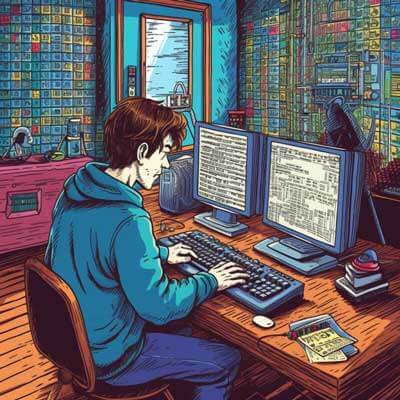
Table of Contents
Overview of Luxon
Luxon is a modern JavaScript library designed for working with dates and times. It provides a clean and simple API for manipulating and formatting date and time values. Luxon is built on top of the native JavaScript Date object, enhancing its capabilities and addressing many of the shortcomings present in earlier libraries like Moment.js. It is especially useful for handling complex date and time operations, including time zones, durations, and intervals.
One of the main advantages of Luxon is its immutability. Once a DateTime object is created, it cannot be changed. This eliminates many common bugs associated with mutable date objects and makes it easier to reason about code. Luxon also supports Internationalization (i18n) and is designed with modern JavaScript standards in mind, making it a suitable choice for both client-side and server-side applications.
Related Article: How To Use Npm Patch Package
Installing Luxon with npm
To use Luxon in a project, it must first be installed via npm (Node Package Manager). This can be done by running the following command in the terminal:
npm install luxon
Once installed, Luxon can be imported into JavaScript files. For example, to use Luxon in a JavaScript file, you can include it as follows:
// Importing the Luxon library import { DateTime } from 'luxon';
This allows access to all the functionalities provided by Luxon, enabling developers to manipulate and format dates easily.
Features of Luxon
Luxon includes several key features designed to enhance date and time handling. These features include:
1. DateTime Objects: Creation and manipulation of date and time objects.
2. Formatting: Customizable date and time formatting.
3. Parsing: Converting strings into DateTime objects.
4. Time Zone Handling: Support for various time zones and daylight saving time.
5. Duration and Interval: Managing time durations and intervals effectively.
6. Internationalization: Support for different locales and languages.
These features address the common challenges developers face when working with dates and times in JavaScript applications.
Creating DateTime Objects
Creating DateTime objects in Luxon is simple and straightforward. The DateTime
class provides several methods for instantiation. The most common method is DateTime.now()
, which returns the current date and time.
// Getting the current date and time const now = DateTime.now(); console.log(now.toString());
Luxon also allows creating DateTime objects from specific date and time values using the fromObject
method. For example:
// Creating a DateTime object from specific values const specificDate = DateTime.fromObject({ year: 2023, month: 10, day: 1 }); console.log(specificDate.toString());
Additionally, DateTime can be created from ISO 8601 formatted strings:
// Creating a DateTime object from an ISO string const isoDate = DateTime.fromISO('2023-10-01T10:00:00'); console.log(isoDate.toString());
These methods provide flexibility in creating DateTime objects based on various use cases.
Related Article: How to Use Force and Legacy Peer Deps in Npm
Formatting Dates
Formatting dates is a crucial aspect of working with DateTime objects. Luxon offers a useful formatting capability through the toFormat
method. This method allows developers to specify a format string that determines how the date will be displayed.
For example, to format a date as "YYYY-MM-DD":
// Formatting the current date const formattedDate = DateTime.now().toFormat('yyyy-MM-dd'); console.log(formattedDate); // Output: 2023-10-01
Luxon supports a wide range of formatting tokens, enabling various output styles. For instance, to display a more human-readable format:
// Formatting a DateTime object to a more readable format const readableDate = DateTime.now().toFormat('MMMM dd, yyyy'); console.log(readableDate); // Output: October 01, 2023
This flexibility allows developers to present dates in a way that suits their applications and user preferences.
Parsing Dates
Parsing dates from strings into DateTime objects is another essential feature of Luxon. The library provides several methods to convert date strings into DateTime instances. The most common method is fromISO
, which parses ISO 8601 formatted strings:
// Parsing an ISO string to a DateTime object const parsedDate = DateTime.fromISO('2023-10-01T10:00:00'); console.log(parsedDate.toString());
Luxon also provides the fromFormat
method, which allows parsing with custom formats. For example:
// Parsing a date string with a custom format const customDate = DateTime.fromFormat('01-10-2023', 'dd-MM-yyyy'); console.log(customDate.toString());
This capability is beneficial for handling various date string formats that may be encountered in real-world applications.
Handling Timezones
Time zone handling is often a complex task in date and time management. Luxon simplifies this process by providing robust support for time zones. Each DateTime object maintains its time zone information, which can be manipulated as needed.
To create a DateTime object in a specific time zone, the setZone
method can be used:
// Creating a DateTime object in a specific time zone const zonedDate = DateTime.now().setZone('America/New_York'); console.log(zonedDate.toString());
Converting between time zones is also straightforward. For example, if you want to convert a date from one time zone to another:
// Converting a DateTime object to a different time zone const newZoneDate = zonedDate.setZone('Europe/London'); console.log(newZoneDate.toString());
This ability to manage time zones is crucial for applications that require accurate date and time representation across different regions.
Working with Durations
Luxon provides a dedicated Duration
class for managing time durations. This class can represent a span of time, such as hours, minutes, and seconds. Creating a Duration object can be done using the Duration.fromObject
method:
// Creating a Duration object const duration = Duration.fromObject({ hours: 1, minutes: 30 }); console.log(duration.toString());
Luxon allows performing arithmetic on durations, enabling addition or subtraction of time:
// Adding durations const additionalDuration = Duration.fromObject({ minutes: 15 }); const totalDuration = duration.plus(additionalDuration); console.log(totalDuration.toString()); // Output: PT1H45M
This functionality makes it easy to handle time intervals and perform calculations related to time durations.
Related Article: How to Fix npm err tracker idealtree already exists
Using Intervals
Intervals in Luxon represent a range of time between two DateTime objects. The Interval
class allows developers to create and manipulate these ranges effectively. To create an interval, the fromDateTimes
method is used:
// Creating an Interval from two DateTime objects const start = DateTime.now(); const end = start.plus({ days: 7 }); const interval = Interval.fromDateTimes(start, end); console.log(interval.toString());
Luxon also provides methods to check if a specific DateTime falls within an interval:
// Checking if a DateTime object is within an interval const dateToCheck = DateTime.now().plus({ days: 3 }); console.log(interval.contains(dateToCheck)); // Output: true
This capability is useful for scheduling and managing events within specific time frames.
Comparing Luxon and Moment.js
Luxon and Moment.js are often compared due to their similar use cases in date and time handling. While Moment.js has been widely used for years, Luxon offers several advantages:
1. Immutability: Luxon’s DateTime objects are immutable, reducing side effects and making code easier to understand.
2. Modern API: Luxon is designed with modern JavaScript in mind, leveraging ES6 features and providing a more consistent interface.
3. Time Zone Support: Luxon has built-in support for time zones and daylight saving time, simplifying common operations.
Moment.js is still a solid choice for many projects, but its large bundle size and mutable objects can lead to complexities. Luxon offers a lightweight alternative with a focus on immutability and a cleaner API.
Using Luxon with React
Integrating Luxon with React is simple and effective. The library can be imported into any React component, allowing date and time manipulation directly within the component lifecycle.
For example, a React component that displays the current date and time can be created as follows:
// Importing React and Luxon import React from 'react'; import { DateTime } from 'luxon'; const CurrentDateTime = () => { const now = DateTime.now(); return ( <div> <h1>Current Date and Time</h1> <p>{now.toFormat('MMMM dd, yyyy HH:mm:ss')}</p> </div> ); }; export default CurrentDateTime;
This component will render the current date and time each time it is rendered. Luxon’s capabilities can be applied within more complex applications, such as managing event schedules, displaying time zones, or formatting user inputs.