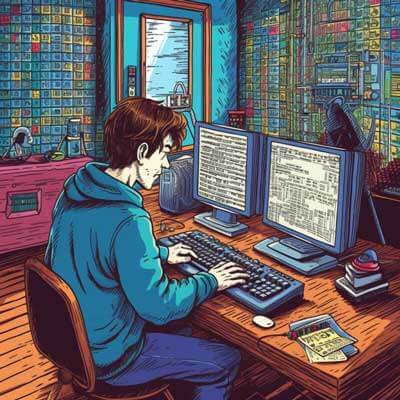
Table of Contents
Overview of Tiny Invariant
Tiny Invariant is a minimalistic JavaScript library designed to enforce runtime assertions. It is particularly useful for ensuring that certain conditions hold true during code execution. When an invariant is not met, it throws an error, which helps identify issues early in the development process. This library is lightweight, focusing solely on assertion checks without any additional overhead.
Related Article: How to use a Next.js performance analyzer library
Installing Tiny Invariant
Installing Tiny Invariant is a simple process. You can add it to your project using npm, which is the package manager for JavaScript. To install, run the following command in your terminal:
npm install tiny-invariant
After installation, you can verify that Tiny Invariant is included in your project by checking your package.json
file. It should appear under the dependencies section.
Purpose of Tiny Invariant
The primary purpose of Tiny Invariant is to assert conditions that must be true at a specific point in your code. These assertions help catch bugs early by throwing errors when expectations are not met. For example, if you expect a variable to be defined before proceeding with an operation, Tiny Invariant can confirm that this condition holds true. If the condition is false, an error is thrown, indicating a problem in the code logic.
Using Tiny Invariant in Projects
Using Tiny Invariant in your project is straightforward. You begin by importing it into your JavaScript or TypeScript file. Here’s how to do that:
// Importing Tiny Invariant import invariant from 'tiny-invariant'; // Example usage const user = null; // This will throw an error since user is null invariant(user, 'User must be defined');
In this example, the invariant checks whether the user
variable is truthy. If it is not, an error is raised with the message 'User must be defined'. This assertion helps prevent potential errors in subsequent code that relies on the user
variable.
Related Article: How to Use npm with Next.js
Benefits of Tiny Invariant
The benefits of using Tiny Invariant include its simplicity and minimalism. As a lightweight library, it does not introduce significant overhead to your application. This means faster load times and better performance. Additionally, Tiny Invariant provides clear error messages, making it easier to debug issues when assertions fail. The library also promotes good coding practices by encouraging developers to think critically about their assumptions and conditions.
Tiny Invariant with TypeScript
Integrating Tiny Invariant with TypeScript is seamless. TypeScript enhances the development experience by providing type safety. When using Tiny Invariant in a TypeScript project, the assertions remain the same, but you can benefit from TypeScript's type checking.
Here's an example of using Tiny Invariant in a TypeScript file:
// Importing Tiny Invariant import invariant from 'tiny-invariant'; interface User { name: string; age: number; } const getUser = (user: User | null) => { // This will throw an error if user is null invariant(user, 'User must be defined'); console.log(user.name); }; // Example usage getUser(null); // This will throw an error
In this TypeScript example, an interface defines the shape of a User object. The getUser
function checks if the user is null before proceeding. If null, an error is thrown, helping to maintain the expected structure of the code.
Common Use Cases for Tiny Invariant
Common use cases for Tiny Invariant include validating inputs, API responses, and function parameters. For example, when developing a function that processes user input, you can assert that the input meets certain criteria.
Here's an example of validating an API response:
import invariant from 'tiny-invariant'; const fetchData = async () => { const response = await fetch('https://api.example.com/data'); const data = await response.json(); // Assert that data is an array invariant(Array.isArray(data), 'Expected data to be an array'); console.log(data); };
In this example, after fetching data from an API, an invariant checks that the data is an array. If it's not, an error is thrown, making it easier to handle unexpected responses.
Comparison with Other Assertion Libraries
When comparing Tiny Invariant to other assertion libraries, several factors come into play. Libraries like assert
from Node.js or chai
offer more extensive functionality, including diverse assertion types. However, Tiny Invariant stands out due to its simplicity and size. It focuses solely on invariant checks, making it a better choice for projects that require lightweight solutions without additional complexity.
For instance, while an assertion library might provide multiple assertion methods (equal, deep equal, etc.), Tiny Invariant is designed to check a single condition and throw an error if that condition is not met. This can lead to cleaner and more focused code.
Related Article: How to Compare Rust Deku and npm
Lightweight Nature of Tiny Invariant
The lightweight nature of Tiny Invariant is a significant advantage. The library is designed to have a minimal footprint, making it easy to include in projects. Its size is a mere few kilobytes, which is negligible compared to bulkier libraries. This aspect is crucial for performance-sensitive applications where every byte counts, especially in web development.
The quick load time results in faster application performance, enhancing the user experience. By reducing unnecessary bloat, developers can maintain cleaner codebases while ensuring robust error handling.
Choosing the Right Version of Tiny Invariant
When selecting the right version of Tiny Invariant for your project, consider compatibility with your existing codebase and dependencies. Ensure that the version aligns with the version of Node.js or the browser environment you are targeting. Always refer to the official documentation for breaking changes or updates.
To check the latest version, run the following command:
npm view tiny-invariant version
This command will display the most current version of Tiny Invariant available on npm. Regularly updating to the latest version can provide new features and improvements, but it's essential to test your application after updates to avoid compatibility issues.