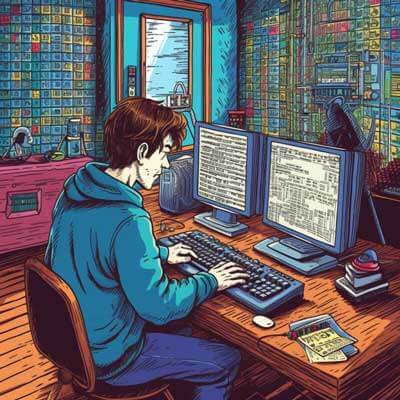
Table of Contents
Overview of Unsupported Engine Issues
Unsupported engine issues frequently arise in the world of Node.js and npm (Node Package Manager). These issues occur when the version of Node.js being used does not meet the engine requirements specified by a package. This can lead to warnings or errors during installation or execution of packages. Understanding how to navigate these issues is crucial for developers to maintain a smooth workflow and ensure compatibility across dependencies.
Related Article: How to Fix npm Self Signed Certificate Error
What Does Unsupported Engine Mean
The term "unsupported engine" refers to the version of Node.js or npm that is not compatible with the package being installed. Each package can specify which versions of Node.js it supports through the "engines" field in its package.json file. If the running version of Node.js does not satisfy these specifications, npm will issue a warning or error. This warning is meant to inform developers that they might be using a version of Node.js that could lead to unexpected behavior or incompatibilities with the package.
Common Causes of Unsupported Engine Errors
Several scenarios can lead to unsupported engine errors. These include:
1. Outdated Node.js Version: Developers may be using an older version of Node.js when a package requires a newer version.
2. Package Updates: When a package is updated, it may introduce new engine requirements that were not present in previous versions.
3. Multiple Node.js Versions: Using version managers like nvm (Node Version Manager) can sometimes lead to confusion about which version is currently active, resulting in unsupported engine issues.
4. Development Environment: Different developers on a team might be using different versions of Node.js, leading to compatibility issues.
Each of these scenarios highlights the importance of keeping track of Node.js versions and package requirements.
Engine Requirements in package.json
The engine requirements for a package are specified in the package.json file using the "engines" field. This field allows package authors to define the versions of Node.js that their package is compatible with. Here is an example of how this looks in a package.json file:
{ "name": "example-package", "version": "1.0.0", "engines": { "node": ">=14.0.0 <15.0.0" }}
In this example, the package requires Node.js version 14.x.x but does not support version 15 or higher. When npm tries to install this package, it checks the current version of Node.js against these requirements. If the version does not match, a warning will be displayed.
Related Article: How to Track the History of npm Packages
How npm Handles Unsupported Engine Versions
When npm encounters an unsupported engine version, it does not prevent the installation of the package by default. Instead, it logs a warning message to inform the developer of the potential issue. This behavior allows developers to make informed decisions about whether to proceed with using a package that may not be fully compatible with their current Node.js version.
For example, a warning might look like this:
npm WARN example-package@1.0.0: The engine "node" is incompatible with this module. Expected version ">=14.0.0 <15.0.0".
This warning serves as a notification that while the package may still install, it might not work as intended.
Specifying Node.js Version Requirements
Setting specific Node.js version requirements in a project helps ensure that all developers on a team are using compatible versions. This can be achieved by defining the required version in the package.json file or by using a tool like .nvmrc for Node Version Manager (nvm).
To specify the version in package.json, you can include the "engines" field as shown earlier. Alternatively, creating a .nvmrc file in the root of your project can specify the Node.js version:
14.17.0
When team members use nvm, they can run nvm use
in the project directory to switch to the specified version. This practice helps maintain consistency across development environments.
Checking Node.js Version for Compatibility
Verifying the current version of Node.js is a simple yet crucial step in ensuring compatibility. This can be done using the following command in the terminal:
node -v
This command will return the currently installed version of Node.js. Developers should compare this version against the engine requirements specified in the package.json files of their dependencies to determine compatibility.
For example, if a developer sees that their Node.js version is 12.x.x and the package requires 14.x.x, they need to upgrade their Node.js version to avoid potential issues.
Resolving Unsupported Engine Warnings
Addressing unsupported engine warnings can be handled in several ways:
1. Upgrade Node.js: The simplest solution is to update Node.js to a version that satisfies the requirements of the packages being used. This can be done through nvm or by downloading the latest version directly from the Node.js website.
2. Downgrade Packages: If upgrading Node.js is not feasible, consider downgrading the package to a version that supports the current Node.js version. This can be achieved by specifying the version during installation:
npm install example-package@1.0.0
3. Forking or Modifying Packages: As a last resort, developers may fork the package repository and modify the package.json to adjust the engine requirements. This is not generally recommended due to potential issues with package maintenance and updates.
Choosing the right approach depends on the context of the project and the specific needs of the development team.
Related Article: How to Fix npm Warn Ebadengine Unsupported Engine
Implications of Using Unsupported Engine Packages
Using packages with unsupported engine versions can lead to various issues, including:
- Runtime Errors: Some features may not be available or may behave differently, leading to unexpected errors during execution.
- Lack of Support: If a package was designed for a specific Node.js version, using it with an unsupported version may mean that the package's maintainers cannot provide support for the issues that arise.
- Security Vulnerabilities: Older versions of Node.js may not receive security updates, potentially exposing applications to vulnerabilities.
Understanding these implications helps developers make informed choices about which packages to use and under what circumstances.
Bypassing Unsupported Engine Checks
Bypassing engine checks can be done, but it should be approached with caution. Developers can use the --engine-strict flag when installing packages. Setting this flag to false allows the installation to proceed even if engine checks fail:
npm install --engine-strict=false
However, using this method can lead to the issues discussed in the previous section. It is generally better to resolve compatibility issues rather than ignore them.
Managing Peer Dependencies
Peer dependencies are another aspect of package management that often leads to confusion and compatibility issues. A peer dependency is a package that is required by another package, but it expects the host application to provide it. This is common in plugin architectures.
To specify a peer dependency in a package, the following syntax is used in the package.json file:
{ "peerDependencies": { "example-plugin": "^2.0.0" }}
When a developer installs a package with peer dependencies, npm will warn them if the required peer dependencies are not installed at the correct versions. To install peer dependencies, the developer must explicitly install them:
npm install example-plugin@^2.0.0
This ensures that all required plugins or extensions are compatible with the main package being used.
Best Practices for Dependency Management
To maintain a robust and efficient development environment, several best practices for dependency management should be followed:
1. Regularly Update Packages: Keeping packages and Node.js up to date helps avoid unsupported engine issues and security vulnerabilities.
2. Use Lock Files: Using package-lock.json or yarn.lock files ensures that all team members use the same versions of dependencies, reducing compatibility issues.
3. Review Engine Requirements: Before installing new packages, review their engine requirements to avoid unsupported engine warnings.
4. Adopt Version Managers: Tools like nvm help manage multiple Node.js versions on a development machine, allowing developers to switch easily between versions based on project needs.
5. Document Dependency Requirements: Clearly document the required Node.js version and any other relevant setup instructions in the project README. This practice aids new developers in quickly setting up the project.