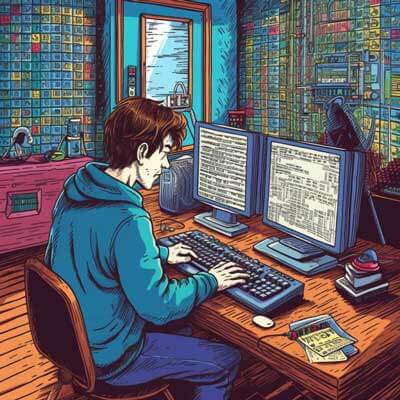
JavaScript is a single-threaded language, which means it executes code in a sequential manner. However, there are scenarios where you may want to introduce delays or pause the execution of your JavaScript code. In this article, we will explore various techniques to achieve this.
1. setTimeout()
One of the simplest ways to introduce a delay in JavaScript is by using the setTimeout()
function. This function allows you to execute a block of code after a specified amount of time has passed. Here’s the syntax:
setTimeout(function, delay, param1, param2, ...)
– function
: The function to be executed after the delay.
– delay
: The number of milliseconds to wait before executing the function.
– param1, param2, ...
: Optional parameters to be passed to the function.
Here’s an example that demonstrates how to use setTimeout()
:
console.log("Start"); setTimeout(function() { console.log("Delayed code"); }, 2000); console.log("End");
In this example, the console will output “Start”, “End”, and then “Delayed code” after a delay of 2000 milliseconds (2 seconds).
It’s important to note that setTimeout()
does not pause the execution of the entire script; it only delays the execution of the specified function. This means that other code outside the setTimeout()
function will continue to execute.
Related Article: How to Implement a Beating Heart Loader in Pure CSS
2. setInterval()
Another way to introduce delays in JavaScript is by using the setInterval()
function. Similar to setTimeout()
, setInterval()
allows you to execute a block of code repeatedly at a specified interval. Here’s the syntax:
setInterval(function, delay, param1, param2, ...)
– function
: The function to be executed at each interval.
– delay
: The number of milliseconds between each execution of the function.
– param1, param2, ...
: Optional parameters to be passed to the function.
Here’s an example that demonstrates how to use setInterval()
:
console.log("Start"); var intervalId = setInterval(function() { console.log("Interval code"); }, 1000); // Stop the interval after 5 seconds setTimeout(function() { clearInterval(intervalId); }, 5000); console.log("End");
In this example, the console will output “Start”, “End”, and then “Interval code” every 1000 milliseconds (1 second) until the interval is stopped after 5 seconds.
Similar to setTimeout()
, setInterval()
does not pause the execution of the entire script. Other code outside the setInterval()
function will continue to execute.
Why Pause Execution in JavaScript?
The need to pause the execution of JavaScript code can arise in various scenarios. Some potential reasons include:
1. Animation: Pausing the execution can be useful when creating animations or transitions, allowing for smoother and more controlled effects.
2. Delayed Actions: Pausing execution can be used to introduce delays between actions, ensuring that certain operations occur in a sequential manner.
3. Simulating Real-Time Behavior: In simulations or games, you may need to introduce pauses to simulate real-time behavior or create interactive experiences.
4. Asynchronous Operations: When working with asynchronous operations, such as fetching data from an API or performing I/O operations, pausing execution can help synchronize the flow of code.
Suggestions and Alternative Ideas
While setTimeout()
and setInterval()
are commonly used for introducing delays in JavaScript, there are some alternative approaches and suggestions to consider:
1. Promises and Async/Await: With the introduction of Promises and Async/Await in JavaScript, you can handle asynchronous operations in a more structured and readable manner. Instead of pausing the execution, consider using Promises and Async/Await to handle delays and asynchronous tasks.
2. Web Workers: If you need to perform heavy processing or time-consuming tasks without blocking the main thread, consider using Web Workers. Web Workers allow you to execute JavaScript code in the background, freeing up the main thread for other operations.
3. CSS Transitions and Animations: For simple animations and transitions, consider using CSS animations and transitions. CSS provides a wide range of properties and effects that can be used to create visually appealing animations without the need for pausing JavaScript execution.
Related Article: The very best software testing tools
Best Practices
When pausing execution in JavaScript, it’s important to keep a few best practices in mind:
1. Avoid Long Delays: Introducing long delays can negatively impact the user experience, making your application feel slow and unresponsive. Consider using shorter delays or asynchronous techniques to avoid blocking the main thread.
2. Use Callbacks or Promises: When working with delays caused by asynchronous operations, consider using callbacks or Promises to handle the flow of code. This promotes a more structured and readable codebase.
3. Be Mindful of Browser Compatibility: While setTimeout()
and setInterval()
are widely supported by modern browsers, it’s important to be mindful of browser compatibility when using other techniques or APIs. Always test your code across different browsers to ensure consistent behavior.
4. Optimize Animations: When creating animations, aim for smooth and performant effects. Use CSS transitions and animations whenever possible, as they are often hardware-accelerated and provide better performance compared to JavaScript-based animations.