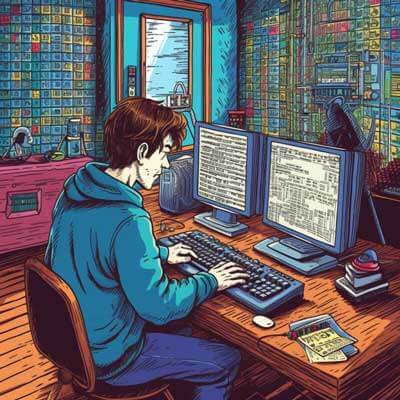
Table of Contents
The Concept of Buffering
In software development, buffering refers to the process of temporarily storing data in a memory buffer before it is processed or consumed. This technique is commonly used to optimize performance and improve efficiency in various applications, including web development.
Buffering allows for the efficient processing of data by minimizing the overhead associated with frequent read and write operations. It enables the application to work with larger chunks of data at once, reducing the number of I/O operations required.
In the context of ReactJS, buffering can be used to optimize the rendering and update process of components. By buffering the changes made to the component's state, ReactJS can batch and optimize the rendering process, resulting in improved performance.
Related Article: How Component Interaction Works in ReactJS
Example: Buffering in ReactJS
import React, { useState, useEffect } from 'react';const BufferingExample = () => { const [buffer, setBuffer] = useState([]); useEffect(() => { // Simulating data fetching or updates const fetchData = async () => { const data = await fetch('https://api.example.com/data'); const jsonData = await data.json(); setBuffer([...buffer, jsonData]); // Buffering changes to the state }; fetchData(); }, []); return ( <div> {buffer.map((data, index) => ( <div key={index}>{data}</div> ))} </div> );};export default BufferingExample;
In this example, we have a component called BufferingExample
that demonstrates the concept of buffering in ReactJS. The component maintains a buffer state using the useState
hook. On component mount, it fetches data from an API and buffers the changes to the state using the setBuffer
function.
The buffered data is then rendered within the component. By buffering the changes to the state and rendering them as a batch, ReactJS can optimize the rendering process and improve performance.
Buffering in ReactJS can be particularly useful in scenarios where frequent updates to the component's state occur, such as real-time data updates or user interactions. By buffering these changes, ReactJS can avoid unnecessary re-renders and optimize the rendering process.
Buffering vs. Streaming
While buffering and streaming are both techniques used in data processing, they serve different purposes and have distinct characteristics.
Buffering involves storing data in a buffer before it is processed or consumed. It aims to optimize performance by reducing the number of I/O operations and working with larger chunks of data at once. Buffering allows for efficient processing and can be particularly useful in scenarios where the data is produced or consumed at different rates.
Streaming, on the other hand, involves continuously sending or receiving data in a sequential manner. It allows for real-time or near-real-time data transmission, where the data is processed and consumed as it arrives. Streaming is commonly used in scenarios such as live video streaming, real-time communication, and event-driven architectures.
While buffering is suitable for scenarios where data can be processed in batches, streaming is more appropriate for situations where immediate processing and consumption of data are required. The choice between buffering and streaming depends on the specific use case and the requirements of the application.
Example: Buffering vs. Streaming in ReactJS
In ReactJS, the choice between buffering and streaming depends on the nature of the data and the requirements of the application. Let's consider an example where we have a real-time chat application.
If the chat messages are received in real-time and need to be displayed as soon as they arrive, streaming would be the preferred approach. The messages can be streamed to the client as they are received, allowing for immediate display and interaction.
On the other hand, if the chat messages are received in batches or need to undergo further processing before being displayed, buffering would be a more suitable approach. The messages can be buffered in the client's state and displayed in batches for improved performance.
Related Article: Exploring Key Features of ReactJS
Additional Resources
- React Buffer Libraries